Algorithms for Image Processing and Computer Vision: A Comprehensive Guide
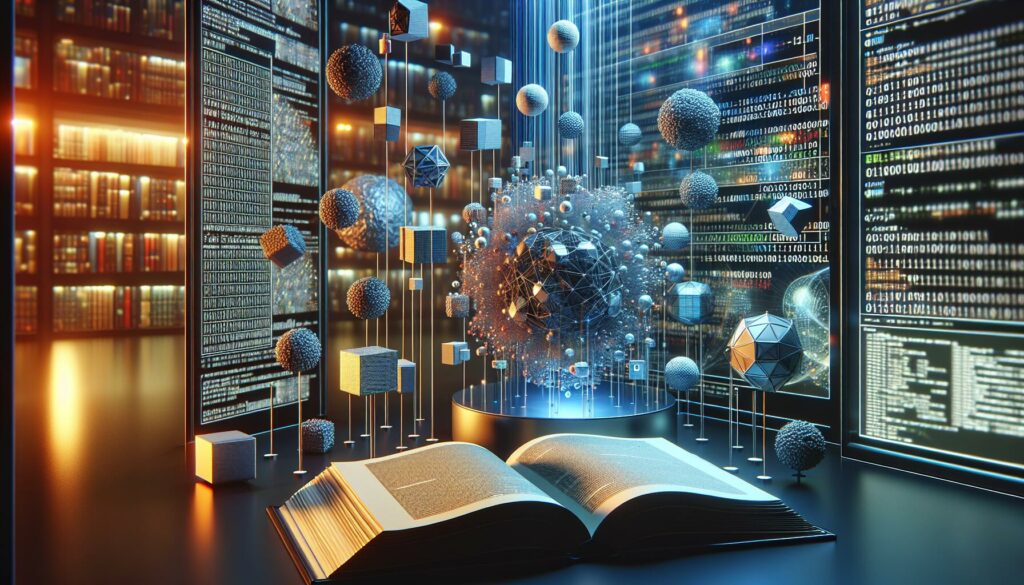
In today’s digital age, image processing and computer vision have become integral parts of numerous applications, from facial recognition systems to autonomous vehicles. As a programmer, understanding the algorithms behind these technologies is crucial for developing cutting-edge solutions. This comprehensive guide will delve into the world of image processing and computer vision algorithms, providing you with the knowledge and tools to tackle complex visual computing challenges.
Table of Contents
- Introduction to Image Processing and Computer Vision
- Basic Image Processing Operations
- Image Filtering Techniques
- Edge Detection Algorithms
- Feature Extraction and Description
- Image Segmentation Algorithms
- Object Detection and Recognition
- Machine Learning in Computer Vision
- Advanced Topics in Computer Vision
- Conclusion and Future Trends
1. Introduction to Image Processing and Computer Vision
Image processing and computer vision are closely related fields that deal with the manipulation and analysis of digital images. While image processing focuses on transforming images to enhance or extract specific information, computer vision aims to interpret and understand the content of images, mimicking human visual perception.
The fundamental building block of digital images is the pixel, which represents the smallest unit of color information. Images are typically stored as 2D arrays of pixel values, with each pixel containing intensity or color information. Understanding this structure is crucial for implementing image processing algorithms effectively.
Key Concepts in Image Processing and Computer Vision:
- Pixel: The basic unit of a digital image
- Resolution: The number of pixels in an image (width x height)
- Color spaces: Different ways to represent color information (e.g., RGB, HSV, CMYK)
- Image histogram: A graphical representation of pixel intensity distribution
- Spatial and frequency domains: Different representations of image information
2. Basic Image Processing Operations
Before diving into more complex algorithms, it’s essential to understand the basic operations that form the foundation of image processing. These operations can be used to manipulate images and prepare them for further analysis.
2.1 Point Operations
Point operations modify individual pixel values without considering neighboring pixels. Some common point operations include:
- Brightness adjustment
- Contrast enhancement
- Thresholding
- Gamma correction
Here’s a simple example of brightness adjustment in Python using the Pillow library:
from PIL import Image
def adjust_brightness(image, factor):
return Image.eval(image, lambda x: x * factor)
# Load an image
img = Image.open("input_image.jpg")
# Adjust brightness
brightened_img = adjust_brightness(img, 1.5)
darkened_img = adjust_brightness(img, 0.7)
# Save the results
brightened_img.save("brightened_image.jpg")
darkened_img.save("darkened_image.jpg")
2.2 Geometric Transformations
Geometric transformations modify the spatial relationship between pixels. Common transformations include:
- Scaling (resizing)
- Rotation
- Translation
- Affine transformations
Here’s an example of image rotation using OpenCV:
import cv2
import numpy as np
def rotate_image(image, angle):
height, width = image.shape[:2]
center = (width // 2, height // 2)
rotation_matrix = cv2.getRotationMatrix2D(center, angle, 1.0)
rotated_image = cv2.warpAffine(image, rotation_matrix, (width, height))
return rotated_image
# Load an image
img = cv2.imread("input_image.jpg")
# Rotate the image by 45 degrees
rotated_img = rotate_image(img, 45)
# Save the result
cv2.imwrite("rotated_image.jpg", rotated_img)
2.3 Histogram Operations
Histogram operations analyze and modify the distribution of pixel intensities in an image. These operations can be used for:
- Histogram equalization
- Histogram matching
- Contrast stretching
Here’s an example of histogram equalization using NumPy and Matplotlib:
import numpy as np
import matplotlib.pyplot as plt
from skimage import io, exposure
def histogram_equalization(image):
return exposure.equalize_hist(image)
# Load an image
img = io.imread("input_image.jpg", as_gray=True)
# Apply histogram equalization
eq_img = histogram_equalization(img)
# Plot the original and equalized images
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(10, 5))
ax1.imshow(img, cmap='gray')
ax1.set_title('Original Image')
ax2.imshow(eq_img, cmap='gray')
ax2.set_title('Equalized Image')
plt.show()
# Plot the histograms
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(10, 5))
ax1.hist(img.ravel(), bins=256)
ax1.set_title('Original Histogram')
ax2.hist(eq_img.ravel(), bins=256)
ax2.set_title('Equalized Histogram')
plt.show()
3. Image Filtering Techniques
Image filtering is a crucial step in many image processing and computer vision applications. Filters can be used to remove noise, enhance edges, or extract specific features from an image. There are two main types of filters: spatial domain filters and frequency domain filters.
3.1 Spatial Domain Filters
Spatial domain filters operate directly on the pixel values of an image. Some common spatial domain filters include:
- Mean filter (Box filter)
- Gaussian filter
- Median filter
- Bilateral filter
Here’s an example of applying a Gaussian filter using OpenCV:
import cv2
import numpy as np
def apply_gaussian_filter(image, kernel_size, sigma):
return cv2.GaussianBlur(image, (kernel_size, kernel_size), sigma)
# Load an image
img = cv2.imread("input_image.jpg")
# Apply Gaussian filter
filtered_img = apply_gaussian_filter(img, 5, 1.0)
# Save the result
cv2.imwrite("filtered_image.jpg", filtered_img)
3.2 Frequency Domain Filters
Frequency domain filters operate on the Fourier transform of an image. These filters are particularly useful for removing periodic noise or separating different frequency components. Common frequency domain filters include:
- Low-pass filter
- High-pass filter
- Band-pass filter
- Notch filter
Here’s an example of applying a low-pass filter in the frequency domain using NumPy and SciPy:
import numpy as np
from scipy import fftpack
import matplotlib.pyplot as plt
def low_pass_filter(image, cutoff_frequency):
# Compute the 2D FFT of the image
fft = fftpack.fft2(image)
fft_shift = fftpack.fftshift(fft)
# Create a mask for the low-pass filter
rows, cols = image.shape
crow, ccol = rows // 2, cols // 2
mask = np.zeros((rows, cols), np.uint8)
mask[crow-cutoff_frequency:crow+cutoff_frequency, ccol-cutoff_frequency:ccol+cutoff_frequency] = 1
# Apply the mask and compute the inverse FFT
fft_shift_filtered = fft_shift * mask
fft_filtered = fftpack.ifftshift(fft_shift_filtered)
filtered_image = np.real(fftpack.ifft2(fft_filtered))
return filtered_image
# Load an image
img = plt.imread("input_image.jpg", as_gray=True)
# Apply low-pass filter
filtered_img = low_pass_filter(img, 30)
# Display the results
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(10, 5))
ax1.imshow(img, cmap='gray')
ax1.set_title('Original Image')
ax2.imshow(filtered_img, cmap='gray')
ax2.set_title('Low-pass Filtered Image')
plt.show()
4. Edge Detection Algorithms
Edge detection is a fundamental operation in image processing and computer vision, used to identify boundaries between different regions in an image. Edge detection algorithms can be broadly categorized into two types: gradient-based methods and Laplacian-based methods.
4.1 Gradient-based Edge Detection
Gradient-based methods detect edges by computing the first-order derivatives of the image intensity. Some popular gradient-based edge detection algorithms include:
- Sobel operator
- Prewitt operator
- Roberts cross operator
- Scharr operator
Here’s an example of Sobel edge detection using OpenCV:
import cv2
import numpy as np
def sobel_edge_detection(image):
# Convert the image to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Apply Gaussian blur to reduce noise
blurred = cv2.GaussianBlur(gray, (3, 3), 0)
# Compute Sobel gradients
sobel_x = cv2.Sobel(blurred, cv2.CV_64F, 1, 0, ksize=3)
sobel_y = cv2.Sobel(blurred, cv2.CV_64F, 0, 1, ksize=3)
# Compute the magnitude of gradients
magnitude = np.sqrt(sobel_x**2 + sobel_y**2)
# Normalize the magnitude to 0-255 range
magnitude = np.uint8(255 * magnitude / np.max(magnitude))
return magnitude
# Load an image
img = cv2.imread("input_image.jpg")
# Apply Sobel edge detection
edges = sobel_edge_detection(img)
# Save the result
cv2.imwrite("sobel_edges.jpg", edges)
4.2 Laplacian-based Edge Detection
Laplacian-based methods detect edges by computing the second-order derivatives of the image intensity. The most common Laplacian-based edge detection algorithm is the Laplacian of Gaussian (LoG) operator, also known as the Marr-Hildreth edge detector.
Here’s an example of Laplacian of Gaussian edge detection using OpenCV:
import cv2
import numpy as np
def laplacian_of_gaussian(image, sigma):
# Convert the image to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Apply Gaussian blur
blurred = cv2.GaussianBlur(gray, (0, 0), sigma)
# Compute Laplacian
laplacian = cv2.Laplacian(blurred, cv2.CV_64F)
# Normalize the Laplacian to 0-255 range
laplacian = np.uint8(255 * laplacian / np.max(np.abs(laplacian)))
return laplacian
# Load an image
img = cv2.imread("input_image.jpg")
# Apply Laplacian of Gaussian edge detection
edges = laplacian_of_gaussian(img, sigma=1.5)
# Save the result
cv2.imwrite("log_edges.jpg", edges)
4.3 Canny Edge Detection
The Canny edge detector is a multi-stage algorithm that combines Gaussian smoothing, gradient computation, non-maximum suppression, and hysteresis thresholding to produce high-quality edge maps. It is widely regarded as one of the best edge detection algorithms.
Here’s an example of Canny edge detection using OpenCV:
import cv2
import numpy as np
def canny_edge_detection(image, low_threshold, high_threshold):
# Convert the image to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Apply Gaussian blur
blurred = cv2.GaussianBlur(gray, (5, 5), 0)
# Apply Canny edge detection
edges = cv2.Canny(blurred, low_threshold, high_threshold)
return edges
# Load an image
img = cv2.imread("input_image.jpg")
# Apply Canny edge detection
edges = canny_edge_detection(img, low_threshold=50, high_threshold=150)
# Save the result
cv2.imwrite("canny_edges.jpg", edges)
5. Feature Extraction and Description
Feature extraction and description are crucial steps in many computer vision tasks, such as object recognition, image matching, and tracking. These techniques aim to identify and describe distinctive characteristics of an image that can be used for further analysis or comparison.
5.1 Corner Detection
Corner detection algorithms identify points in an image where there are significant changes in intensity in multiple directions. Some popular corner detection methods include:
- Harris corner detector
- Shi-Tomasi corner detector
- FAST (Features from Accelerated Segment Test)
Here’s an example of Harris corner detection using OpenCV:
import cv2
import numpy as np
def harris_corner_detection(image, block_size, ksize, k):
# Convert the image to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Compute Harris corner response
dst = cv2.cornerHarris(gray, block_size, ksize, k)
# Dilate the result to mark the corners
dst_dilated = cv2.dilate(dst, None)
# Threshold for an optimal value, mark corners in red
image[dst_dilated > 0.01 * dst_dilated.max()] = [0, 0, 255]
return image
# Load an image
img = cv2.imread("input_image.jpg")
# Apply Harris corner detection
corners = harris_corner_detection(img.copy(), block_size=2, ksize=3, k=0.04)
# Save the result
cv2.imwrite("harris_corners.jpg", corners)
5.2 Blob Detection
Blob detection algorithms identify regions in an image that differ in properties, such as brightness or color, compared to surrounding areas. Common blob detection methods include:
- Laplacian of Gaussian (LoG)
- Difference of Gaussians (DoG)
- Determinant of Hessian (DoH)
Here’s an example of blob detection using OpenCV’s SimpleBlobDetector:
import cv2
import numpy as np
def blob_detection(image):
# Set up the detector with default parameters
params = cv2.SimpleBlobDetector_Params()
# Filter by area
params.filterByArea = True
params.minArea = 100
params.maxArea = 5000
# Filter by circularity
params.filterByCircularity = True
params.minCircularity = 0.1
# Filter by convexity
params.filterByConvexity = True
params.minConvexity = 0.87
# Filter by inertia
params.filterByInertia = True
params.minInertiaRatio = 0.01
# Create a detector with the parameters
detector = cv2.SimpleBlobDetector_create(params)
# Detect blobs
keypoints = detector.detect(image)
# Draw detected blobs as red circles
im_with_keypoints = cv2.drawKeypoints(image, keypoints, np.array([]), (0, 0, 255), cv2.DRAW_MATCHES_FLAGS_DRAW_RICH_KEYPOINTS)
return im_with_keypoints
# Load an image
img = cv2.imread("input_image.jpg")
# Apply blob detection
blobs = blob_detection(img)
# Save the result
cv2.imwrite("blob_detection.jpg", blobs)
5.3 Feature Descriptors
Feature descriptors are used to represent the characteristics of detected features in a compact and robust manner. Some popular feature descriptors include:
- SIFT (Scale-Invariant Feature Transform)
- SURF (Speeded Up Robust Features)
- ORB (Oriented FAST and Rotated BRIEF)
- BRIEF (Binary Robust Independent Elementary Features)
Here’s an example of ORB feature detection and description using OpenCV:
import cv2
import numpy as np
def orb_features(image, num_features=500):
# Initialize ORB detector
orb = cv2.ORB_create(nfeatures=num_features)
# Detect keypoints and compute descriptors
keypoints, descriptors = orb.detectAndCompute(image, None)
# Draw keypoints on the image
im_with_keypoints = cv2.drawKeypoints(image, keypoints, None, color=(0, 255, 0), flags=0)
return im_with_keypoints, keypoints, descriptors
# Load an image
img = cv2.imread("input_image.jpg")
# Apply ORB feature detection and description
img_with_features, keypoints, descriptors = orb_features(img)
# Save the result
cv2.imwrite("orb_features.jpg", img_with_features)
print(f"Number of detected features: {len(keypoints)}")
print(f"Descriptor shape: {descriptors.shape}")
6. Image Segmentation Algorithms
Image segmentation is the process of partitioning an image into multiple segments or regions, each corresponding to a different object or part of the image. Segmentation is a crucial step in many computer vision applications, as it helps to simplify the representation of an image and make it easier to analyze.
6.1 Thresholding
Thresholding is one of the simplest segmentation techniques, which separates an image into foreground and background based on pixel intensity values. Common thresholding methods include:
- Global thresholding
- Otsu’s method
- Adaptive thresholding
Here’s an example of Otsu’s thresholding using OpenCV:
import cv2
import numpy as np
def otsu_thresholding(image):
# Convert the image to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Apply Otsu's thresholding
_, threshold = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY + cv2.THRESH_OTSU)
return threshold
# Load an image
img = cv2.imread("input_image.jpg")
# Apply Otsu's thresholding
segmented = otsu_thresholding(img)
# Save the result
cv2.imwrite("otsu_thresholding.jpg", segmented)
6.2 Region-based Segmentation
Region-based segmentation techniques group pixels into regions based on similarity criteria. Some popular region-based segmentation methods include:
- Region growing
- Split and merge
- Watershed algorithm
Here’s an example of the watershed algorithm using OpenCV:
import cv2
import numpy as np
def watershed_segmentation(image):
# Convert the image to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Apply Otsu's thresholding
_, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)
# Noise removal
kernel = np.ones((3,3), np.uint8)
opening = cv2.morphologyEx(thresh, cv2.MORPH_OPEN, kernel, iterations=2)
# Sure background area
sure_bg = cv2.dilate(opening, kernel, iterations=3)
# Finding sure foreground area
dist_transform = cv2.distanceTransform(opening, cv2.DIST_L2, 5)
_, sure_fg = cv2.threshold(dist_transform, 0.7*dist_transform.max(), 255, 0)
# Finding unknown region
sure_fg = np.uint8(sure_fg)
unknown = cv2.subtract(sure_bg, sure_fg)
# Marker labelling
_, markers = cv2.connectedComponents(sure_fg)
markers = markers + 1
markers[unknown == 255] = 0
# Apply watershed algorithm
markers = cv2.watershed(image, markers)
image[markers == -1] = [255, 0, 0]
return image
# Load an image
img = cv2.imread("input_image.jpg")
# Apply watershed segmentation
segmented = watershed_segmentation(img)
# Save the result
cv2.imwrite("watershed_segmentation.jpg", segmented)
6.3 Edge-based Segmentation
Edge-based segmentation techniques use edge detection algorithms to identify boundaries between different regions in an image. These methods are often combined with other segmentation techniques to improve results.
Here’s an example of edge-based segmentation using the Canny edge detector and contour finding:
import cv2
import numpy as np
def edge_based_segmentation(image):
# Convert the image to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Apply Gaussian blur
blurred = cv2.GaussianBlur(gray, (5, 5), 0)
# Detect edges using Canny edge detector
edges = cv2.Canny(blurred, 50, 150)
# Find contours
contours, _ = cv2.findContours(edges, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# Create a mask for the segmented regions
mask = np.zeros(image.shape[:2], np.uint8)
# Draw contours on the mask
cv2.drawContours(mask, contours, -1, (255), 2)
# Apply the mask to the original image
segmented = cv2.bitwise_and(image, image, mask=mask)
return segmented
# Load an image
img = cv2.imread("input_image.jpg")
# Apply edge-based segmentation
segmented = edge_based_segmentation(img)
# Save the result
cv2.imwrite("edge_based_segmentation.jpg", segmented)
7. Object Detection and Recognition
Object detection and recognition are fundamental tasks in computer vision that involve identifying and localizing specific objects within an image. These techniques have numerous applications, including autonomous vehicles, surveillance systems, and image retrieval.
7.1 Template Matching
Template matching is a simple technique for finding areas of an image that match a template image. It works well for detecting objects with a fixed appearance but is less effective for objects with varying scales or orientations.
Here’s an example of template matching using OpenCV:
import cv2
import numpy as np
def template_matching(image, template):
# Convert images to grayscale
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
gray_template = cv2.cvtColor(template, cv2.COLOR_BGR2GRAY)
# Perform template matching
result = cv2.matchTemplate(gray_image, gray_template, cv2.TM_CCOEFF_NORMED)
# Set a threshold for matching
threshold = 0.8
locations = np.where(result >= threshold)
# Draw rectangles around the matched regions
w, h = gray_template.shape[::-1]
for pt in zip(*locations[::-1]):
cv2.rectangle(image, pt, (pt[0] + w, pt[1] + h), (0, 255, 0), 2)
return image
# Load the main image and the template
img = cv2.imread("main_image.jpg")
template = cv2.imread("template.jpg")
# Perform template matching
result = template_matching(img, template)
# Save the result
cv2.imwrite("template_matching_result.jpg", result)
7.2 Haar Cascade Classifiers
Haar Cascade Classifiers are machine learning-based approaches for object detection. They are particularly effective for detecting faces and other objects with distinct features. OpenCV provides pre-trained Haar Cascade models for various objects.
Here’s an example of face detection using Haar Cascade Classifier:
import cv2
import numpy as np
def haar_cascade_face_detection(image):
# Load the pre-trained face detection model
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + 'haarcascade_frontalface_default.xml')
# Convert the image to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Detect faces
faces = face_cascade.detectMultiScale(gray, scaleFactor=1.1, minNeighbors=5, minSize=(30, 30))
# Draw rectangles around the detected faces
for (x, y, w, h) in faces:
cv2.rectangle(image, (x, y), (x+w, y+h), (0, 255, 0), 2)
return image
# Load an image
img = cv2.imread("input_image.jpg")
# Perform face detection
result = haar_cascade_face_detection(img)
# Save the result
cv2.imwrite("face_detection_result.jpg", result)
7.3 Deep Learning-based Object Detection
Deep learning-based object detection algorithms have achieved state-of-the-art performance in recent years. Some popular deep learning-based object detection models include:
- YOLO (You Only Look Once)
- SSD (Single Shot Detector)
- Faster R-CNN
- RetinaNet
Here’s an example of object detection using the YOLO (You Only Look Once) algorithm with OpenCV’s deep neural network (dnn) module:
import cv2
import numpy as np
def yolo_object_detection(image, confidence_threshold=0.5, nms_threshold=0.3):
# Load YOLO model
net = cv2.dnn.readNetFromDarknet("yolov3.cfg", "yolov3.weights")
# Load class names
with open("coco.names", "r") as f:
classes = [line.strip() for line in f.readlines()]
# Get output layer names
layer_names = net.getLayerNames()
output_layers = [layer_names[i[0] - 1] for i in net.getUnconnectedOutLayers()]
# Prepare the image for input to the neural network
blob = cv2.dnn.blobFromImage(image, 1/255.0, (416, 416), swapRB=True, crop=False)
net.setInput(blob)
# Forward pass through the network
outs = net.forward(output_layers)
# Post-processing
class_ids = []
confidences = []
boxes = []
for out in outs:
for detection in out:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > confidence_threshold:
center_x = int(detection[0] * image.shape[1])
center_y = int(detection[1] * image.shape[0])
w = int(detection[2] * image.shape[1])
h = int(detection[3] * image.shape[0])
x = int(center_x - w / 2)
y = int(center_y - h / 2)
class_ids.append(class_id)
confidences.append(float(confidence))
boxes.append([x, y, w, h])
# Apply non-maximum suppression
indices = cv2.dnn.NMSBoxes(boxes, confidences, confidence_threshold, nms_threshold)
# Draw bounding boxes and labels
for