Analyzing Time vs. Space Trade-offs in Code: Mastering Efficient Programming
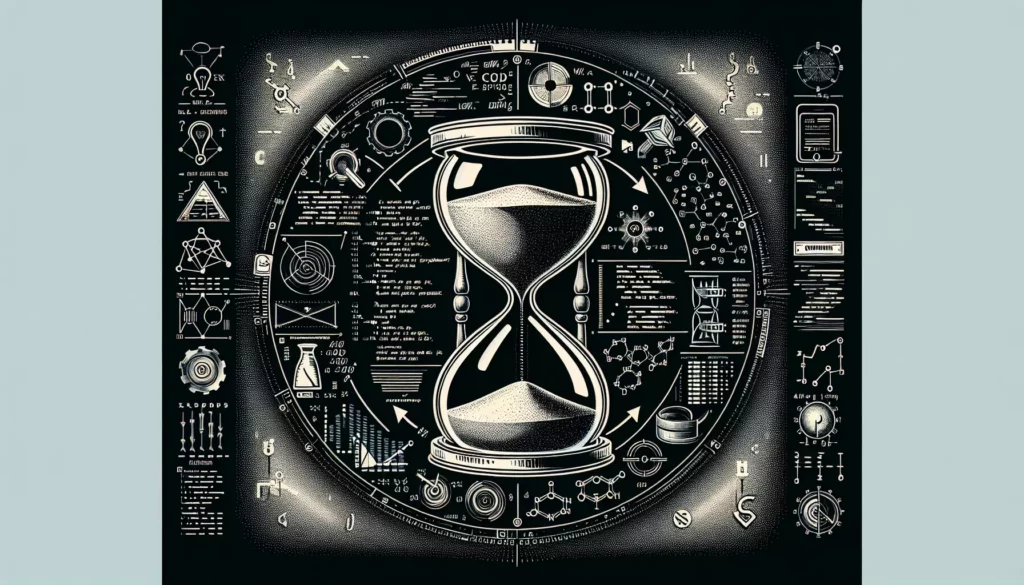
In the world of programming and algorithm design, one of the most crucial skills developers need to master is the ability to analyze and optimize their code for both time and space efficiency. This balancing act between time complexity and space complexity is often referred to as the “time-space trade-off.” Understanding and effectively managing these trade-offs can significantly impact the performance and scalability of your software applications.
In this comprehensive guide, we’ll dive deep into the concept of time vs. space trade-offs, explore various scenarios where these trade-offs come into play, and provide practical strategies for optimizing your code. Whether you’re a beginner programmer or preparing for technical interviews at top tech companies, this knowledge will prove invaluable in your journey to becoming a more efficient and effective developer.
Understanding Time Complexity and Space Complexity
Before we delve into the intricacies of time-space trade-offs, it’s essential to have a solid grasp of time complexity and space complexity:
Time Complexity
Time complexity refers to the amount of time an algorithm takes to complete as a function of the input size. It’s typically expressed using Big O notation, which describes the upper bound of the growth rate of an algorithm’s running time. Common time complexities include:
- O(1) – Constant time
- O(log n) – Logarithmic time
- O(n) – Linear time
- O(n log n) – Linearithmic time
- O(n^2) – Quadratic time
- O(2^n) – Exponential time
Space Complexity
Space complexity, on the other hand, refers to the amount of memory an algorithm uses as a function of the input size. Like time complexity, it’s also expressed using Big O notation. Common space complexities include:
- O(1) – Constant space
- O(n) – Linear space
- O(n^2) – Quadratic space
Now that we have a basic understanding of time and space complexity, let’s explore how they interact and the trade-offs we often encounter in programming.
The Time-Space Trade-off Paradigm
The time-space trade-off is a fundamental concept in computer science and algorithm design. It refers to the relationship between the time complexity and space complexity of an algorithm. Often, we can improve the time complexity of an algorithm by using more memory (increasing space complexity), or we can reduce the space complexity at the cost of increased running time.
This trade-off is not always a straightforward one-to-one relationship. Sometimes, a small increase in space usage can lead to a significant improvement in time complexity, while in other cases, a large amount of additional memory might only yield a modest speedup.
Let’s examine some common scenarios where time-space trade-offs come into play:
1. Memoization and Dynamic Programming
Memoization is a technique used to optimize recursive algorithms by storing the results of expensive function calls and returning the cached result when the same inputs occur again. This is a classic example of trading space for time.
Consider the following naive implementation of the Fibonacci sequence:
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
This implementation has a time complexity of O(2^n), which is extremely inefficient for large values of n. However, we can improve it using memoization:
def fibonacci_memoized(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_memoized(n-1, memo) + fibonacci_memoized(n-2, memo)
return memo[n]
The memoized version has a time complexity of O(n) and a space complexity of O(n). We’ve traded additional space (to store the memo dictionary) for a significant improvement in time complexity.
2. Hash Tables for Fast Lookup
Hash tables are a prime example of trading space for time. By using additional memory to store key-value pairs, we can achieve constant-time O(1) average-case lookup, insertion, and deletion operations.
Consider the problem of finding two numbers in an array that sum up to a target value. A naive approach would involve nested loops, resulting in O(n^2) time complexity:
def two_sum_naive(nums, target):
for i in range(len(nums)):
for j in range(i+1, len(nums)):
if nums[i] + nums[j] == target:
return [i, j]
return []
Using a hash table, we can optimize this to O(n) time complexity:
def two_sum_optimized(nums, target):
num_map = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_map:
return [num_map[complement], i]
num_map[num] = i
return []
In this optimized version, we’ve increased the space complexity to O(n) by using a hash table, but we’ve reduced the time complexity from O(n^2) to O(n).
3. Precomputation and Lookup Tables
Precomputation involves calculating and storing results in advance to speed up future operations. This technique is particularly useful when you have a fixed set of inputs and need to perform frequent lookups.
For example, consider a function that needs to calculate the factorial of numbers from 1 to 20 multiple times:
def factorial(n):
if n == 0 or n == 1:
return 1
return n * factorial(n-1)
# Without precomputation
result = factorial(15) # Calculated every time it's needed
We can improve performance by precomputing the factorials:
factorials = [1] # 0! = 1
for i in range(1, 21):
factorials.append(factorials[-1] * i)
# With precomputation
result = factorials[15] # O(1) lookup
Here, we’ve increased the space complexity by storing the precomputed values, but we’ve reduced the time complexity of subsequent lookups to O(1).
Analyzing Trade-offs: When to Optimize for Time or Space
When faced with a time-space trade-off, how do you decide whether to optimize for time or space? Here are some factors to consider:
1. Problem Constraints
Always start by carefully analyzing the problem constraints. Some questions to ask include:
- What are the input size limits?
- Are there any time or memory constraints specified?
- How frequently will the algorithm be executed?
For example, if you’re working on a problem with strict memory limitations (e.g., embedded systems or mobile devices), you might need to prioritize space efficiency over time efficiency.
2. Scalability Requirements
Consider the long-term scalability of your solution. Will the input size grow significantly over time? If so, prioritizing time efficiency might be more important, even if it comes at the cost of increased space usage.
3. Frequency of Operations
Analyze how often different operations will be performed. If certain operations are executed frequently, it might be worth trading space for time to optimize these operations.
4. Hardware Constraints
Take into account the hardware environment where your code will run. Modern systems often have abundant memory but may be constrained by CPU performance. In such cases, trading space for time might be more beneficial.
5. Maintainability and Readability
Sometimes, a more space-efficient algorithm might be significantly more complex and harder to maintain. Consider the trade-off between optimization and code readability, especially for long-term projects.
Practical Strategies for Optimizing Time-Space Trade-offs
Now that we understand the concept of time-space trade-offs and factors to consider, let’s explore some practical strategies for optimizing your code:
1. Use Appropriate Data Structures
Choosing the right data structure can significantly impact both time and space efficiency. For example:
- Use hash tables for fast lookup when memory isn’t a constraint.
- Consider using a binary search tree instead of an array for ordered data with frequent insertions and deletions.
- Use a heap for maintaining a dynamic set of elements with priority.
2. Implement Lazy Evaluation
Lazy evaluation involves delaying the computation of a value until it’s actually needed. This can save both time and space by avoiding unnecessary calculations. Python’s generators are a great example of lazy evaluation:
def fibonacci_generator():
a, b = 0, 1
while True:
yield a
a, b = b, a + b
# Generate Fibonacci numbers on-demand
fib = fibonacci_generator()
print(next(fib)) # 0
print(next(fib)) # 1
print(next(fib)) # 1
print(next(fib)) # 2
3. Use Dynamic Programming
Dynamic programming can often provide an excellent balance between time and space efficiency. By breaking down complex problems into simpler subproblems and storing their results, you can avoid redundant computations while keeping space usage reasonable.
4. Optimize Algorithms
Always look for opportunities to optimize your algorithms. Some techniques include:
- Eliminating unnecessary operations or loop iterations
- Using more efficient sorting or searching algorithms when applicable
- Applying mathematical optimizations or formulas to reduce computation
5. Consider In-place Algorithms
In-place algorithms modify the input data structure directly, without using additional data structures. These algorithms can be highly space-efficient but may sacrifice some readability or increase time complexity. For example, in-place array reversal:
def reverse_array_in_place(arr):
left, right = 0, len(arr) - 1
while left < right:
arr[left], arr[right] = arr[right], arr[left]
left += 1
right -= 1
return arr
# Usage
my_array = [1, 2, 3, 4, 5]
reverse_array_in_place(my_array)
print(my_array) # [5, 4, 3, 2, 1]
6. Profile and Benchmark Your Code
Use profiling tools to identify performance bottlenecks in your code. This can help you focus your optimization efforts on the parts of your program that will yield the most significant improvements.
Real-world Examples of Time-Space Trade-offs
Let’s examine some real-world scenarios where time-space trade-offs come into play:
1. Database Indexing
Database indexes are a classic example of trading space for time. By creating an index, we use additional storage space to maintain a separate data structure that allows for faster querying and sorting of data. This significantly improves read performance at the cost of increased write times and storage requirements.
2. Caching in Web Applications
Caching frequently accessed data in memory (e.g., using Redis or Memcached) is a common technique to improve web application performance. This trade-off uses additional memory to store cached results, reducing the need for expensive database queries or computations.
3. Compression Algorithms
Data compression algorithms often involve a trade-off between compression ratio (space savings) and compression/decompression speed (time efficiency). Some algorithms prioritize higher compression ratios at the cost of slower processing, while others offer faster compression/decompression with less space savings.
4. Graphics Rendering
In computer graphics and game development, techniques like texture atlasing and mesh optimization often involve trade-offs between memory usage and rendering performance. For example, using larger textures can improve visual quality but requires more memory and may impact load times.
Conclusion
Mastering the art of analyzing and optimizing time-space trade-offs is a crucial skill for any programmer, especially those preparing for technical interviews at top tech companies. By understanding the fundamental concepts, considering various factors, and applying practical strategies, you can make informed decisions to balance time and space efficiency in your code.
Remember that there’s rarely a one-size-fits-all solution when it comes to optimizing algorithms. Each problem and scenario may require a different approach, and it’s essential to analyze the specific requirements and constraints before deciding on the best course of action.
As you continue to develop your programming skills, make it a habit to critically evaluate your code for potential time-space trade-offs. Practice implementing different optimization techniques and analyze their impact on performance. With time and experience, you’ll develop an intuition for making the right trade-offs and writing more efficient, scalable code.
Keep in mind that premature optimization can sometimes lead to unnecessarily complex code. Always start with a clear, correct implementation, and then optimize based on profiling results and actual performance requirements. By striking the right balance between time efficiency, space efficiency, and code readability, you’ll be well-equipped to tackle complex programming challenges and excel in technical interviews.