Algorithms for Social Network Analysis: Unraveling the Web of Connections
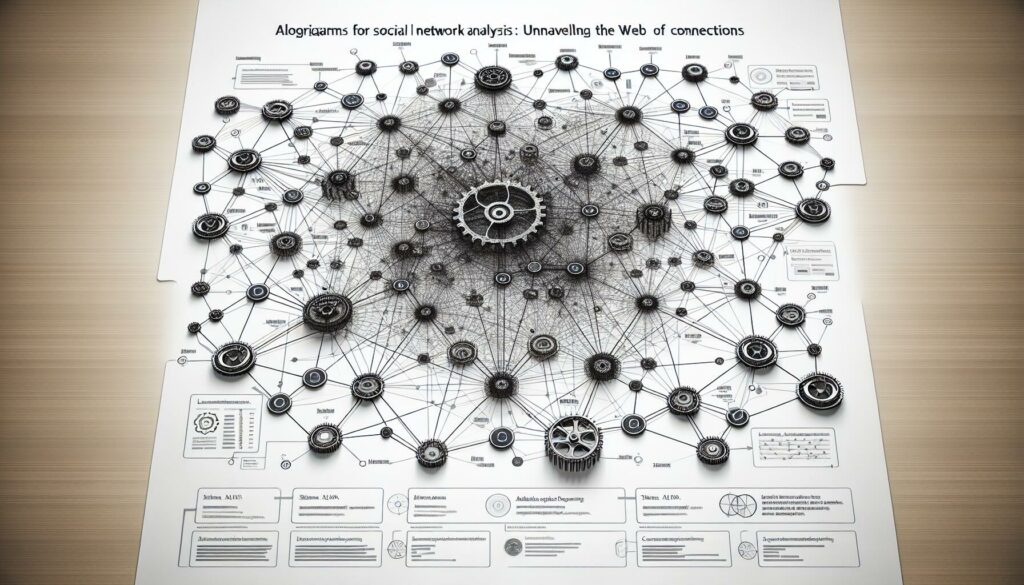
In today’s interconnected world, social networks have become an integral part of our daily lives. From Facebook and Twitter to LinkedIn and Instagram, these platforms have revolutionized the way we communicate, share information, and build relationships. Behind the scenes, complex algorithms power these networks, enabling them to function efficiently and provide valuable insights into human behavior and social dynamics. In this comprehensive guide, we’ll explore the fascinating world of algorithms for social network analysis, their applications, and their impact on our digital landscape.
Understanding Social Network Analysis
Before diving into the algorithms, it’s essential to grasp the concept of social network analysis (SNA). SNA is the process of investigating social structures through the use of network and graph theories. It characterizes networked structures in terms of nodes (individual actors, people, or things within the network) and the ties, edges, or links (relationships or interactions) that connect them.
Social network analysis has applications in various fields, including:
- Sociology
- Psychology
- Anthropology
- Computer Science
- Economics
- Biology
- Information Science
- Marketing
By analyzing social networks, researchers and data scientists can uncover patterns of interaction, identify influential individuals, detect communities, and predict the spread of information or behaviors within a network.
Key Concepts in Social Network Analysis
Before we delve into specific algorithms, let’s familiarize ourselves with some fundamental concepts in social network analysis:
1. Nodes and Edges
In a social network graph, nodes represent individual entities (such as people, organizations, or web pages), while edges represent the relationships or interactions between these entities.
2. Directed vs. Undirected Graphs
In directed graphs, edges have a specific direction, indicating a one-way relationship (e.g., person A follows person B on Twitter). Undirected graphs have bidirectional edges, representing mutual relationships (e.g., friendship on Facebook).
3. Weighted Graphs
Weighted graphs assign values to edges, representing the strength or importance of relationships (e.g., frequency of communication between two individuals).
4. Centrality
Centrality measures help identify the most important or influential nodes in a network based on various criteria, such as connectivity, position, or information flow.
5. Community Detection
Community detection algorithms aim to identify groups of nodes that are more densely connected to each other than to the rest of the network.
Essential Algorithms for Social Network Analysis
Now that we’ve covered the basics, let’s explore some of the most important algorithms used in social network analysis:
1. Breadth-First Search (BFS)
Breadth-First Search is a fundamental graph traversal algorithm that explores a graph level by level. In social network analysis, BFS is often used to find the shortest path between two nodes or to calculate the degrees of separation between individuals.
Here’s a simple implementation of BFS in Python:
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
visited.add(start)
while queue:
vertex = queue.popleft()
print(vertex, end=" ")
for neighbor in graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
print("BFS traversal:")
bfs(graph, 'A')
2. Depth-First Search (DFS)
Depth-First Search is another graph traversal algorithm that explores as far as possible along each branch before backtracking. DFS is useful for detecting cycles in a graph, finding connected components, and solving problems like maze generation.
Here’s a simple implementation of DFS in Python:
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start, end=" ")
for neighbor in graph[start]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
print("DFS traversal:")
dfs(graph, 'A')
3. PageRank
PageRank is a famous algorithm originally developed by Google’s co-founders to rank web pages in search results. In social network analysis, PageRank can be used to measure the importance or influence of nodes in a network based on the structure of incoming links.
Here’s a simplified implementation of the PageRank algorithm in Python:
import numpy as np
def pagerank(graph, damping_factor=0.85, epsilon=1e-8, max_iterations=100):
num_nodes = len(graph)
matrix = np.zeros((num_nodes, num_nodes))
for i, neighbors in enumerate(graph):
for neighbor in neighbors:
matrix[neighbor, i] = 1 / len(neighbors)
matrix = matrix.T
pagerank_vector = np.ones(num_nodes) / num_nodes
for _ in range(max_iterations):
prev_pagerank = pagerank_vector.copy()
pagerank_vector = (1 - damping_factor) / num_nodes + damping_factor * matrix.dot(prev_pagerank)
if np.sum(np.abs(pagerank_vector - prev_pagerank)) < epsilon:
break
return pagerank_vector
# Example usage
graph = [
[1, 2], # Node 0 links to nodes 1 and 2
[2, 3], # Node 1 links to nodes 2 and 3
[3], # Node 2 links to node 3
[0, 1] # Node 3 links to nodes 0 and 1
]
pagerank_scores = pagerank(graph)
for i, score in enumerate(pagerank_scores):
print(f"Node {i}: {score:.4f}")
4. Betweenness Centrality
Betweenness centrality is a measure of centrality based on shortest paths. For each pair of nodes in a network, there exists at least one shortest path between them, and the betweenness centrality of a node is the number of these shortest paths that pass through the node.
Here’s a Python implementation of the betweenness centrality algorithm using NetworkX:
import networkx as nx
def betweenness_centrality(graph):
G = nx.Graph(graph)
betweenness = nx.betweenness_centrality(G)
return betweenness
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
centrality_scores = betweenness_centrality(graph)
for node, score in centrality_scores.items():
print(f"Node {node}: {score:.4f}")
5. Louvain Method for Community Detection
The Louvain method is a popular algorithm for detecting communities in large networks. It optimizes modularity, a measure of the strength of division of a network into communities.
Here’s an example of using the Louvain method with the python-louvain library:
import community
import networkx as nx
def louvain_community_detection(graph):
G = nx.Graph(graph)
partition = community.best_partition(G)
return partition
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
communities = louvain_community_detection(graph)
for node, community_id in communities.items():
print(f"Node {node}: Community {community_id}")
Applications of Social Network Analysis Algorithms
The algorithms we’ve discussed have numerous practical applications in various fields:
1. Influence Marketing
By identifying influential nodes in a social network, companies can target their marketing efforts more effectively. Algorithms like PageRank and betweenness centrality can help identify key influencers who have the potential to spread information or promote products to a wider audience.
2. Recommendation Systems
Social network analysis algorithms can be used to improve recommendation systems by considering the preferences and behaviors of connected individuals. For example, Netflix and Amazon use these algorithms to suggest movies, TV shows, or products based on your connections and their preferences.
3. Fraud Detection
By analyzing patterns of connections and interactions in financial networks, algorithms can help detect suspicious activities or potential fraud. Unusual patterns in transaction networks or sudden changes in connectivity can be indicators of fraudulent behavior.
4. Epidemiology
Social network analysis plays a crucial role in understanding the spread of diseases. By modeling social interactions and applying algorithms like BFS or DFS, researchers can simulate the propagation of infections and evaluate the effectiveness of intervention strategies.
5. Organizational Analysis
Companies can use social network analysis to understand the flow of information within their organization, identify key personnel, and optimize team structures. Community detection algorithms can reveal informal groups or departments that may not be apparent in the official organizational chart.
Challenges and Ethical Considerations
While social network analysis algorithms offer powerful insights, they also come with challenges and ethical considerations:
1. Privacy Concerns
The collection and analysis of social network data raise significant privacy concerns. It’s crucial to ensure that personal information is protected and that individuals have control over their data.
2. Data Quality and Bias
The accuracy of social network analysis depends on the quality and completeness of the underlying data. Incomplete or biased data can lead to misleading results and reinforce existing societal biases.
3. Scalability
As social networks grow larger, the computational complexity of many algorithms increases significantly. Developing scalable algorithms for massive networks remains an ongoing challenge.
4. Interpretability
The results of complex network analysis algorithms can be difficult to interpret, especially for non-experts. Improving the explainability of these algorithms is crucial for their wider adoption and trustworthiness.
5. Ethical Use
The insights gained from social network analysis can be powerful but must be used responsibly. There’s a need for clear guidelines and regulations to prevent misuse or manipulation of social network data.
Future Trends in Social Network Analysis
As technology continues to evolve, so do the algorithms and techniques for social network analysis. Some emerging trends include:
1. Dynamic Network Analysis
Developing algorithms that can analyze and predict changes in network structures over time is becoming increasingly important. This includes studying the evolution of communities, the spread of information, and the emergence of new connections.
2. Multi-layer Network Analysis
Real-world networks often consist of multiple interconnected layers (e.g., different types of relationships or communication channels). Algorithms that can analyze these complex, multi-layer networks are an active area of research.
3. Integration with Machine Learning
Combining social network analysis with machine learning techniques, such as graph neural networks, is opening up new possibilities for more accurate predictions and deeper insights into network dynamics.
4. Privacy-Preserving Algorithms
As privacy concerns grow, there’s an increasing focus on developing algorithms that can analyze social networks while preserving individual privacy. Techniques like differential privacy and federated learning are being explored in this context.
5. Real-time Analysis
With the increasing velocity of data generation in social networks, there’s a growing need for algorithms that can perform analysis in real-time, enabling faster decision-making and more responsive systems.
Conclusion
Algorithms for social network analysis have become indispensable tools in our increasingly connected world. From uncovering hidden patterns in human interactions to powering recommendation systems and fighting the spread of misinformation, these algorithms play a crucial role in shaping our digital landscape.
As we’ve explored in this article, there’s a rich variety of algorithms available for different aspects of social network analysis, each with its own strengths and applications. Whether you’re a data scientist, a sociologist, or simply curious about the underlying mechanics of social networks, understanding these algorithms can provide valuable insights into the complex web of human connections that surrounds us.
As technology continues to evolve, we can expect even more sophisticated algorithms and techniques to emerge, offering deeper insights and new capabilities in social network analysis. However, it’s crucial to approach this field with a balanced perspective, considering both the immense potential and the ethical implications of these powerful analytical tools.
By responsibly harnessing the power of social network analysis algorithms, we can gain a better understanding of our interconnected world, make more informed decisions, and potentially address some of the most pressing challenges facing our global society.