Algorithms for Environmental Modeling and Simulation: Empowering Sustainable Solutions
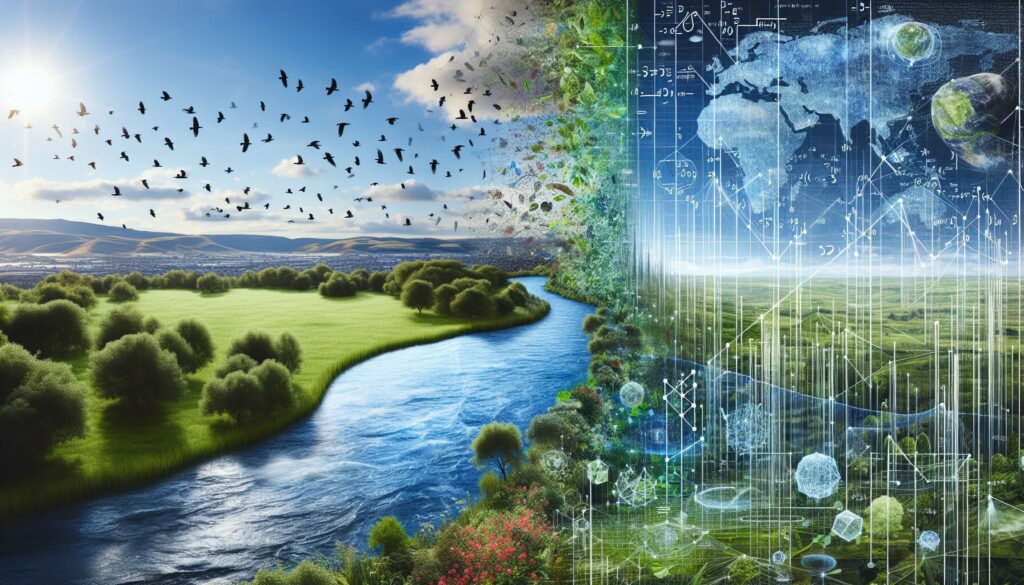
In the age of increasing environmental concerns and the urgent need for sustainable solutions, the role of algorithms in environmental modeling and simulation has become more critical than ever. These powerful computational tools allow scientists, researchers, and policymakers to better understand complex environmental systems, predict future scenarios, and develop effective strategies for conservation and mitigation. In this comprehensive guide, we’ll explore the fascinating world of algorithms for environmental modeling and simulation, their applications, and their impact on our planet’s future.
Understanding Environmental Modeling and Simulation
Before diving into the algorithms, it’s essential to grasp the concepts of environmental modeling and simulation:
- Environmental Modeling: The process of creating mathematical representations of environmental systems to study their behavior, interactions, and responses to various factors.
- Environmental Simulation: The use of computer programs to run these models, allowing for the exploration of different scenarios and the prediction of future outcomes.
These techniques are crucial for addressing a wide range of environmental challenges, from climate change and air pollution to ecosystem management and resource conservation.
Key Algorithms for Environmental Modeling and Simulation
Let’s explore some of the most important algorithms used in environmental modeling and simulation:
1. Cellular Automata
Cellular Automata (CA) are discrete models used to simulate complex systems based on simple rules. In environmental modeling, CA are particularly useful for simulating spatial processes such as forest fires, urban growth, and land-use changes.
A basic implementation of a 1D cellular automaton in Python might look like this:
import numpy as np
def cellular_automaton(rule, size, steps):
# Initialize the cellular automaton
ca = np.zeros(size, dtype=int)
ca[size // 2] = 1 # Set the middle cell to 1
# Run the simulation
for _ in range(steps):
new_ca = np.zeros(size, dtype=int)
for i in range(size):
# Get the state of the cell and its neighbors
left = ca[(i - 1) % size]
center = ca[i]
right = ca[(i + 1) % size]
# Apply the rule
state = 4 * left + 2 * center + right
new_ca[i] = (rule >> state) & 1
ca = new_ca
print(''.join(['#' if cell else ' ' for cell in ca]))
# Example usage
cellular_automaton(rule=90, size=50, steps=25)
2. Agent-Based Models
Agent-Based Models (ABMs) simulate the actions and interactions of autonomous agents within a system. These models are particularly useful for studying complex adaptive systems in ecology, such as predator-prey relationships or the spread of invasive species.
Here’s a simple example of an agent-based model simulating the movement of particles in a 2D space:
import numpy as np
import matplotlib.pyplot as plt
class Particle:
def __init__(self, x, y):
self.x = x
self.y = y
def move(self):
self.x += np.random.randn()
self.y += np.random.randn()
def simulate_particles(num_particles, steps):
particles = [Particle(np.random.rand(), np.random.rand()) for _ in range(num_particles)]
for _ in range(steps):
for particle in particles:
particle.move()
x = [p.x for p in particles]
y = [p.y for p in particles]
plt.clf()
plt.scatter(x, y)
plt.xlim(0, 1)
plt.ylim(0, 1)
plt.pause(0.1)
# Example usage
simulate_particles(num_particles=50, steps=100)
3. Differential Equations Solvers
Many environmental processes can be described using differential equations. Numerical methods for solving these equations are crucial for simulating complex systems such as climate models, fluid dynamics, and population dynamics.
Here’s an example of solving a simple differential equation (exponential growth) using the Euler method:
import numpy as np
import matplotlib.pyplot as plt
def euler_method(f, y0, t):
y = np.zeros(len(t))
y[0] = y0
for i in range(1, len(t)):
h = t[i] - t[i-1]
y[i] = y[i-1] + h * f(y[i-1], t[i-1])
return y
# Define the differential equation: dy/dt = ky
def exponential_growth(y, t, k=0.1):
return k * y
# Set up the time array
t = np.linspace(0, 50, 1000)
# Solve the equation
y = euler_method(lambda y, t: exponential_growth(y, t), y0=1, t=t)
# Plot the results
plt.plot(t, y)
plt.xlabel('Time')
plt.ylabel('Population')
plt.title('Exponential Growth Model')
plt.show()
4. Machine Learning Algorithms
Machine learning algorithms, including neural networks and decision trees, are increasingly used in environmental modeling for tasks such as species distribution modeling, remote sensing image analysis, and climate prediction.
Here’s a simple example of using a decision tree for environmental classification:
from sklearn.tree import DecisionTreeClassifier
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Generate a synthetic dataset
X, y = make_classification(n_samples=1000, n_features=5, n_classes=3, n_informative=5, random_state=42)
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create and train the decision tree classifier
clf = DecisionTreeClassifier(random_state=42)
clf.fit(X_train, y_train)
# Make predictions on the test set
y_pred = clf.predict(X_test)
# Calculate the accuracy
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy:.2f}")
Applications of Environmental Modeling and Simulation Algorithms
The algorithms discussed above find applications in various environmental domains:
1. Climate Change Modeling
Climate models use a combination of differential equations, cellular automata, and machine learning algorithms to simulate the Earth’s climate system. These models help scientists predict future climate scenarios, assess the impact of human activities, and develop mitigation strategies.
2. Ecosystem Dynamics
Agent-based models and differential equations are often used to simulate ecosystem dynamics, including predator-prey relationships, species interactions, and biodiversity patterns. These simulations help ecologists understand complex ecological processes and inform conservation efforts.
3. Air and Water Quality Management
Algorithms for fluid dynamics and pollutant dispersion are crucial for modeling air and water quality. These models help environmental agencies predict pollution levels, assess the impact of emissions, and develop effective pollution control strategies.
4. Natural Disaster Prediction and Management
Environmental modeling algorithms play a vital role in predicting and managing natural disasters such as hurricanes, floods, and wildfires. These models combine weather data, topographical information, and historical patterns to forecast potential hazards and guide emergency response efforts.
5. Renewable Energy Planning
Algorithms for environmental modeling are essential in planning and optimizing renewable energy systems. They help in assessing wind patterns for wind farms, solar radiation for photovoltaic installations, and hydrological conditions for hydroelectric power plants.
Challenges and Future Directions
While algorithms for environmental modeling and simulation have made significant strides, several challenges and opportunities for future development remain:
1. Data Quality and Availability
Environmental models often require large amounts of high-quality data. Improving data collection techniques, sensor networks, and remote sensing technologies is crucial for enhancing the accuracy and reliability of environmental simulations.
2. Computational Complexity
Many environmental models, especially those dealing with climate systems or large-scale ecosystems, are computationally intensive. Developing more efficient algorithms and leveraging high-performance computing resources are ongoing challenges in the field.
3. Model Uncertainty
Environmental systems are inherently complex and subject to various uncertainties. Improving methods for quantifying and communicating model uncertainty is essential for informed decision-making based on simulation results.
4. Integration of Multiple Scales
Many environmental processes operate across multiple spatial and temporal scales. Developing algorithms that can effectively integrate these different scales remains a significant challenge in environmental modeling.
5. Artificial Intelligence and Machine Learning
The integration of AI and machine learning techniques with traditional environmental modeling approaches offers exciting possibilities for improving model accuracy, handling big data, and discovering new patterns in environmental systems.
Conclusion
Algorithms for environmental modeling and simulation are powerful tools in our quest to understand and protect our planet. From predicting climate change to managing ecosystems and natural resources, these computational techniques play a crucial role in addressing some of the most pressing environmental challenges of our time.
As we continue to refine these algorithms and develop new approaches, the field of environmental modeling and simulation will undoubtedly contribute even more to our ability to create sustainable solutions and safeguard the health of our planet for future generations.
By mastering these algorithms and understanding their applications, aspiring environmental scientists, data analysts, and software developers can make significant contributions to this vital field. Whether you’re interested in climate science, ecology, or sustainable resource management, the world of environmental modeling and simulation offers exciting opportunities to apply your coding skills to real-world problems and make a positive impact on the environment.