An Overview of Branch and Bound Algorithms: Efficient Problem-Solving Techniques
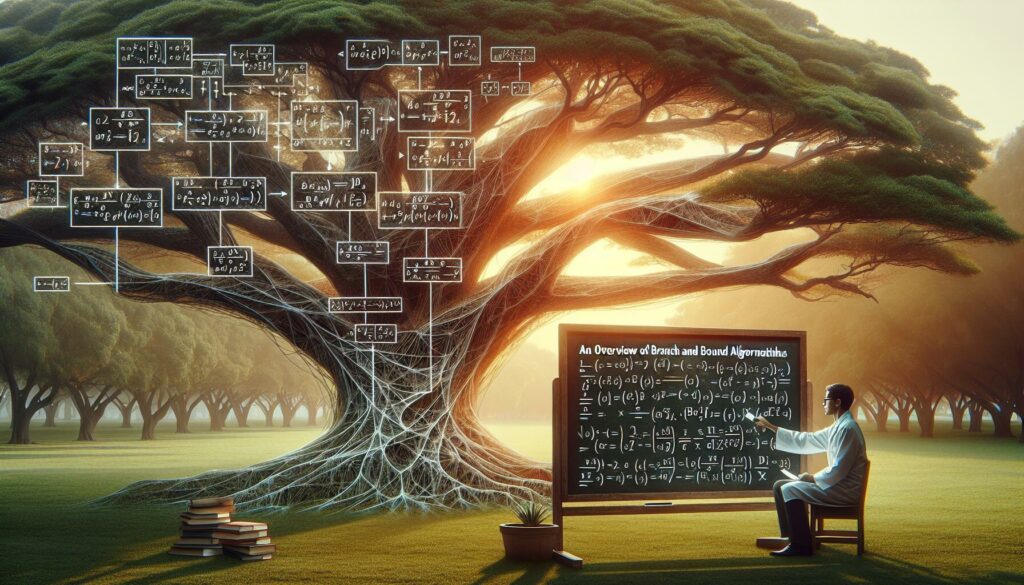
In the world of computer science and algorithmic problem-solving, efficiency is key. As programmers and developers, we’re constantly seeking ways to optimize our code and solve complex problems more effectively. One powerful technique that has proven invaluable in this pursuit is the Branch and Bound algorithm. In this comprehensive guide, we’ll dive deep into the world of Branch and Bound algorithms, exploring their principles, applications, and implementation strategies.
What is a Branch and Bound Algorithm?
Branch and Bound is a algorithmic paradigm used for solving optimization problems, particularly in discrete and combinatorial optimization. It’s a systematic method for solving problems by breaking them down into smaller subproblems (branching) and eliminating solutions that are known to be suboptimal (bounding).
The core idea behind Branch and Bound is to partition the solution space into smaller subsets, evaluate these subsets, and discard those that cannot possibly contain the optimal solution. This process continues recursively until the optimal solution is found or all possibilities have been exhausted.
Key Components of Branch and Bound Algorithms
To understand Branch and Bound algorithms better, let’s break down their key components:
1. Branching
Branching refers to the process of dividing a problem into smaller subproblems. This is typically done by making a decision or choice at each step, creating multiple branches in the solution space.
2. Bounding
Bounding involves calculating lower and upper bounds for the optimal solution within each subproblem. These bounds help in determining whether a particular branch is worth exploring further or can be safely discarded.
3. Pruning
Pruning is the process of eliminating branches that are guaranteed not to contain the optimal solution. This is done by comparing the bounds of a branch with the best known solution so far.
4. Search Strategy
The search strategy determines the order in which branches are explored. Common strategies include depth-first search, breadth-first search, and best-first search.
How Branch and Bound Algorithms Work
The general process of a Branch and Bound algorithm can be summarized as follows:
- Initialize the problem with the entire solution space.
- Select a branching variable and create subproblems (branches).
- Calculate bounds for each subproblem.
- Prune branches that cannot lead to a better solution than the current best.
- Select the most promising branch to explore further.
- Repeat steps 2-5 until the optimal solution is found or all branches are explored.
Advantages of Branch and Bound Algorithms
Branch and Bound algorithms offer several advantages in problem-solving:
- Optimality: They guarantee finding the optimal solution if one exists.
- Efficiency: By pruning unnecessary branches, they can significantly reduce the search space.
- Flexibility: The algorithm can be adapted to various problem types and domains.
- Anytime property: They can provide increasingly better solutions as the algorithm progresses, even if interrupted before completion.
Common Applications of Branch and Bound
Branch and Bound algorithms find applications in various fields and problem types:
1. Traveling Salesman Problem (TSP)
The TSP is a classic optimization problem where the goal is to find the shortest possible route that visits each city exactly once and returns to the starting city. Branch and Bound is often used to solve TSP instances efficiently.
2. Knapsack Problem
In the Knapsack Problem, we need to select a subset of items with maximum value while respecting a weight constraint. Branch and Bound can be applied to find the optimal solution.
3. Job Scheduling
Branch and Bound can be used to optimize job scheduling problems, where tasks need to be assigned to resources in the most efficient manner.
4. Integer Programming
Many integer programming problems can be solved using Branch and Bound techniques, especially when combined with linear programming relaxations.
Implementing a Branch and Bound Algorithm
Let’s look at a simple implementation of a Branch and Bound algorithm for solving the 0/1 Knapsack Problem. This problem involves selecting items to maximize value while respecting a weight limit.
class Item:
def __init__(self, weight, value):
self.weight = weight
self.value = value
def knapsack_branch_and_bound(items, capacity):
n = len(items)
best_value = 0
best_solution = []
def bound(k, weight, value):
if weight > capacity:
return 0
bound_value = value
j = k
while j < n and weight + items[j].weight <= capacity:
weight += items[j].weight
bound_value += items[j].value
j += 1
if j < n:
bound_value += (capacity - weight) * items[j].value / items[j].weight
return bound_value
def branch_and_bound(k, weight, value, solution):
nonlocal best_value, best_solution
if k == n:
if value > best_value:
best_value = value
best_solution = solution[:]
return
if weight + items[k].weight <= capacity:
solution.append(k)
branch_and_bound(k + 1, weight + items[k].weight, value + items[k].value, solution)
solution.pop()
if bound(k + 1, weight, value) > best_value:
branch_and_bound(k + 1, weight, value, solution)
items.sort(key=lambda x: x.value / x.weight, reverse=True)
branch_and_bound(0, 0, 0, [])
return best_value, best_solution
# Example usage
items = [Item(10, 60), Item(20, 100), Item(30, 120)]
capacity = 50
max_value, selected_items = knapsack_branch_and_bound(items, capacity)
print(f"Maximum value: {max_value}")
print(f"Selected items: {selected_items}")
This implementation uses a recursive approach with bounding and pruning to solve the Knapsack Problem efficiently. The bound
function calculates an upper bound on the possible value for a given branch, which is used for pruning unpromising branches.
Best Practices for Implementing Branch and Bound Algorithms
When implementing Branch and Bound algorithms, consider the following best practices:
1. Choose an Effective Branching Strategy
The choice of branching variable can significantly impact the algorithm’s efficiency. Select variables that are likely to lead to good solutions or eliminate large portions of the search space.
2. Develop Tight Bounds
Tighter bounds lead to more effective pruning. Invest time in developing accurate and computationally efficient bounding functions.
3. Implement Efficient Data Structures
Use appropriate data structures to manage the search tree and priority queue (if using best-first search). This can greatly affect the algorithm’s performance, especially for large problem instances.
4. Consider Problem-Specific Heuristics
Incorporate problem-specific knowledge or heuristics to guide the search more effectively. This can lead to finding good solutions faster.
5. Implement Incremental Bound Updates
When possible, update bounds incrementally rather than recalculating them from scratch at each node. This can save significant computation time.
Advanced Techniques in Branch and Bound
As you become more comfortable with basic Branch and Bound algorithms, consider exploring these advanced techniques:
1. Parallel Branch and Bound
Leverage parallel computing to explore multiple branches simultaneously, potentially speeding up the search process significantly.
2. Hybrid Algorithms
Combine Branch and Bound with other optimization techniques like dynamic programming or metaheuristics for improved performance on specific problem types.
3. Constraint Programming Integration
Incorporate constraint programming techniques to enhance the bounding and pruning processes in Branch and Bound algorithms.
4. Machine Learning-Enhanced Branch and Bound
Use machine learning models to predict promising branches or estimate bounds, potentially improving the algorithm’s efficiency.
Common Challenges and Solutions
While Branch and Bound algorithms are powerful, they can face certain challenges:
Challenge 1: Exponential Worst-Case Complexity
Solution: Implement aggressive pruning strategies and consider approximation algorithms for very large problem instances.
Challenge 2: Memory Limitations
Solution: Use depth-first search strategies or implement memory-efficient tree representations to manage large search spaces.
Challenge 3: Difficulty in Determining Good Bounds
Solution: Invest time in developing problem-specific bounding functions and consider using relaxations of the original problem for bound estimation.
Challenge 4: Balancing Exploration and Exploitation
Solution: Experiment with different search strategies and implement adaptive approaches that balance between depth-first and best-first search.
Branch and Bound in Practice: Real-World Examples
To illustrate the practical applications of Branch and Bound algorithms, let’s look at some real-world examples:
1. Supply Chain Optimization
Branch and Bound algorithms are used in supply chain management to optimize inventory levels, distribution routes, and production schedules. For example, a large retailer might use Branch and Bound to determine the most cost-effective way to distribute products from warehouses to stores while meeting demand constraints.
2. VLSI Circuit Design
In Very Large Scale Integration (VLSI) circuit design, Branch and Bound techniques are employed to solve placement and routing problems. These algorithms help in optimizing the layout of components on a chip to minimize area, wire length, and power consumption.
3. Portfolio Optimization
Financial institutions use Branch and Bound algorithms for portfolio optimization problems. These algorithms help in selecting the best combination of investments to maximize returns while managing risk within specified constraints.
4. Network Design
Telecommunications companies apply Branch and Bound algorithms to design optimal network topologies. This involves determining the most efficient way to connect nodes in a network while minimizing costs and maximizing performance.
Future Directions in Branch and Bound Research
As technology advances, several exciting directions are emerging in Branch and Bound research:
1. Quantum Branch and Bound
Researchers are exploring how quantum computing principles can be applied to Branch and Bound algorithms, potentially leading to significant speedups for certain problem classes.
2. Adaptive and Self-Tuning Algorithms
Development of Branch and Bound algorithms that can adapt their strategies and parameters based on the problem instance and runtime behavior.
3. Integration with Deep Learning
Exploring ways to leverage deep learning models to enhance various aspects of Branch and Bound algorithms, such as branching decisions and bound estimations.
4. Application to New Domains
Extending Branch and Bound techniques to emerging fields like computational biology, autonomous systems, and climate modeling.
Conclusion
Branch and Bound algorithms represent a powerful and flexible approach to solving complex optimization problems. By systematically exploring the solution space and pruning unpromising branches, these algorithms can efficiently find optimal solutions to a wide range of problems.
As we’ve seen, the key to successful implementation lies in choosing effective branching strategies, developing tight bounds, and leveraging problem-specific knowledge. While challenges exist, particularly for large-scale problems, ongoing research and advancements in computing power continue to expand the horizons of what’s possible with Branch and Bound techniques.
For aspiring programmers and seasoned developers alike, mastering Branch and Bound algorithms opens up a world of possibilities in tackling optimization challenges across various domains. Whether you’re optimizing supply chains, designing circuits, or solving classic computational problems, the principles of Branch and Bound provide a robust framework for approaching complex decision-making tasks.
As you continue your journey in algorithmic problem-solving, remember that Branch and Bound is not just a technique, but a way of thinking about problems. It encourages us to break down complex challenges, make informed decisions, and continuously refine our approach based on new information. These skills are invaluable not just in coding, but in tackling real-world problems across all areas of life and work.
So, the next time you’re faced with a daunting optimization problem, consider reaching for the Branch and Bound toolbox. With practice and creativity, you’ll find it to be a powerful ally in your quest for efficient and optimal solutions.