Anatomy of a Coding Interview: What to Do at Each Step
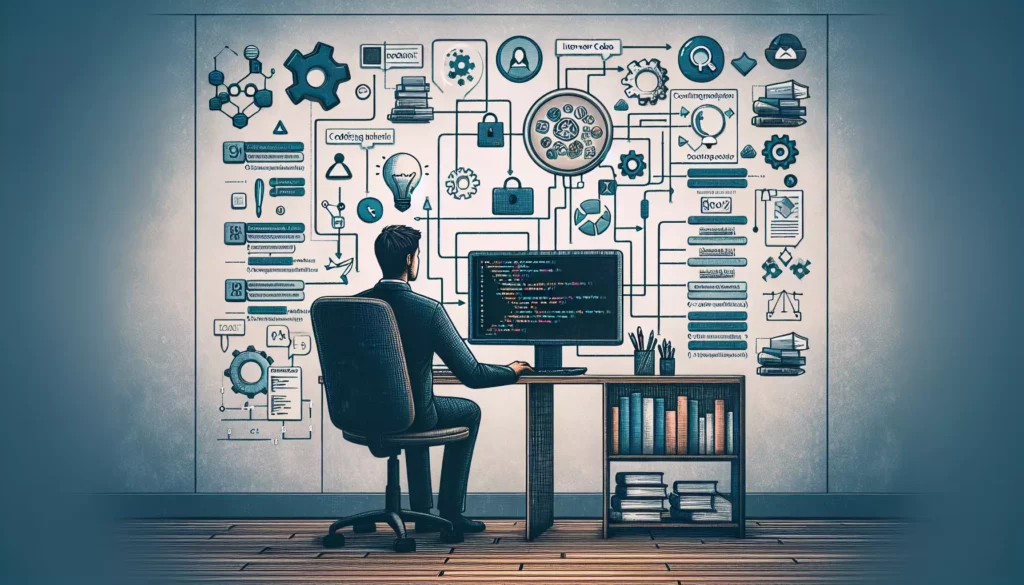
Coding interviews can be intimidating, especially for those new to the tech industry or aspiring developers looking to land their dream job. Whether you’re aiming for a position at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or any other tech firm, understanding the structure of a coding interview and knowing how to navigate each step is crucial for success. In this comprehensive guide, we’ll break down the anatomy of a typical coding interview and provide you with actionable strategies for each stage of the process.
1. Introduction and Icebreaker
The first few minutes of your coding interview are critical for setting the tone and establishing rapport with your interviewer. Here’s what you should focus on during this phase:
What to expect:
- Brief introductions
- Small talk or icebreaker questions
- Overview of the interview structure
What to do:
- Be friendly and professional
- Prepare a concise self-introduction
- Show enthusiasm for the role and company
- Ask clarifying questions about the interview process if needed
Remember, your interviewer is not just assessing your technical skills but also evaluating how well you might fit into the team. A positive first impression can set the stage for a successful interview.
2. Problem Presentation
After the introduction, your interviewer will present you with a coding problem. This is where the real challenge begins.
What to expect:
- A clear problem statement
- Possible constraints or requirements
- Sample input and expected output
What to do:
- Listen carefully and take notes
- Ask clarifying questions to ensure you understand the problem fully
- Repeat the problem back to the interviewer to confirm your understanding
- Inquire about any assumptions you can make
Example clarifying questions:
- “Can I assume the input will always be valid?”
- “What should I do if the input is empty or null?”
- “Are there any time or space complexity requirements?”
Taking the time to fully understand the problem can save you from heading down the wrong path later in the interview.
3. Problem-Solving and Brainstorming
Before diving into coding, it’s crucial to think through your approach and discuss potential solutions with your interviewer.
What to expect:
- Time to think and brainstorm
- Encouragement to share your thought process
- Possible hints or guidance from the interviewer
What to do:
- Think out loud and explain your reasoning
- Consider multiple approaches to solving the problem
- Discuss trade-offs between different solutions
- Use examples to illustrate your ideas
- Draw diagrams or flowcharts if it helps explain your thought process
During this phase, don’t be afraid to start with a brute-force solution and then work towards optimizing it. Interviewers often want to see how you approach problem-solving, not just the final solution.
4. Outlining the Solution
Before you start coding, it’s beneficial to outline your proposed solution. This step helps organize your thoughts and allows the interviewer to provide feedback early on.
What to expect:
- Time to structure your approach
- Feedback or suggestions from the interviewer
What to do:
- Sketch out the high-level steps of your algorithm
- Discuss the data structures you plan to use
- Estimate the time and space complexity of your solution
- Ask for feedback on your proposed approach
Here’s an example of how you might outline a solution for a problem that asks you to find the two numbers in an array that sum up to a target value:
1. Create a hash map to store complements
2. Iterate through the array
3. For each number, calculate its complement (target - number)
4. Check if the complement exists in the hash map
- If yes, return the current number and its complement
- If no, add the current number to the hash map
5. If no solution is found, return an appropriate result (e.g., empty array or null)
By outlining your solution, you demonstrate your ability to plan and structure your code before implementation.
5. Coding the Solution
Now comes the part where you actually write the code. This is your chance to showcase your programming skills and attention to detail.
What to expect:
- A coding environment (whiteboard, shared document, or IDE)
- Time pressure to complete the implementation
- Potential questions or interruptions from the interviewer
What to do:
- Write clean, readable code
- Use meaningful variable and function names
- Comment your code where necessary
- Explain your implementation as you code
- Handle edge cases and error conditions
Here’s an example of how you might implement the two-sum problem in Python, following the outline we created earlier:
def two_sum(nums, target):
complement_map = {} # Hash map to store complements
for i, num in enumerate(nums):
complement = target - num
if complement in complement_map:
return [complement_map[complement], i]
complement_map[num] = i
return None # No solution found
# Example usage
nums = [2, 7, 11, 15]
target = 9
result = two_sum(nums, target)
print(result) # Output: [0, 1]
Remember to vocalize your thought process as you code. This helps the interviewer understand your reasoning and can also earn you partial credit if you don’t complete the entire solution.
6. Testing and Debugging
After implementing your solution, it’s crucial to test and debug your code. This demonstrates your attention to detail and your ability to ensure code quality.
What to expect:
- Time to review and test your code
- Possible prompts from the interviewer to consider specific test cases
What to do:
- Walk through your code step-by-step with a simple example
- Test with the provided sample input
- Consider edge cases and test them (e.g., empty input, single element, duplicate values)
- Identify and fix any bugs or logical errors
- Optimize your code if time allows
Let’s continue with our two-sum example and add some test cases:
# Test cases
print(two_sum([2, 7, 11, 15], 9)) # Expected: [0, 1]
print(two_sum([3, 2, 4], 6)) # Expected: [1, 2]
print(two_sum([3, 3], 6)) # Expected: [0, 1]
print(two_sum([1, 2, 3, 4], 10)) # Expected: None
print(two_sum([], 0)) # Expected: None
print(two_sum([1], 1)) # Expected: None
By thoroughly testing your code, you demonstrate your commitment to producing reliable and robust solutions.
7. Time and Space Complexity Analysis
Understanding and articulating the efficiency of your solution is a critical skill that interviewers look for, especially in more advanced positions.
What to expect:
- Questions about the time and space complexity of your solution
- Discussions on potential optimizations
What to do:
- Analyze the time complexity of your algorithm (e.g., O(n), O(n log n), O(n^2))
- Discuss the space complexity and any trade-offs made
- Explain how your solution scales with larger inputs
- Propose potential optimizations or alternative approaches
For our two-sum example, we could provide the following analysis:
“The time complexity of this solution is O(n), where n is the number of elements in the input array. We iterate through the array once, and hash map operations (insertion and lookup) are generally O(1) on average. The space complexity is also O(n) in the worst case, as we might need to store all elements in the hash map before finding a solution.”
8. Follow-up Questions and Variations
Many interviewers will present follow-up questions or variations of the original problem to test your adaptability and deeper understanding.
What to expect:
- Modifications to the original problem
- Questions about handling larger datasets or different constraints
- Discussions on real-world applications of the problem
What to do:
- Listen carefully to the new requirements or constraints
- Discuss how these changes would affect your current solution
- Propose modifications or entirely new approaches if necessary
- Consider trade-offs between different solutions
For the two-sum problem, a follow-up question might be:
“How would you modify your solution if the input array was sorted? Could you achieve a better space complexity?”
In this case, you could discuss a two-pointer approach that would have O(n) time complexity and O(1) space complexity:
def two_sum_sorted(nums, target):
left, right = 0, len(nums) - 1
while left < right:
current_sum = nums[left] + nums[right]
if current_sum == target:
return [left, right]
elif current_sum < target:
left += 1
else:
right -= 1
return None # No solution found
9. Questions for the Interviewer
At the end of the interview, you’ll usually have the opportunity to ask questions. This is your chance to demonstrate your interest in the role and the company.
What to expect:
- Time allocated for your questions
- Openness to discuss various aspects of the role and company
What to do:
- Prepare thoughtful questions in advance
- Ask about the team structure and day-to-day responsibilities
- Inquire about growth opportunities and company culture
- Show genuine interest in the interviewer’s experience at the company
Example questions you might ask:
- “What does a typical day look like for someone in this role?”
- “How does the team approach continuous learning and professional development?”
- “Can you tell me about a recent project the team has worked on that you found particularly exciting?”
- “What are the biggest challenges facing the team or department right now?”
10. Closing the Interview
As the interview comes to an end, it’s important to leave a positive final impression.
What to expect:
- A brief wrap-up from the interviewer
- Information about next steps in the hiring process
What to do:
- Thank the interviewer for their time
- Reiterate your interest in the position
- Ask about the timeline for next steps
- Request the interviewer’s contact information for follow-up if appropriate
A good closing statement might be:
“Thank you so much for taking the time to meet with me today. I really enjoyed our discussion and learning more about the role. I’m very excited about the possibility of joining your team and contributing to [specific project or company goal]. Could you tell me what the next steps in the process might be?”
Conclusion
Mastering the anatomy of a coding interview takes practice and preparation. By understanding what to expect at each stage and knowing how to navigate through them effectively, you can significantly improve your performance and increase your chances of landing that dream job in tech.
Remember, the key to success in coding interviews is not just about solving the problem, but also about demonstrating your problem-solving process, communication skills, and ability to work through challenges. Practice regularly, stay calm under pressure, and always be ready to explain your thought process.
As you prepare for your coding interviews, consider using resources like AlgoCademy to hone your skills. With interactive coding tutorials, AI-powered assistance, and a focus on algorithmic thinking and problem-solving, platforms like AlgoCademy can help you build the confidence and competence needed to excel in technical interviews.
Good luck with your upcoming interviews, and remember that each interview, regardless of the outcome, is an opportunity to learn and improve. Happy coding!