Algorithms in Distributed Computing Environments: Powering Modern Tech
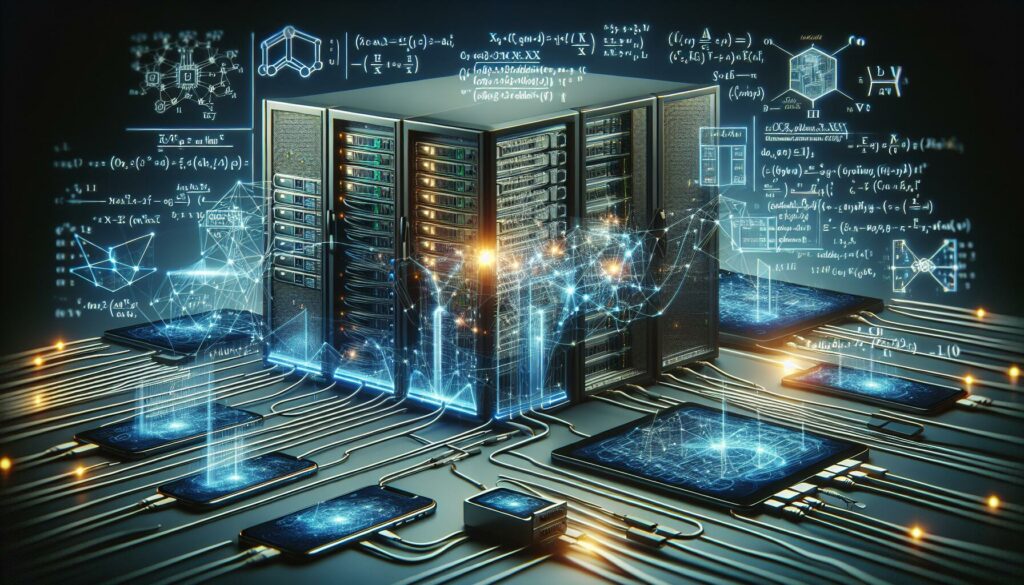
In today’s interconnected world, distributed computing has become the backbone of many technological advancements. From cloud services to blockchain networks, distributed systems are everywhere. At the heart of these complex systems lie powerful algorithms that enable efficient communication, coordination, and problem-solving across multiple nodes. In this comprehensive guide, we’ll explore the fascinating world of algorithms in distributed computing environments, their importance, and how they’re shaping the future of technology.
Understanding Distributed Computing
Before diving into the algorithms, let’s first understand what distributed computing is and why it’s so crucial in modern tech landscapes.
What is Distributed Computing?
Distributed computing refers to a model in which components of a software system are shared among multiple computers to improve efficiency and performance. Instead of all the computation happening on a single machine, the workload is distributed across a network of interconnected computers, each playing a part in the overall process.
Why is Distributed Computing Important?
Distributed computing offers several advantages over traditional centralized systems:
- Scalability: Distributed systems can easily scale by adding more nodes to the network.
- Fault Tolerance: If one node fails, the system can continue to operate using the remaining nodes.
- Resource Sharing: It allows for efficient sharing of resources like processing power and storage.
- Improved Performance: Tasks can be executed in parallel, leading to faster completion times.
Key Algorithms in Distributed Computing
Now that we understand the basics, let’s explore some of the fundamental algorithms that power distributed computing environments.
1. Consensus Algorithms
Consensus algorithms are crucial for ensuring that all nodes in a distributed system agree on a single data value or state, even in the presence of failures.
Paxos Algorithm
Paxos is a family of protocols for solving consensus in a network of unreliable processors. It ensures that a distributed system can continue to operate correctly as long as a majority of its processors are working.
// Simplified Paxos pseudocode
function propose(value):
// Phase 1a: Prepare
send prepare(n) to all acceptors
if majority respond with promise(n, accepted_value):
// Phase 2a: Accept
send accept(n, value) to all acceptors
if majority respond with accepted(n):
return value
else:
return failure
Raft Algorithm
Raft is designed to be more understandable than Paxos while providing the same guarantees. It separates key elements of consensus such as leader election, log replication, and safety.
// Simplified Raft leader election pseudocode
function start_election():
current_term += 1
voted_for = self
reset_election_timer()
send request_vote to all other servers
if receive votes from majority:
become_leader()
else:
return to follower state
2. Distributed Hash Tables (DHTs)
DHTs are a class of decentralized distributed systems that provide a lookup service similar to a hash table. They are fundamental to many peer-to-peer systems.
Chord Protocol
Chord is a protocol and algorithm for a peer-to-peer distributed hash table. It specifies how keys are assigned to nodes and how a node can discover the value for a given key by communicating with a few other nodes.
// Chord finger table update pseudocode
function update_finger_table(node):
for i in range(m): // m is the number of bits in the key/node identifiers
finger[i] = find_successor((n + 2^i) mod 2^m)
notify(successor)
3. Leader Election Algorithms
Leader election is the process of designating a single process as the organizer of some task distributed among several computers (nodes).
Bully Algorithm
The Bully algorithm is used to elect a coordinator in a distributed system. When a node notices that the leader is no longer responding, it initiates an election.
// Bully Algorithm pseudocode
function start_election():
for node in higher_priority_nodes:
send election_message to node
if no response within timeout:
become_leader()
send coordinator_message to all nodes
else:
wait for coordinator_message
4. Clock Synchronization Algorithms
In distributed systems, maintaining a consistent view of time across all nodes is crucial for many operations.
Network Time Protocol (NTP)
NTP is widely used to synchronize computer clocks over packet-switched, variable-latency data networks.
// Simplified NTP client pseudocode
function synchronize_time():
server_time = request_time_from_server()
round_trip_delay = (receive_time - send_time) - server_processing_time
offset = ((server_time - send_time) + (server_time - receive_time)) / 2
adjust_local_clock(offset)
5. Gossip Protocols
Gossip protocols (also known as epidemic protocols) are a class of communication protocols inspired by the way social networks disseminate information.
// Basic Gossip Protocol pseudocode
function gossip(message):
while true:
random_peer = select_random_peer()
send message to random_peer
wait for some time
if received new information:
update local state
Challenges in Distributed Algorithms
While distributed algorithms offer numerous benefits, they also come with their own set of challenges:
1. Network Partitions
Network partitions occur when a network is split into isolated sub-networks, often due to network failures. This can lead to inconsistencies in distributed systems.
2. Byzantine Faults
Byzantine faults refer to arbitrary behavior by faulty nodes, including malicious actions. Algorithms must be designed to handle such scenarios.
3. Scalability
As the number of nodes in a distributed system grows, ensuring efficient communication and coordination becomes increasingly challenging.
4. Consistency vs. Availability
The CAP theorem states that it’s impossible for a distributed data store to simultaneously provide more than two out of the following three guarantees: Consistency, Availability, and Partition tolerance.
Implementing Distributed Algorithms: Best Practices
When implementing distributed algorithms, consider the following best practices:
1. Use Idempotent Operations
Idempotent operations can be applied multiple times without changing the result beyond the initial application. This is crucial in distributed systems where messages may be duplicated or reordered.
// Example of an idempotent operation in Python
def set_max_value(current_max, new_value):
return max(current_max, new_value)
2. Implement Proper Error Handling
In distributed systems, failures are common. Implement robust error handling and retry mechanisms.
// Retry mechanism pseudocode
function perform_operation_with_retry(max_retries):
retries = 0
while retries < max_retries:
try:
result = perform_operation()
return result
catch Error as e:
retries += 1
wait(exponential_backoff(retries))
throw MaxRetriesExceeded
3. Use Versioning
Implement versioning for data to handle conflicts and ensure consistency.
// Versioned data structure example
class VersionedData:
def __init__(self, value):
self.value = value
self.version = 0
def update(self, new_value):
self.value = new_value
self.version += 1
4. Implement Proper Logging and Monitoring
Comprehensive logging and monitoring are essential for debugging and maintaining distributed systems.
// Logging example in Python
import logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
def perform_critical_operation():
logger.info("Starting critical operation")
# ... operation code ...
logger.info("Critical operation completed")
The Future of Distributed Computing Algorithms
As technology continues to evolve, so do the algorithms that power distributed computing. Here are some trends shaping the future:
1. Quantum Distributed Computing
Quantum computing principles are being applied to distributed systems, potentially revolutionizing fields like cryptography and optimization.
2. AI-Driven Distributed Systems
Machine learning algorithms are being integrated into distributed systems to improve decision-making, resource allocation, and fault prediction.
3. Edge Computing Algorithms
With the rise of IoT and edge devices, new algorithms are being developed to efficiently process data at the network edge.
4. Blockchain and Distributed Ledger Technologies
These technologies are driving innovation in consensus algorithms and distributed data storage.
Conclusion
Algorithms in distributed computing environments form the backbone of modern technology infrastructure. From ensuring consensus among nodes to efficiently routing data across vast networks, these algorithms enable the scalable, fault-tolerant systems we rely on every day.
As aspiring software engineers and computer scientists, understanding these algorithms is crucial. They not only provide insights into how large-scale systems operate but also offer valuable lessons in problem-solving, system design, and algorithmic thinking.
The field of distributed computing is constantly evolving, presenting exciting opportunities for innovation and research. Whether you’re preparing for technical interviews at top tech companies or looking to contribute to cutting-edge distributed systems, mastering these algorithms will undoubtedly give you a competitive edge.
Remember, the journey to becoming proficient in distributed algorithms is ongoing. Continuous learning, practical implementation, and staying updated with the latest developments in the field are key to success. Happy coding, and may your distributed systems always reach consensus!