Algorithms in Sensor Networks and IoT Devices: Powering the Connected World
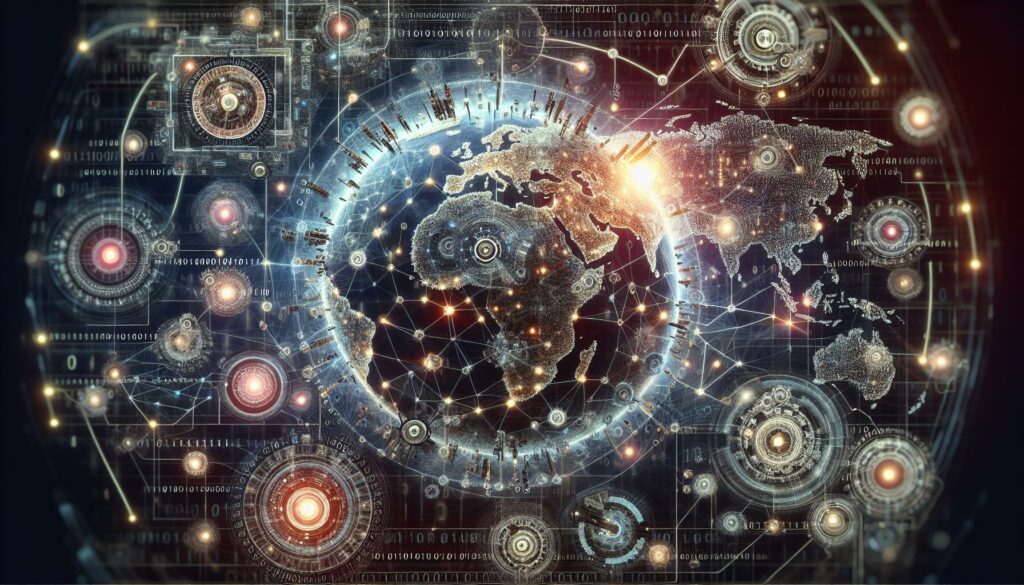
In today’s interconnected world, sensor networks and Internet of Things (IoT) devices have become ubiquitous, transforming the way we interact with our environment and collect data. At the heart of these technologies lie sophisticated algorithms that enable efficient communication, data processing, and decision-making. This article delves into the fascinating world of algorithms in sensor networks and IoT devices, exploring their importance, types, and applications.
Understanding Sensor Networks and IoT Devices
Before we dive into the algorithms, let’s briefly define sensor networks and IoT devices:
Sensor Networks
Sensor networks consist of interconnected sensor nodes that collect and transmit data about their environment. These networks can be deployed in various settings, from industrial facilities to natural habitats, to monitor and gather information about temperature, humidity, pressure, motion, and more.
IoT Devices
Internet of Things (IoT) devices are physical objects embedded with sensors, software, and network connectivity, allowing them to collect and exchange data. Examples include smart home devices, wearable fitness trackers, and industrial monitoring systems.
The Role of Algorithms in Sensor Networks and IoT
Algorithms play a crucial role in the functioning of sensor networks and IoT devices. They are responsible for:
- Data collection and processing
- Network communication and routing
- Energy management
- Security and privacy
- Decision-making and actuation
Let’s explore some of the key algorithms used in these areas:
1. Data Collection and Processing Algorithms
Sampling Algorithms
Sampling algorithms determine how frequently sensors collect data. They balance the need for accurate information with energy conservation and network bandwidth limitations.
Example: Adaptive Sampling
Adaptive sampling algorithms adjust the sampling rate based on the rate of change in the measured variable. For instance, a temperature sensor might sample more frequently when the temperature is changing rapidly and less frequently when it’s stable.
def adaptive_sampling(current_value, previous_value, threshold, min_interval, max_interval):
change_rate = abs(current_value - previous_value)
if change_rate > threshold:
return min_interval
else:
return max_interval
Data Aggregation Algorithms
Data aggregation algorithms combine data from multiple sensors to reduce the amount of information transmitted through the network, saving energy and bandwidth.
Example: Cluster-based Data Aggregation
In cluster-based data aggregation, sensor nodes are grouped into clusters. Each cluster has a cluster head that aggregates data from its members before sending it to the base station.
def cluster_aggregation(cluster_data):
aggregated_data = {
"avg": sum(cluster_data) / len(cluster_data),
"max": max(cluster_data),
"min": min(cluster_data)
}
return aggregated_data
2. Network Communication and Routing Algorithms
Topology Control Algorithms
Topology control algorithms manage the network structure to optimize communication efficiency and energy consumption.
Example: Minimum Spanning Tree (MST) Algorithm
The MST algorithm creates an optimal tree-like structure that connects all nodes with the minimum total edge weight, reducing energy consumption in communication.
def kruskal_mst(graph):
edges = sorted(graph.edges, key=lambda x: x[2])
parent = {node: node for node in graph.nodes}
mst = []
def find(node):
if parent[node] != node:
parent[node] = find(parent[node])
return parent[node]
def union(u, v):
root_u, root_v = find(u), find(v)
parent[root_u] = root_v
for u, v, weight in edges:
if find(u) != find(v):
union(u, v)
mst.append((u, v, weight))
return mst
Routing Algorithms
Routing algorithms determine the optimal path for data to travel from source to destination in a sensor network or IoT system.
Example: Low-Energy Adaptive Clustering Hierarchy (LEACH)
LEACH is a popular routing protocol for wireless sensor networks that uses clustering to distribute energy consumption evenly among sensor nodes.
import random
def leach_cluster_head_selection(nodes, p):
cluster_heads = []
for node in nodes:
if random.random() < p:
cluster_heads.append(node)
return cluster_heads
def leach_routing(nodes, cluster_heads):
clusters = {ch: [] for ch in cluster_heads}
for node in nodes:
if node not in cluster_heads:
nearest_ch = min(cluster_heads, key=lambda ch: distance(node, ch))
clusters[nearest_ch].append(node)
return clusters
3. Energy Management Algorithms
Sleep Scheduling Algorithms
Sleep scheduling algorithms manage the active and sleep states of sensor nodes to conserve energy while maintaining network coverage and connectivity.
Example: Randomized Independent Scheduling (RIS)
RIS is a simple yet effective sleep scheduling algorithm where each node independently decides whether to be active or sleep based on a probability.
import random
def ris_sleep_schedule(nodes, active_probability):
active_nodes = []
for node in nodes:
if random.random() < active_probability:
active_nodes.append(node)
return active_nodes
Energy-Aware Routing Algorithms
Energy-aware routing algorithms consider the remaining energy of nodes when making routing decisions to prolong the network lifetime.
Example: Maximum Residual Energy Path Routing
This algorithm selects the path with the maximum residual energy to route data, balancing energy consumption across the network.
def max_residual_energy_path(graph, source, destination):
def path_energy(path):
return min(graph.nodes[node]['energy'] for node in path)
def dfs(node, path):
if node == destination:
return path
max_energy_path = None
max_energy = float('-inf')
for neighbor in graph.neighbors(node):
if neighbor not in path:
new_path = dfs(neighbor, path + [neighbor])
if new_path:
path_energy_val = path_energy(new_path)
if path_energy_val > max_energy:
max_energy = path_energy_val
max_energy_path = new_path
return max_energy_path
return dfs(source, [source])
4. Security and Privacy Algorithms
Encryption Algorithms
Encryption algorithms protect sensitive data transmitted through sensor networks and IoT devices from unauthorized access.
Example: Advanced Encryption Standard (AES)
AES is a widely used symmetric encryption algorithm that provides strong security for data in transit and at rest.
from Crypto.Cipher import AES
from Crypto.Random import get_random_bytes
def encrypt_aes(data, key):
cipher = AES.new(key, AES.MODE_EAX)
ciphertext, tag = cipher.encrypt_and_digest(data)
return (cipher.nonce, tag, ciphertext)
def decrypt_aes(nonce, tag, ciphertext, key):
cipher = AES.new(key, AES.MODE_EAX, nonce)
data = cipher.decrypt_and_verify(ciphertext, tag)
return data
# Example usage
key = get_random_bytes(16) # 128-bit key
data = b"Sensitive sensor data"
encrypted = encrypt_aes(data, key)
decrypted = decrypt_aes(*encrypted, key)
print(decrypted.decode())
Secure Routing Algorithms
Secure routing algorithms protect the network from various attacks, such as sinkhole attacks, wormhole attacks, and Sybil attacks.
Example: Secure LEACH (S-LEACH)
S-LEACH is an extension of the LEACH protocol that adds security features to protect against various attacks.
import random
import hashlib
def s_leach_cluster_head_selection(nodes, p, round_number, node_ids):
cluster_heads = []
for node in nodes:
node_id = node_ids[node]
hash_input = f"{node_id}:{round_number}"
hash_value = hashlib.sha256(hash_input.encode()).hexdigest()
if int(hash_value, 16) / (2**256) < p:
cluster_heads.append(node)
return cluster_heads
def s_leach_routing(nodes, cluster_heads, node_ids):
clusters = {ch: [] for ch in cluster_heads}
for node in nodes:
if node not in cluster_heads:
nearest_ch = min(cluster_heads, key=lambda ch: distance(node, ch))
clusters[nearest_ch].append(node)
return clusters
5. Decision-Making and Actuation Algorithms
Machine Learning Algorithms
Machine learning algorithms enable IoT devices to make intelligent decisions based on sensor data and learned patterns.
Example: K-Nearest Neighbors (KNN) for Anomaly Detection
KNN can be used to detect anomalies in sensor readings by comparing new data points to historical data.
from sklearn.neighbors import NearestNeighbors
import numpy as np
def knn_anomaly_detection(data, k, threshold):
nn = NearestNeighbors(n_neighbors=k)
nn.fit(data)
distances, _ = nn.kneighbors(data)
avg_distances = np.mean(distances, axis=1)
anomalies = np.where(avg_distances > threshold)[0]
return anomalies
# Example usage
historical_data = np.random.rand(1000, 5) # 1000 samples, 5 features
new_data = np.random.rand(100, 5) # 100 new samples
anomalies = knn_anomaly_detection(historical_data, k=5, threshold=0.5)
print(f"Detected {len(anomalies)} anomalies")
Fuzzy Logic Algorithms
Fuzzy logic algorithms allow IoT devices to make decisions based on imprecise or uncertain input data.
Example: Fuzzy Logic for Temperature Control
This example demonstrates a simple fuzzy logic system for controlling temperature in an IoT-enabled smart home.
import numpy as np
import skfuzzy as fuzz
from skfuzzy import control as ctrl
# Input variables
temperature = ctrl.Antecedent(np.arange(0, 41, 1), 'temperature')
humidity = ctrl.Antecedent(np.arange(0, 101, 1), 'humidity')
# Output variable
ac_power = ctrl.Consequent(np.arange(0, 101, 1), 'ac_power')
# Fuzzy sets
temperature['cold'] = fuzz.trimf(temperature.universe, [0, 0, 20])
temperature['comfortable'] = fuzz.trimf(temperature.universe, [15, 22, 28])
temperature['hot'] = fuzz.trimf(temperature.universe, [25, 40, 40])
humidity['dry'] = fuzz.trimf(humidity.universe, [0, 0, 40])
humidity['normal'] = fuzz.trimf(humidity.universe, [30, 50, 70])
humidity['humid'] = fuzz.trimf(humidity.universe, [60, 100, 100])
ac_power['low'] = fuzz.trimf(ac_power.universe, [0, 0, 50])
ac_power['medium'] = fuzz.trimf(ac_power.universe, [25, 50, 75])
ac_power['high'] = fuzz.trimf(ac_power.universe, [50, 100, 100])
# Fuzzy rules
rule1 = ctrl.Rule(temperature['hot'] | humidity['humid'], ac_power['high'])
rule2 = ctrl.Rule(temperature['comfortable'] & humidity['normal'], ac_power['medium'])
rule3 = ctrl.Rule(temperature['cold'] | humidity['dry'], ac_power['low'])
# Control system
ac_ctrl = ctrl.ControlSystem([rule1, rule2, rule3])
ac_simulation = ctrl.ControlSystemSimulation(ac_ctrl)
# Example usage
ac_simulation.input['temperature'] = 30
ac_simulation.input['humidity'] = 60
ac_simulation.compute()
print(f"AC Power: {ac_simulation.output['ac_power']:.2f}%")
Applications of Algorithms in Sensor Networks and IoT
The algorithms discussed above find applications in various domains, including:
- Smart Cities: Traffic management, waste management, and urban planning
- Environmental Monitoring: Air quality monitoring, wildlife tracking, and natural disaster prediction
- Healthcare: Remote patient monitoring, elderly care, and epidemic tracking
- Industrial IoT: Predictive maintenance, supply chain optimization, and asset tracking
- Smart Agriculture: Precision farming, livestock monitoring, and crop yield optimization
- Smart Homes: Energy management, security systems, and home automation
Challenges and Future Directions
While algorithms have greatly advanced the capabilities of sensor networks and IoT devices, several challenges remain:
- Energy Efficiency: Developing more energy-efficient algorithms to extend the battery life of sensor nodes and IoT devices
- Scalability: Creating algorithms that can handle the exponential growth of connected devices
- Security and Privacy: Enhancing security measures to protect against evolving cyber threats and ensure user privacy
- Real-time Processing: Developing algorithms that can process and analyze data in real-time for time-sensitive applications
- Interoperability: Designing algorithms that can work across different platforms and protocols to enable seamless integration of diverse IoT devices
Future research in this field is likely to focus on:
- Edge computing algorithms to reduce latency and bandwidth usage
- AI and machine learning algorithms for more intelligent decision-making
- Blockchain-based algorithms for enhanced security and decentralized control
- Quantum algorithms for solving complex optimization problems in large-scale IoT networks
Conclusion
Algorithms are the backbone of sensor networks and IoT devices, enabling efficient data collection, processing, and decision-making. As these technologies continue to evolve and permeate various aspects of our lives, the importance of developing robust, efficient, and secure algorithms cannot be overstated.
By understanding and implementing these algorithms, developers and engineers can create more intelligent, energy-efficient, and secure IoT systems. This knowledge is crucial for anyone looking to enter the field of IoT development or enhance their existing skills in this rapidly growing domain.
As we move towards an increasingly connected world, the role of algorithms in sensor networks and IoT devices will only grow in importance. By staying up-to-date with the latest developments in this field and continuously improving our algorithmic skills, we can contribute to shaping a smarter, more efficient, and more sustainable future.