Algorithms for Real-Time Systems: Ensuring Timely and Reliable Performance
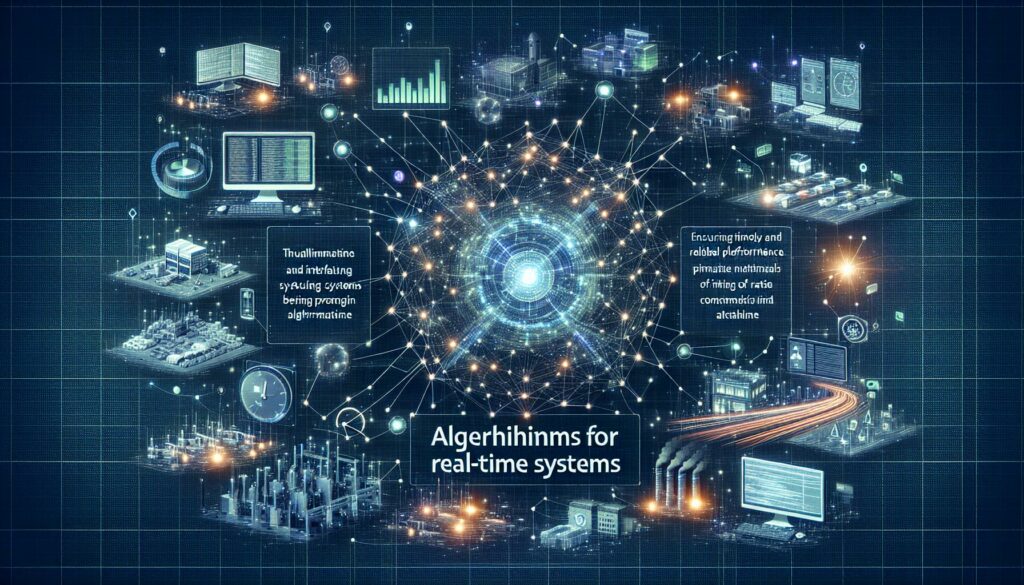
In the fast-paced world of modern computing, real-time systems play a crucial role in numerous applications, from avionics and automotive control to industrial automation and multimedia streaming. These systems are characterized by their need to respond to events and complete tasks within strict time constraints. To meet these demanding requirements, specialized algorithms have been developed to ensure timely and reliable performance. In this comprehensive guide, we’ll explore the intricacies of algorithms for real-time systems, their importance, and how they contribute to the efficiency and safety of critical applications.
Understanding Real-Time Systems
Before diving into the algorithms, it’s essential to grasp the concept of real-time systems and their unique characteristics:
- Time-Constrained: Real-time systems must complete tasks within specific time limits, often referred to as deadlines.
- Predictability: The system’s behavior should be deterministic, ensuring consistent and reliable performance.
- Concurrency: Multiple tasks often need to be executed simultaneously, requiring efficient resource management.
- Fault Tolerance: Real-time systems must be robust and capable of handling unexpected events or failures.
Real-time systems are typically classified into two categories:
- Hard Real-Time Systems: These systems have strict deadlines that must be met under all circumstances. Missing a deadline can lead to catastrophic consequences (e.g., aircraft control systems).
- Soft Real-Time Systems: While these systems also have deadlines, occasional missed deadlines are tolerable and may only result in degraded performance (e.g., video streaming).
Key Algorithms for Real-Time Systems
Now that we understand the basics of real-time systems, let’s explore some of the fundamental algorithms used to ensure their efficient operation:
1. Rate Monotonic Scheduling (RMS)
Rate Monotonic Scheduling is a fixed-priority preemptive scheduling algorithm widely used in real-time systems. It assigns priorities to tasks based on their periods, with shorter periods receiving higher priorities.
Key Features:
- Optimal for systems with fixed-period tasks
- Easy to implement and analyze
- Guarantees schedulability if CPU utilization is below a certain threshold
Implementation Example:
struct Task {
int period;
int execution_time;
int priority;
};
void rate_monotonic_scheduling(Task tasks[], int n) {
// Sort tasks by period (ascending order)
sort(tasks, tasks + n, [](const Task &a, const Task &b) {
return a.period < b.period;
});
// Assign priorities
for (int i = 0; i < n; i++) {
tasks[i].priority = n - i;
}
}
2. Earliest Deadline First (EDF)
Earliest Deadline First is a dynamic priority scheduling algorithm that assigns priorities based on the urgency of task deadlines. The task with the earliest deadline always receives the highest priority.
Key Features:
- Optimal for both periodic and aperiodic tasks
- Can achieve 100% CPU utilization in certain conditions
- More flexible than RMS but potentially more complex to implement
Implementation Example:
struct Task {
int deadline;
int execution_time;
int priority;
};
void earliest_deadline_first(Task tasks[], int n, int current_time) {
// Sort tasks by deadline (ascending order)
sort(tasks, tasks + n, [](const Task &a, const Task &b) {
return a.deadline < b.deadline;
});
// Assign priorities
for (int i = 0; i < n; i++) {
tasks[i].priority = n - i;
}
}
3. Deadline Monotonic Scheduling (DMS)
Deadline Monotonic Scheduling is similar to RMS but assigns priorities based on relative deadlines instead of periods. It’s particularly useful when task deadlines are shorter than their periods.
Key Features:
- Optimal for systems with fixed relative deadlines
- Can handle tasks with deadlines different from their periods
- Generalizes RMS for more flexible scheduling scenarios
Implementation Example:
struct Task {
int deadline;
int period;
int execution_time;
int priority;
};
void deadline_monotonic_scheduling(Task tasks[], int n) {
// Sort tasks by deadline (ascending order)
sort(tasks, tasks + n, [](const Task &a, const Task &b) {
return a.deadline < b.deadline;
});
// Assign priorities
for (int i = 0; i < n; i++) {
tasks[i].priority = n - i;
}
}
4. Least Slack Time First (LST)
Least Slack Time First is a dynamic priority algorithm that assigns priorities based on the slack time of tasks. Slack time is the difference between the time remaining until the deadline and the remaining execution time.
Key Features:
- Highly responsive to dynamic changes in task execution times
- Can achieve high CPU utilization
- More complex to implement and analyze compared to RMS or EDF
Implementation Example:
struct Task {
int deadline;
int remaining_execution_time;
int priority;
};
void least_slack_time_first(Task tasks[], int n, int current_time) {
// Calculate slack time and sort tasks
sort(tasks, tasks + n, [current_time](const Task &a, const Task &b) {
int slack_a = (a.deadline - current_time) - a.remaining_execution_time;
int slack_b = (b.deadline - current_time) - b.remaining_execution_time;
return slack_a < slack_b;
});
// Assign priorities
for (int i = 0; i < n; i++) {
tasks[i].priority = n - i;
}
}
Advanced Algorithms and Techniques
While the algorithms mentioned above form the foundation of real-time scheduling, several advanced techniques have been developed to address more complex scenarios and improve system performance:
1. Mixed Criticality Scheduling
Mixed criticality systems involve tasks with different levels of importance or criticality. Scheduling algorithms for these systems must ensure that high-criticality tasks meet their deadlines while maximizing the performance of lower-criticality tasks.
Key Concepts:
- Multiple execution time estimates for tasks based on criticality levels
- Dynamic adjustment of task priorities and execution times
- Mode changes to handle overload situations
2. Multiprocessor Scheduling
As multi-core processors become more prevalent, algorithms for scheduling real-time tasks across multiple processors have gained importance. These algorithms must consider task allocation, migration, and load balancing to maximize system utilization and meet timing constraints.
Popular Multiprocessor Scheduling Algorithms:
- Global EDF (G-EDF)
- Partitioned EDF (P-EDF)
- Clustered scheduling
3. Energy-Aware Scheduling
For battery-powered devices and energy-constrained systems, energy-aware scheduling algorithms aim to minimize power consumption while still meeting real-time constraints. These algorithms often leverage techniques such as Dynamic Voltage and Frequency Scaling (DVFS) to optimize energy usage.
Key Techniques:
- Task splitting and merging
- Processor speed adjustment
- Sleep state management
4. Feedback Control Scheduling
Feedback control scheduling applies control theory principles to real-time scheduling, allowing for dynamic adaptation to changing system conditions. This approach can help maintain desired performance metrics such as CPU utilization or deadline miss ratios.
Components of Feedback Control Scheduling:
- Reference input (desired performance)
- Feedback loop (monitoring actual performance)
- Controller (adjusting scheduling parameters)
Implementing Real-Time Algorithms: Best Practices and Considerations
When implementing algorithms for real-time systems, several best practices and considerations should be kept in mind to ensure reliable and efficient operation:
1. Worst-Case Execution Time (WCET) Analysis
Accurate estimation of task execution times is crucial for real-time scheduling. WCET analysis techniques help determine the maximum time a task may take to execute, ensuring that schedulability analysis is based on realistic assumptions.
WCET Analysis Techniques:
- Static analysis of code paths
- Measurement-based approaches
- Hybrid methods combining static and dynamic analysis
2. Handling Aperiodic and Sporadic Tasks
Real-time systems often need to handle both periodic tasks and aperiodic or sporadic events. Techniques such as aperiodic servers (e.g., polling server, deferrable server) can be used to integrate these tasks into the scheduling framework without compromising the predictability of periodic tasks.
3. Resource Sharing and Priority Inversion
When tasks share resources, priority inversion can occur, potentially leading to missed deadlines. Protocols such as Priority Inheritance Protocol (PIP) and Priority Ceiling Protocol (PCP) can be implemented to mitigate these issues and ensure predictable resource access.
4. Overload Management
Real-time systems must be designed to handle overload situations gracefully. Techniques such as task shedding, mode changes, and adaptive scheduling can help maintain system stability and ensure that critical tasks continue to meet their deadlines even under high load conditions.
5. Fault Tolerance and Error Handling
Implementing robust error detection and recovery mechanisms is essential for real-time systems, especially in safety-critical applications. Techniques such as watchdog timers, redundancy, and graceful degradation can help maintain system integrity in the face of faults or unexpected events.
Real-World Applications of Real-Time Algorithms
The algorithms and techniques discussed in this article find application in a wide range of real-world systems:
1. Automotive Systems
Modern vehicles rely heavily on real-time systems for functions such as engine control, anti-lock braking, and advanced driver assistance systems (ADAS). These systems often employ a mix of scheduling algorithms to handle tasks with different criticality levels and timing requirements.
2. Avionics
Aircraft control systems are prime examples of hard real-time systems where meeting deadlines is crucial for safety. Rate Monotonic Scheduling and its variants are commonly used in avionics software to ensure predictable and timely execution of critical tasks.
3. Industrial Automation
Manufacturing and process control systems require real-time responsiveness to maintain production quality and safety. Algorithms such as EDF and LST are often employed to handle dynamic task sets and optimize resource utilization in these environments.
4. Multimedia and Gaming
While not always hard real-time, multimedia applications and video games benefit from soft real-time scheduling to maintain smooth playback and responsive user interactions. Techniques such as frame-based scheduling and adaptive algorithms are commonly used in these domains.
5. Robotics
Robotic systems, from industrial manipulators to autonomous vehicles, rely on real-time algorithms to process sensor data, make decisions, and control actuators within tight timing constraints. Mixed criticality scheduling is often employed to balance safety-critical tasks with less critical functionalities.
Conclusion
Algorithms for real-time systems form the backbone of many critical applications in our modern world. From the foundational scheduling algorithms like Rate Monotonic Scheduling and Earliest Deadline First to advanced techniques such as mixed criticality scheduling and feedback control, these algorithms ensure that time-sensitive tasks are executed reliably and efficiently.
As technology continues to advance, the importance of real-time systems is only set to grow. The Internet of Things (IoT), edge computing, and autonomous systems are all areas where real-time performance is crucial. Understanding and implementing these algorithms effectively will be essential for developers and engineers working on the next generation of time-critical applications.
By mastering the principles and techniques discussed in this article, you’ll be well-equipped to tackle the challenges of real-time system design and implementation. Whether you’re working on safety-critical embedded systems or optimizing performance in multimedia applications, the knowledge of real-time algorithms will prove invaluable in creating robust, efficient, and responsive systems.
Remember that while these algorithms provide powerful tools for real-time scheduling, their effective application requires careful analysis of system requirements, thorough testing, and ongoing optimization. As you delve deeper into the world of real-time systems, continue to explore new research and emerging techniques in this rapidly evolving field.