Algorithms for Detecting Cycles in Graphs: A Comprehensive Guide
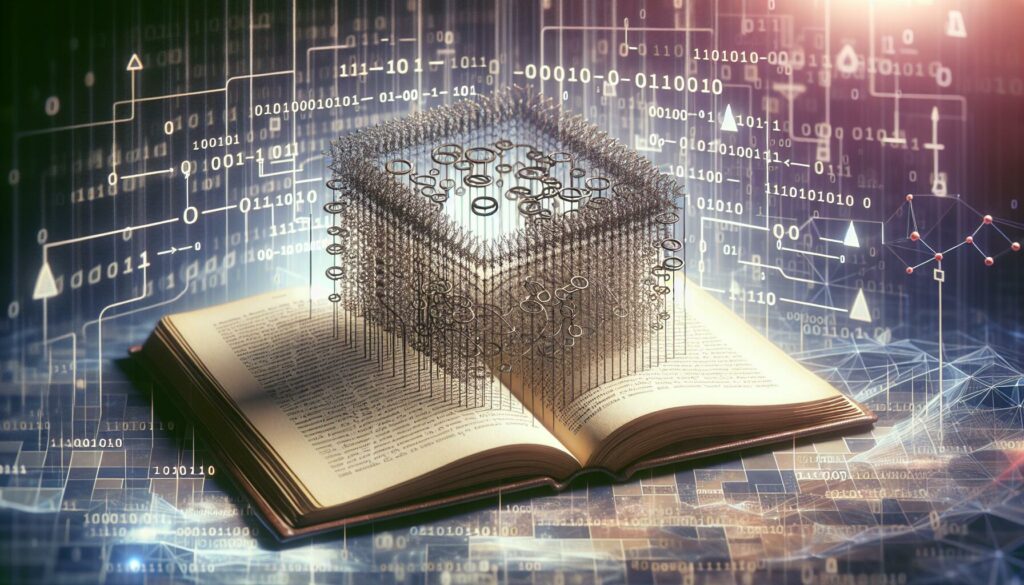
In the world of computer science and graph theory, detecting cycles in graphs is a fundamental problem with wide-ranging applications. Whether you’re preparing for technical interviews at top tech companies or simply honing your algorithmic skills, understanding cycle detection algorithms is crucial. This comprehensive guide will walk you through various algorithms for detecting cycles in graphs, their implementations, and real-world applications.
Table of Contents
- Introduction to Graph Cycles
- Depth-First Search (DFS) for Cycle Detection
- Union-Find Algorithm for Cycle Detection
- Floyd’s Cycle-Finding Algorithm
- Topological Sort for Cycle Detection in Directed Graphs
- Real-world Applications of Cycle Detection
- Optimization Techniques and Time Complexity Analysis
- Practice Problems and Interview Preparation
- Conclusion
1. Introduction to Graph Cycles
Before diving into the algorithms, let’s establish a clear understanding of what a cycle in a graph is. A cycle in a graph is a path of edges and vertices wherein a vertex is reachable from itself. In other words, it’s a closed loop in the graph structure.
Cycles can occur in both directed and undirected graphs:
- Undirected Graph Cycle: A path that starts and ends at the same vertex, with at least one edge.
- Directed Graph Cycle: A path that starts and ends at the same vertex, following the direction of the edges, with at least one edge.
Detecting cycles in graphs is important for various reasons:
- Identifying circular dependencies in systems
- Detecting deadlocks in operating systems
- Finding loops in linked data structures
- Analyzing social networks and relationships
- Optimizing transportation and logistics routes
Now that we have a foundation, let’s explore the algorithms used to detect cycles in graphs.
2. Depth-First Search (DFS) for Cycle Detection
Depth-First Search (DFS) is a versatile graph traversal algorithm that can be adapted for cycle detection. The basic idea is to keep track of vertices in the current path and check if the current vertex is already in the path.
Algorithm Steps:
- Start DFS from any arbitrary vertex.
- For each visited vertex, keep track of it in the recursion stack.
- For every visited vertex v, if there is an adjacent vertex u which is already in the recursion stack, then there is a cycle in the graph.
- If all adjacent vertices are processed and no cycle is found, remove the vertex from the recursion stack.
Implementation in Python:
class Graph:
def __init__(self, vertices):
self.V = vertices
self.graph = [[] for _ in range(vertices)]
def add_edge(self, u, v):
self.graph[u].append(v)
def is_cyclic_util(self, v, visited, rec_stack):
visited[v] = True
rec_stack[v] = True
for neighbor in self.graph[v]:
if not visited[neighbor]:
if self.is_cyclic_util(neighbor, visited, rec_stack):
return True
elif rec_stack[neighbor]:
return True
rec_stack[v] = False
return False
def is_cyclic(self):
visited = [False] * self.V
rec_stack = [False] * self.V
for node in range(self.V):
if not visited[node]:
if self.is_cyclic_util(node, visited, rec_stack):
return True
return False
# Example usage
g = Graph(4)
g.add_edge(0, 1)
g.add_edge(1, 2)
g.add_edge(2, 3)
g.add_edge(3, 1) # This edge creates a cycle
print("Graph contains cycle: " + str(g.is_cyclic()))
This implementation works for directed graphs. For undirected graphs, you’d need to modify the add_edge
method to add edges in both directions and adjust the cycle detection logic slightly.
Time and Space Complexity:
- Time Complexity: O(V + E), where V is the number of vertices and E is the number of edges in the graph.
- Space Complexity: O(V) for the recursion stack and visited array.
3. Union-Find Algorithm for Cycle Detection
The Union-Find algorithm, also known as the Disjoint Set Union algorithm, is particularly useful for detecting cycles in undirected graphs. It works by maintaining disjoint sets of vertices and merging these sets as edges are processed.
Algorithm Steps:
- Create disjoint sets for each vertex.
- For each edge (u, v) in the graph:
- Find the set that u belongs to (let’s call it set1)
- Find the set that v belongs to (let’s call it set2)
- If set1 is the same as set2, a cycle is detected
- Otherwise, union set1 and set2
Implementation in Python:
class DisjointSet:
def __init__(self, vertices):
self.parent = list(range(vertices))
self.rank = [0] * vertices
def find(self, item):
if self.parent[item] != item:
self.parent[item] = self.find(self.parent[item])
return self.parent[item]
def union(self, x, y):
xroot = self.find(x)
yroot = self.find(y)
if self.rank[xroot] < self.rank[yroot]:
self.parent[xroot] = yroot
elif self.rank[xroot] > self.rank[yroot]:
self.parent[yroot] = xroot
else:
self.parent[yroot] = xroot
self.rank[xroot] += 1
class Graph:
def __init__(self, vertices):
self.V = vertices
self.graph = []
def add_edge(self, u, v):
self.graph.append([u, v])
def is_cyclic(self):
ds = DisjointSet(self.V)
for u, v in self.graph:
x = ds.find(u)
y = ds.find(v)
if x == y:
return True
ds.union(x, y)
return False
# Example usage
g = Graph(3)
g.add_edge(0, 1)
g.add_edge(1, 2)
g.add_edge(2, 0) # This edge creates a cycle
print("Graph contains cycle: " + str(g.is_cyclic()))
Time and Space Complexity:
- Time Complexity: O(E * α(V)), where E is the number of edges, V is the number of vertices, and α is the inverse Ackermann function, which grows very slowly and is effectively constant for all practical values of V.
- Space Complexity: O(V) for storing the disjoint sets.
4. Floyd’s Cycle-Finding Algorithm
Floyd’s Cycle-Finding Algorithm, also known as the “tortoise and hare” algorithm, is primarily used for detecting cycles in linked lists but can be adapted for certain graph structures, particularly those that can be represented as a sequence of nodes.
Algorithm Steps:
- Initialize two pointers, slow and fast, to the head of the linked list or starting node of the graph.
- Move the slow pointer one step and the fast pointer two steps at a time.
- If there’s a cycle, the fast pointer will eventually meet the slow pointer.
- If the fast pointer reaches the end (null), there’s no cycle.
Implementation in Python:
class Node:
def __init__(self, value):
self.value = value
self.next = None
def detect_cycle(head):
if not head or not head.next:
return False
slow = head
fast = head.next
while slow != fast:
if not fast or not fast.next:
return False
slow = slow.next
fast = fast.next.next
return True
# Example usage
# Create a linked list with a cycle
head = Node(1)
head.next = Node(2)
head.next.next = Node(3)
head.next.next.next = Node(4)
head.next.next.next.next = head.next # Create cycle
print("Linked list contains cycle: " + str(detect_cycle(head)))
While this example uses a linked list, the concept can be applied to graphs where each node has a single outgoing edge, effectively forming a sequence of nodes.
Time and Space Complexity:
- Time Complexity: O(n), where n is the number of nodes.
- Space Complexity: O(1), as it uses only two pointers regardless of the input size.
5. Topological Sort for Cycle Detection in Directed Graphs
Topological sorting is an algorithm used to linearly order the vertices of a Directed Acyclic Graph (DAG). If a topological sort is not possible, it indicates the presence of a cycle. This method is particularly useful for detecting cycles in directed graphs.
Algorithm Steps:
- Compute in-degree (number of incoming edges) for each vertex.
- Enqueue all vertices with in-degree 0 to a queue.
- While the queue is not empty:
- Dequeue a vertex
- For each adjacent vertex, reduce its in-degree by 1
- If in-degree becomes 0, enqueue the vertex
- If all vertices are processed, no cycle exists. Otherwise, there’s a cycle.
Implementation in Python:
from collections import defaultdict, deque
class Graph:
def __init__(self, vertices):
self.graph = defaultdict(list)
self.V = vertices
def add_edge(self, u, v):
self.graph[u].append(v)
def is_cyclic(self):
in_degree = [0] * self.V
for i in self.graph:
for j in self.graph[i]:
in_degree[j] += 1
queue = deque()
for i in range(self.V):
if in_degree[i] == 0:
queue.append(i)
cnt = 0
while queue:
u = queue.popleft()
cnt += 1
for i in self.graph[u]:
in_degree[i] -= 1
if in_degree[i] == 0:
queue.append(i)
return cnt != self.V
# Example usage
g = Graph(4)
g.add_edge(0, 1)
g.add_edge(1, 2)
g.add_edge(2, 3)
g.add_edge(3, 1) # This edge creates a cycle
print("Graph contains cycle: " + str(g.is_cyclic()))
Time and Space Complexity:
- Time Complexity: O(V + E), where V is the number of vertices and E is the number of edges.
- Space Complexity: O(V) for the queue and in-degree array.
6. Real-world Applications of Cycle Detection
Cycle detection algorithms have numerous practical applications across various domains:
- Deadlock Detection in Operating Systems: Cycles in resource allocation graphs can indicate potential deadlocks.
- Detecting Circular Dependencies: In software engineering, cycle detection helps identify circular dependencies in module imports or class hierarchies.
- Financial Fraud Detection: Cycles in transaction graphs can reveal potential money laundering or fraudulent activities.
- Network Topology Analysis: Identifying loops in network topologies is crucial for routing protocols and network optimization.
- Garbage Collection in Programming Languages: Cycle detection is used in garbage collection algorithms to identify and clean up circular references.
- Ecosystem Analysis: In biology, cycles in food webs or ecological relationships can be studied using these algorithms.
- Compiler Optimization: Detecting cycles in control flow graphs helps in code optimization and analysis.
- Social Network Analysis: Identifying cycles in social graphs can reveal interesting group dynamics or circular chains of influence.
7. Optimization Techniques and Time Complexity Analysis
When working with large graphs or in performance-critical applications, it’s important to optimize cycle detection algorithms. Here are some techniques and considerations:
Optimization Techniques:
- Graph Representation: Choose between adjacency list and adjacency matrix based on graph density. Adjacency lists are generally more efficient for sparse graphs.
- Iterative vs. Recursive: In some languages, an iterative implementation of DFS might be more efficient than a recursive one, especially for deep graphs.
- Early Termination: Stop the algorithm as soon as a cycle is detected, rather than processing the entire graph.
- Parallelization: For very large graphs, consider parallel processing techniques to distribute the workload across multiple cores or machines.
- Bit Manipulation: Use bit vectors instead of boolean arrays for marking visited nodes to save space and potentially improve cache performance.
- Path Compression: In the Union-Find algorithm, implement path compression to optimize the find operation.
Time Complexity Analysis:
Let’s compare the time complexities of the algorithms we’ve discussed:
- DFS: O(V + E)
- Union-Find: O(E * α(V)), where α is the inverse Ackermann function
- Floyd’s Cycle-Finding: O(n) for n nodes
- Topological Sort: O(V + E)
The choice of algorithm depends on the specific problem constraints:
- For general graphs, DFS or Topological Sort are good choices.
- For undirected graphs, Union-Find can be very efficient, especially with path compression and union by rank optimizations.
- For linked structures or graphs with single successors, Floyd’s algorithm is simple and memory-efficient.
8. Practice Problems and Interview Preparation
To solidify your understanding and prepare for technical interviews, consider practicing with these problems:
- LeetCode 207: Course Schedule – Detect if it’s possible to finish all courses given prerequisites (cycle detection in a directed graph).
- LeetCode 261: Graph Valid Tree – Determine if an undirected graph is a valid tree (no cycles and all connected).
- LeetCode 802: Find Eventual Safe States – Find all safe nodes in a directed graph (nodes not part of or leading to a cycle).
- LeetCode 684: Redundant Connection – Find an edge that can be removed to make the graph acyclic.
- LeetCode 1192: Critical Connections in a Network – Find all critical connections in a network (edges whose removal would disconnect the graph).
When preparing for interviews, focus on:
- Understanding the underlying principles of each algorithm
- Identifying which algorithm is best suited for different graph types and problem constraints
- Practicing implementation from scratch
- Analyzing time and space complexity
- Discussing potential optimizations and trade-offs
9. Conclusion
Detecting cycles in graphs is a fundamental problem in computer science with wide-ranging applications. We’ve explored several algorithms, each with its strengths and ideal use cases:
- Depth-First Search (DFS) offers a versatile approach for both directed and undirected graphs.
- The Union-Find algorithm excels in undirected graphs and has near-constant time complexity for practical inputs.
- Floyd’s Cycle-Finding Algorithm is memory-efficient and works well for linked structures.
- Topological Sort provides an elegant solution for directed graphs while offering additional insights into the graph structure.
As you continue to develop your skills in graph algorithms, remember that understanding these cycle detection methods is crucial not just for solving specific problems, but for developing a deeper intuition about graph structures and their properties. This knowledge will serve you well in technical interviews and real-world software development scenarios.
Keep practicing, exploring different graph problems, and don’t hesitate to dive deeper into advanced topics like strongly connected components, minimum spanning trees, and graph coloring algorithms. The world of graph theory is rich and full of fascinating challenges waiting to be solved!