An Introduction to Algorithm Design Patterns
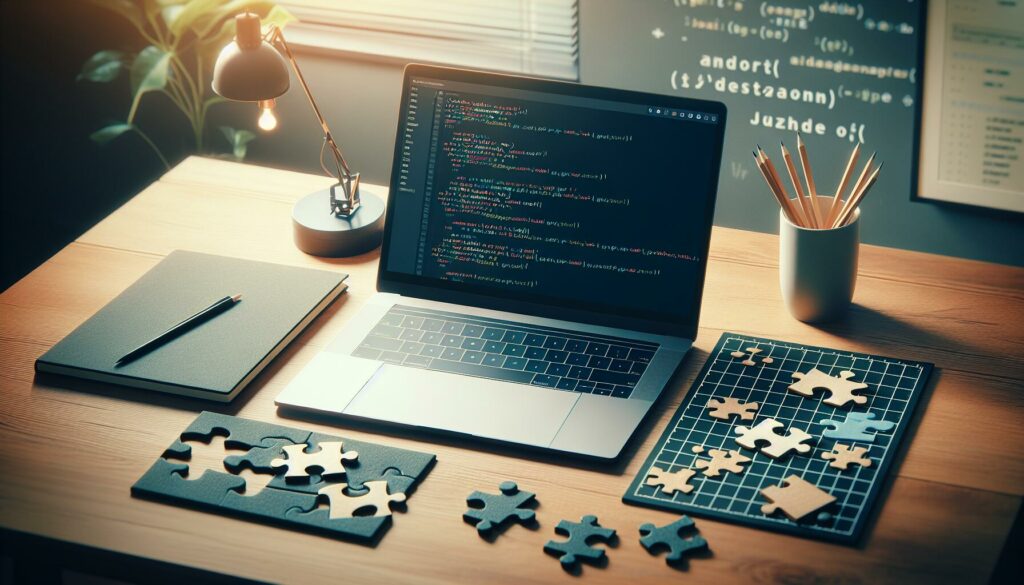
In the world of computer science and software engineering, algorithm design patterns are essential tools that help developers create efficient and elegant solutions to complex problems. These patterns are reusable templates that can be applied to various scenarios, saving time and improving code quality. In this comprehensive guide, we’ll explore the concept of algorithm design patterns, their importance, and some of the most commonly used patterns in modern software development.
What Are Algorithm Design Patterns?
Algorithm design patterns are general, reusable solutions to common problems that occur in software design. They are not finished designs or code that can be directly copied and pasted into your program. Instead, they are templates or guidelines that can be adapted to solve specific issues in your code.
These patterns have been developed and refined over time by experienced programmers and computer scientists. They represent best practices and proven solutions that can be applied across different programming languages and paradigms.
Why Are Algorithm Design Patterns Important?
Understanding and utilizing algorithm design patterns offers several benefits to developers:
- Efficiency: Design patterns provide optimized solutions to common problems, saving time and computational resources.
- Readability: They create a common language among developers, making code easier to understand and maintain.
- Scalability: Many design patterns are inherently scalable, allowing your code to grow and adapt to changing requirements.
- Problem-solving: Familiarity with design patterns enhances your ability to approach and solve complex programming challenges.
- Best practices: Design patterns often incorporate software engineering best practices, leading to more robust and maintainable code.
Common Algorithm Design Patterns
Let’s explore some of the most widely used algorithm design patterns:
1. Divide and Conquer
The divide and conquer pattern involves breaking a problem into smaller, more manageable subproblems, solving them independently, and then combining the results to solve the original problem. This pattern is particularly useful for optimization and searching algorithms.
Example: Merge Sort
Merge Sort is a classic example of the divide and conquer pattern. It works by dividing the unsorted list into n sublists, each containing one element, then repeatedly merging sublists to produce new sorted sublists until there is only one sublist remaining.
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
2. Dynamic Programming
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It is particularly useful when subproblems overlap and when the problem has an optimal substructure property.
Example: Fibonacci Sequence
Calculating Fibonacci numbers using dynamic programming can significantly improve performance compared to a naive recursive approach.
def fibonacci(n):
if n <= 1:
return n
fib = [0] * (n + 1)
fib[1] = 1
for i in range(2, n + 1):
fib[i] = fib[i-1] + fib[i-2]
return fib[n]
3. Greedy Algorithms
Greedy algorithms make the locally optimal choice at each step with the hope of finding a global optimum. While they don’t always yield the best solution, they are often used for optimization problems where finding an approximate solution quickly is more important than finding the perfect solution.
Example: Coin Change Problem
Given a set of coin denominations and a target amount, find the minimum number of coins needed to make up that amount.
def coin_change(coins, amount):
coins.sort(reverse=True)
count = 0
for coin in coins:
while amount >= coin:
amount -= coin
count += 1
return count if amount == 0 else -1
4. Backtracking
Backtracking is an algorithmic technique that considers searching every possible combination in order to solve a computational problem. It builds candidates to the solution incrementally and abandons a candidate as soon as it determines that the candidate cannot lead to a valid solution.
Example: N-Queens Problem
The N-Queens problem involves placing N chess queens on an N×N chessboard so that no two queens threaten each other.
def solve_n_queens(n):
def is_safe(board, row, col):
# Check if a queen can be placed on board[row][col]
# Check this row on left side
for i in range(col):
if board[row][i] == 1:
return False
# Check upper diagonal on left side
for i, j in zip(range(row, -1, -1), range(col, -1, -1)):
if board[i][j] == 1:
return False
# Check lower diagonal on left side
for i, j in zip(range(row, n, 1), range(col, -1, -1)):
if board[i][j] == 1:
return False
return True
def solve(board, col):
if col >= n:
return True
for i in range(n):
if is_safe(board, i, col):
board[i][col] = 1
if solve(board, col + 1):
return True
board[i][col] = 0
return False
board = [[0 for _ in range(n)] for _ in range(n)]
if solve(board, 0) == False:
print("Solution does not exist")
return False
return board
# Example usage
solution = solve_n_queens(8)
if solution:
for row in solution:
print(row)
5. Two Pointers
The two pointers technique is a way to iterate through a data structure, typically an array or linked list, using two pointers that move through the structure in a specific way. This pattern is often used to solve problems with minimal space complexity.
Example: Remove Duplicates from Sorted Array
def remove_duplicates(nums):
if not nums:
return 0
# Initialize the slow pointer
slow = 0
# Fast pointer iterates through the array
for fast in range(1, len(nums)):
if nums[fast] != nums[slow]:
slow += 1
nums[slow] = nums[fast]
# Return the length of the array with duplicates removed
return slow + 1
6. Sliding Window
The sliding window technique is used to perform operations on a specific window size of an array or linked list. It can be used to solve problems related to arrays, strings, and subarrays in a more efficient manner.
Example: Maximum Sum Subarray of Size K
def max_sum_subarray(arr, k):
if len(arr) < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(k, len(arr)):
window_sum = window_sum - arr[i-k] + arr[i]
max_sum = max(max_sum, window_sum)
return max_sum
Applying Algorithm Design Patterns in Real-World Scenarios
Understanding these design patterns is crucial, but knowing when and how to apply them in real-world scenarios is equally important. Let’s explore some practical applications:
1. Search Engines
Search engines like Google use a combination of design patterns to efficiently crawl, index, and retrieve web pages. The divide and conquer pattern is often used in distributed computing to parallelize the crawling process. Dynamic programming techniques might be employed in ranking algorithms to optimize search results.
2. Financial Systems
In financial systems, the greedy algorithm pattern can be used for portfolio optimization or currency exchange. Dynamic programming is often applied in options pricing models like the Black-Scholes model.
3. Game Development
Game developers frequently use the backtracking pattern for puzzle-solving mechanisms or AI pathfinding. The two pointers technique might be used for collision detection in 2D games.
4. Network Routing
Routing algorithms in computer networks often employ the greedy algorithm pattern to find the shortest path between nodes. The sliding window technique is used in network protocols for flow control and congestion avoidance.
5. Image Processing
Image processing applications may use the divide and conquer pattern for tasks like image compression or feature detection. The sliding window technique is commonly used for image filtering and edge detection.
Best Practices for Using Algorithm Design Patterns
While algorithm design patterns are powerful tools, it’s important to use them judiciously. Here are some best practices to keep in mind:
- Understand the problem thoroughly: Before applying a design pattern, make sure you fully understand the problem and its constraints.
- Choose the right pattern: Not every problem requires a complex design pattern. Sometimes, a simple solution is more appropriate.
- Consider performance implications: While design patterns can improve code structure, they may sometimes introduce performance overhead. Always consider the trade-offs.
- Combine patterns when necessary: Real-world problems often require a combination of different design patterns to achieve the best solution.
- Keep it simple: Don’t over-engineer your solution. Use design patterns to simplify your code, not to make it more complex.
- Document your approach: When using a design pattern, document why you chose it and how it’s implemented. This helps other developers (including your future self) understand your code.
Conclusion
Algorithm design patterns are invaluable tools in a developer’s toolkit. They provide tested, reusable solutions to common programming problems, helping to create more efficient, readable, and maintainable code. By understanding these patterns and knowing when to apply them, you can significantly improve your problem-solving skills and the quality of your software.
As you continue your journey in software development, make it a point to study and practice these design patterns. Start by identifying them in existing codebases, then try implementing them in your own projects. Remember, mastering these patterns is not about memorizing code, but about understanding the underlying principles and knowing how to adapt them to different situations.
The world of algorithm design patterns is vast and continually evolving. As new technologies emerge and new problems arise, new patterns are developed to address them. Stay curious, keep learning, and don’t be afraid to experiment with different approaches. With time and practice, you’ll develop an intuition for when and how to use these powerful tools effectively.
Happy coding!