Ant Colony Optimization: Nature-Inspired Algorithm for Solving Complex Problems
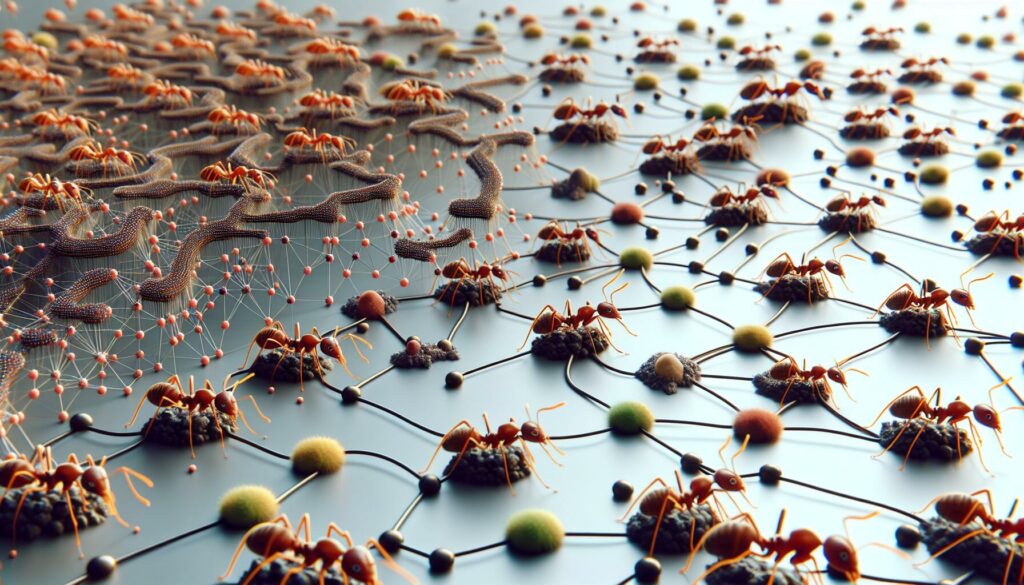
In the vast world of algorithms and computational problem-solving, nature has often been a source of inspiration. One such fascinating example is Ant Colony Optimization (ACO), an algorithm that mimics the behavior of ants to solve complex optimization problems. In this comprehensive guide, we’ll explore the intricacies of ACO, its applications, and how it relates to the broader field of coding education and algorithmic thinking.
What is Ant Colony Optimization?
Ant Colony Optimization is a probabilistic technique for solving computational problems that can be reduced to finding good paths through graphs. Inspired by the foraging behavior of ants, ACO was first introduced by Marco Dorigo in his PhD thesis in 1992. The algorithm has since gained significant attention in the field of artificial intelligence and optimization.
The core idea behind ACO is to mimic the way ants find the shortest path between their colony and a food source. In nature, ants initially explore the area around their nest randomly. Upon finding food, they return to the colony, laying down pheromone trails. If other ants find such a path, they are likely to follow it, reinforcing the trail if they also find food. Over time, the shortest path emerges as the one with the strongest pheromone concentration.
How Does Ant Colony Optimization Work?
The ACO algorithm translates this natural behavior into a computational method for solving problems. Here’s a step-by-step breakdown of how it works:
- Initialization: Set up the problem as a graph with nodes and edges.
- Ant Distribution: Place artificial ants randomly on the graph nodes.
- Solution Construction: Each ant constructs a solution by moving from node to node.
- Pheromone Update: After all ants complete their tours, update pheromone levels on the edges.
- Iteration: Repeat steps 2-4 for a set number of iterations or until a satisfactory solution is found.
The movement of ants is guided by two factors:
- Pheromone trails: Edges with higher pheromone levels are more likely to be chosen.
- Heuristic information: Problem-specific information that guides ants towards promising solutions.
Implementing Ant Colony Optimization
Let’s look at a basic implementation of ACO in Python to solve the Traveling Salesman Problem (TSP), a classic optimization challenge:
import numpy as np
class AntColonyOptimizer:
def __init__(self, distances, n_ants, n_iterations, alpha, beta, evaporation_rate, Q):
self.distances = distances
self.n_ants = n_ants
self.n_iterations = n_iterations
self.alpha = alpha # pheromone importance
self.beta = beta # distance importance
self.evaporation_rate = evaporation_rate
self.Q = Q # pheromone deposit factor
self.n_cities = len(distances)
self.pheromones = np.ones((self.n_cities, self.n_cities))
def run(self):
best_path = None
best_path_length = float('inf')
for iteration in range(self.n_iterations):
paths = self.construct_solutions()
self.update_pheromones(paths)
iteration_best_path = min(paths, key=lambda x: self.path_length(x))
iteration_best_path_length = self.path_length(iteration_best_path)
if iteration_best_path_length < best_path_length:
best_path = iteration_best_path
best_path_length = iteration_best_path_length
return best_path, best_path_length
def construct_solutions(self):
paths = []
for ant in range(self.n_ants):
path = self.construct_path()
paths.append(path)
return paths
def construct_path(self):
path = [np.random.randint(self.n_cities)]
while len(path) < self.n_cities:
next_city = self.choose_next_city(path)
path.append(next_city)
return path
def choose_next_city(self, path):
current_city = path[-1]
unvisited_cities = list(set(range(self.n_cities)) - set(path))
probabilities = self.calculate_probabilities(current_city, unvisited_cities)
return np.random.choice(unvisited_cities, p=probabilities)
def calculate_probabilities(self, current_city, unvisited_cities):
pheromone_values = np.array([self.pheromones[current_city][j] for j in unvisited_cities])
distance_values = np.array([1 / self.distances[current_city][j] for j in unvisited_cities])
probabilities = (pheromone_values ** self.alpha) * (distance_values ** self.beta)
return probabilities / sum(probabilities)
def update_pheromones(self, paths):
self.pheromones *= (1 - self.evaporation_rate)
for path in paths:
path_length = self.path_length(path)
for i in range(self.n_cities):
start, end = path[i], path[(i + 1) % self.n_cities]
self.pheromones[start][end] += self.Q / path_length
def path_length(self, path):
return sum(self.distances[path[i]][path[(i + 1) % self.n_cities]] for i in range(self.n_cities))
# Example usage
distances = np.array([
[0, 2, 3, 4],
[2, 0, 5, 6],
[3, 5, 0, 1],
[4, 6, 1, 0]
])
aco = AntColonyOptimizer(distances, n_ants=10, n_iterations=100, alpha=1, beta=2, evaporation_rate=0.1, Q=1)
best_path, best_path_length = aco.run()
print(f"Best path: {best_path}")
print(f"Best path length: {best_path_length}")
This implementation demonstrates the core concepts of ACO, including pheromone deposition, evaporation, and probabilistic decision-making. It’s a simplified version, but it captures the essence of how ACO works in practice.
Applications of Ant Colony Optimization
ACO has found applications in various fields, showcasing its versatility in solving complex optimization problems:
- Routing Problems: Besides the Traveling Salesman Problem, ACO is used in vehicle routing, network routing, and logistics optimization.
- Scheduling: Job shop scheduling, project scheduling, and timetabling problems benefit from ACO’s ability to find near-optimal solutions.
- Image Processing: ACO has been applied to edge detection and image segmentation tasks.
- Bioinformatics: Protein folding and DNA sequencing problems have been approached using ACO techniques.
- Network Security: Intrusion detection systems and network traffic optimization utilize ACO algorithms.
Advantages and Limitations of Ant Colony Optimization
Like any algorithm, ACO comes with its own set of strengths and weaknesses:
Advantages:
- Inherent parallelism: Multiple artificial ants can search for solutions simultaneously.
- Adaptability: ACO can adapt to changes in the environment, making it suitable for dynamic problems.
- Positive feedback: The pheromone system leads to the rapid discovery of good solutions.
- Versatility: ACO can be applied to a wide range of optimization problems.
Limitations:
- Theoretical analysis: The theoretical analysis of convergence time is difficult.
- Time to convergence: ACO algorithms can be slower compared to other optimization techniques for certain problems.
- Parameter tuning: The performance of ACO is sensitive to parameter settings, which may require careful tuning.
ACO in the Context of Coding Education and AlgoCademy
Understanding and implementing algorithms like Ant Colony Optimization is crucial for developing strong problem-solving skills in computer science. In the context of coding education platforms like AlgoCademy, ACO serves as an excellent example of how nature-inspired algorithms can solve complex computational problems.
Here’s how ACO aligns with the goals of coding education:
- Algorithmic Thinking: ACO encourages students to think about problem-solving in terms of graph traversal, probabilistic decision-making, and iterative improvement.
- Optimization Concepts: It introduces important optimization concepts like local vs. global optima, exploration vs. exploitation, and the importance of heuristics.
- Real-world Applications: ACO’s applications in various fields demonstrate how theoretical computer science concepts translate to practical problem-solving.
- Interdisciplinary Connections: The algorithm’s inspiration from biology highlights the interdisciplinary nature of computer science.
- Programming Practice: Implementing ACO provides practice in working with graphs, probability, and iterative algorithms.
Integrating ACO into Coding Education
For platforms like AlgoCademy, incorporating ACO into the curriculum could involve:
- Interactive Tutorials: Step-by-step guides on implementing ACO, starting with simple problems and progressing to more complex applications.
- Visualization Tools: Interactive visualizations of ants traversing graphs, helping students understand how pheromone trails influence path selection.
- Coding Challenges: Problems that can be solved efficiently using ACO, encouraging students to implement and optimize the algorithm.
- Comparative Analysis: Assignments comparing ACO with other optimization algorithms, helping students understand the strengths and weaknesses of different approaches.
- Real-world Case Studies: Examining how companies like Amazon or Google might use ACO-like algorithms for logistics or network optimization.
Advanced Topics in Ant Colony Optimization
As students progress in their understanding of ACO, several advanced topics can be explored:
1. Hybrid Algorithms
Combining ACO with other optimization techniques like genetic algorithms or local search methods can lead to more powerful hybrid algorithms. These hybrids often outperform pure ACO in certain problem domains.
2. Multi-objective Optimization
Extending ACO to handle multiple, often conflicting objectives is an active area of research. This involves modifying the algorithm to maintain a set of non-dominated solutions (Pareto front) rather than a single best solution.
3. Parallel and Distributed ACO
Implementing ACO on parallel or distributed computing systems can significantly speed up the optimization process, especially for large-scale problems. This topic introduces concepts of parallel computing and algorithm design.
4. Continuous Optimization with ACO
While ACO was originally designed for discrete optimization problems, variations have been developed for continuous optimization. This extension broadens the applicability of ACO to a wider range of real-world problems.
5. Theoretical Foundations
Delving into the mathematical foundations of ACO, including convergence proofs and complexity analysis, provides a deeper understanding of the algorithm’s behavior and limitations.
Preparing for Technical Interviews with ACO Knowledge
Understanding ACO can be valuable for technical interviews, especially for positions involving optimization, artificial intelligence, or algorithm design. Here are some ways ACO knowledge can be applied in interview scenarios:
- Problem-solving Approach: Demonstrating knowledge of ACO showcases an ability to approach complex problems with innovative, nature-inspired solutions.
- Algorithm Design: ACO principles can be adapted to design custom algorithms for specific optimization problems presented in interviews.
- Complexity Analysis: Discussing the time and space complexity of ACO implementations demonstrates an understanding of algorithm efficiency.
- Trade-off Discussions: ACO provides a good basis for discussing trade-offs between solution quality and computational time, a common theme in technical interviews.
- System Design: For more advanced positions, knowledge of how ACO can be applied to large-scale systems (e.g., in logistics or networking) can be valuable.
Conclusion
Ant Colony Optimization stands as a testament to the power of nature-inspired computing. Its ability to solve complex optimization problems by mimicking the simple behavior of ants is both elegant and effective. For students and professionals in computer science, understanding ACO offers insights into advanced algorithm design, optimization techniques, and the broader field of artificial intelligence.
As coding education platforms like AlgoCademy continue to evolve, incorporating algorithms like ACO into their curricula provides students with valuable tools for problem-solving and algorithmic thinking. Whether you’re a beginner learning the basics of programming or an experienced developer preparing for technical interviews at top tech companies, the principles behind ACO offer valuable lessons in computational problem-solving.
By studying and implementing ACO, learners not only gain practical coding skills but also develop a deeper appreciation for the interdisciplinary nature of computer science. As we continue to face increasingly complex computational challenges, algorithms inspired by nature, like ACO, will undoubtedly play a crucial role in shaping the future of technology and problem-solving.