Algorithmic Trading: Basics and Strategies
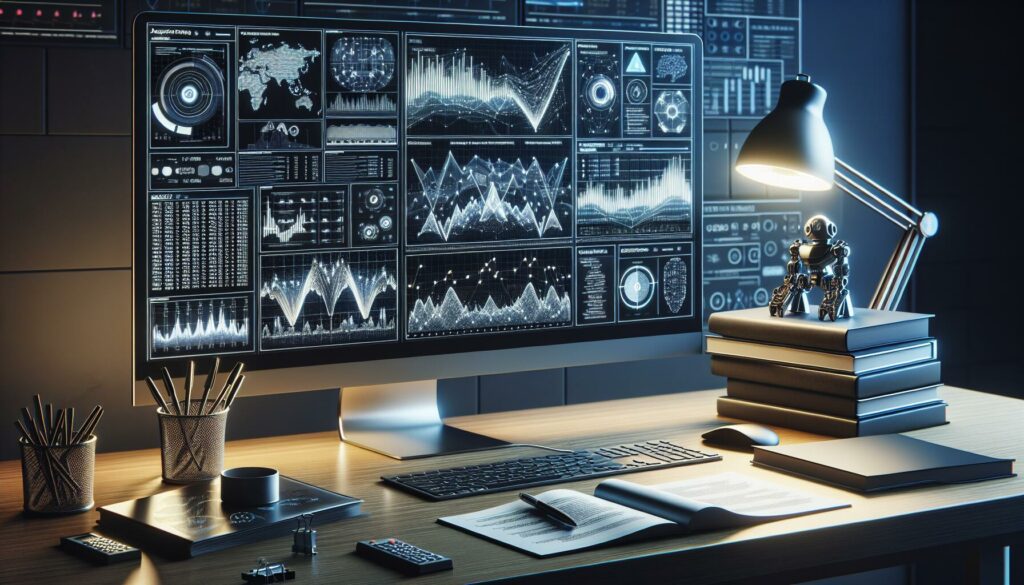
In today’s fast-paced financial markets, algorithmic trading has become an essential tool for investors and traders seeking to gain a competitive edge. This sophisticated approach to trading leverages computer algorithms to execute trades at speeds and frequencies that are impossible for human traders to match. In this comprehensive guide, we’ll explore the fundamentals of algorithmic trading, its benefits, popular strategies, and how you can get started in this exciting field.
What is Algorithmic Trading?
Algorithmic trading, also known as algo trading or automated trading, refers to the use of computer programs and mathematical models to make trading decisions and execute orders in financial markets. These algorithms analyze market data, identify trading opportunities, and place trades automatically based on predefined rules and conditions.
The primary goals of algorithmic trading include:
- Executing trades at the best possible prices
- Minimizing the impact of large orders on market prices
- Reducing transaction costs
- Implementing complex trading strategies consistently
- Eliminating emotional decision-making in trading
Benefits of Algorithmic Trading
Algorithmic trading offers several advantages over traditional manual trading methods:
- Speed and Efficiency: Algorithms can analyze vast amounts of data and execute trades in milliseconds, far surpassing human capabilities.
- Consistency: Automated systems follow predefined rules without emotional bias, ensuring consistent execution of trading strategies.
- Reduced Errors: By eliminating human errors in order placement and execution, algorithmic trading minimizes costly mistakes.
- Backtesting: Traders can test their strategies on historical data to evaluate performance before risking real capital.
- Diversification: Algorithms can monitor and trade multiple markets and instruments simultaneously, enabling better portfolio diversification.
- 24/7 Trading: Automated systems can operate around the clock, taking advantage of opportunities in global markets.
Key Components of Algorithmic Trading Systems
An effective algorithmic trading system typically consists of the following components:
- Data Feed: A reliable source of real-time market data, including price quotes, order book information, and other relevant market indicators.
- Strategy Engine: The core algorithm that analyzes market data, identifies trading opportunities, and generates trading signals based on predefined rules.
- Risk Management Module: A component that monitors and controls the system’s exposure to risk, implementing stop-loss orders and position sizing rules.
- Execution Engine: The module responsible for converting trading signals into actual orders and submitting them to the market.
- Performance Analytics: Tools for monitoring and analyzing the system’s performance, including profit and loss tracking, trade statistics, and risk metrics.
Popular Algorithmic Trading Strategies
Algorithmic trading encompasses a wide range of strategies, from simple rule-based systems to complex machine learning models. Here are some popular algorithmic trading strategies:
1. Trend Following Strategies
Trend following strategies aim to capitalize on sustained price movements in a particular direction. These strategies typically use technical indicators to identify and follow trends. Common indicators include:
- Moving Averages
- MACD (Moving Average Convergence Divergence)
- Relative Strength Index (RSI)
- Bollinger Bands
Here’s a simple example of a trend following strategy using a moving average crossover:
def trend_following_strategy(data):
# Calculate short-term and long-term moving averages
short_ma = data['close'].rolling(window=20).mean()
long_ma = data['close'].rolling(window=50).mean()
# Generate buy/sell signals
signals = pd.Series(index=data.index)
signals[short_ma > long_ma] = 1 # Buy signal
signals[short_ma < long_ma] = -1 # Sell signal
return signals
2. Mean Reversion Strategies
Mean reversion strategies are based on the assumption that asset prices tend to fluctuate around a long-term average or “mean” value. These strategies aim to profit from temporary deviations from this mean by buying when prices are below the average and selling when they are above.
A simple mean reversion strategy might use Bollinger Bands:
def mean_reversion_strategy(data, window=20, num_std=2):
# Calculate Bollinger Bands
rolling_mean = data['close'].rolling(window=window).mean()
rolling_std = data['close'].rolling(window=window).std()
upper_band = rolling_mean + (rolling_std * num_std)
lower_band = rolling_mean - (rolling_std * num_std)
# Generate buy/sell signals
signals = pd.Series(index=data.index)
signals[data['close'] < lower_band] = 1 # Buy signal
signals[data['close'] > upper_band] = -1 # Sell signal
return signals
3. Statistical Arbitrage
Statistical arbitrage strategies seek to profit from price discrepancies between related securities or markets. These strategies often involve pairs trading, where two correlated assets are traded simultaneously to capitalize on temporary divergences in their price relationship.
Here’s a basic pairs trading strategy:
def pairs_trading_strategy(data_a, data_b, window=20, threshold=2):
# Calculate the spread between two assets
spread = data_a['close'] - data_b['close']
# Calculate z-score of the spread
z_score = (spread - spread.rolling(window=window).mean()) / spread.rolling(window=window).std()
# Generate buy/sell signals
signals = pd.Series(index=data_a.index)
signals[z_score > threshold] = -1 # Sell asset A, buy asset B
signals[z_score < -threshold] = 1 # Buy asset A, sell asset B
return signals
4. Market Making Strategies
Market making strategies aim to profit from the bid-ask spread by continuously quoting buy and sell prices for a financial instrument. These strategies are often employed by high-frequency trading firms and require sophisticated infrastructure to execute effectively.
A simplified market making strategy might look like this:
def market_making_strategy(order_book, spread_threshold):
best_bid = order_book['bids'][0][0]
best_ask = order_book['asks'][0][0]
current_spread = best_ask - best_bid
if current_spread > spread_threshold:
# Place buy order slightly above best bid
buy_price = best_bid + 0.01
# Place sell order slightly below best ask
sell_price = best_ask - 0.01
return {'buy': buy_price, 'sell': sell_price}
else:
return None # Do not place orders if spread is too narrow
5. Machine Learning-based Strategies
As artificial intelligence and machine learning technologies advance, more sophisticated algorithmic trading strategies are emerging. These strategies use techniques such as neural networks, support vector machines, and reinforcement learning to identify patterns and make predictions in financial markets.
Here’s a simple example of using a machine learning model for trading:
from sklearn.ensemble import RandomForestClassifier
def ml_trading_strategy(data, features, target, train_size=0.8):
# Prepare features and target
X = data[features]
y = data[target]
# Split data into training and testing sets
split_index = int(len(data) * train_size)
X_train, X_test = X[:split_index], X[split_index:]
y_train, y_test = y[:split_index], y[split_index:]
# Train Random Forest model
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Make predictions
predictions = model.predict(X_test)
return predictions
Getting Started with Algorithmic Trading
If you’re interested in developing your own algorithmic trading systems, here are some steps to get started:
- Learn the Basics: Familiarize yourself with financial markets, trading concepts, and basic programming skills.
- Choose a Programming Language: Popular languages for algo trading include Python, R, and C++. Python is often recommended for beginners due to its simplicity and rich ecosystem of financial libraries.
- Master Data Analysis: Learn to work with financial data, including cleaning, preprocessing, and visualization techniques.
- Study Quantitative Finance: Understand concepts like portfolio theory, risk management, and statistical analysis.
- Explore Trading Platforms: Familiarize yourself with popular trading platforms and APIs, such as Interactive Brokers, Alpaca, or Quantopian.
- Develop and Backtest Strategies: Create simple trading strategies and test them on historical data using backtesting frameworks like Backtrader or Zipline.
- Practice with Paper Trading: Before risking real money, test your strategies in a simulated environment using paper trading accounts.
- Continuously Learn and Improve: Stay updated on new developments in algorithmic trading, machine learning, and financial markets.
Essential Libraries and Tools for Algorithmic Trading
To streamline your algorithmic trading development process, consider using the following Python libraries and tools:
- NumPy and Pandas: For efficient data manipulation and numerical computations
- Matplotlib and Seaborn: For data visualization and charting
- Scikit-learn: For implementing machine learning models
- TA-Lib: For technical analysis and indicator calculations
- Backtrader or Zipline: For backtesting trading strategies
- PyAlgoTrade: An event-driven algorithmic trading library
- Quantopian: A web-based platform for developing and backtesting trading algorithms
Challenges and Considerations in Algorithmic Trading
While algorithmic trading offers numerous benefits, it also comes with its own set of challenges and considerations:
- Market Complexity: Financial markets are complex systems influenced by numerous factors, making it challenging to develop consistently profitable algorithms.
- Overfitting: There’s a risk of creating strategies that perform well on historical data but fail in live trading due to overfitting.
- Technology Infrastructure: Effective algo trading requires robust hardware, low-latency network connections, and reliable data feeds.
- Regulatory Compliance: Algorithmic trading is subject to various regulations, which can vary by jurisdiction and market.
- Market Impact: Large-scale algorithmic trading can potentially impact market dynamics and liquidity.
- Competition: The algo trading space is highly competitive, with large institutions investing heavily in advanced technologies and talent.
- Continuous Adaptation: Successful strategies may become less effective over time as markets evolve, requiring constant refinement and innovation.
The Future of Algorithmic Trading
As technology continues to advance, the future of algorithmic trading looks promising and exciting. Some trends to watch include:
- Artificial Intelligence and Deep Learning: More sophisticated AI models are being developed to analyze complex market patterns and make predictions.
- Big Data Analytics: The integration of alternative data sources, such as satellite imagery and social media sentiment, to gain unique insights.
- Quantum Computing: The potential use of quantum computers to solve complex optimization problems in portfolio management and risk assessment.
- Blockchain and Decentralized Finance (DeFi): The emergence of new trading opportunities and platforms in the cryptocurrency and DeFi space.
- Natural Language Processing (NLP): Advanced NLP techniques to analyze news, earnings reports, and other textual data for trading signals.
Conclusion
Algorithmic trading has revolutionized the financial markets, offering traders and investors powerful tools to implement complex strategies, reduce costs, and capitalize on fleeting market opportunities. As you embark on your journey into algorithmic trading, remember that success in this field requires a combination of programming skills, financial knowledge, and a deep understanding of market dynamics.
Start by mastering the basics, experimenting with simple strategies, and gradually building your expertise. Embrace continuous learning and stay adaptable, as the world of algorithmic trading is constantly evolving. With dedication and perseverance, you can develop the skills needed to create sophisticated trading algorithms and potentially thrive in this exciting and challenging field.
Remember, while algorithmic trading can be highly rewarding, it also carries significant risks. Always practice responsible risk management, thoroughly test your strategies, and never risk more capital than you can afford to lose. Happy coding and trading!