An Introduction to Signal Processing Algorithms
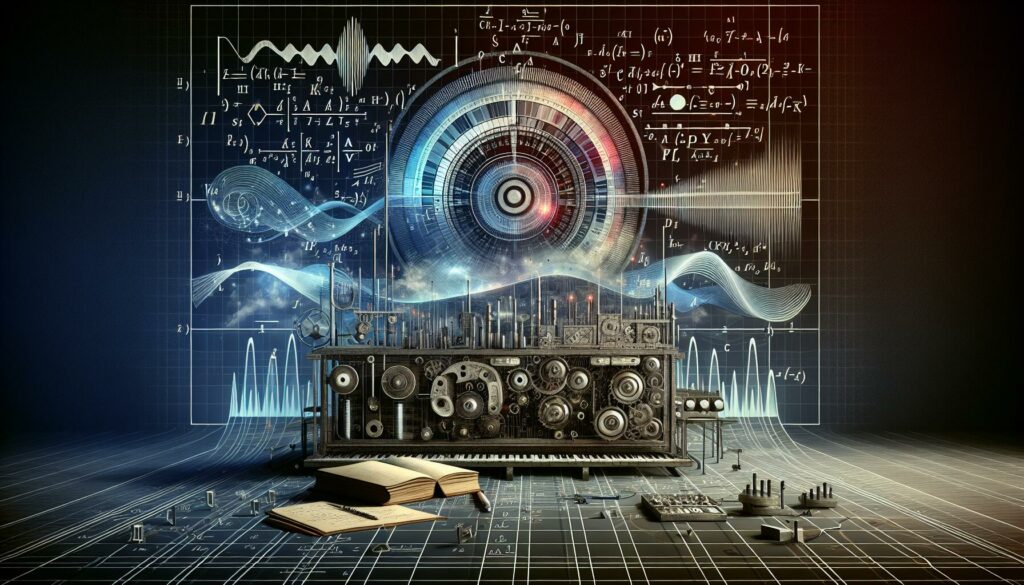
Signal processing is a fundamental aspect of modern technology, playing a crucial role in various fields such as telecommunications, audio and video processing, medical imaging, and more. As we delve into the world of signal processing algorithms, we’ll explore the core concepts, techniques, and applications that make this field so essential in our digital age. Whether you’re a budding engineer, a curious programmer, or simply interested in understanding the technology behind everyday devices, this comprehensive guide will provide you with a solid foundation in signal processing algorithms.
1. Understanding Signals and Systems
Before we dive into specific algorithms, it’s important to grasp the basics of signals and systems. A signal is any time-varying quantity that carries information, while a system is any process that operates on a signal to extract, modify, or transform that information.
1.1 Types of Signals
Signals can be classified into various categories:
- Continuous-time signals: Signals that are defined for all points in time (e.g., analog signals)
- Discrete-time signals: Signals that are defined only at specific time instances (e.g., digital signals)
- Periodic signals: Signals that repeat at regular intervals
- Aperiodic signals: Signals that do not repeat
- Deterministic signals: Signals whose future values can be predicted precisely
- Random signals: Signals whose future values are unpredictable and can only be described statistically
1.2 Properties of Systems
Systems can be characterized by various properties:
- Linearity: A system is linear if it obeys the principles of superposition and homogeneity
- Time-invariance: A system is time-invariant if its behavior does not change over time
- Causality: A causal system’s output depends only on past and present inputs, not future inputs
- Stability: A stable system produces bounded outputs for bounded inputs
- Memory: A system with memory has an output that depends on past inputs as well as the current input
2. Fundamental Signal Processing Techniques
Now that we have a basic understanding of signals and systems, let’s explore some fundamental signal processing techniques that form the basis for more advanced algorithms.
2.1 Sampling and Quantization
Sampling is the process of converting a continuous-time signal into a discrete-time signal by taking measurements at regular intervals. The sampling rate, or sampling frequency, determines how often samples are taken. The Nyquist-Shannon sampling theorem states that to accurately reconstruct a signal, the sampling rate must be at least twice the highest frequency component in the signal.
Quantization is the process of converting a continuous-amplitude signal into a discrete-amplitude signal. This is typically done by rounding the continuous values to the nearest discrete level.
2.2 Fourier Transform
The Fourier Transform is a powerful mathematical tool that decomposes a signal into its constituent frequencies. It allows us to analyze signals in the frequency domain, which can reveal important characteristics that may not be apparent in the time domain.
There are several variants of the Fourier Transform:
- Continuous Fourier Transform (CFT): For continuous-time signals
- Discrete Fourier Transform (DFT): For discrete-time signals
- Fast Fourier Transform (FFT): An efficient algorithm for computing the DFT
Here’s a simple implementation of the DFT in Python:
import numpy as np
def dft(x):
N = len(x)
n = np.arange(N)
k = n.reshape((N, 1))
e = np.exp(-2j * np.pi * k * n / N)
return np.dot(e, x)
# Example usage
x = np.array([1, 2, 3, 4])
X = dft(x)
print(X)
2.3 Convolution
Convolution is a mathematical operation that combines two signals to produce a third signal. It’s fundamental in signal processing and is used in various applications, such as filtering and system analysis.
The convolution of two discrete-time signals x[n] and h[n] is defined as:
y[n] = x[n] * h[n] = Σ(k=-∞ to ∞) x[k] * h[n-k]
Here’s a Python implementation of discrete convolution:
import numpy as np
def convolve(x, h):
return np.convolve(x, h, mode='full')
# Example usage
x = np.array([1, 2, 3, 4])
h = np.array([0.5, 0.5])
y = convolve(x, h)
print(y)
3. Digital Filters
Digital filters are systems designed to remove unwanted components or features from a signal. They are widely used in signal processing applications to improve signal quality, extract specific frequency components, or suppress noise.
3.1 Finite Impulse Response (FIR) Filters
FIR filters are characterized by their finite impulse response. They are always stable and can have linear phase, making them suitable for many applications. The output of an FIR filter is a weighted sum of the current and past input values:
y[n] = b[0]*x[n] + b[1]*x[n-1] + ... + b[M]*x[n-M]
Where b[k] are the filter coefficients and M is the filter order.
Here’s a simple implementation of an FIR filter in Python:
import numpy as np
def fir_filter(x, b):
return np.convolve(x, b, mode='valid')
# Example usage: Low-pass filter
n = np.arange(61)
b = np.sinc(0.1 * (n - 30))
b /= np.sum(b) # Normalize
x = np.random.randn(1000) # Input signal
y = fir_filter(x, b)
3.2 Infinite Impulse Response (IIR) Filters
IIR filters have an infinite impulse response and use feedback. They can achieve sharper cutoff frequencies with fewer coefficients compared to FIR filters, but they may have stability issues and non-linear phase response. The general form of an IIR filter is:
y[n] = (b[0]*x[n] + b[1]*x[n-1] + ... + b[M]*x[n-M]) - (a[1]*y[n-1] + a[2]*y[n-2] + ... + a[N]*y[n-N])
Where b[k] and a[k] are the filter coefficients, and M and N are the feedforward and feedback filter orders, respectively.
Here’s a simple implementation of an IIR filter in Python:
from scipy import signal
def iir_filter(x, b, a):
return signal.lfilter(b, a, x)
# Example usage: Butterworth low-pass filter
b, a = signal.butter(4, 0.1) # 4th order, cutoff frequency 0.1
x = np.random.randn(1000) # Input signal
y = iir_filter(x, b, a)
4. Adaptive Filters
Adaptive filters are self-adjusting digital filters that can modify their characteristics in response to changing input signal properties. They are particularly useful in applications where the signal or noise characteristics are unknown or time-varying.
4.1 Least Mean Squares (LMS) Algorithm
The LMS algorithm is one of the most widely used adaptive filter algorithms due to its simplicity and robustness. It aims to minimize the mean square error between the desired signal and the filter output.
Here’s a basic implementation of the LMS algorithm:
import numpy as np
def lms_filter(x, d, M, mu):
N = len(x)
w = np.zeros(M)
y = np.zeros(N)
e = np.zeros(N)
for n in range(M, N):
x_n = x[n-M:n][::-1]
y[n] = np.dot(w, x_n)
e[n] = d[n] - y[n]
w += 2 * mu * e[n] * x_n
return y, e, w
# Example usage
N = 1000
x = np.random.randn(N)
d = np.sin(0.1 * np.arange(N)) + 0.1 * np.random.randn(N)
M = 32
mu = 0.01
y, e, w = lms_filter(x, d, M, mu)
5. Spectral Analysis
Spectral analysis involves examining the frequency content of a signal. It’s a crucial technique in many signal processing applications, from speech recognition to radar systems.
5.1 Power Spectral Density (PSD)
The Power Spectral Density describes how the power of a signal is distributed across different frequencies. For a discrete-time signal, the PSD can be estimated using various methods, including the periodogram and Welch’s method.
Here’s an example of how to compute the PSD using Welch’s method in Python:
from scipy import signal
import numpy as np
import matplotlib.pyplot as plt
# Generate a test signal
t = np.linspace(0, 1, 1000, endpoint=False)
x = np.sin(2*np.pi*10*t) + 0.5*np.sin(2*np.pi*20*t) + 0.1*np.random.randn(len(t))
# Compute PSD using Welch's method
f, Pxx = signal.welch(x, fs=1000, nperseg=256)
# Plot the results
plt.semilogy(f, Pxx)
plt.xlabel('Frequency [Hz]')
plt.ylabel('PSD [V**2/Hz]')
plt.show()
6. Time-Frequency Analysis
Time-frequency analysis techniques allow us to analyze how the frequency content of a signal changes over time. This is particularly useful for non-stationary signals whose properties change over time.
6.1 Short-Time Fourier Transform (STFT)
The STFT divides a longer time signal into shorter segments of equal length and then computes the Fourier transform separately on each shorter segment. This allows us to see how frequency content changes over time.
Here’s an example of how to compute and visualize the STFT using Python:
from scipy import signal
import numpy as np
import matplotlib.pyplot as plt
# Generate a chirp signal
t = np.linspace(0, 10, 1000)
w = signal.chirp(t, f0=1, f1=10, t1=10, method='linear')
# Compute STFT
f, t, Zxx = signal.stft(w, fs=100, nperseg=64)
# Plot the results
plt.pcolormesh(t, f, np.abs(Zxx), shading='gouraud')
plt.title('STFT Magnitude')
plt.ylabel('Frequency [Hz]')
plt.xlabel('Time [sec]')
plt.colorbar(label='Magnitude')
plt.show()
6.2 Wavelet Transform
The Wavelet Transform provides a multi-resolution analysis of signals, allowing for better time-frequency localization compared to the STFT. It uses wavelets, which are oscillating functions that are localized in both time and frequency.
Here’s an example of how to perform a wavelet transform using Python:
import numpy as np
import pywt
import matplotlib.pyplot as plt
# Generate a test signal
t = np.linspace(0, 1, 1000, endpoint=False)
x = np.sin(2*np.pi*10*t) + 0.5*np.sin(2*np.pi*20*t)
# Perform wavelet transform
scales = np.arange(1, 128)
coeffs, freqs = pywt.cwt(x, scales, 'morl')
# Plot the results
plt.imshow(abs(coeffs), extent=[0, 1, 1, 128], cmap='jet', aspect='auto')
plt.ylabel('Scale')
plt.xlabel('Time')
plt.title('Continuous Wavelet Transform')
plt.colorbar(label='Magnitude')
plt.show()
7. Applications of Signal Processing Algorithms
Signal processing algorithms find applications in numerous fields. Here are some notable examples:
7.1 Audio Processing
Signal processing is extensively used in audio applications, including:
- Noise reduction
- Audio compression (e.g., MP3)
- Speech recognition
- Music analysis and synthesis
7.2 Image and Video Processing
Many image and video processing techniques rely on signal processing algorithms:
- Image enhancement and restoration
- Image compression (e.g., JPEG)
- Object detection and recognition
- Video stabilization
7.3 Biomedical Signal Processing
Signal processing plays a crucial role in analyzing and interpreting biomedical signals:
- ECG analysis
- EEG processing
- Medical imaging (e.g., MRI, CT)
- Brain-computer interfaces
7.4 Communications
Modern communication systems heavily rely on signal processing:
- Modulation and demodulation
- Channel equalization
- Error correction coding
- Spread spectrum techniques
7.5 Radar and Sonar
Signal processing is fundamental in radar and sonar systems:
- Target detection and tracking
- Doppler processing
- Beamforming
- Synthetic aperture radar (SAR) imaging
8. Challenges and Future Directions
As technology advances, signal processing algorithms continue to evolve to meet new challenges and opportunities:
8.1 Big Data and Real-Time Processing
The increasing volume and velocity of data require more efficient algorithms and hardware implementations to process signals in real-time or near real-time.
8.2 Machine Learning Integration
The integration of machine learning techniques with traditional signal processing algorithms is opening up new possibilities for adaptive and intelligent signal processing systems.
8.3 Quantum Signal Processing
As quantum computing technology advances, researchers are exploring quantum algorithms for signal processing tasks, which could potentially offer significant speedups for certain problems.
8.4 Edge Computing
The trend towards edge computing is driving the development of more efficient and compact signal processing algorithms that can run on resource-constrained devices.
Conclusion
Signal processing algorithms form the backbone of many modern technologies, enabling us to extract meaningful information from complex signals and design sophisticated systems. From basic techniques like filtering and Fourier analysis to advanced methods like adaptive filtering and wavelet transforms, these algorithms provide powerful tools for analyzing and manipulating signals in various domains.
As we’ve seen, the field of signal processing is vast and interdisciplinary, with applications ranging from everyday consumer electronics to cutting-edge scientific research. By understanding the fundamentals of signal processing algorithms, you’re equipped with knowledge that’s applicable across numerous fields and technologies.
As technology continues to advance, signal processing algorithms will undoubtedly play an increasingly important role in shaping our digital world. Whether you’re a student, researcher, or professional, a solid grasp of these concepts will serve you well in navigating the complexities of modern signal processing and its myriad applications.