Algorithms for Load Balancing in Networks: Optimizing Performance and Reliability
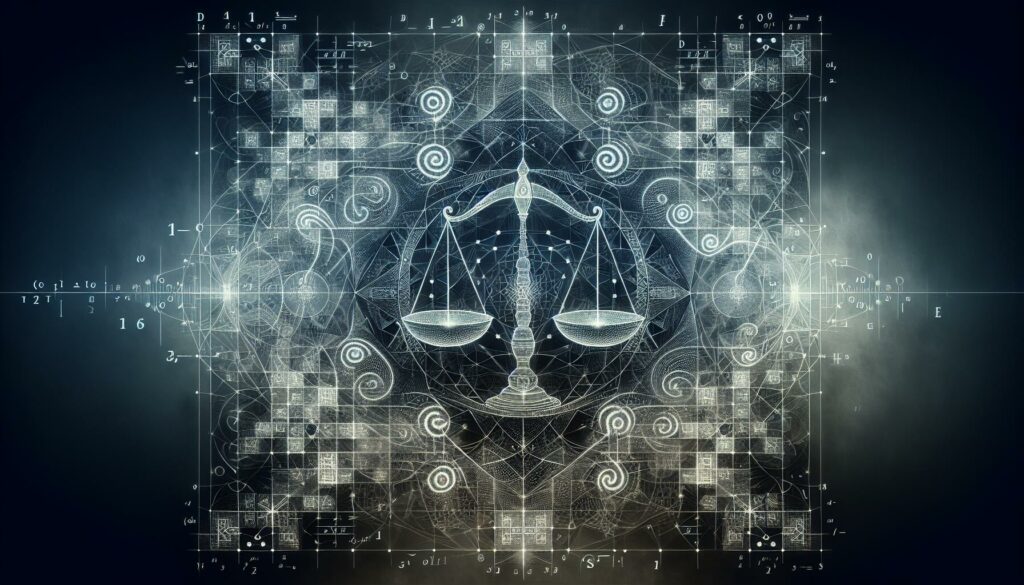
In today’s interconnected world, where digital services and applications are accessed by millions of users simultaneously, the importance of efficient network management cannot be overstated. One crucial aspect of maintaining a robust and responsive network infrastructure is load balancing. Load balancing algorithms play a pivotal role in distributing network traffic across multiple servers or resources, ensuring optimal performance, high availability, and improved user experience. In this comprehensive guide, we’ll delve deep into the world of load balancing algorithms, exploring their significance, types, and implementation strategies in modern networks.
Understanding Load Balancing
Before we dive into the specific algorithms, it’s essential to grasp the concept of load balancing and its importance in network management.
What is Load Balancing?
Load balancing is the process of distributing incoming network traffic across multiple servers or resources to ensure no single server becomes overwhelmed, leading to poor performance or failure. The primary goals of load balancing are:
- Optimizing resource utilization
- Maximizing throughput
- Minimizing response time
- Avoiding overload of any single resource
- Ensuring high availability and reliability
Why is Load Balancing Important?
In the era of cloud computing, microservices, and distributed systems, load balancing has become more critical than ever. Here’s why:
- Scalability: Load balancing allows systems to handle increased traffic by distributing it across multiple servers, enabling horizontal scaling.
- High Availability: By preventing any single point of failure, load balancing ensures that services remain available even if one or more servers fail.
- Improved Performance: Distributing traffic evenly helps maintain consistent performance across all servers, reducing latency and improving user experience.
- Flexibility: Load balancing enables easy addition or removal of servers from the pool, facilitating maintenance and upgrades without service interruption.
- Cost-Effectiveness: By optimizing resource utilization, load balancing can help reduce infrastructure costs and improve energy efficiency.
Types of Load Balancing Algorithms
Load balancing algorithms can be broadly categorized into two main types: static and dynamic. Let’s explore each type and some popular algorithms within these categories.
Static Load Balancing Algorithms
Static algorithms distribute traffic based on predefined rules and do not consider the current state of the servers. These algorithms are simple to implement but may not always provide optimal distribution in dynamic environments.
1. Round Robin
The Round Robin algorithm is one of the simplest and most widely used load balancing techniques. It works by cycling through a list of servers and sending each new request to the next server in line.
class RoundRobinLoadBalancer:
def __init__(self, servers):
self.servers = servers
self.current_index = 0
def get_next_server(self):
server = self.servers[self.current_index]
self.current_index = (self.current_index + 1) % len(self.servers)
return server
Pros:
- Simple to implement and understand
- Fair distribution of requests
Cons:
- Doesn’t consider server capacity or current load
- May not be optimal for servers with different capabilities
2. Weighted Round Robin
The Weighted Round Robin algorithm is an extension of the Round Robin method that assigns a weight to each server based on its capacity. Servers with higher weights receive more requests.
class WeightedRoundRobinLoadBalancer:
def __init__(self, servers, weights):
self.servers = servers
self.weights = weights
self.current_index = 0
self.current_weight = 0
def get_next_server(self):
while True:
self.current_index = (self.current_index + 1) % len(self.servers)
if self.current_index == 0:
self.current_weight -= 1
if self.current_weight <= 0:
self.current_weight = max(self.weights)
if self.weights[self.current_index] >= self.current_weight:
return self.servers[self.current_index]
Pros:
- Considers server capacity
- More flexible than simple Round Robin
Cons:
- Still doesn’t account for real-time server load
- Requires manual configuration of weights
3. IP Hash
The IP Hash algorithm uses the client’s IP address to determine which server should receive the request. This ensures that requests from the same client are always sent to the same server, which can be useful for maintaining session consistency.
import hashlib
class IPHashLoadBalancer:
def __init__(self, servers):
self.servers = servers
def get_server_for_ip(self, ip_address):
hash_value = hashlib.md5(ip_address.encode()).hexdigest()
server_index = int(hash_value, 16) % len(self.servers)
return self.servers[server_index]
Pros:
- Ensures session persistence
- Useful for applications that require sticky sessions
Cons:
- May lead to uneven distribution if client IP ranges are not diverse
- Doesn’t consider server load or capacity
Dynamic Load Balancing Algorithms
Dynamic algorithms take into account the current state of the servers and adjust the distribution of traffic accordingly. These algorithms are more complex but can provide better performance and resource utilization.
4. Least Connection
The Least Connection algorithm directs traffic to the server with the fewest active connections. This approach helps to balance the load more evenly across servers, especially when connection times vary significantly.
class LeastConnectionLoadBalancer:
def __init__(self, servers):
self.servers = {server: 0 for server in servers}
def get_next_server(self):
server = min(self.servers, key=self.servers.get)
self.servers[server] += 1
return server
def release_server(self, server):
self.servers[server] -= 1
Pros:
- Adapts to varying connection times
- Prevents overloading of any single server
Cons:
- Doesn’t consider server capacity differences
- May not be optimal for short-lived connections
5. Least Response Time
The Least Response Time algorithm selects the server with the lowest response time to handle incoming requests. This approach considers both the number of active connections and the server’s response time.
import time
class LeastResponseTimeLoadBalancer:
def __init__(self, servers):
self.servers = {server: {'connections': 0, 'response_time': 0} for server in servers}
def get_next_server(self):
server = min(self.servers, key=lambda s: (self.servers[s]['connections'], self.servers[s]['response_time']))
self.servers[server]['connections'] += 1
return server
def update_response_time(self, server, response_time):
self.servers[server]['response_time'] = response_time
def release_server(self, server):
self.servers[server]['connections'] -= 1
Pros:
- Considers both server load and performance
- Adapts to changing server conditions
Cons:
- Requires continuous monitoring of server response times
- May introduce overhead in calculating response times
6. Resource-Based (CPU/Memory)
Resource-Based algorithms distribute traffic based on the current CPU or memory utilization of servers. This approach ensures that servers are not overloaded and can handle incoming requests efficiently.
class ResourceBasedLoadBalancer:
def __init__(self, servers):
self.servers = {server: {'cpu': 0, 'memory': 0} for server in servers}
def get_next_server(self):
server = min(self.servers, key=lambda s: (self.servers[s]['cpu'], self.servers[s]['memory']))
return server
def update_server_resources(self, server, cpu_usage, memory_usage):
self.servers[server]['cpu'] = cpu_usage
self.servers[server]['memory'] = memory_usage
Pros:
- Considers actual server resource utilization
- Prevents overloading of servers
Cons:
- Requires continuous monitoring of server resources
- May introduce overhead in collecting and processing resource data
Implementing Load Balancing in Networks
Now that we’ve explored various load balancing algorithms, let’s discuss how these algorithms are implemented in real-world network environments.
Hardware vs. Software Load Balancers
Load balancers can be implemented as either hardware appliances or software solutions:
Hardware Load Balancers
Hardware load balancers are dedicated devices designed specifically for load balancing tasks. They often provide high performance and can handle large volumes of traffic.
Pros:
- High performance and low latency
- Purpose-built for load balancing tasks
- Often include advanced features and security capabilities
Cons:
- Higher upfront costs
- Limited scalability
- May require specialized knowledge for configuration and maintenance
Software Load Balancers
Software load balancers are applications that run on standard servers or virtual machines. They offer greater flexibility and can be easily scaled or customized.
Pros:
- Lower cost and greater flexibility
- Easy to scale and customize
- Can be deployed in cloud environments
Cons:
- May have lower performance compared to hardware solutions
- Requires server resources to run
- May require more maintenance and updates
Load Balancing in Cloud Environments
Cloud providers offer load balancing services that can be easily integrated into cloud-based applications. These services often provide a combination of algorithms and features to suit various use cases.
Amazon Web Services (AWS) Elastic Load Balancing
AWS offers three types of load balancers:
- Application Load Balancer (ALB): For HTTP/HTTPS traffic
- Network Load Balancer (NLB): For TCP/UDP traffic
- Classic Load Balancer: Legacy option for EC2-Classic network
These load balancers support various algorithms, including round robin and least outstanding requests.
Google Cloud Load Balancing
Google Cloud provides several load balancing options:
- HTTP(S) Load Balancing: For global HTTP(S) traffic
- TCP/SSL Load Balancing: For global TCP/SSL traffic
- Network Load Balancing: For regional TCP/UDP traffic
Google Cloud load balancers use a combination of algorithms, including round robin and weighted round robin.
Microsoft Azure Load Balancer
Azure Load Balancer offers both public and internal load balancing options. It supports various distribution modes, including:
- Hash-based distribution
- Source IP affinity (session persistence)
- Tupled distribution (5-tuple or 3-tuple)
Implementing Custom Load Balancing Solutions
For organizations with specific requirements or those looking to implement load balancing in on-premises environments, custom solutions can be developed using open-source tools or programming languages.
NGINX as a Load Balancer
NGINX is a popular web server that can also function as a load balancer. Here’s an example of a simple NGINX configuration for load balancing:
http {
upstream backend {
server backend1.example.com;
server backend2.example.com;
server backend3.example.com;
}
server {
listen 80;
location / {
proxy_pass http://backend;
}
}
}
This configuration uses the round robin algorithm by default, but NGINX also supports other algorithms like least connections and IP hash.
HAProxy
HAProxy is another popular open-source load balancer. Here’s an example configuration:
frontend http-in
bind *:80
default_backend servers
backend servers
balance roundrobin
server server1 192.168.1.10:80 check
server server2 192.168.1.11:80 check
server server3 192.168.1.12:80 check
HAProxy supports various algorithms, including round robin, least connections, and source IP hash.
Best Practices for Load Balancing
To ensure optimal performance and reliability when implementing load balancing, consider the following best practices:
- Choose the right algorithm: Select a load balancing algorithm that best suits your application’s needs and traffic patterns.
- Monitor server health: Implement health checks to ensure that traffic is only directed to healthy servers.
- Use SSL offloading: Offload SSL/TLS processing to the load balancer to reduce the computational burden on backend servers.
- Implement session persistence: Use session persistence (sticky sessions) when necessary to maintain user state across requests.
- Scale horizontally: Design your application to scale horizontally, allowing for easy addition of new servers to the load-balanced pool.
- Consider geographic distribution: For global applications, use DNS-based load balancing or content delivery networks (CDNs) to direct users to the nearest data center.
- Implement redundancy: Use multiple load balancers in a high-availability configuration to avoid single points of failure.
- Optimize backend servers: Ensure that backend servers are properly optimized and configured to handle incoming traffic efficiently.
- Monitor and analyze performance: Regularly monitor load balancer and server performance, and analyze logs to identify potential issues or areas for improvement.
- Test and simulate various scenarios: Conduct load testing and simulate different traffic patterns to ensure your load balancing solution can handle expected and unexpected situations.
Conclusion
Load balancing is a critical component of modern network infrastructure, enabling organizations to build scalable, high-performance, and reliable applications. By understanding and implementing appropriate load balancing algorithms, network administrators and developers can ensure optimal resource utilization and improved user experience.
As we’ve explored in this guide, there are various load balancing algorithms available, each with its own strengths and weaknesses. The choice of algorithm depends on factors such as application requirements, traffic patterns, and infrastructure capabilities. Whether you opt for a simple round robin approach or a more sophisticated dynamic algorithm, the key is to continuously monitor and optimize your load balancing strategy to meet the evolving needs of your network and users.
In the context of coding education and programming skills development, understanding load balancing algorithms and their implementation is crucial for aspiring software engineers and system administrators. As applications grow in complexity and scale, the ability to design and manage distributed systems becomes increasingly important. By mastering these concepts, developers can create more resilient and efficient applications that can handle the demands of modern web and cloud environments.
As you continue your journey in software development and system design, remember that load balancing is just one piece of the puzzle in building robust and scalable applications. Stay curious, keep learning, and always strive to optimize your code and infrastructure for the best possible performance and user experience.