Applying Mathematical Induction in Algorithm Design
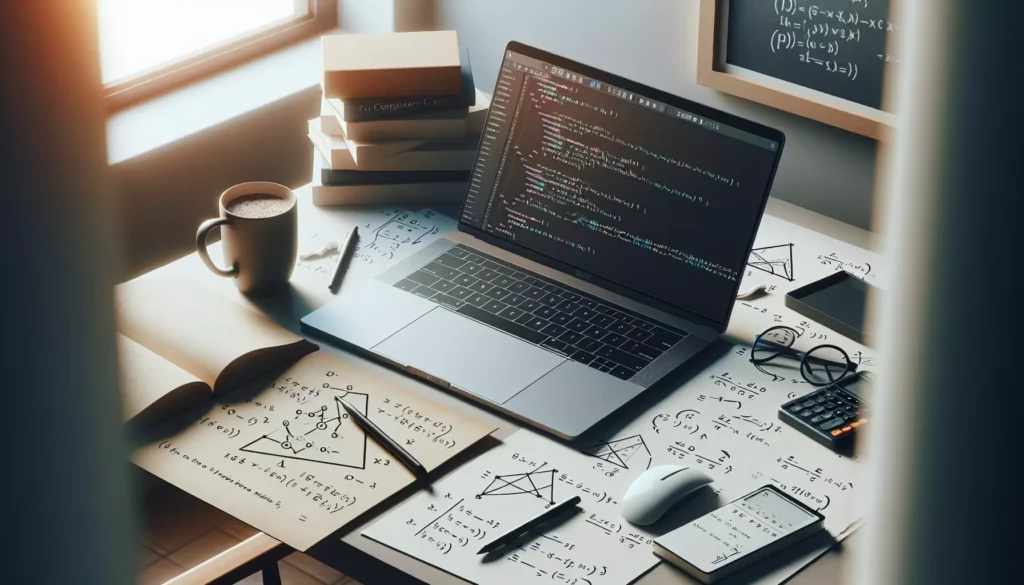
Mathematical induction is a powerful proof technique that plays a crucial role in algorithm design and analysis. In this comprehensive guide, we’ll explore how mathematical induction can be applied to develop, verify, and optimize algorithms. We’ll cover the fundamental principles of induction, its application in various algorithmic scenarios, and provide practical examples to illustrate its importance in computer science.
Understanding Mathematical Induction
Before diving into its applications in algorithm design, let’s review the basic concept of mathematical induction. Mathematical induction is a method of proving that a statement is true for all natural numbers. It consists of two main steps:
- Base case: Prove that the statement is true for the smallest value (usually n = 1 or n = 0).
- Inductive step: Assume the statement is true for some arbitrary value k, and then prove that it must be true for k + 1.
If both steps are proven, then the statement is considered true for all natural numbers greater than or equal to the base case.
Applying Induction in Algorithm Design
Mathematical induction is particularly useful in algorithm design for several reasons:
- Proving correctness of recursive algorithms
- Analyzing time and space complexity
- Verifying loop invariants
- Designing divide-and-conquer algorithms
- Optimizing dynamic programming solutions
Let’s explore each of these applications in detail.
1. Proving Correctness of Recursive Algorithms
Recursive algorithms are natural candidates for inductive proofs. The base case of the induction often corresponds to the base case of the recursion, while the inductive step aligns with the recursive call.
Example: Proving the correctness of a recursive factorial function
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n - 1)
To prove this algorithm is correct, we can use induction:
- Base case: For n = 0 and n = 1, the function correctly returns 1.
- Inductive step: Assume factorial(k) is correct for some k ≥ 1. We need to prove factorial(k + 1) is correct:
factorial(k + 1) = (k + 1) * factorial(k)
= (k + 1) * k! (by inductive hypothesis)
= (k + 1)!
Therefore, by induction, the factorial function is correct for all non-negative integers.
2. Analyzing Time and Space Complexity
Induction is often used to prove the time and space complexity of algorithms, especially those with recursive structures or iterative processes that grow with input size.
Example: Proving the time complexity of the Merge Sort algorithm
Claim: The time complexity of Merge Sort is O(n log n) for an input of size n.
Proof by induction:
- Base case: For n = 1, the algorithm takes constant time, which is O(1 * log 1) = O(0) = O(1).
- Inductive step: Assume the claim is true for all inputs of size k < n. For an input of size n, Merge Sort:
- Divides the input into two halves of size n/2
- Recursively sorts each half
- Merges the sorted halves in O(n) time
This gives us the recurrence relation:
T(n) = 2T(n/2) + O(n)
By the inductive hypothesis, T(n/2) = O((n/2) log(n/2))
Substituting:
T(n) = 2O((n/2) log(n/2)) + O(n)
= O(n log(n/2)) + O(n)
= O(n log n – n) + O(n)
= O(n log n)
Thus, by induction, we’ve proven that Merge Sort has a time complexity of O(n log n) for all input sizes.
3. Verifying Loop Invariants
Loop invariants are conditions that hold true before, during, and after each iteration of a loop. Induction can be used to prove that a loop invariant holds throughout the execution of an algorithm.
Example: Verifying the loop invariant for Binary Search
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
Loop invariant: If the target exists in the array, it is always within the range [left, right].
Proof by induction:
- Base case: Before the first iteration, left = 0 and right = len(arr) – 1, so the entire array is covered.
- Inductive step: Assume the invariant holds before an iteration. We need to prove it holds after the iteration:
- If arr[mid] == target, we’ve found the element, and the invariant holds.
- If arr[mid] < target, we know the target must be in the right half, so setting left = mid + 1 maintains the invariant.
- If arr[mid] > target, we know the target must be in the left half, so setting right = mid – 1 maintains the invariant.
Therefore, by induction, the loop invariant holds throughout the execution of the binary search algorithm.
4. Designing Divide-and-Conquer Algorithms
Divide-and-conquer algorithms naturally lend themselves to inductive reasoning. The division step reduces the problem size, while the conquering step combines solutions, mirroring the structure of an inductive proof.
Example: Designing a divide-and-conquer algorithm for the maximum subarray problem
Problem: Given an array of integers, find the contiguous subarray with the largest sum.
Algorithm idea:
- Divide the array into two halves.
- Recursively find the maximum subarray in each half.
- Find the maximum subarray that crosses the midpoint.
- Return the maximum of the three subarrays found.
def max_crossing_subarray(arr, low, mid, high):
left_sum = float('-inf')
sum = 0
for i in range(mid, low - 1, -1):
sum += arr[i]
if sum > left_sum:
left_sum = sum
right_sum = float('-inf')
sum = 0
for i in range(mid + 1, high + 1):
sum += arr[i]
if sum > right_sum:
right_sum = sum
return left_sum + right_sum
def max_subarray(arr, low, high):
if low == high:
return arr[low]
mid = (low + high) // 2
left_sum = max_subarray(arr, low, mid)
right_sum = max_subarray(arr, mid + 1, high)
cross_sum = max_crossing_subarray(arr, low, mid, high)
return max(left_sum, right_sum, cross_sum)
The correctness of this algorithm can be proven using induction:
- Base case: For an array of size 1, the algorithm correctly returns the single element.
- Inductive step: Assume the algorithm works correctly for all arrays of size k < n. For an array of size n:
- The algorithm correctly finds the maximum subarray in each half (by the inductive hypothesis).
- It correctly finds the maximum crossing subarray.
- By returning the maximum of these three, it must find the overall maximum subarray.
Thus, by induction, the algorithm correctly solves the maximum subarray problem for all array sizes.
5. Optimizing Dynamic Programming Solutions
Dynamic programming often involves proving that a recursive formula correctly solves a problem for all input sizes. Induction is a natural fit for verifying these formulas.
Example: Optimizing the Fibonacci sequence calculation
Consider the following dynamic programming solution for calculating the nth Fibonacci number:
def fibonacci(n):
if n <= 1:
return n
fib = [0] * (n + 1)
fib[1] = 1
for i in range(2, n + 1):
fib[i] = fib[i-1] + fib[i-2]
return fib[n]
To prove this algorithm correctly computes Fibonacci numbers, we can use induction:
- Base cases: fib[0] = 0 and fib[1] = 1 are correct by definition.
- Inductive step: Assume fib[k] is correct for all k < i. We need to prove fib[i] is correct:
fib[i] = fib[i-1] + fib[i-2]
This is the definition of the Fibonacci sequence, so if fib[i-1] and fib[i-2] are correct, fib[i] must be correct.
Therefore, by induction, the algorithm correctly computes all Fibonacci numbers.
Advanced Applications of Induction in Algorithms
Beyond these fundamental applications, mathematical induction can be used in more advanced algorithmic techniques and analysis:
1. Amortized Analysis
Amortized analysis is a method for analyzing the time complexity of algorithms that perform a sequence of operations. Induction can be used to prove that the amortized cost of an operation is bounded over any sequence of operations.
Example: Analyzing the amortized cost of dynamic array resizing
Consider a dynamic array that doubles in size when it’s full. We can use the potential method of amortized analysis:
- Define the potential Φ(i) as the number of elements in the array after the ith operation.
- The amortized cost of an insertion is the actual cost plus the change in potential.
- Use induction to prove that the amortized cost of each insertion is O(1).
2. Proving Correctness of Greedy Algorithms
Greedy algorithms make locally optimal choices at each step. Induction can be used to prove that these local choices lead to a globally optimal solution.
Example: Proving the correctness of Kruskal’s algorithm for Minimum Spanning Trees
Induction can be used to prove that at each step, the edges selected by Kruskal’s algorithm are part of some minimum spanning tree.
3. Analyzing Randomized Algorithms
For randomized algorithms, induction can be used to prove expected running times or probability bounds.
Example: Analyzing the expected number of comparisons in Quicksort
Induction can be used to prove that the expected number of comparisons in Quicksort is O(n log n).
Common Pitfalls and Best Practices
When applying mathematical induction in algorithm design and analysis, it’s important to be aware of common pitfalls and follow best practices:
Pitfalls:
- Incorrect base case: Ensure that the base case(s) are correctly identified and proven.
- Weak induction hypothesis: Make sure the induction hypothesis is strong enough to prove the next case.
- Circular reasoning: Avoid using the statement you’re trying to prove in your proof.
- Overlooking edge cases: Consider all possible scenarios, including boundary conditions.
Best Practices:
- Start with a clear statement: Clearly state what you’re trying to prove before beginning the induction.
- Use strong induction when necessary: Sometimes, assuming the statement is true for all values less than k (instead of just k) can make the proof easier.
- Consider multiple base cases: Some problems may require proving multiple base cases before the induction can proceed.
- Combine with other proof techniques: Induction can often be combined with other methods like contradiction or the pigeonhole principle for more complex proofs.
Conclusion
Mathematical induction is an indispensable tool in the algorithm designer’s toolkit. Its applications range from proving the correctness of simple recursive algorithms to analyzing complex data structures and optimizing dynamic programming solutions. By mastering the use of induction in algorithm design and analysis, you’ll be better equipped to develop efficient, correct algorithms and rigorously analyze their performance.
As you continue your journey in algorithm design, remember that induction is not just a theoretical concept—it’s a practical tool that can guide your thinking and help you solve real-world computational problems. Practice applying induction to various algorithmic scenarios, and you’ll find it becomes an intuitive part of your problem-solving process.
Whether you’re preparing for technical interviews, developing software, or pursuing advanced studies in computer science, a solid understanding of mathematical induction and its applications in algorithms will serve you well. Keep exploring, keep practicing, and don’t hesitate to apply inductive reasoning to new and challenging problems you encounter in your coding journey.