An Overview of Computational Complexity Theory
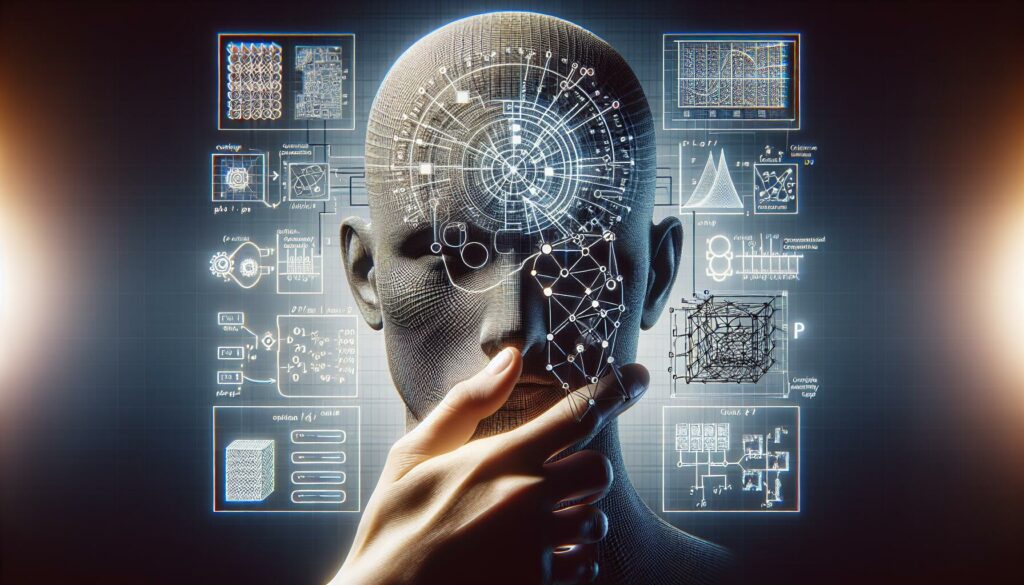
In the vast realm of computer science and algorithm design, one of the most fundamental and intriguing areas of study is computational complexity theory. This field forms the backbone of understanding how efficiently algorithms can solve problems and what limits exist in computational problem-solving. For anyone diving into the world of coding, especially those preparing for technical interviews at major tech companies, grasping the concepts of computational complexity is crucial.
In this comprehensive guide, we’ll explore the intricacies of computational complexity theory, its importance in algorithm design, and how it relates to practical coding challenges. Whether you’re a beginner just starting your journey in programming or an experienced developer looking to solidify your understanding, this article will provide valuable insights into this essential aspect of computer science.
What is Computational Complexity Theory?
Computational complexity theory is a branch of theoretical computer science that focuses on classifying computational problems according to their inherent difficulty. It provides a framework for understanding the resources (such as time and memory) required to solve computational problems and the limitations of algorithmic solutions.
The primary goals of computational complexity theory include:
- Classifying problems based on their difficulty
- Determining the efficiency of algorithms
- Identifying the boundaries between solvable and unsolvable problems
- Exploring the relationships between different computational problems
By studying computational complexity, we can gain insights into the fundamental nature of computation and develop more efficient algorithms for solving real-world problems.
Key Concepts in Computational Complexity
To understand computational complexity theory, it’s essential to familiarize yourself with several key concepts:
1. Time Complexity
Time complexity refers to the amount of time an algorithm takes to complete as a function of the input size. It’s typically expressed using Big O notation, which describes the upper bound of the growth rate of an algorithm’s running time.
Common time complexities include:
- O(1): Constant time
- O(log n): Logarithmic time
- O(n): Linear time
- O(n log n): Linearithmic time
- O(n^2): Quadratic time
- O(2^n): Exponential time
For example, a simple linear search algorithm has a time complexity of O(n), while a binary search algorithm has a time complexity of O(log n).
2. Space Complexity
Space complexity refers to the amount of memory an algorithm uses as a function of the input size. Like time complexity, it’s often expressed using Big O notation.
Common space complexities include:
- O(1): Constant space
- O(n): Linear space
- O(n^2): Quadratic space
An algorithm that uses a fixed amount of memory regardless of input size has a space complexity of O(1), while an algorithm that creates a new array of size n has a space complexity of O(n).
3. Problem Classes
Computational complexity theory classifies problems into different complexity classes based on the resources required to solve them. Some important problem classes include:
- P (Polynomial time): Problems that can be solved in polynomial time by a deterministic Turing machine
- NP (Nondeterministic Polynomial time): Problems that can be verified in polynomial time
- NP-Complete: The hardest problems in NP, to which all other NP problems can be reduced
- NP-Hard: Problems that are at least as hard as the hardest problems in NP
Understanding these problem classes is crucial for analyzing the difficulty of computational problems and designing efficient algorithms.
The Importance of Computational Complexity in Algorithm Design
Computational complexity plays a vital role in algorithm design and analysis. Here are some key reasons why understanding complexity is essential for programmers and computer scientists:
1. Efficiency and Scalability
By analyzing the time and space complexity of algorithms, we can determine their efficiency and scalability. This knowledge helps us choose the most appropriate algorithm for a given problem, especially when dealing with large datasets or time-sensitive applications.
For example, consider two sorting algorithms: bubble sort and merge sort. Bubble sort has an average and worst-case time complexity of O(n^2), while merge sort has a time complexity of O(n log n) for all cases. For small datasets, the difference might be negligible, but as the input size grows, merge sort becomes significantly more efficient.
2. Resource Optimization
Understanding the complexity of algorithms allows developers to optimize resource usage, such as CPU time and memory. This is particularly important in resource-constrained environments or when dealing with large-scale systems.
For instance, if you’re developing an application for a mobile device with limited memory, you might prioritize algorithms with lower space complexity to ensure smooth performance.
3. Problem-Solving Strategies
Knowledge of computational complexity helps in developing problem-solving strategies. When faced with a new problem, understanding its complexity class can guide you towards appropriate solution approaches.
For example, if you encounter an NP-Hard problem, you might consider using approximation algorithms or heuristics instead of seeking an exact solution, especially for large inputs.
4. Performance Prediction
Complexity analysis allows developers to predict how an algorithm will perform as input sizes increase. This is crucial for anticipating scalability issues and planning for future growth.
By understanding the complexity of your algorithms, you can estimate how they will behave with larger datasets and make informed decisions about system architecture and design.
Common Complexity Classes and Their Significance
Let’s delve deeper into some of the most important complexity classes and their significance in algorithm design and problem-solving:
1. P (Polynomial Time)
Problems in the class P are considered efficiently solvable. These are problems for which polynomial-time algorithms exist. Many common algorithmic problems fall into this category, including:
- Sorting
- Searching in a sorted array
- Matrix multiplication
- Shortest path in a graph (e.g., Dijkstra’s algorithm)
When designing algorithms, aiming for polynomial time solutions is often considered a benchmark for efficiency.
2. NP (Nondeterministic Polynomial Time)
The class NP includes all problems in P, as well as problems for which a solution can be verified in polynomial time, even if finding the solution might take longer. Some notable problems in NP include:
- Boolean Satisfiability Problem (SAT)
- Traveling Salesman Problem
- Graph Coloring
- Subset Sum
The relationship between P and NP is one of the most famous open questions in computer science, known as the P vs NP problem. If P = NP, it would have profound implications for cryptography and algorithm design.
3. NP-Complete
NP-Complete problems are the hardest problems in NP. They have the property that if an efficient (polynomial-time) algorithm is found for any NP-Complete problem, it can be used to solve all problems in NP efficiently. Some well-known NP-Complete problems include:
- Boolean Satisfiability Problem (SAT)
- Traveling Salesman Problem (decision version)
- Graph Coloring
- Hamiltonian Cycle Problem
When faced with an NP-Complete problem, it’s often more practical to look for approximate solutions or heuristics rather than exact algorithms, especially for large inputs.
4. NP-Hard
NP-Hard problems are at least as hard as the hardest problems in NP. These problems might not be in NP themselves, but any problem in NP can be reduced to them in polynomial time. Some examples of NP-Hard problems include:
- Traveling Salesman Problem (optimization version)
- Graph Partitioning
- Longest Path Problem
NP-Hard problems are considered intractable for large inputs, and solutions often involve approximation algorithms, heuristics, or solving for special cases.
Analyzing Algorithm Complexity: A Practical Approach
Understanding how to analyze the complexity of algorithms is a crucial skill for any programmer. Here’s a step-by-step approach to determining the time and space complexity of an algorithm:
1. Identify the Input
Determine what constitutes the input to your algorithm and how it affects the number of operations. Common inputs include the size of an array, the number of nodes in a graph, or the number of digits in a number.
2. Count the Operations
Analyze the algorithm to count the number of basic operations (such as comparisons, arithmetic operations, or assignments) as a function of the input size. Pay special attention to loops and recursive calls, as these often dominate the complexity.
3. Express in Big O Notation
Express the count of operations in Big O notation, focusing on the dominant term and disregarding constants and lower-order terms. For example, if your analysis shows 3n^2 + 2n + 1 operations, the Big O notation would be O(n^2).
4. Consider Best, Average, and Worst Cases
Analyze the complexity for different scenarios, including the best case, average case, and worst case. This provides a more comprehensive understanding of the algorithm’s performance across various inputs.
5. Analyze Space Complexity
Determine the amount of additional memory the algorithm uses as a function of the input size. Consider variables, data structures, and recursive call stacks.
Example: Analyzing Binary Search
Let’s apply these steps to analyze the time complexity of a binary search algorithm:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
- Input: The input is an array of size n and a target value.
- Count operations: In each iteration, we perform a comparison and potentially update left or right. The number of iterations is determined by how many times we can divide n by 2 until we reach 1.
- Express in Big O: The number of iterations is logâ‚‚(n), so the time complexity is O(log n).
- Cases: The best case is O(1) if the target is at the middle. The average and worst cases are both O(log n).
- Space complexity: The algorithm uses a constant amount of extra space, so the space complexity is O(1).
Common Pitfalls in Complexity Analysis
When analyzing algorithm complexity, there are several common pitfalls to avoid:
1. Ignoring Constants in Big O Notation
While Big O notation disregards constants, it’s important to remember that constants can still have a significant impact on performance, especially for smaller inputs. For example, an O(n) algorithm with a large constant factor might be slower than an O(n log n) algorithm for small to medium-sized inputs.
2. Overlooking Space Complexity
It’s easy to focus solely on time complexity and forget about space complexity. However, in many real-world scenarios, memory usage can be just as critical as runtime performance.
3. Misidentifying the Dominant Term
When dealing with multiple terms, it’s crucial to correctly identify the dominant term. For example, in the expression n^2 + n log n, the n^2 term dominates for large n, so the complexity would be O(n^2), not O(n^2 + n log n).
4. Assuming Worst-Case Complexity Always Applies
While it’s important to consider worst-case scenarios, always assuming worst-case performance can lead to overly pessimistic estimates. In practice, average-case performance might be more relevant.
5. Neglecting the Impact of Data Structures
The choice of data structure can significantly affect an algorithm’s complexity. For example, using a hash table instead of an array can change a lookup operation from O(n) to O(1) on average.
Advanced Topics in Computational Complexity
For those looking to delve deeper into computational complexity theory, there are several advanced topics worth exploring:
1. Amortized Analysis
Amortized analysis is a method for analyzing the time complexity of algorithms that perform a sequence of operations. It considers the average performance of each operation in the worst-case sequence of operations, rather than focusing on the worst-case cost of a single operation.
This type of analysis is particularly useful for data structures like dynamic arrays, where occasional expensive operations (like resizing) are balanced out by many cheap operations.
2. Randomized Algorithms
Randomized algorithms incorporate random choices into their logic. These algorithms can sometimes achieve better average-case performance than deterministic algorithms. Analyzing the complexity of randomized algorithms often involves probabilistic techniques.
Examples of randomized algorithms include QuickSort with random pivot selection and randomized primality testing algorithms.
3. Approximation Algorithms
For NP-Hard optimization problems, finding exact solutions in polynomial time is often infeasible. Approximation algorithms aim to find near-optimal solutions in polynomial time. The analysis of these algorithms involves studying their approximation ratio, which measures how close the algorithm’s solution is to the optimal solution.
4. Online Algorithms
Online algorithms must process input piece-by-piece in a serial fashion, without having the entire input available from the start. The analysis of online algorithms often involves competitive analysis, which compares the performance of the online algorithm to an optimal offline algorithm that has access to the entire input in advance.
5. Parameterized Complexity
Parameterized complexity is a branch of computational complexity theory that focuses on classifying computational problems based on their inherent difficulty with respect to multiple parameters. This approach can sometimes identify efficient algorithms for problems that are intractable when considering only the input size.
Practical Applications of Computational Complexity Theory
Understanding computational complexity has numerous practical applications in software development and computer science:
1. Algorithm Selection and Optimization
Knowledge of computational complexity allows developers to choose the most appropriate algorithms for specific problems and optimize them for better performance. This is particularly important when dealing with large datasets or time-critical applications.
2. System Design and Scalability
When designing large-scale systems, understanding the complexity of various components helps in predicting how the system will perform as the data volume or user base grows. This insight is crucial for creating scalable architectures.
3. Database Query Optimization
In database management systems, query optimization relies heavily on complexity analysis to determine the most efficient way to execute a given query.
4. Cryptography
Many cryptographic systems rely on the assumed complexity of certain mathematical problems. For example, the security of RSA encryption is based on the difficulty of factoring large numbers, which is believed to be a computationally hard problem.
5. Machine Learning and AI
In machine learning and artificial intelligence, understanding the complexity of learning algorithms is crucial for developing efficient and scalable models. It also helps in analyzing the feasibility of solving certain problems using AI techniques.
6. Compiler Optimization
Compilers use complexity analysis to make decisions about code optimization, such as choosing between different loop structures or deciding when to inline functions.
Tools and Techniques for Complexity Analysis
Several tools and techniques can aid in the analysis of algorithm complexity:
1. Profiling Tools
Profiling tools can help measure the actual runtime and memory usage of algorithms, providing empirical data to complement theoretical analysis. Examples include:
- Python’s cProfile
- Java’s VisualVM
- Valgrind for C and C++
2. Big O Calculators
Online tools and libraries can help calculate Big O notation for given code snippets or mathematical expressions. While these shouldn’t replace manual analysis, they can be useful for quick checks or learning purposes.
3. Visualization Tools
Visualization tools can help in understanding the growth of functions and comparing different complexity classes. Tools like Desmos or Python’s matplotlib can be used to plot complexity functions.
4. Asymptotic Analysis Techniques
Techniques such as the Master Theorem for analyzing recursive algorithms, or the substitution method for solving recurrence relations, are invaluable for complexity analysis.
5. Benchmarking Frameworks
Benchmarking frameworks allow for systematic comparison of algorithm performance across different inputs and implementations. Examples include:
- Google Benchmark for C++
- JMH (Java Microbenchmark Harness) for Java
- Criterion for Haskell
Conclusion
Computational complexity theory is a fundamental aspect of computer science that provides a framework for understanding the efficiency and limitations of algorithms. By mastering the concepts of time and space complexity, problem classification, and analysis techniques, developers can create more efficient and scalable solutions to computational problems.
As you continue your journey in coding and algorithm design, remember that understanding complexity is not just about theoretical knowledge—it’s a practical skill that can significantly impact the quality and performance of your software. Whether you’re preparing for technical interviews, optimizing existing systems, or tackling new algorithmic challenges, a solid grasp of computational complexity will be an invaluable asset.
Keep practicing complexity analysis, explore advanced topics, and always consider the efficiency of your algorithms. With time and experience, you’ll develop an intuition for complexity that will guide you towards better design decisions and more elegant solutions to complex problems.