Applying Probability and Combinatorics in Coding Interviews
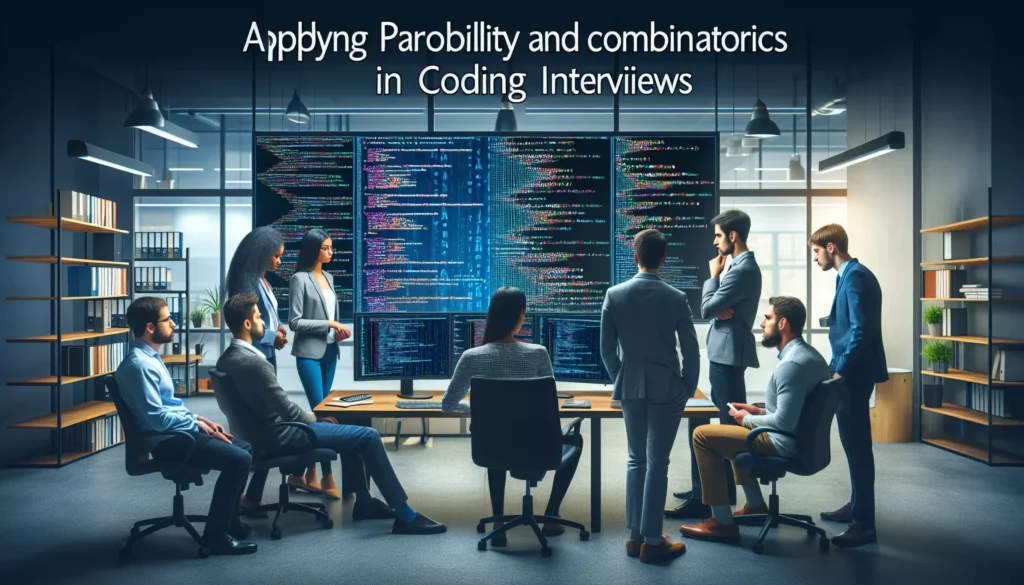
In the world of coding interviews, particularly those conducted by major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google), candidates are often challenged with problems that go beyond simple algorithmic implementations. These companies are looking for individuals who can think critically, solve complex problems, and apply mathematical concepts to real-world scenarios. Two areas that frequently appear in these interviews are probability and combinatorics. In this comprehensive guide, we’ll explore how these mathematical concepts are applied in coding interviews and provide strategies for tackling such problems.
Understanding the Importance of Probability and Combinatorics
Before diving into specific problems and solutions, it’s crucial to understand why probability and combinatorics are important in the context of coding interviews and software development in general:
- Data Analysis: Probability theory is fundamental in analyzing large datasets and making predictions based on historical data.
- Algorithm Design: Many efficient algorithms, especially in the realm of randomized algorithms, rely on probabilistic concepts.
- System Design: When designing large-scale systems, understanding probability helps in estimating system behavior and performance under various conditions.
- Optimization Problems: Combinatorics is often used in solving optimization problems, which are common in software engineering.
- Machine Learning: Both probability and combinatorics play crucial roles in machine learning algorithms and models.
Common Probability Concepts in Coding Interviews
Let’s explore some of the key probability concepts that often appear in coding interviews:
1. Basic Probability Rules
Understanding the fundamental rules of probability is essential. These include:
- The probability of an event is always between 0 and 1.
- The sum of probabilities of all possible outcomes is 1.
- For independent events A and B: P(A and B) = P(A) * P(B)
- For mutually exclusive events A and B: P(A or B) = P(A) + P(B)
2. Expected Value
The expected value is the average outcome of an experiment if it is repeated many times. It’s calculated by multiplying each possible outcome by its probability and summing these products.
def expected_value(outcomes, probabilities):
return sum(outcome * prob for outcome, prob in zip(outcomes, probabilities))
3. Conditional Probability
Conditional probability is the probability of an event occurring given that another event has already occurred. It’s often represented as P(A|B), read as “the probability of A given B”.
def conditional_probability(p_a_and_b, p_b):
return p_a_and_b / p_b if p_b != 0 else 0
4. Bayes’ Theorem
Bayes’ Theorem is used to calculate conditional probabilities and is particularly useful in machine learning and data analysis.
def bayes_theorem(p_a, p_b_given_a, p_b):
return (p_b_given_a * p_a) / p_b if p_b != 0 else 0
Common Combinatorics Concepts in Coding Interviews
Combinatorics problems often involve counting, arranging, or selecting objects. Here are some key concepts:
1. Permutations
Permutations are used when we need to arrange all elements of a set in a specific order. The number of permutations of n distinct objects is n!.
def permutations(n):
if n == 0:
return 1
return n * permutations(n - 1)
2. Combinations
Combinations are used when we need to select a subset of elements from a larger set, where order doesn’t matter. The number of ways to choose k items from n items is denoted as C(n,k) or (n choose k).
def combinations(n, k):
return permutations(n) // (permutations(k) * permutations(n - k))
3. Pigeonhole Principle
The pigeonhole principle states that if you have n items to put into m containers, and n > m, then at least one container must contain more than one item. This principle is often used in proving the existence of certain arrangements.
4. Inclusion-Exclusion Principle
This principle is used to calculate the number of elements in the union of multiple sets. It’s particularly useful when dealing with overlapping sets.
def inclusion_exclusion(set_a, set_b):
return len(set_a) + len(set_b) - len(set_a.intersection(set_b))
Applying Probability and Combinatorics in Coding Problems
Now that we’ve covered the basics, let’s look at some common types of problems you might encounter in coding interviews and how to approach them.
Problem 1: Shuffling an Array
Implement a function to shuffle an array randomly with equal probability for each permutation.
import random
def fisher_yates_shuffle(arr):
n = len(arr)
for i in range(n - 1, 0, -1):
j = random.randint(0, i)
arr[i], arr[j] = arr[j], arr[i]
return arr
This implementation uses the Fisher-Yates shuffle algorithm, which ensures that each permutation has an equal probability of 1/n! where n is the number of elements in the array.
Problem 2: Sampling from a Stream
Implement a function that can sample k items from a stream of unknown length with equal probability.
import random
def reservoir_sampling(stream, k):
reservoir = []
for i, item in enumerate(stream):
if i < k:
reservoir.append(item)
else:
j = random.randint(0, i)
if j < k:
reservoir[j] = item
return reservoir
This implementation uses the reservoir sampling algorithm, which ensures that each item in the stream has an equal probability of being selected, regardless of the stream’s length.
Problem 3: Calculating Probability of Winning a Game
Given a game where you have a p probability of winning each round, calculate the probability of winning exactly k out of n rounds.
def probability_of_winning(p, n, k):
return combinations(n, k) * (p ** k) * ((1 - p) ** (n - k))
This solution uses the binomial probability formula, combining concepts from both probability and combinatorics.
Problem 4: Estimating Pi using Monte Carlo Method
Implement a function to estimate the value of Pi using random number generation.
import random
def estimate_pi(num_points):
inside_circle = 0
total_points = num_points
for _ in range(total_points):
x = random.uniform(-1, 1)
y = random.uniform(-1, 1)
if x*x + y*y <= 1:
inside_circle += 1
return 4 * inside_circle / total_points
This method uses the concept of probability to estimate Pi. As the number of points increases, the estimate becomes more accurate.
Strategies for Solving Probability and Combinatorics Problems
When faced with a probability or combinatorics problem in a coding interview, consider the following strategies:
- Identify the Type of Problem: Is it a counting problem (combinatorics) or a probability problem? This will guide your approach.
- Break Down the Problem: Complex problems can often be broken down into simpler subproblems.
- Use Visualization: Drawing diagrams or using tree structures can help in understanding and solving the problem.
- Consider Edge Cases: Think about extreme scenarios and how they affect your solution.
- Use Simulation: For complex probability problems, consider using simulation techniques like Monte Carlo methods.
- Leverage Programming Concepts: Use appropriate data structures and algorithms to implement your solution efficiently.
- Practice, Practice, Practice: The more problems you solve, the better you’ll become at recognizing patterns and applying concepts.
Advanced Topics in Probability and Combinatorics
As you progress in your preparation for coding interviews, you may encounter more advanced topics. Here are a few to be aware of:
1. Markov Chains
Markov chains are mathematical systems that transition from one state to another according to certain probabilistic rules. They’re often used in modeling complex systems and in machine learning algorithms.
import numpy as np
def markov_chain(transition_matrix, initial_state, steps):
state = initial_state
for _ in range(steps):
state = np.random.choice(len(state), p=transition_matrix[state])
return state
2. Dynamic Programming with Probability
Many probability problems can be solved efficiently using dynamic programming techniques. This is particularly useful when dealing with problems that have overlapping subproblems.
3. Probability Distributions
Understanding various probability distributions (e.g., normal, Poisson, binomial) and when to apply them can be crucial in certain interview questions, especially those related to data analysis or machine learning.
4. Graph Theory and Probability
Some advanced problems combine concepts from graph theory and probability. For example, random walks on graphs or probabilistic analysis of graph algorithms.
Conclusion
Mastering probability and combinatorics for coding interviews is a journey that requires both theoretical understanding and practical application. By familiarizing yourself with the core concepts, practicing a variety of problems, and developing problem-solving strategies, you’ll be well-prepared to tackle these challenges in your interviews.
Remember, the goal is not just to solve specific problems, but to develop a problem-solving mindset that can be applied to a wide range of scenarios. As you prepare, focus on understanding the underlying principles and how they can be applied in different contexts.
Keep in mind that major tech companies like those in FAANG value candidates who can think critically and apply mathematical concepts to real-world problems. By honing your skills in probability and combinatorics, you’re not only preparing for interviews but also developing valuable skills that will serve you throughout your career in software development and beyond.
As you continue your journey with AlgoCademy, take advantage of the interactive coding tutorials, AI-powered assistance, and step-by-step guidance to reinforce these concepts and practice applying them in coding scenarios. With dedication and consistent practice, you’ll be well-equipped to tackle even the most challenging probability and combinatorics problems in your coding interviews.