Algorithms for 3D Rendering and Graphics: A Comprehensive Guide
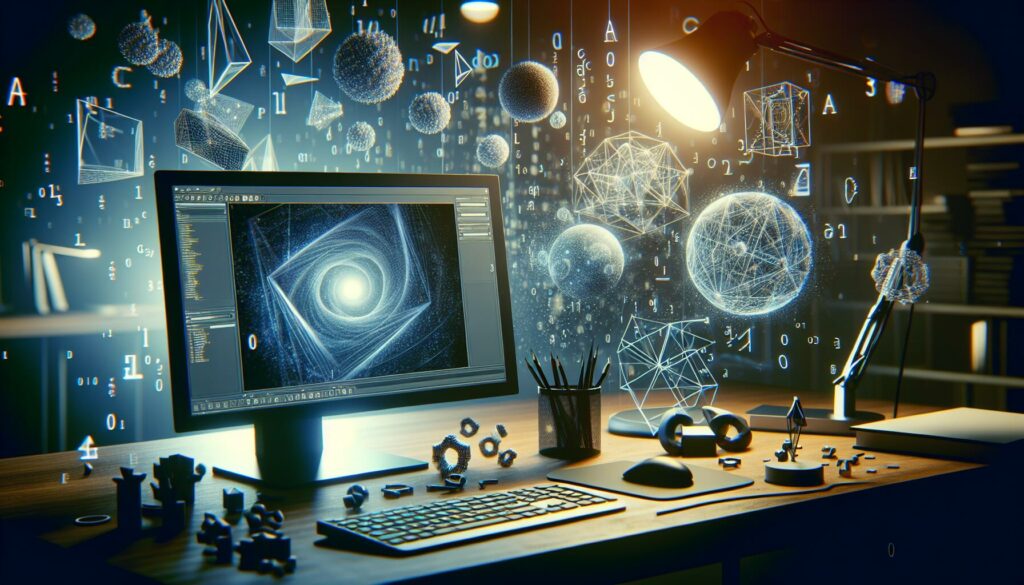
In the world of computer graphics and game development, 3D rendering and graphics algorithms play a crucial role in creating immersive and visually stunning experiences. These algorithms form the backbone of modern video games, animated movies, virtual reality applications, and computer-aided design software. In this comprehensive guide, we’ll explore the fundamental concepts and key algorithms used in 3D rendering and graphics, providing you with a solid foundation to understand and implement these techniques in your own projects.
1. Introduction to 3D Rendering
3D rendering is the process of generating a 2D image from a 3D scene. This involves several steps, including modeling, texturing, lighting, and rendering. The goal is to create a realistic or stylized representation of a three-dimensional environment on a two-dimensional screen.
1.1 The Rendering Pipeline
The rendering pipeline is a series of steps that transform 3D data into a 2D image. The main stages of the pipeline include:
- Vertex Processing
- Primitive Assembly
- Rasterization
- Fragment Processing
- Output Merging
Each of these stages involves specific algorithms and techniques that we’ll explore in more detail throughout this article.
2. Fundamental Concepts in 3D Graphics
Before diving into specific algorithms, it’s essential to understand some fundamental concepts in 3D graphics:
2.1 Coordinate Systems
3D graphics rely on various coordinate systems to represent objects and their positions in space:
- World Space: The global coordinate system of the entire 3D scene.
- Object Space: The local coordinate system of individual 3D objects.
- Camera Space: The coordinate system relative to the viewer’s perspective.
- Screen Space: The 2D coordinate system of the final rendered image.
2.2 Vertices, Edges, and Faces
3D objects are typically represented as a collection of vertices (points), edges (lines connecting vertices), and faces (polygons formed by edges). The most common primitive used in 3D graphics is the triangle, as it’s the simplest polygon that can represent a plane in 3D space.
2.3 Transformations
Transformations are used to modify the position, orientation, and scale of 3D objects. The three primary types of transformations are:
- Translation: Moving an object in 3D space.
- Rotation: Changing the orientation of an object around an axis.
- Scaling: Changing the size of an object.
These transformations are typically represented using matrices, which allow for efficient computation and composition of multiple transformations.
3. Vertex Processing Algorithms
Vertex processing is the first stage of the rendering pipeline, where individual vertices of 3D objects are transformed and prepared for rendering.
3.1 Model-View-Projection (MVP) Transformation
The MVP transformation is a crucial step in vertex processing that converts vertices from object space to clip space. It involves three main transformations:
- Model Transform: Converts vertices from object space to world space.
- View Transform: Converts vertices from world space to camera space.
- Projection Transform: Projects vertices from camera space to clip space.
Here’s a simplified example of how to implement the MVP transformation in C++:
#include <glm/glm.hpp>
#include <glm/gtc/matrix_transform.hpp>
glm::mat4 calculateMVP(const glm::mat4& model, const glm::mat4& view, const glm::mat4& projection) {
return projection * view * model;
}
// Usage
glm::mat4 model = glm::translate(glm::mat4(1.0f), glm::vec3(0.0f, 0.0f, -5.0f));
glm::mat4 view = glm::lookAt(glm::vec3(0.0f, 0.0f, 3.0f), glm::vec3(0.0f, 0.0f, 0.0f), glm::vec3(0.0f, 1.0f, 0.0f));
glm::mat4 projection = glm::perspective(glm::radians(45.0f), 4.0f / 3.0f, 0.1f, 100.0f);
glm::mat4 mvp = calculateMVP(model, view, projection);
3.2 Vertex Skinning
Vertex skinning is an algorithm used for animating 3D characters. It involves associating vertices with one or more bones in a skeleton and calculating their positions based on the bones’ transformations. The most common approach is Linear Blend Skinning (LBS).
Here’s a simplified implementation of LBS in C++:
struct Vertex {
glm::vec3 position;
std::vector<int> boneIndices;
std::vector<float> boneWeights;
};
glm::vec3 calculateSkinnedPosition(const Vertex& vertex, const std::vector<glm::mat4>& boneTransforms) {
glm::vec3 skinnedPosition(0.0f);
for (size_t i = 0; i < vertex.boneIndices.size(); ++i) {
int boneIndex = vertex.boneIndices[i];
float boneWeight = vertex.boneWeights[i];
skinnedPosition += boneWeight * (boneTransforms[boneIndex] * glm::vec4(vertex.position, 1.0f));
}
return skinnedPosition;
}
4. Rasterization Algorithms
Rasterization is the process of converting vector graphics (e.g., triangles) into a raster image (pixels on the screen). This stage is crucial for determining which pixels are covered by each primitive.
4.1 Scanline Algorithm
The scanline algorithm is a classic approach to rasterization. It works by scanning the image horizontally, line by line, and determining which pixels are inside each triangle.
Here’s a simplified implementation of the scanline algorithm in C++:
struct Edge {
float x, yMax, slope;
};
void scanlineRasterization(const std::vector<glm::vec2>& vertices, int screenWidth, int screenHeight) {
std::vector<std::vector<Edge>> edgeTable(screenHeight);
// Build edge table
for (size_t i = 0; i < vertices.size(); ++i) {
const glm::vec2& v1 = vertices[i];
const glm::vec2& v2 = vertices[(i + 1) % vertices.size()];
if (v1.y != v2.y) {
Edge edge;
edge.yMax = std::max(v1.y, v2.y);
edge.x = (v1.y < v2.y) ? v1.x : v2.x;
edge.slope = (v2.x - v1.x) / (v2.y - v1.y);
int yMin = static_cast<int>(std::min(v1.y, v2.y));
edgeTable[yMin].push_back(edge);
}
}
// Process scanlines
std::vector<Edge> activeEdgeList;
for (int y = 0; y < screenHeight; ++y) {
// Add new edges to the active edge list
activeEdgeList.insert(activeEdgeList.end(), edgeTable[y].begin(), edgeTable[y].end());
// Remove inactive edges
activeEdgeList.erase(std::remove_if(activeEdgeList.begin(), activeEdgeList.end(),
[y](const Edge& edge) { return edge.yMax <= y; }), activeEdgeList.end());
// Sort active edges by x-coordinate
std::sort(activeEdgeList.begin(), activeEdgeList.end(),
[](const Edge& a, const Edge& b) { return a.x < b.x; });
// Fill pixels between edge pairs
for (size_t i = 0; i < activeEdgeList.size(); i += 2) {
int xStart = static_cast<int>(std::ceil(activeEdgeList[i].x));
int xEnd = static_cast<int>(std::ceil(activeEdgeList[i + 1].x));
for (int x = xStart; x < xEnd; ++x) {
// Set pixel color here
}
}
// Update x-coordinates for the next scanline
for (Edge& edge : activeEdgeList) {
edge.x += edge.slope;
}
}
}
4.2 Edge Function Algorithm
The edge function algorithm is a more modern approach to rasterization that allows for efficient parallel processing on GPUs. It uses barycentric coordinates to determine if a pixel is inside a triangle.
Here’s a simplified implementation of the edge function algorithm in C++:
struct Triangle {
glm::vec2 v0, v1, v2;
};
float edgeFunction(const glm::vec2& a, const glm::vec2& b, const glm::vec2& c) {
return (c.x - a.x) * (b.y - a.y) - (c.y - a.y) * (b.x - a.x);
}
void edgeFunctionRasterization(const Triangle& triangle, int screenWidth, int screenHeight) {
glm::vec2 bboxMin(std::numeric_limits<float>::max());
glm::vec2 bboxMax(std::numeric_limits<float>::lowest());
// Compute bounding box
bboxMin = glm::min(bboxMin, triangle.v0);
bboxMin = glm::min(bboxMin, triangle.v1);
bboxMin = glm::min(bboxMin, triangle.v2);
bboxMax = glm::max(bboxMax, triangle.v0);
bboxMax = glm::max(bboxMax, triangle.v1);
bboxMax = glm::max(bboxMax, triangle.v2);
// Clip bounding box to screen dimensions
bboxMin = glm::max(bboxMin, glm::vec2(0.0f));
bboxMax = glm::min(bboxMax, glm::vec2(screenWidth - 1, screenHeight - 1));
// Rasterize
for (int y = static_cast<int>(bboxMin.y); y <= static_cast<int>(bboxMax.y); ++y) {
for (int x = static_cast<int>(bboxMin.x); x <= static_cast<int>(bboxMax.x); ++x) {
glm::vec2 pixelCenter(x + 0.5f, y + 0.5f);
float w0 = edgeFunction(triangle.v1, triangle.v2, pixelCenter);
float w1 = edgeFunction(triangle.v2, triangle.v0, pixelCenter);
float w2 = edgeFunction(triangle.v0, triangle.v1, pixelCenter);
if (w0 >= 0 && w1 >= 0 && w2 >= 0) {
// Pixel is inside the triangle
// Set pixel color here
}
}
}
}
5. Texture Mapping Algorithms
Texture mapping is the process of applying 2D images to 3D surfaces to add detail and realism. There are several algorithms used for texture mapping, including:
5.1 Bilinear Interpolation
Bilinear interpolation is used to sample textures when the texture coordinates don’t exactly match texel centers. It interpolates between the four nearest texels to produce a smoother result.
Here’s a simplified implementation of bilinear interpolation in C++:
glm::vec4 bilinearInterpolation(const glm::vec2& uv, const std::vector<glm::vec4>& texture, int textureWidth, int textureHeight) {
float u = uv.x * (textureWidth - 1);
float v = uv.y * (textureHeight - 1);
int x0 = static_cast<int>(u);
int y0 = static_cast<int>(v);
int x1 = std::min(x0 + 1, textureWidth - 1);
int y1 = std::min(y0 + 1, textureHeight - 1);
float s = u - x0;
float t = v - y0;
glm::vec4 c00 = texture[y0 * textureWidth + x0];
glm::vec4 c10 = texture[y0 * textureWidth + x1];
glm::vec4 c01 = texture[y1 * textureWidth + x0];
glm::vec4 c11 = texture[y1 * textureWidth + x1];
return (1 - s) * (1 - t) * c00 +
s * (1 - t) * c10 +
(1 - s) * t * c01 +
s * t * c11;
}
5.2 Mip Mapping
Mip mapping is a technique used to reduce aliasing artifacts when rendering textures at different scales. It involves creating a pyramid of pre-filtered texture images at different resolutions.
Here’s a simplified implementation of mip map generation in C++:
std::vector<std::vector<glm::vec4>> generateMipMaps(const std::vector<glm::vec4>& baseTexture, int baseWidth, int baseHeight) {
std::vector<std::vector<glm::vec4>> mipMaps;
mipMaps.push_back(baseTexture);
int width = baseWidth;
int height = baseHeight;
while (width > 1 || height > 1) {
int newWidth = std::max(1, width / 2);
int newHeight = std::max(1, height / 2);
std::vector<glm::vec4> newMipMap(newWidth * newHeight);
for (int y = 0; y < newHeight; ++y) {
for (int x = 0; x < newWidth; ++x) {
glm::vec4 sum(0.0f);
int count = 0;
for (int dy = 0; dy < 2; ++dy) {
for (int dx = 0; dx < 2; ++dx) {
int sx = x * 2 + dx;
int sy = y * 2 + dy;
if (sx < width && sy < height) {
sum += mipMaps.back()[sy * width + sx];
++count;
}
}
}
newMipMap[y * newWidth + x] = sum / static_cast<float>(count);
}
}
mipMaps.push_back(newMipMap);
width = newWidth;
height = newHeight;
}
return mipMaps;
}
6. Lighting and Shading Algorithms
Lighting and shading algorithms are crucial for creating realistic or stylized 3D graphics. They determine how light interacts with surfaces and how the final color of each pixel is calculated.
6.1 Phong Shading
Phong shading is a popular lighting model that calculates lighting per-pixel, providing smooth results. It consists of three components: ambient, diffuse, and specular lighting.
Here’s a simplified implementation of Phong shading in GLSL:
#version 330 core
in vec3 FragPos;
in vec3 Normal;
uniform vec3 lightPos;
uniform vec3 viewPos;
uniform vec3 lightColor;
uniform vec3 objectColor;
out vec4 FragColor;
void main() {
// Ambient
float ambientStrength = 0.1;
vec3 ambient = ambientStrength * lightColor;
// Diffuse
vec3 norm = normalize(Normal);
vec3 lightDir = normalize(lightPos - FragPos);
float diff = max(dot(norm, lightDir), 0.0);
vec3 diffuse = diff * lightColor;
// Specular
float specularStrength = 0.5;
vec3 viewDir = normalize(viewPos - FragPos);
vec3 reflectDir = reflect(-lightDir, norm);
float spec = pow(max(dot(viewDir, reflectDir), 0.0), 32);
vec3 specular = specularStrength * spec * lightColor;
vec3 result = (ambient + diffuse + specular) * objectColor;
FragColor = vec4(result, 1.0);
}
6.2 Physically Based Rendering (PBR)
Physically Based Rendering (PBR) is a more advanced lighting model that aims to simulate real-world light behavior. It uses principles of physics to create more accurate and realistic lighting results.
Here’s a simplified implementation of a PBR shader in GLSL:
#version 330 core
in vec3 FragPos;
in vec3 Normal;
in vec2 TexCoords;
uniform vec3 lightPos;
uniform vec3 viewPos;
uniform vec3 lightColor;
uniform sampler2D albedoMap;
uniform sampler2D roughnessMap;
uniform sampler2D metallicMap;
out vec4 FragColor;
const float PI = 3.14159265359;
vec3 fresnelSchlick(float cosTheta, vec3 F0) {
return F0 + (1.0 - F0) * pow(1.0 - cosTheta, 5.0);
}
float DistributionGGX(vec3 N, vec3 H, float roughness) {
float a = roughness * roughness;
float a2 = a * a;
float NdotH = max(dot(N, H), 0.0);
float NdotH2 = NdotH * NdotH;
float num = a2;
float denom = (NdotH2 * (a2 - 1.0) + 1.0);
denom = PI * denom * denom;
return num / denom;
}
float GeometrySchlickGGX(float NdotV, float roughness) {
float r = (roughness + 1.0);
float k = (r * r) / 8.0;
float num = NdotV;
float denom = NdotV * (1.0 - k) + k;
return num / denom;
}
float GeometrySmith(vec3 N, vec3 V, vec3 L, float roughness) {
float NdotV = max(dot(N, V), 0.0);
float NdotL = max(dot(N, L), 0.0);
float ggx2 = GeometrySchlickGGX(NdotV, roughness);
float ggx1 = GeometrySchlickGGX(NdotL, roughness);
return ggx1 * ggx2;
}
void main() {
vec3 albedo = texture(albedoMap, TexCoords).rgb;
float roughness = texture(roughnessMap, TexCoords).r;
float metallic = texture(metallicMap, TexCoords).r;
vec3 N = normalize(Normal);
vec3 V = normalize(viewPos - FragPos);
vec3 F0 = vec3(0.04);
F0 = mix(F0, albedo, metallic);
vec3 Lo = vec3(0.0);
// Calculate per-light radiance
vec3 L = normalize(lightPos - FragPos);
vec3 H = normalize(V + L);
float distance = length(lightPos - FragPos);
float attenuation = 1.0 / (distance * distance);
vec3 radiance = lightColor * attenuation;
// Cook-Torrance BRDF
float NDF = DistributionGGX(N, H, roughness);
float G = GeometrySmith(N, V, L, roughness);
vec3 F = fresnelSchlick(max(dot(H, V), 0.0), F0);
vec3 numerator = NDF * G * F;
float denominator = 4.0 * max(dot(N, V), 0.0) * max(dot(N, L), 0.0);
vec3 specular = numerator / max(denominator, 0.001);
vec3 kS = F;
vec3 kD = vec3(1.0) - kS;
kD *= 1.0 - metallic;
float NdotL = max(dot(N, L), 0.0);
Lo += (kD * albedo / PI + specular) * radiance * NdotL;
vec3 ambient = vec3(0.03) * albedo;
vec3 color = ambient + Lo;
// HDR tonemapping
color = color / (color + vec3(1.0));
// Gamma correction
color = pow(color, vec3(1.0/2.2));
FragColor = vec4(color, 1.0);
}
7. Visibility and Occlusion Algorithms
Visibility and occlusion algorithms are essential for determining which parts of a 3D scene are visible from a given viewpoint. These algorithms help improve rendering performance and create realistic shadows.
7.1 Z-Buffer Algorithm
The Z-buffer algorithm is a widely used technique for handling visibility in 3D rendering. It stores the depth (Z-value) of each pixel and only renders fragments that are closer to the camera than the previously stored depth.
Here’s a simplified implementation of the Z-buffer algorithm in C++:
struct Fragment {
glm::vec3 color;
float depth;
};
void zBufferRendering(std::vector<Fragment>& framebuffer, std::vector<float>& depthBuffer, int width, int height) {
// Initialize depth buffer with maximum depth
std::fill(depthBuffer.begin(), depthBuffer.end(), 1.0f);
// For each triangle in the scene
for (const auto& triangle : scene.triangles) {
// Rasterize the triangle (simplified)
for (int y = 0; y < height; ++y) {
for (int x = 0; x < width; ++x) {
Fragment fragment = rasterizeTriangle(triangle, x, y);
if (fragment.depth < depthBuffer[y * width + x]) {
depthBuffer[y * width + x] = fragment.depth;
framebuffer[y * width + x] = fragment;
}
}
}
}
}
7.2 Occlusion Culling
Occlusion culling is a technique used to improve rendering performance by identifying and skipping the rendering of objects that are not visible from the current viewpoint. One popular approach is Hierarchical Z-Buffer Occlusion Culling.
Here’s a simplified implementation of Hierarchical Z-Buffer Occlusion Culling in C++:
struct OctreeNode {
AABB boundingBox;
std::vector<OctreeNode*> children;
std::vector<Object*> objects;
};
bool isVisible(const OctreeNode* node, const Camera& camera, const std::vector<std::vector<float>>& hierarchicalZBuffer) {
// Project bounding box to screen space
AABB screenSpaceBB = projectToScreenSpace(node->boundingBox, camera);
// Check if bounding box is outside the view frustum
if (!isInsideViewFrustum(screenSpaceBB, camera)) {
return false;
}
// Check against hierarchical Z-buffer
int level = 0;
while (level < hierarchicalZBuffer.size()) {
float maxDepth = getMaxDepth(screenSpaceBB, hierarchicalZBuffer[level]);
if (maxDepth < screenSpaceBB.minZ) {
return false; // Occluded
}
if (maxDepth >= screenSpaceBB.maxZ) {
return true; // Visible
}
++level;
}
return true; // Potentially visible
}
void renderScene(OctreeNode* root, const Camera& camera, std::vector<std::vector<float>>& hierarchicalZBuffer) {
if (!isVisible(root, camera, hierarchicalZBuffer)) {
return;
}
if (root->children.empty()) {
for (const auto& object : root->objects) {
renderObject(object, camera);
}
} else {
for (const auto& child : root->children) {
renderScene(child, camera, hierarchicalZBuffer);
}
}
}
8. Anti-Aliasing Algorithms
Anti-aliasing algorithms are used to reduce jagged edges and improve the overall image quality in 3D rendering. There are several techniques available, each with its own trade-offs between quality and performance.
8.1 Multisample Anti-Aliasing (MSAA)
MSAA is a popular anti-aliasing technique that samples multiple points within each pixel and combines the results to produce a smoother image. Here’s a simplified implementation of 4x MSAA in C++:
struct Sample {
glm::vec2 offset;
float weight;
};
const std::array<Sample, 4> msaaSamples = {{
{{0.125f, 0.625f}, 0.25f},
{{0.375f, 0.125f}, 0.25f},
{{0.625f, 0.875f}, 0.25f},
{{0.875f, 0.375f}, 0.25f}
}};
glm::vec4 msaaRendering(const Triangle& triangle, int x, int y) {
glm::vec4 color(0.0f);
float depth = std::numeric_limits<float>::max();
for (const auto& sample : msaaSamples) {
glm::vec2 samplePos(x + sample.offset.x, y + sample.offset.y);
if (isInsideTriangle(triangle, samplePos)) {
float sampleDepth = interpolateDepth(triangle, samplePos);
if (sampleDepth < depth) {
depth = sampleDepth;
color = interpolateColor(triangle, samplePos) * sample.weight;
}
}
}
return color;
}
8.2 Fast Approximate Anti-Aliasing (FXAA)
FXAA is a post-processing anti-aliasing technique that analyzes the rendered image and smooths out edges. It’s computationally efficient and can be applied as a final step in the rendering pipeline.
Here’s a simplified implementation of FXAA in GLSL:
#version 330 core
in vec2 TexCoords;
out vec4 FragColor;
uniform sampler2D screenTexture;
uniform vec2 screenSize;
const float FXAA_SPAN_MAX = 8.0;
const float FXAA_REDUCE_MUL = 1.0 / 8.0;
const float FXAA_REDUCE_MIN = 1.0 / 128.0;
void main() {
vec2 texelSize = 1.0 / screenSize;
vec3 rgbNW = texture(screenTexture, TexCoords + vec2(-1.0, -1.0) * texelSize).rgb;
vec3 rgbNE = texture(screenTexture, TexCoords + vec2(1.0, -1.0) * texelSize).rgb;
vec3 rgbSW = texture(screenTexture, TexCoords + vec2(-1.0, 1.0) * texelSize).rgb;
vec3 rgbSE = texture(screenTexture, TexCoords + vec2(1.0, 1.0) * texelSize).rgb;
vec3 rgbM = texture(screenTexture, TexCoords).rgb;
vec3 luma = vec3(0.299, 0.587, 0.114);
float lumaNW = dot(rgbNW, luma);
float lumaNE = dot(rgbNE, luma);
float lumaSW = dot(rgbSW, luma);
float lumaSE = dot(rgbSE, luma);
float lumaM = dot(rgbM, luma);
float lumaMin = min(lumaM, min(min(lumaNW, lumaNE), min(lumaSW, lumaSE)));
float lumaMax = max(lumaM, max(max(lumaNW, lumaNE), max(lumaSW, lumaSE)));
vec2 dir;
dir.x = -((lumaNW + lumaNE) - (lumaSW + lumaS