Algorithms for Memory-Constrained Devices: Optimizing Performance in Limited Environments
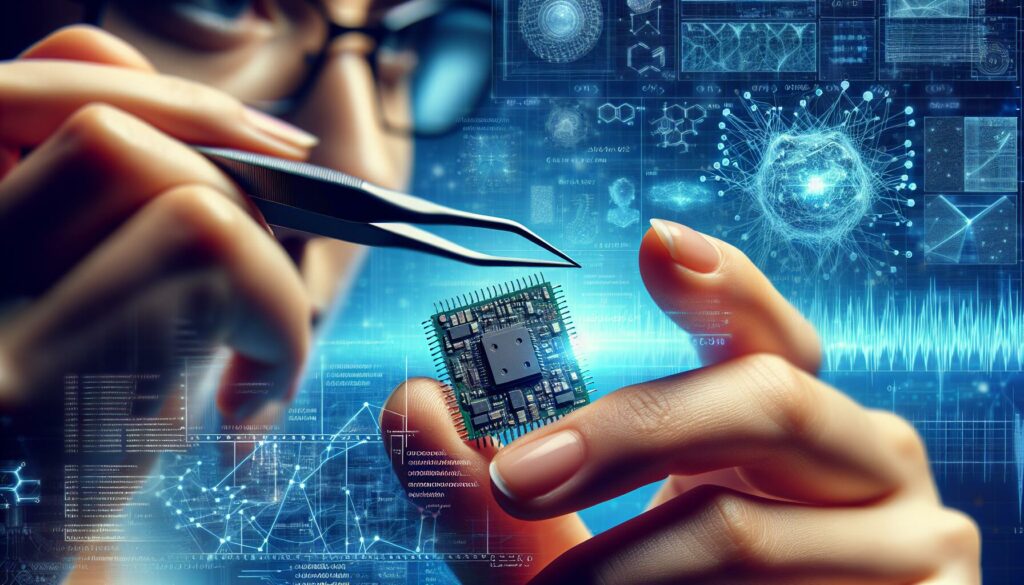
In today’s rapidly evolving technological landscape, developers face unique challenges when creating applications for memory-constrained devices. These devices, ranging from Internet of Things (IoT) sensors to wearable technology, often have limited processing power and storage capabilities. As a result, it’s crucial to implement efficient algorithms that can perform complex tasks while minimizing memory usage. This article will explore various algorithms and techniques specifically designed for memory-constrained environments, providing valuable insights for developers working on such platforms.
Understanding Memory-Constrained Devices
Before diving into specific algorithms, it’s essential to understand what we mean by memory-constrained devices. These are typically embedded systems or small-scale computing devices with limited RAM and storage capacity. Examples include:
- IoT sensors and actuators
- Wearable devices (e.g., fitness trackers, smartwatches)
- Industrial control systems
- Automotive embedded systems
- Low-power microcontrollers
These devices often have memory constraints ranging from a few kilobytes to a few megabytes, making it challenging to implement complex algorithms and data structures commonly used in larger systems.
Key Considerations for Memory-Constrained Algorithms
When developing algorithms for memory-constrained devices, several factors need to be taken into account:
- Memory usage: Algorithms should minimize the amount of RAM required for execution.
- Time complexity: Despite limited resources, algorithms should still maintain reasonable execution times.
- Power consumption: Efficient algorithms can help reduce power consumption, which is crucial for battery-operated devices.
- Scalability: Algorithms should be able to handle varying input sizes without significant performance degradation.
- Simplicity: Simpler algorithms are often easier to implement and maintain on resource-constrained devices.
With these considerations in mind, let’s explore some algorithms and techniques that are well-suited for memory-constrained environments.
1. In-Place Algorithms
In-place algorithms are designed to operate directly on the input data structure without requiring additional memory proportional to the input size. These algorithms are particularly useful in memory-constrained environments as they minimize the overall memory footprint.
Example: In-Place Array Reversal
Consider the problem of reversing an array in-place. Here’s an efficient algorithm that accomplishes this task:
void reverseArray(int arr[], int start, int end) {
while (start < end) {
int temp = arr[start];
arr[start] = arr[end];
arr[end] = temp;
start++;
end--;
}
}
This algorithm uses only a constant amount of extra memory (the temp
variable) regardless of the array size, making it ideal for memory-constrained devices.
Applications of In-Place Algorithms
- Sorting algorithms (e.g., Bubble Sort, Selection Sort)
- Matrix transposition
- String manipulation (e.g., removing duplicates, reversing words)
2. Streaming Algorithms
Streaming algorithms process data in a sequential, incremental manner without storing the entire dataset in memory. These algorithms are particularly useful when dealing with large datasets or continuous streams of data on memory-constrained devices.
Example: Moving Average
Calculating the moving average of a data stream is a common task in signal processing and time series analysis. Here’s a simple streaming algorithm to compute the moving average:
class MovingAverage {
private:
int size;
double sum;
std::queue<int> window;
public:
MovingAverage(int windowSize) : size(windowSize), sum(0.0) {}
double next(int val) {
if (window.size() == size) {
sum -= window.front();
window.pop();
}
window.push(val);
sum += val;
return sum / window.size();
}
};
This algorithm maintains a fixed-size window of the most recent values and updates the average incrementally, using only O(windowSize) memory.
Applications of Streaming Algorithms
- Data sampling and filtering
- Frequency estimation (e.g., Count-Min Sketch)
- Anomaly detection in sensor data
3. Divide and Conquer Algorithms
Divide and conquer algorithms break down complex problems into smaller, manageable subproblems. While not inherently memory-efficient, these algorithms can be adapted for memory-constrained environments by carefully managing recursion and using iterative implementations where possible.
Example: Merge Sort with Limited Memory
Merge Sort is typically not considered memory-efficient due to its need for additional memory during the merge step. However, we can adapt it for memory-constrained devices using an in-place merge technique:
void merge(int arr[], int left, int mid, int right) {
int start2 = mid + 1;
// If the direct merge is already sorted
if (arr[mid] <= arr[start2]) {
return;
}
// Two pointers to maintain start of both arrays to merge
while (left <= mid && start2 <= right) {
// If element 1 is in right place
if (arr[left] <= arr[start2]) {
left++;
}
else {
int value = arr[start2];
int index = start2;
// Shift all the elements between element 1
// element 2, right by 1.
while (index != left) {
arr[index] = arr[index - 1];
index--;
}
arr[left] = value;
// Update all the pointers
left++;
mid++;
start2++;
}
}
}
void mergeSort(int arr[], int left, int right) {
if (left < right) {
int mid = left + (right - left) / 2;
mergeSort(arr, left, mid);
mergeSort(arr, mid + 1, right);
merge(arr, left, mid, right);
}
}
This implementation performs the merge step in-place, significantly reducing the memory overhead compared to the standard Merge Sort algorithm.
Applications of Adapted Divide and Conquer Algorithms
- Sorting large datasets on memory-constrained devices
- Efficient matrix multiplication
- Fast Fourier Transform (FFT) for signal processing
4. Bit Manipulation Techniques
Bit manipulation techniques can be incredibly useful in memory-constrained environments, as they allow for efficient storage and manipulation of data using minimal memory.
Example: Counting Set Bits
Counting the number of set bits (1s) in a binary number is a common operation in low-level programming. Here’s an efficient algorithm using bit manipulation:
int countSetBits(int n) {
int count = 0;
while (n) {
count += n & 1;
n >>= 1;
}
return count;
}
This algorithm uses constant memory and has a time complexity of O(log n), where n is the input number.
Applications of Bit Manipulation Techniques
- Efficient data compression
- Implementing set operations (union, intersection, etc.)
- Low-level device control and register manipulation
5. Dynamic Programming with Space Optimization
Dynamic Programming (DP) is a powerful technique for solving optimization problems. However, traditional DP approaches often require significant memory to store intermediate results. For memory-constrained devices, we can optimize DP algorithms to use minimal space.
Example: Fibonacci Sequence with Constant Space
The Fibonacci sequence is a classic example of dynamic programming. Here’s a space-optimized version that uses constant memory:
int fibonacci(int n) {
if (n <= 1) return n;
int prev = 0, curr = 1;
for (int i = 2; i <= n; i++) {
int next = prev + curr;
prev = curr;
curr = next;
}
return curr;
}
This algorithm calculates the nth Fibonacci number using only O(1) space, regardless of the input size.
Applications of Space-Optimized Dynamic Programming
- Optimal path finding in resource-constrained environments
- Efficient string matching algorithms
- Knapsack problem variants for IoT devices
6. Probabilistic Algorithms
Probabilistic algorithms trade perfect accuracy for improved space and time efficiency. These algorithms are particularly useful in memory-constrained environments where approximate results are acceptable.
Example: Bloom Filter
A Bloom filter is a space-efficient probabilistic data structure used to test whether an element is a member of a set. Here’s a simple implementation:
class BloomFilter {
private:
vector<bool> bitArray;
int size;
vector<function<int(string)>> hashFunctions;
public:
BloomFilter(int size, vector<function<int(string)>> hashes)
: bitArray(size, false), size(size), hashFunctions(hashes) {}
void add(const string& item) {
for (const auto& hashFunc : hashFunctions) {
int index = hashFunc(item) % size;
bitArray[index] = true;
}
}
bool mightContain(const string& item) {
for (const auto& hashFunc : hashFunctions) {
int index = hashFunc(item) % size;
if (!bitArray[index]) return false;
}
return true;
}
};
This Bloom filter implementation uses a fixed amount of memory and provides probabilistic membership testing with a small false positive rate.
Applications of Probabilistic Algorithms
- Network packet filtering and routing
- Duplicate detection in data streams
- Approximate counting in IoT sensor networks
7. Compression Algorithms
Compression algorithms play a crucial role in memory-constrained environments by reducing the storage requirements for data. While some compression algorithms can be complex, there are simpler variants suitable for resource-limited devices.
Example: Run-Length Encoding (RLE)
Run-Length Encoding is a simple form of data compression that replaces consecutive data elements with a single data value and count. Here’s a basic implementation:
string runLengthEncode(const string& input) {
string encoded;
int count = 1;
for (int i = 1; i <= input.length(); i++) {
if (i < input.length() && input[i] == input[i-1]) {
count++;
} else {
encoded += input[i-1] + to_string(count);
count = 1;
}
}
return encoded;
}
This algorithm provides a simple way to compress data with repeated characters, which can be particularly useful for certain types of sensor data or simple image compression.
Applications of Compression Algorithms
- Efficient data storage on IoT devices
- Reducing data transmission costs in sensor networks
- Simple image and audio compression for wearable devices
Best Practices for Implementing Algorithms on Memory-Constrained Devices
When working with memory-constrained devices, it’s important to follow certain best practices to ensure optimal performance and resource utilization:
- Prefer stack allocation over heap allocation: Stack allocation is generally faster and more predictable in terms of memory usage.
- Use static memory allocation when possible: This can help avoid fragmentation issues associated with dynamic memory allocation.
- Minimize global variables: Excessive use of global variables can lead to increased memory usage and potential conflicts.
- Optimize data structures: Choose compact data representations and consider using bit fields for boolean flags.
- Implement efficient memory management: If dynamic allocation is necessary, consider implementing a custom memory allocator tailored to your specific needs.
- Profile and optimize: Use profiling tools to identify memory bottlenecks and optimize critical sections of your code.
- Consider trade-offs: Sometimes, trading a bit of processing time for reduced memory usage can be beneficial in memory-constrained environments.
Conclusion
Developing algorithms for memory-constrained devices presents unique challenges that require careful consideration of resource limitations. By leveraging techniques such as in-place algorithms, streaming approaches, bit manipulation, and space-optimized dynamic programming, developers can create efficient solutions that perform well even in resource-limited environments.
As the Internet of Things and embedded systems continue to proliferate, the demand for memory-efficient algorithms will only increase. By mastering these techniques and staying aware of the latest developments in this field, developers can create powerful applications that push the boundaries of what’s possible on memory-constrained devices.
Remember that the key to success in this domain lies in understanding the specific constraints of your target devices and carefully balancing the trade-offs between memory usage, processing time, and functionality. With the right approach and a solid grasp of these algorithmic techniques, you’ll be well-equipped to tackle the challenges of developing for memory-constrained environments.