Algorithms in Aerospace and Navigation Systems: Powering the Future of Flight
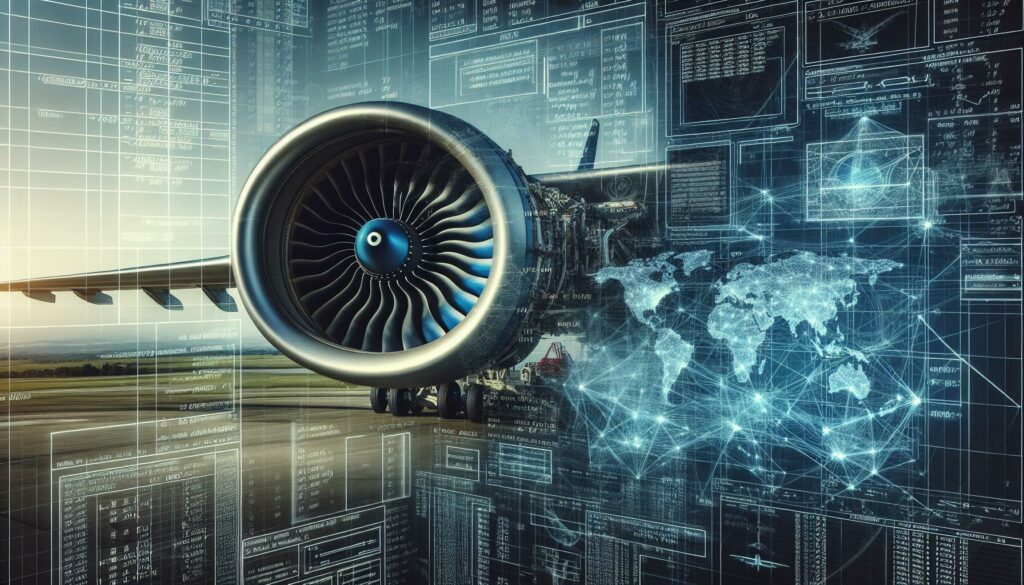
In the vast expanse of the sky and the infinite reaches of space, algorithms play a crucial role in guiding aircraft and spacecraft safely to their destinations. The field of aerospace and navigation systems relies heavily on complex mathematical calculations and sophisticated software to ensure precision, efficiency, and safety in air and space travel. In this comprehensive guide, we’ll explore the fascinating world of algorithms in aerospace and navigation systems, their applications, and their impact on modern aviation and space exploration.
1. Introduction to Aerospace Algorithms
Aerospace algorithms are specialized sets of instructions designed to solve problems and optimize processes in aviation and space technology. These algorithms are the backbone of modern navigation systems, flight control systems, and mission planning tools. They enable everything from the smooth takeoff and landing of commercial airliners to the precise maneuvering of satellites in orbit.
1.1 The Importance of Algorithms in Aerospace
Algorithms in aerospace serve several critical functions:
- Navigation and route planning
- Flight control and stability
- Collision avoidance
- Fuel optimization
- Weather prediction and analysis
- Mission planning for space exploration
As the complexity of aerospace systems increases, so does the sophistication of the algorithms that power them. Let’s delve deeper into some of the key areas where algorithms make a significant impact.
2. Navigation Algorithms
Navigation is at the heart of aerospace operations. Whether it’s a commercial airliner flying across continents or a spacecraft navigating the solar system, accurate navigation is crucial for mission success and safety.
2.1 Global Positioning System (GPS) Algorithms
GPS is a satellite-based navigation system that provides location and time information in all weather conditions. The algorithms used in GPS processing are complex and involve several steps:
- Trilateration: Determining position based on the distance from multiple satellites
- Kalman filtering: Reducing errors and noise in GPS signals
- Ionospheric correction: Accounting for signal delays caused by the ionosphere
Here’s a simplified example of how trilateration works in Python:
import numpy as np
def trilateration(satellite_positions, distances):
A = 2 * (satellite_positions[1:] - satellite_positions[0])
b = distances[1:]**2 - distances[0]**2 - np.sum(satellite_positions[1:]**2 - satellite_positions[0]**2, axis=1)
return np.linalg.lstsq(A, b, rcond=None)[0]
# Example usage
satellite_positions = np.array([[0, 0, 0], [1, 0, 0], [0, 1, 0], [0, 0, 1]])
distances = np.array([1, 1, 1, 1])
position = trilateration(satellite_positions, distances)
print(f"Estimated position: {position}")
2.2 Inertial Navigation System (INS) Algorithms
INS uses accelerometers and gyroscopes to continuously calculate the position, orientation, and velocity of an aircraft or spacecraft without external references. Key algorithms in INS include:
- Attitude estimation algorithms
- Velocity and position integration algorithms
- Error correction and sensor fusion algorithms
2.3 Path Planning Algorithms
Path planning algorithms are essential for finding optimal routes for aircraft and spacecraft. Some popular algorithms include:
- A* (A-star) algorithm for finding the shortest path
- Rapidly-exploring Random Trees (RRT) for real-time path planning in complex environments
- Dijkstra’s algorithm for finding the shortest path in a weighted graph
Here’s a basic implementation of the A* algorithm in Python:
import heapq
def heuristic(a, b):
return abs(b[0] - a[0]) + abs(b[1] - a[1])
def a_star(grid, start, goal):
neighbors = [(0,1),(0,-1),(1,0),(-1,0)]
close_set = set()
came_from = {}
gscore = {start:0}
fscore = {start:heuristic(start, goal)}
open_set = []
heapq.heappush(open_set, (fscore[start], start))
while open_set:
current = heapq.heappop(open_set)[1]
if current == goal:
path = []
while current in came_from:
path.append(current)
current = came_from[current]
path.append(start)
path.reverse()
return path
close_set.add(current)
for i, j in neighbors:
neighbor = current[0] + i, current[1] + j
tentative_g_score = gscore[current] + 1
if 0 <= neighbor[0] < len(grid) and 0 <= neighbor[1] < len(grid[0]):
if grid[neighbor[0]][neighbor[1]] == 1:
continue
else:
continue
if neighbor in close_set and tentative_g_score >= gscore.get(neighbor, 0):
continue
if tentative_g_score < gscore.get(neighbor, 0) or neighbor not in [i[1]for i in open_set]:
came_from[neighbor] = current
gscore[neighbor] = tentative_g_score
fscore[neighbor] = gscore[neighbor] + heuristic(neighbor, goal)
heapq.heappush(open_set, (fscore[neighbor], neighbor))
return False
# Example usage
grid = [[0, 0, 0, 0, 1],
[1, 1, 0, 1, 0],
[0, 0, 0, 0, 0],
[0, 1, 1, 1, 0],
[0, 0, 0, 1, 0]]
start = (0, 0)
goal = (4, 4)
path = a_star(grid, start, goal)
print(f"Path found: {path}")
3. Flight Control Algorithms
Flight control algorithms are responsible for maintaining the stability and control of aircraft and spacecraft. These algorithms process data from various sensors and adjust control surfaces to ensure safe and efficient flight.
3.1 Stability Augmentation Systems (SAS)
SAS algorithms improve the stability characteristics of an aircraft by providing artificial damping. They typically involve:
- Pitch damping
- Yaw damping
- Roll damping
3.2 Fly-by-Wire Systems
Fly-by-wire systems use computers to process pilot inputs and control the aircraft. Key algorithms in fly-by-wire systems include:
- Control law algorithms
- Envelope protection algorithms
- Fault detection and isolation algorithms
3.3 Autopilot Algorithms
Autopilot systems use a combination of algorithms to maintain flight parameters and navigate the aircraft. These include:
- PID (Proportional-Integral-Derivative) control algorithms
- Altitude hold algorithms
- Heading hold algorithms
- Waypoint navigation algorithms
Here’s a simple implementation of a PID controller in Python:
class PIDController:
def __init__(self, kp, ki, kd):
self.kp = kp
self.ki = ki
self.kd = kd
self.previous_error = 0
self.integral = 0
def compute(self, setpoint, measured_value, dt):
error = setpoint - measured_value
self.integral += error * dt
derivative = (error - self.previous_error) / dt
output = self.kp * error + self.ki * self.integral + self.kd * derivative
self.previous_error = error
return output
# Example usage
pid = PIDController(1.0, 0.1, 0.05)
setpoint = 100
measured_value = 0
dt = 0.1
for _ in range(100):
control_output = pid.compute(setpoint, measured_value, dt)
# In a real system, you would apply this control output to your actuators
measured_value += control_output * dt
print(f"Measured value: {measured_value:.2f}")
4. Collision Avoidance Algorithms
Collision avoidance is critical for the safety of air and space travel. Advanced algorithms are used to predict and prevent potential collisions.
4.1 Traffic Collision Avoidance System (TCAS)
TCAS is an aircraft collision avoidance system that monitors the airspace around an aircraft for other aircraft equipped with a corresponding active transponder. TCAS algorithms involve:
- Threat detection algorithms
- Resolution advisory algorithms
- Coordination algorithms between aircraft
4.2 Sense and Avoid Algorithms for Unmanned Aerial Vehicles (UAVs)
UAVs require sophisticated algorithms to detect and avoid obstacles autonomously. These algorithms often use a combination of sensors and computer vision techniques:
- Obstacle detection algorithms
- Path replanning algorithms
- Risk assessment algorithms
5. Fuel Optimization Algorithms
Fuel efficiency is a major concern in aerospace, both for economic and environmental reasons. Sophisticated algorithms are used to optimize fuel consumption.
5.1 Flight Profile Optimization
These algorithms determine the most fuel-efficient flight profile, considering factors such as:
- Altitude
- Speed
- Weather conditions
- Air traffic
5.2 Real-time Fuel Optimization
During flight, real-time optimization algorithms continuously adjust flight parameters to minimize fuel consumption. These algorithms often use machine learning techniques to improve over time.
6. Weather Prediction and Analysis Algorithms
Accurate weather prediction is crucial for safe and efficient aerospace operations. Weather algorithms process vast amounts of data to provide accurate forecasts.
6.1 Numerical Weather Prediction (NWP) Models
NWP models use complex algorithms to simulate atmospheric processes and predict future weather conditions. Key components include:
- Data assimilation algorithms
- Atmospheric physics algorithms
- Statistical post-processing algorithms
6.2 Turbulence Detection Algorithms
Algorithms for detecting and predicting turbulence help improve flight safety and comfort. These often involve:
- Pattern recognition algorithms
- Machine learning algorithms for turbulence prediction
7. Space Navigation and Mission Planning Algorithms
Space navigation presents unique challenges that require specialized algorithms.
7.1 Orbital Mechanics Algorithms
These algorithms calculate and predict the motion of objects in space, considering factors such as:
- Gravitational forces
- Atmospheric drag
- Solar radiation pressure
7.2 Interplanetary Navigation Algorithms
For deep space missions, algorithms must account for the complex gravitational interactions between celestial bodies. Key techniques include:
- Lambert’s problem solvers for orbit determination
- Gravity assist maneuver optimization
- Trajectory correction maneuver planning
7.3 Autonomous Navigation for Space Exploration
As spacecraft venture farther into space, autonomous navigation becomes increasingly important. These algorithms often involve:
- Star tracking algorithms for attitude determination
- Terrain relative navigation for planetary landings
- Simultaneous localization and mapping (SLAM) for rover navigation
8. Machine Learning in Aerospace Algorithms
Machine learning is increasingly being applied to aerospace algorithms, enhancing their capabilities and enabling new applications.
8.1 Predictive Maintenance
Machine learning algorithms analyze sensor data to predict when aircraft components are likely to fail, allowing for proactive maintenance.
8.2 Autonomous Flight Control
Reinforcement learning algorithms are being developed to create more adaptive and robust flight control systems.
8.3 Anomaly Detection
Machine learning algorithms can detect unusual patterns in flight data, potentially identifying safety issues before they become critical.
9. Challenges and Future Directions
As aerospace technology continues to advance, algorithms face new challenges and opportunities:
9.1 Increasing Complexity
As aerospace systems become more complex, algorithms must be able to handle increasingly large and diverse datasets while maintaining real-time performance.
9.2 Cybersecurity
With the increasing reliance on digital systems, developing robust algorithms for cybersecurity in aerospace is crucial.
9.3 Integration of Unmanned Systems
As unmanned aerial vehicles become more prevalent, algorithms must be developed to safely integrate them into existing air traffic systems.
9.4 Quantum Computing
The advent of quantum computing may revolutionize aerospace algorithms, particularly in areas such as optimization and cryptography.
Conclusion
Algorithms are the unsung heroes of aerospace and navigation systems, working tirelessly behind the scenes to ensure the safety, efficiency, and reliability of air and space travel. From the complex calculations that guide spacecraft through the solar system to the real-time decisions that keep commercial airliners on course, these mathematical marvels are pushing the boundaries of what’s possible in aerospace technology.
As we look to the future, the role of algorithms in aerospace will only grow more significant. With advancements in artificial intelligence, quantum computing, and other cutting-edge technologies, we can expect to see even more sophisticated algorithms that will enable new capabilities in aviation and space exploration.
Whether you’re a student of aerospace engineering, a software developer working in the aviation industry, or simply someone fascinated by the technology that powers flight, understanding the algorithms behind aerospace and navigation systems provides a window into the incredible complexity and ingenuity of modern aerospace technology. As we continue to reach for the stars, it’s clear that algorithms will be our steadfast companions, guiding us safely through the vast expanses of air and space.