Algorithms for Fraud Detection Systems: Safeguarding Digital Transactions
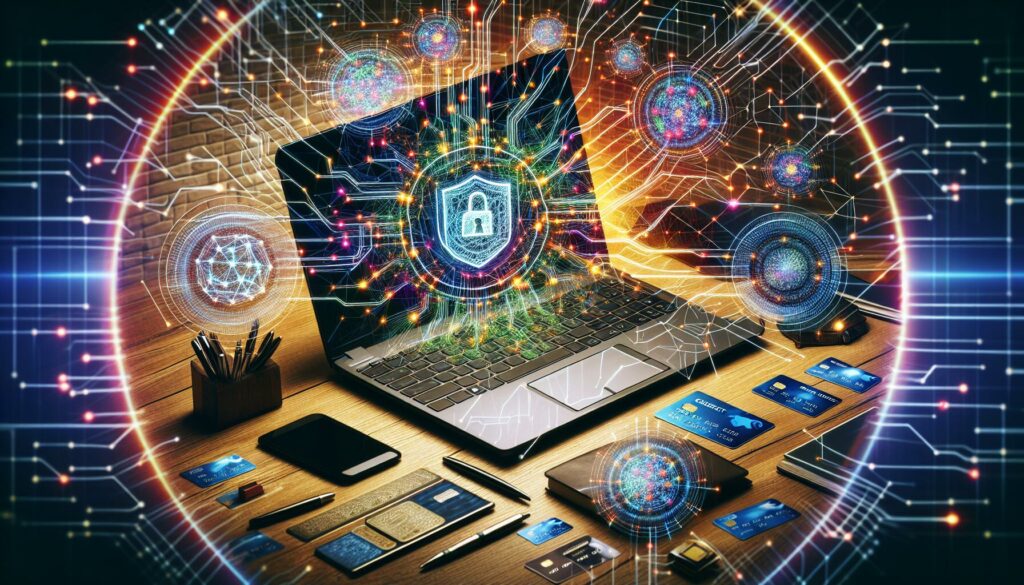
In today’s digital age, where online transactions have become the norm, the importance of robust fraud detection systems cannot be overstated. As cybercriminals become increasingly sophisticated, businesses and financial institutions must stay one step ahead to protect their assets and customers. This is where advanced algorithms for fraud detection come into play. In this comprehensive guide, we’ll explore the world of fraud detection algorithms, their implementation, and how they contribute to creating safer digital ecosystems.
Understanding Fraud Detection Systems
Before diving into specific algorithms, it’s crucial to understand what fraud detection systems are and why they’re essential in modern digital landscapes.
What is a Fraud Detection System?
A fraud detection system is a set of processes and technologies designed to identify and prevent fraudulent activities in various contexts, such as financial transactions, insurance claims, or user authentications. These systems use a combination of rules, statistical analysis, and machine learning algorithms to detect patterns and anomalies that may indicate fraudulent behavior.
The Importance of Fraud Detection
Effective fraud detection is critical for several reasons:
- Financial Protection: It safeguards businesses and individuals from monetary losses.
- Reputation Management: It helps maintain trust and credibility with customers and partners.
- Regulatory Compliance: Many industries require robust fraud prevention measures to comply with legal standards.
- Operational Efficiency: By automating fraud detection, businesses can reduce manual review processes and focus on genuine transactions.
Key Algorithms in Fraud Detection
Now, let’s explore some of the most effective algorithms used in modern fraud detection systems.
1. Logistic Regression
Logistic regression is a statistical method used for predicting binary outcomes. In fraud detection, it can be used to calculate the probability of a transaction being fraudulent based on various input features.
How it works:
- The algorithm is trained on historical data with known fraud outcomes.
- It learns to assign weights to different features (e.g., transaction amount, time, location).
- For new transactions, it calculates a probability score between 0 and 1.
- A threshold is set to classify transactions as fraudulent or legitimate.
Implementation example:
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
# Assume X is your feature matrix and y is your target variable
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
model = LogisticRegression()
model.fit(X_train, y_train)
# Predict probabilities for new transactions
fraud_probabilities = model.predict_proba(X_test)[:, 1]
# Classify based on a threshold (e.g., 0.5)
predictions = (fraud_probabilities > 0.5).astype(int)
2. Decision Trees and Random Forests
Decision trees are simple yet powerful algorithms that make decisions based on a series of questions. Random forests take this concept further by creating an ensemble of decision trees to improve accuracy and reduce overfitting.
How it works:
- Multiple decision trees are created, each trained on a random subset of the data and features.
- Each tree makes a prediction for a given transaction.
- The final prediction is typically the majority vote from all trees.
Implementation example:
from sklearn.ensemble import RandomForestClassifier
# Create and train the model
rf_model = RandomForestClassifier(n_estimators=100, random_state=42)
rf_model.fit(X_train, y_train)
# Make predictions
predictions = rf_model.predict(X_test)
# Get feature importance
feature_importance = rf_model.feature_importances_
3. Neural Networks
Neural networks, particularly deep learning models, have shown remarkable performance in fraud detection due to their ability to learn complex patterns from large datasets.
How it works:
- Input features are fed into a network of interconnected nodes (neurons).
- The network learns to recognize patterns associated with fraudulent activities.
- Multiple hidden layers allow the model to capture intricate relationships in the data.
Implementation example using TensorFlow:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
model = Sequential([
Dense(64, activation='relu', input_shape=(num_features,)),
Dense(32, activation='relu'),
Dense(16, activation='relu'),
Dense(1, activation='sigmoid')
])
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
model.fit(X_train, y_train, epochs=50, batch_size=32, validation_split=0.2)
4. Anomaly Detection Algorithms
Anomaly detection algorithms focus on identifying patterns that deviate significantly from the norm. These are particularly useful for detecting new types of fraud that may not be present in historical data.
Common anomaly detection techniques:
- Isolation Forest
- One-Class SVM
- Local Outlier Factor (LOF)
Implementation example using Isolation Forest:
from sklearn.ensemble import IsolationForest
iso_forest = IsolationForest(contamination=0.1, random_state=42)
predictions = iso_forest.fit_predict(X)
# -1 indicates anomalies, 1 indicates normal instances
anomalies = X[predictions == -1]
5. Time Series Analysis
Time series analysis is crucial for detecting fraud patterns that evolve over time. Techniques like ARIMA (AutoRegressive Integrated Moving Average) and Prophet can be used to forecast expected behavior and flag significant deviations.
Implementation example using Facebook’s Prophet:
from fbprophet import Prophet
# Assume df is your DataFrame with 'ds' (date) and 'y' (metric) columns
model = Prophet()
model.fit(df)
future = model.make_future_dataframe(periods=30) # Forecast 30 periods ahead
forecast = model.predict(future)
# Compare actual values with forecasted values to detect anomalies
Challenges in Implementing Fraud Detection Algorithms
While these algorithms are powerful, implementing them effectively comes with several challenges:
1. Imbalanced Datasets
Fraudulent transactions are typically rare events, leading to highly imbalanced datasets. This can cause models to be biased towards the majority class (legitimate transactions).
Solutions:
- Oversampling techniques like SMOTE (Synthetic Minority Over-sampling Technique)
- Undersampling the majority class
- Using appropriate evaluation metrics (e.g., precision-recall curve, F1 score)
2. Feature Engineering
Creating relevant features that capture fraud patterns is crucial for model performance. This often requires domain expertise and creative thinking.
Effective feature engineering techniques:
- Aggregating transaction history (e.g., average spending in the last 7 days)
- Creating time-based features (e.g., time since last transaction)
- Utilizing external data sources (e.g., IP geolocation)
3. Real-time Processing
Fraud detection often needs to happen in real-time, requiring efficient algorithms and infrastructure.
Strategies for real-time processing:
- Using streaming data processing frameworks like Apache Kafka or Apache Flink
- Implementing lightweight models for quick inference
- Utilizing cloud services for scalable processing
4. Evolving Fraud Patterns
Fraudsters continuously adapt their techniques, making it challenging for static models to remain effective.
Approaches to address evolving patterns:
- Regularly retraining models on recent data
- Implementing online learning algorithms
- Using ensemble methods that combine multiple models
Advanced Techniques in Fraud Detection
As fraud detection systems evolve, more sophisticated techniques are being employed to stay ahead of fraudsters:
1. Graph-based Algorithms
Graph algorithms can uncover complex relationships and networks of fraudulent activities that may not be apparent in traditional tabular data.
Key concepts:
- Node representation: Entities like users, transactions, or devices
- Edge representation: Relationships or interactions between entities
- Community detection: Identifying clusters of potentially fraudulent activities
Implementation example using NetworkX:
import networkx as nx
# Create a graph
G = nx.Graph()
# Add nodes and edges based on your data
# G.add_node(...)
# G.add_edge(...)
# Perform community detection
communities = nx.community.greedy_modularity_communities(G)
# Analyze communities for potential fraud rings
2. Unsupervised Learning for Anomaly Detection
Unsupervised learning techniques can be particularly useful for detecting novel fraud patterns without relying on labeled data.
Popular unsupervised techniques:
- Autoencoders for dimensionality reduction and anomaly detection
- Clustering algorithms like K-means or DBSCAN
- Self-Organizing Maps (SOMs)
Implementation example of an autoencoder:
from tensorflow.keras.models import Model
from tensorflow.keras.layers import Input, Dense
input_dim = X.shape[1]
input_layer = Input(shape=(input_dim,))
encoded = Dense(64, activation='relu')(input_layer)
encoded = Dense(32, activation='relu')(encoded)
decoded = Dense(64, activation='relu')(encoded)
decoded = Dense(input_dim, activation='linear')(decoded)
autoencoder = Model(input_layer, decoded)
autoencoder.compile(optimizer='adam', loss='mse')
autoencoder.fit(X, X, epochs=50, batch_size=32, validation_split=0.2)
# Use the trained model to reconstruct data
reconstructed = autoencoder.predict(X)
# Calculate reconstruction error
mse = np.mean(np.power(X - reconstructed, 2), axis=1)
# Transactions with high reconstruction error are potential anomalies
3. Ensemble Methods
Combining multiple models can often lead to better performance and robustness in fraud detection.
Common ensemble techniques:
- Bagging (e.g., Random Forests)
- Boosting (e.g., XGBoost, LightGBM)
- Stacking multiple diverse models
Implementation example using XGBoost:
import xgboost as xgb
dtrain = xgb.DMatrix(X_train, label=y_train)
dtest = xgb.DMatrix(X_test, label=y_test)
params = {
'max_depth': 6,
'eta': 0.3,
'objective': 'binary:logistic',
'eval_metric': 'auc'
}
model = xgb.train(params, dtrain, num_boost_round=100, evals=[(dtest, 'test')])
# Make predictions
predictions = model.predict(dtest)
Evaluating Fraud Detection Systems
Properly evaluating the performance of fraud detection algorithms is crucial for ensuring their effectiveness and continuous improvement.
Key Evaluation Metrics
- Precision: The proportion of true positive predictions among all positive predictions.
- Recall: The proportion of true positive predictions among all actual positive instances.
- F1 Score: The harmonic mean of precision and recall.
- Area Under the ROC Curve (AUC-ROC): Measures the model’s ability to distinguish between classes.
- Precision-Recall Curve: Particularly useful for imbalanced datasets.
Cross-Validation Techniques
To ensure robust evaluation, consider using:
- K-fold cross-validation
- Stratified K-fold for imbalanced datasets
- Time-based cross-validation for time series data
Example of Model Evaluation
from sklearn.metrics import precision_recall_curve, average_precision_score
from sklearn.model_selection import cross_val_score
# Assuming 'model' is your trained classifier and X, y are your data
# Perform cross-validation
cv_scores = cross_val_score(model, X, y, cv=5, scoring='f1')
print(f"Cross-validation F1 scores: {cv_scores}")
print(f"Mean F1 score: {cv_scores.mean()}")
# Calculate precision-recall curve
y_scores = model.predict_proba(X)[:, 1]
precision, recall, _ = precision_recall_curve(y, y_scores)
average_precision = average_precision_score(y, y_scores)
# Plot precision-recall curve
plt.figure()
plt.step(recall, precision, where='post')
plt.xlabel('Recall')
plt.ylabel('Precision')
plt.title(f'Precision-Recall Curve: AP={average_precision:0.2f}')
Ethical Considerations in Fraud Detection
As we implement increasingly sophisticated fraud detection systems, it’s crucial to consider the ethical implications:
1. Fairness and Bias
Ensure that your algorithms do not discriminate against certain groups based on protected characteristics like race, gender, or age.
Strategies for promoting fairness:
- Regularly audit your models for bias
- Use techniques like adversarial debiasing
- Ensure diverse representation in your training data
2. Transparency and Explainability
In many jurisdictions, there are legal requirements for explaining automated decisions, especially those that significantly impact individuals.
Approaches to improve explainability:
- Use interpretable models where possible (e.g., decision trees)
- Implement techniques like SHAP (SHapley Additive exPlanations) values for black-box models
- Provide clear explanations to users when their transactions are flagged
3. Privacy Concerns
Fraud detection often involves handling sensitive personal and financial data.
Best practices for data privacy:
- Implement strong data encryption and access controls
- Anonymize data where possible
- Comply with relevant data protection regulations (e.g., GDPR, CCPA)
Future Trends in Fraud Detection Algorithms
As technology evolves, so do the methods for detecting fraud. Here are some emerging trends to watch:
1. Federated Learning
This approach allows multiple parties to train models collaboratively without sharing raw data, addressing privacy concerns while leveraging diverse datasets.
2. Quantum Computing
As quantum computers become more accessible, they could revolutionize cryptography and enable more complex fraud detection algorithms.
3. Continuous Learning Systems
Models that can adapt in real-time to new fraud patterns without full retraining will become increasingly important.
4. Integration of Behavioral Biometrics
Incorporating user behavior patterns (e.g., typing rhythm, mouse movements) into fraud detection systems can provide an additional layer of security.
Conclusion
Fraud detection is a critical component of modern digital systems, requiring a sophisticated blend of statistical techniques, machine learning algorithms, and domain expertise. As we’ve explored in this comprehensive guide, there are numerous approaches to implementing effective fraud detection systems, each with its strengths and challenges.
Key takeaways include:
- The importance of choosing the right algorithm(s) for your specific use case
- The need for continuous adaptation to evolving fraud patterns
- The critical role of feature engineering and data preprocessing
- The value of ensemble methods and advanced techniques like graph-based algorithms
- The necessity of robust evaluation metrics and cross-validation techniques
- The ethical considerations that must be addressed in fraud detection systems
As fraud detection technologies continue to advance, staying informed about the latest algorithms and best practices is crucial for developers, data scientists, and business leaders alike. By leveraging these powerful tools responsibly and effectively, we can create safer digital environments and protect individuals and organizations from the ever-present threat of fraud.
Remember, the field of fraud detection is dynamic and ever-evolving. Continuous learning, experimentation, and adaptation are key to staying ahead in this critical area of technology and security.