Algorithms in Robotics: Pathfinding and Navigation
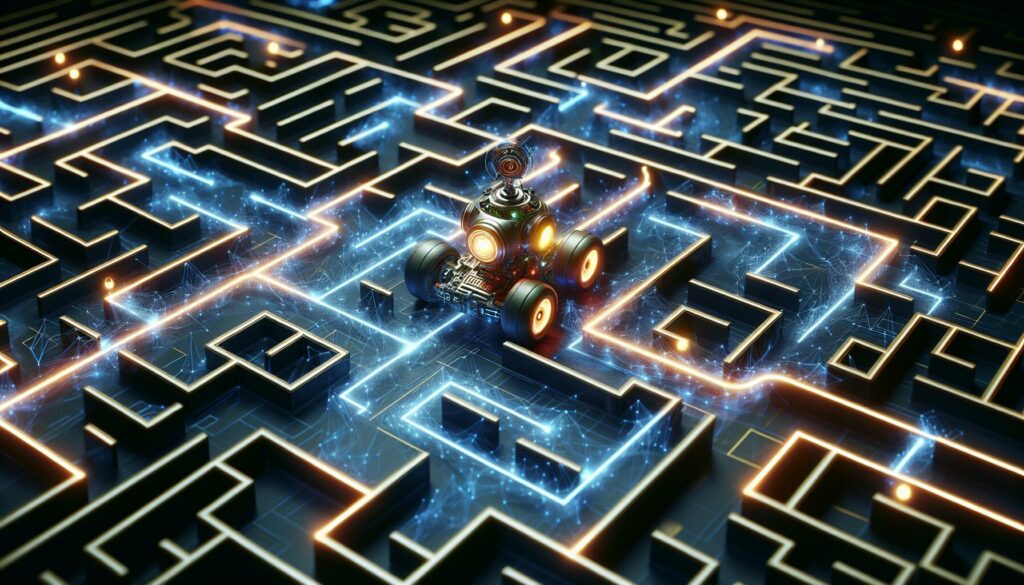
In the ever-evolving world of robotics, the ability for machines to navigate their environment efficiently and safely is paramount. This capability relies heavily on sophisticated algorithms for pathfinding and navigation. As we delve into this fascinating topic, we’ll explore how these algorithms work, their applications in various robotic systems, and how they contribute to the broader field of artificial intelligence and automation.
Understanding Pathfinding and Navigation in Robotics
Pathfinding and navigation are fundamental components of robotics that enable autonomous machines to move from one point to another while avoiding obstacles and optimizing their route. These processes involve several key elements:
- Environment mapping
- Localization
- Path planning
- Obstacle avoidance
- Real-time decision making
Each of these elements requires sophisticated algorithms to process sensor data, make calculations, and execute actions. Let’s explore some of the most common algorithms used in robotic pathfinding and navigation.
Popular Pathfinding Algorithms in Robotics
1. A* (A-Star) Algorithm
The A* algorithm is one of the most widely used pathfinding algorithms in robotics due to its efficiency and flexibility. It combines the benefits of Dijkstra’s algorithm (which finds the shortest path) and Best-First Search (which uses heuristics to guide the search).
Here’s a basic implementation of the A* algorithm in Python:
import heapq
def heuristic(a, b):
return abs(b[0] - a[0]) + abs(b[1] - a[1])
def a_star(grid, start, goal):
neighbors = [(0,1),(0,-1),(1,0),(-1,0),(1,1),(1,-1),(-1,1),(-1,-1)]
close_set = set()
came_from = {}
gscore = {start:0}
fscore = {start:heuristic(start, goal)}
oheap = []
heapq.heappush(oheap, (fscore[start], start))
while oheap:
current = heapq.heappop(oheap)[1]
if current == goal:
path = []
while current in came_from:
path.append(current)
current = came_from[current]
path.append(start)
path.reverse()
return path
close_set.add(current)
for i, j in neighbors:
neighbor = current[0] + i, current[1] + j
tentative_g_score = gscore[current] + heuristic(current, neighbor)
if 0 <= neighbor[0] < len(grid) and 0 <= neighbor[1] < len(grid[0]):
if grid[neighbor[0]][neighbor[1]] == 1:
continue
else:
continue
if neighbor in close_set and tentative_g_score >= gscore.get(neighbor, 0):
continue
if tentative_g_score < gscore.get(neighbor, 0) or neighbor not in [i[1]for i in oheap]:
came_from[neighbor] = current
gscore[neighbor] = tentative_g_score
fscore[neighbor] = gscore[neighbor] + heuristic(neighbor, goal)
heapq.heappush(oheap, (fscore[neighbor], neighbor))
return False
This implementation uses a grid-based environment, where 0 represents free space and 1 represents obstacles. The algorithm finds the shortest path from the start point to the goal point, avoiding obstacles along the way.
2. Dijkstra’s Algorithm
Dijkstra’s algorithm is another fundamental pathfinding algorithm that finds the shortest path between nodes in a graph. While it’s less efficient than A* for most robotics applications, it’s still widely used, especially in scenarios where the heuristic function for A* is difficult to define.
Here’s a simple implementation of Dijkstra’s algorithm:
import heapq
def dijkstra(graph, start, end):
distances = {node: float('infinity') for node in graph}
distances[start] = 0
pq = [(0, start)]
previous = {node: None for node in graph}
while pq:
current_distance, current_node = heapq.heappop(pq)
if current_node == end:
path = []
while current_node:
path.append(current_node)
current_node = previous[current_node]
return path[::-1]
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
previous[neighbor] = current_node
heapq.heappush(pq, (distance, neighbor))
return None
This implementation uses a graph represented as a dictionary of dictionaries, where each key is a node, and its value is another dictionary representing its neighbors and the weights of the edges connecting them.
3. Rapidly-exploring Random Tree (RRT)
The RRT algorithm is particularly useful for robotics applications in high-dimensional spaces or environments with complex obstacles. It works by randomly sampling points in the configuration space and connecting them to the nearest existing point in the tree.
Here’s a basic implementation of the RRT algorithm:
import random
import math
class Node:
def __init__(self, x, y):
self.x = x
self.y = y
self.parent = None
def distance(node1, node2):
return math.sqrt((node1.x - node2.x)**2 + (node1.y - node2.y)**2)
def nearest_node(nodes, point):
return min(nodes, key=lambda node: distance(node, point))
def is_collision_free(node1, node2, obstacles):
# Implement collision detection here
return True
def rrt(start, goal, obstacles, max_iterations=1000, step_size=0.1):
nodes = [start]
for _ in range(max_iterations):
random_point = Node(random.uniform(0, 1), random.uniform(0, 1))
nearest = nearest_node(nodes, random_point)
theta = math.atan2(random_point.y - nearest.y, random_point.x - nearest.x)
new_node = Node(nearest.x + step_size * math.cos(theta),
nearest.y + step_size * math.sin(theta))
if is_collision_free(nearest, new_node, obstacles):
new_node.parent = nearest
nodes.append(new_node)
if distance(new_node, goal) < step_size:
goal.parent = new_node
return nodes + [goal]
return None
This implementation creates a tree of nodes in a 2D space, avoiding obstacles (not implemented in this basic version) and attempting to reach the goal point.
Navigation Algorithms in Robotics
While pathfinding algorithms focus on finding a route from one point to another, navigation algorithms deal with the broader task of guiding a robot through its environment. This includes localization (determining the robot’s position), mapping (building a representation of the environment), and motion planning (deciding how to move).
1. Simultaneous Localization and Mapping (SLAM)
SLAM is a crucial algorithm in robotics that allows a robot to construct a map of an unknown environment while simultaneously keeping track of its location within it. This is particularly useful for robots operating in new or changing environments.
Here’s a simplified example of a basic SLAM algorithm:
import numpy as np
class SimpleSlam:
def __init__(self, map_size):
self.map = np.zeros(map_size)
self.position = np.array([0, 0])
self.orientation = 0
def move(self, distance, angle):
self.orientation += angle
self.position += distance * np.array([np.cos(self.orientation), np.sin(self.orientation)])
def observe(self, measurements):
for distance, angle in measurements:
x = int(self.position[0] + distance * np.cos(self.orientation + angle))
y = int(self.position[1] + distance * np.sin(self.orientation + angle))
if 0 <= x < self.map.shape[0] and 0 <= y < self.map.shape[1]:
self.map[x, y] = 1
def update(self, movement, measurements):
self.move(*movement)
self.observe(measurements)
# Usage
slam = SimpleSlam((100, 100))
slam.update((1, 0), [(5, 0), (4, np.pi/4), (6, -np.pi/4)])
print(slam.map)
This simple SLAM implementation maintains a binary occupancy grid as its map, updates the robot’s position based on movement commands, and updates the map based on sensor measurements.
2. Potential Field Algorithm
The Potential Field algorithm is a reactive navigation method that treats the robot as a particle under the influence of an artificial potential field. The goal attracts the robot (like a gravitational force), while obstacles repel it (like an electrostatic force).
Here’s a basic implementation of the Potential Field algorithm:
import numpy as np
def attractive_potential(position, goal, a=1):
return 0.5 * a * np.sum((position - goal)**2)
def repulsive_potential(position, obstacle, b=1, q_star=1):
d = np.linalg.norm(position - obstacle)
if d <= q_star:
return 0.5 * b * (1/d - 1/q_star)**2
else:
return 0
def total_potential(position, goal, obstacles, a=1, b=1, q_star=1):
u_att = attractive_potential(position, goal, a)
u_rep = sum(repulsive_potential(position, obs, b, q_star) for obs in obstacles)
return u_att + u_rep
def potential_field_planner(start, goal, obstacles, step_size=0.1, max_iterations=1000):
path = [start]
position = start
for _ in range(max_iterations):
if np.linalg.norm(position - goal) < step_size:
return path
# Calculate the negative gradient of the potential field
grad = np.zeros(2)
eps = 1e-5
for i in range(2):
e = np.zeros(2)
e[i] = eps
grad[i] = (total_potential(position + e, goal, obstacles) -
total_potential(position - e, goal, obstacles)) / (2 * eps)
# Move in the direction of the negative gradient
position = position - step_size * grad / np.linalg.norm(grad)
path.append(position)
return path
# Usage
start = np.array([0, 0])
goal = np.array([10, 10])
obstacles = [np.array([5, 5]), np.array([7, 7])]
path = potential_field_planner(start, goal, obstacles)
print(path)
This implementation calculates the total potential field as the sum of attractive and repulsive potentials, then uses gradient descent to find a path to the goal.
Applications of Pathfinding and Navigation Algorithms in Robotics
The algorithms we’ve discussed have a wide range of applications in robotics, including:
- Autonomous Vehicles: Self-driving cars use a combination of these algorithms to navigate roads, avoid obstacles, and reach their destination safely.
- Warehouse Robots: In large warehouses, robots use pathfinding algorithms to efficiently navigate between shelves and pick up or deliver items.
- Drone Navigation: Drones use these algorithms for tasks ranging from package delivery to search and rescue operations.
- Robot Vacuum Cleaners: These household robots use navigation algorithms to efficiently clean floors while avoiding obstacles.
- Mars Rovers: NASA’s Mars rovers use sophisticated navigation algorithms to explore the Martian surface autonomously.
Challenges and Future Directions
While current pathfinding and navigation algorithms have enabled significant advancements in robotics, several challenges remain:
- Dynamic Environments: Many algorithms struggle in rapidly changing environments. Developing algorithms that can quickly adapt to changes is an active area of research.
- Scalability: As robots are deployed in larger and more complex environments, ensuring that algorithms can scale efficiently becomes crucial.
- Multi-Robot Systems: Coordinating navigation among multiple robots introduces additional complexity that current algorithms don’t always handle well.
- Energy Efficiency: For mobile robots with limited battery life, finding paths that minimize energy consumption is becoming increasingly important.
- Ethical Navigation: As robots interact more with humans, incorporating ethical considerations into navigation decisions (e.g., prioritizing human safety) is a growing concern.
Future research in this field is likely to focus on addressing these challenges, as well as integrating machine learning techniques to improve the adaptability and efficiency of navigation algorithms.
Conclusion
Pathfinding and navigation algorithms are the unsung heroes of modern robotics, enabling machines to move through the world with increasing autonomy and intelligence. From the A* algorithm guiding a robot through a maze, to SLAM allowing a drone to map an unknown environment, these algorithms are fundamental to the functioning of countless robotic systems.
As we continue to push the boundaries of what’s possible in robotics, these algorithms will undoubtedly evolve, becoming more sophisticated, efficient, and adaptable. The future of robotics is bright, and it’s paved with intelligent pathfinding and navigation algorithms.
Whether you’re a budding roboticist, a seasoned programmer, or simply curious about the inner workings of the autonomous machines around us, understanding these algorithms provides valuable insight into the complex world of robotics. As we’ve seen, many of these algorithms can be implemented with relatively simple code, opening up a world of possibilities for experimentation and innovation.
So the next time you see a robot vacuum navigating around your furniture, or hear about a self-driving car successfully completing a journey, you’ll have a deeper appreciation for the clever algorithms working behind the scenes, guiding these machines on their way.