Algorithms for Financial Modeling and Analysis: Empowering Data-Driven Decision Making
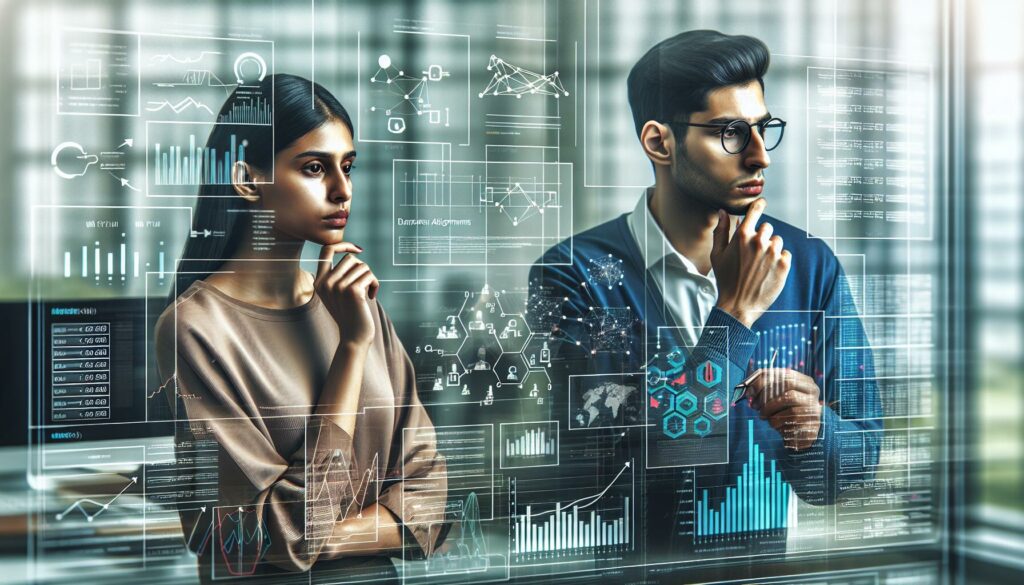
In today’s fast-paced financial world, the ability to make informed decisions based on data-driven insights is crucial. Financial modeling and analysis play a pivotal role in this process, and algorithms have become indispensable tools for professionals in the field. This comprehensive guide will explore the world of algorithms for financial modeling and analysis, providing you with the knowledge and skills to leverage these powerful techniques in your financial decision-making processes.
Understanding Financial Modeling and Analysis
Before diving into the algorithms, it’s essential to have a clear understanding of financial modeling and analysis:
- Financial Modeling: The process of creating a mathematical representation of a financial situation or scenario to predict future outcomes and evaluate potential risks and opportunities.
- Financial Analysis: The examination and interpretation of financial data to assess the performance, stability, and potential of a company, investment, or financial instrument.
These two disciplines work hand in hand to provide valuable insights for decision-makers in finance, investment, and business strategy.
The Role of Algorithms in Financial Modeling and Analysis
Algorithms play a crucial role in financial modeling and analysis by:
- Processing large volumes of financial data quickly and accurately
- Identifying patterns and trends that may not be apparent to human analysts
- Automating complex calculations and simulations
- Enabling real-time analysis and decision-making
- Improving the accuracy and reliability of financial forecasts and predictions
Now, let’s explore some of the key algorithms used in financial modeling and analysis:
1. Time Series Analysis Algorithms
Time series analysis is fundamental in finance for analyzing historical data and making predictions. Some popular algorithms include:
a) Autoregressive Integrated Moving Average (ARIMA)
ARIMA is a widely used algorithm for time series forecasting. It combines autoregression (AR), differencing (I), and moving average (MA) components to model and predict future values based on past observations.
import pandas as pd
import numpy as np
from statsmodels.tsa.arima.model import ARIMA
# Load time series data
data = pd.read_csv('stock_prices.csv', index_col='date', parse_dates=True)
# Fit ARIMA model
model = ARIMA(data['price'], order=(1, 1, 1))
results = model.fit()
# Make predictions
forecast = results.forecast(steps=30)
b) Exponential Smoothing
Exponential smoothing is a method for smoothing time series data and making short-term forecasts. It assigns exponentially decreasing weights to older observations.
from statsmodels.tsa.holtwinters import ExponentialSmoothing
# Fit exponential smoothing model
model = ExponentialSmoothing(data['price'], trend='add', seasonal='add', seasonal_periods=12)
results = model.fit()
# Make predictions
forecast = results.forecast(steps=30)
2. Regression Algorithms
Regression analysis is used to model the relationship between variables and make predictions. Common regression algorithms in finance include:
a) Linear Regression
Linear regression is used to model the linear relationship between a dependent variable and one or more independent variables.
from sklearn.linear_model import LinearRegression
import numpy as np
# Prepare data
X = np.array(data['feature']).reshape(-1, 1)
y = np.array(data['target'])
# Fit linear regression model
model = LinearRegression()
model.fit(X, y)
# Make predictions
predictions = model.predict(X_new)
b) Logistic Regression
Logistic regression is used for binary classification problems, such as predicting the probability of default or bankruptcy.
from sklearn.linear_model import LogisticRegression
# Prepare data
X = data[['feature1', 'feature2', 'feature3']]
y = data['target']
# Fit logistic regression model
model = LogisticRegression()
model.fit(X, y)
# Make predictions
probabilities = model.predict_proba(X_new)
3. Machine Learning Algorithms
Machine learning algorithms have gained popularity in financial modeling and analysis due to their ability to handle complex, non-linear relationships and large datasets.
a) Random Forest
Random Forest is an ensemble learning method that combines multiple decision trees to make predictions. It’s particularly useful for feature selection and handling non-linear relationships.
from sklearn.ensemble import RandomForestRegressor
# Prepare data
X = data[['feature1', 'feature2', 'feature3']]
y = data['target']
# Fit Random Forest model
model = RandomForestRegressor(n_estimators=100, random_state=42)
model.fit(X, y)
# Make predictions
predictions = model.predict(X_new)
b) Support Vector Machines (SVM)
SVM is a powerful algorithm for both classification and regression tasks. It’s particularly useful for handling high-dimensional data and non-linear relationships.
from sklearn.svm import SVR
# Prepare data
X = data[['feature1', 'feature2', 'feature3']]
y = data['target']
# Fit SVM model
model = SVR(kernel='rbf')
model.fit(X, y)
# Make predictions
predictions = model.predict(X_new)
4. Optimization Algorithms
Optimization algorithms are crucial for portfolio management and risk analysis. They help find the best solution among a set of possibilities.
a) Mean-Variance Optimization
Mean-Variance Optimization is used to construct portfolios that maximize expected return for a given level of risk or minimize risk for a given level of expected return.
import numpy as np
from scipy.optimize import minimize
def portfolio_variance(weights, cov_matrix):
return np.dot(weights.T, np.dot(cov_matrix, weights))
def portfolio_return(weights, expected_returns):
return np.sum(weights * expected_returns)
def optimize_portfolio(expected_returns, cov_matrix, target_return):
num_assets = len(expected_returns)
constraints = ({'type': 'eq', 'fun': lambda x: np.sum(x) - 1},
{'type': 'eq', 'fun': lambda x: portfolio_return(x, expected_returns) - target_return})
bounds = tuple((0, 1) for _ in range(num_assets))
result = minimize(portfolio_variance, num_assets*[1./num_assets], args=(cov_matrix,),
method='SLSQP', bounds=bounds, constraints=constraints)
return result.x
b) Monte Carlo Simulation
Monte Carlo simulation is used to model the probability of different outcomes in a process that cannot easily be predicted due to the intervention of random variables.
import numpy as np
import matplotlib.pyplot as plt
def monte_carlo_simulation(initial_price, mu, sigma, dt, T, num_simulations):
num_steps = int(T / dt)
prices = np.zeros((num_simulations, num_steps + 1))
prices[:, 0] = initial_price
for t in range(1, num_steps + 1):
z = np.random.standard_normal(num_simulations)
prices[:, t] = prices[:, t-1] * np.exp((mu - 0.5 * sigma**2) * dt + sigma * np.sqrt(dt) * z)
return prices
# Run simulation
initial_price = 100
mu = 0.1 # Expected return
sigma = 0.2 # Volatility
dt = 1/252 # Daily time step
T = 1 # Time horizon (1 year)
num_simulations = 1000
simulated_prices = monte_carlo_simulation(initial_price, mu, sigma, dt, T, num_simulations)
# Plot results
plt.figure(figsize=(10, 6))
plt.plot(simulated_prices.T, alpha=0.1)
plt.title('Monte Carlo Simulation of Stock Price')
plt.xlabel('Time Steps')
plt.ylabel('Stock Price')
plt.show()
5. Natural Language Processing (NLP) Algorithms
NLP algorithms are increasingly used in financial analysis to extract insights from unstructured text data, such as news articles, social media posts, and company reports.
a) Sentiment Analysis
Sentiment analysis is used to determine the sentiment (positive, negative, or neutral) of text data, which can be valuable for predicting market trends or assessing public opinion about a company or financial instrument.
from textblob import TextBlob
def analyze_sentiment(text):
blob = TextBlob(text)
sentiment = blob.sentiment.polarity
if sentiment > 0:
return 'Positive'
elif sentiment < 0:
return 'Negative'
else:
return 'Neutral'
# Example usage
news_article = "The company reported strong earnings, beating analyst expectations."
sentiment = analyze_sentiment(news_article)
print(f"Sentiment: {sentiment}")
b) Named Entity Recognition (NER)
NER is used to identify and classify named entities (e.g., companies, people, locations) in text, which can be useful for extracting relevant information from financial documents.
import spacy
nlp = spacy.load("en_core_web_sm")
def extract_entities(text):
doc = nlp(text)
entities = [(ent.text, ent.label_) for ent in doc.ents]
return entities
# Example usage
text = "Apple Inc. reported record profits last quarter, according to CEO Tim Cook."
entities = extract_entities(text)
print("Extracted entities:")
for entity, label in entities:
print(f"{entity} - {label}")
6. Deep Learning Algorithms
Deep learning algorithms, particularly neural networks, have gained traction in financial modeling and analysis due to their ability to handle complex, non-linear relationships and large datasets.
a) Long Short-Term Memory (LSTM) Networks
LSTM networks are a type of recurrent neural network (RNN) that are particularly well-suited for sequence prediction problems, such as time series forecasting in finance.
from keras.models import Sequential
from keras.layers import LSTM, Dense
import numpy as np
def create_lstm_model(input_shape):
model = Sequential()
model.add(LSTM(50, activation='relu', input_shape=input_shape))
model.add(Dense(1))
model.compile(optimizer='adam', loss='mse')
return model
# Prepare data (assume X_train and y_train are prepared)
X_train = np.reshape(X_train, (X_train.shape[0], X_train.shape[1], 1))
# Create and train the model
model = create_lstm_model((X_train.shape[1], 1))
model.fit(X_train, y_train, epochs=100, batch_size=32)
# Make predictions
predictions = model.predict(X_test)
b) Convolutional Neural Networks (CNN)
CNNs are typically associated with image processing, but they can also be applied to financial time series data for pattern recognition and feature extraction.
from keras.models import Sequential
from keras.layers import Conv1D, MaxPooling1D, Flatten, Dense
def create_cnn_model(input_shape):
model = Sequential()
model.add(Conv1D(filters=64, kernel_size=3, activation='relu', input_shape=input_shape))
model.add(MaxPooling1D(pool_size=2))
model.add(Flatten())
model.add(Dense(50, activation='relu'))
model.add(Dense(1))
model.compile(optimizer='adam', loss='mse')
return model
# Prepare data (assume X_train and y_train are prepared)
X_train = np.reshape(X_train, (X_train.shape[0], X_train.shape[1], 1))
# Create and train the model
model = create_cnn_model((X_train.shape[1], 1))
model.fit(X_train, y_train, epochs=100, batch_size=32)
# Make predictions
predictions = model.predict(X_test)
Implementing Algorithms in Financial Modeling and Analysis
When implementing algorithms for financial modeling and analysis, consider the following best practices:
- Data Preprocessing: Ensure your data is clean, normalized, and properly formatted before applying algorithms.
- Feature Selection: Choose relevant features that have a significant impact on your target variable.
- Model Validation: Use techniques like cross-validation to assess the performance and generalizability of your models.
- Hyperparameter Tuning: Optimize the hyperparameters of your algorithms to improve their performance.
- Ensemble Methods: Combine multiple algorithms to create more robust and accurate models.
- Interpretability: Use techniques like SHAP (SHapley Additive exPlanations) values to interpret the output of complex models.
- Continuous Monitoring: Regularly monitor and update your models to ensure they remain accurate as market conditions change.
Challenges and Considerations
While algorithms offer powerful tools for financial modeling and analysis, it’s important to be aware of potential challenges:
- Overfitting: Be cautious of models that perform well on training data but fail to generalize to new, unseen data.
- Data Quality: The accuracy of your models depends heavily on the quality and reliability of your input data.
- Market Dynamics: Financial markets are complex and dynamic, and past performance doesn’t guarantee future results.
- Regulatory Compliance: Ensure your models and algorithms comply with relevant financial regulations and guidelines.
- Ethical Considerations: Be mindful of potential biases in your data and models, and consider the ethical implications of your financial decisions.
Conclusion
Algorithms have revolutionized financial modeling and analysis, enabling professionals to process vast amounts of data, uncover hidden patterns, and make more informed decisions. From time series analysis and regression to machine learning and deep learning techniques, the range of algorithms available provides powerful tools for tackling complex financial problems.
As you continue to explore and implement these algorithms in your financial modeling and analysis work, remember that they are tools to augment human expertise, not replace it. Combining algorithmic insights with domain knowledge, critical thinking, and ethical considerations will lead to the most effective and responsible financial decision-making.
By mastering these algorithms and understanding their applications in finance, you’ll be well-equipped to navigate the complexities of modern financial markets and drive data-driven success in your organization.