Algorithmic Thinking: Developing Problem-Solving Skills for Programming Success
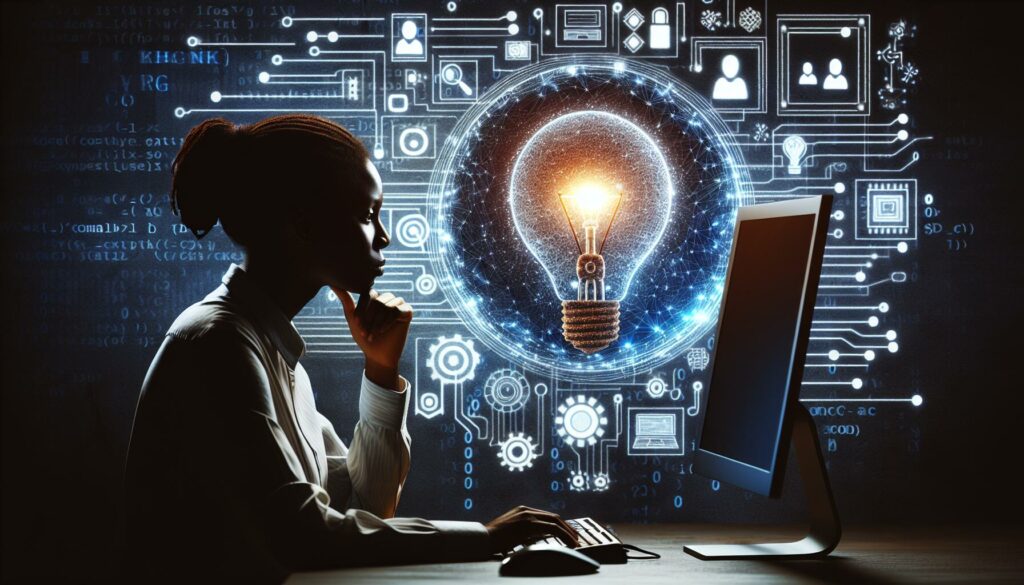
In the ever-evolving world of technology, the ability to think algorithmically and solve complex problems efficiently is a crucial skill for programmers and aspiring software engineers. Algorithmic thinking forms the foundation of computer science and is essential for tackling challenges in coding, data structures, and software development. In this comprehensive guide, we’ll explore the concept of algorithmic thinking, its importance in programming, and practical strategies to develop and enhance your problem-solving skills.
What is Algorithmic Thinking?
Algorithmic thinking is a cognitive process that involves breaking down complex problems into smaller, more manageable steps to arrive at a solution. It’s the ability to think logically and systematically, creating a series of well-defined instructions (algorithms) to solve a given problem. This approach is not limited to computer programming; it’s a valuable skill applicable to various fields and everyday life situations.
In the context of programming, algorithmic thinking enables developers to:
- Analyze problems efficiently
- Design effective solutions
- Optimize code for better performance
- Debug and troubleshoot issues systematically
- Adapt to new programming languages and paradigms
The Importance of Algorithmic Thinking in Programming
Mastering algorithmic thinking is crucial for several reasons:
- Problem-solving efficiency: It helps you approach complex problems methodically, breaking them down into manageable components.
- Code optimization: Understanding algorithms allows you to write more efficient and performant code.
- Scalability: Algorithmic thinking enables you to design solutions that can handle large-scale data and operations.
- Adaptability: The skills acquired through algorithmic thinking are transferable across different programming languages and paradigms.
- Career advancement: Strong problem-solving skills are highly valued in the tech industry, especially for roles at major companies like FAANG (Facebook, Amazon, Apple, Netflix, Google).
Key Components of Algorithmic Thinking
To develop strong algorithmic thinking skills, it’s essential to understand and practice the following components:
1. Problem Analysis
The first step in algorithmic thinking is to thoroughly analyze the problem at hand. This involves:
- Identifying the inputs and desired outputs
- Understanding the constraints and limitations
- Recognizing patterns or similarities to known problems
- Breaking down the problem into smaller, manageable sub-problems
2. Algorithm Design
Once you’ve analyzed the problem, the next step is to design an algorithm to solve it. This process includes:
- Choosing appropriate data structures
- Outlining the steps required to solve the problem
- Considering different approaches and selecting the most efficient one
- Ensuring the algorithm handles all possible scenarios and edge cases
3. Implementation
After designing the algorithm, you need to implement it in code. This stage involves:
- Translating the algorithm into a programming language
- Writing clean, readable, and maintainable code
- Implementing error handling and input validation
- Testing the implementation with various inputs
4. Optimization
Once you have a working solution, it’s important to optimize it for better performance. This includes:
- Analyzing the time and space complexity of your algorithm
- Identifying bottlenecks and inefficiencies
- Applying optimization techniques to improve performance
- Considering trade-offs between time complexity, space complexity, and code readability
Strategies to Develop Algorithmic Thinking Skills
Improving your algorithmic thinking abilities requires practice and dedication. Here are some effective strategies to enhance your skills:
1. Solve Coding Challenges Regularly
Engaging in coding challenges and competitive programming platforms can significantly improve your problem-solving skills. Websites like LeetCode, HackerRank, and CodeForces offer a wide range of problems to practice on. Start with easier problems and gradually increase the difficulty as you improve.
2. Study Classic Algorithms and Data Structures
Familiarize yourself with fundamental algorithms and data structures. Understanding these building blocks will help you recognize patterns and apply them to solve new problems. Some essential topics to study include:
- Sorting algorithms (e.g., Bubble Sort, Merge Sort, Quick Sort)
- Searching algorithms (e.g., Binary Search, Depth-First Search, Breadth-First Search)
- Data structures (e.g., Arrays, Linked Lists, Trees, Graphs, Hash Tables)
- Dynamic Programming
- Greedy Algorithms
3. Practice Pseudocode Writing
Before diving into coding, practice writing pseudocode for your algorithms. This helps you focus on the logical flow of your solution without getting bogged down in language-specific syntax. Pseudocode also makes it easier to communicate your ideas to others and spot potential issues early in the problem-solving process.
4. Analyze and Optimize Existing Code
Review and analyze code written by others, including open-source projects and solutions to coding challenges. Try to understand the reasoning behind different approaches and look for ways to optimize or improve the code. This practice will expose you to various problem-solving techniques and coding styles.
5. Collaborate and Discuss with Others
Engage in discussions with fellow programmers, participate in coding forums, and join programming communities. Explaining your thought process to others and learning from their approaches can significantly enhance your algorithmic thinking skills. Consider joining or forming a study group to tackle challenging problems together.
6. Implement Algorithms from Scratch
Challenge yourself to implement well-known algorithms and data structures from scratch. This exercise will deepen your understanding of how these fundamental building blocks work and improve your ability to design custom solutions for unique problems.
7. Use Visualization Tools
Utilize algorithm visualization tools to better understand how different algorithms work. Websites like VisuAlgo and Algorithm Visualizer provide interactive visualizations of various algorithms and data structures, making it easier to grasp complex concepts.
Common Problem-Solving Techniques
As you develop your algorithmic thinking skills, it’s important to familiarize yourself with common problem-solving techniques. These approaches can serve as a starting point when tackling new challenges:
1. Divide and Conquer
This technique involves breaking down a complex problem into smaller, more manageable sub-problems. Solve each sub-problem independently and then combine the results to solve the original problem. Examples of algorithms that use this approach include Merge Sort and Quick Sort.
2. Dynamic Programming
Dynamic Programming is used to solve problems with overlapping subproblems and optimal substructure. It involves breaking down a problem into simpler subproblems and storing the results of these subproblems to avoid redundant computations. This technique is particularly useful for optimization problems.
3. Greedy Algorithms
Greedy algorithms make locally optimal choices at each step with the hope of finding a global optimum. While not always guaranteed to find the best solution, greedy algorithms are often simple to implement and can be very efficient for certain types of problems.
4. Backtracking
Backtracking is used to solve problems that require exploring all possible solutions. It involves building a solution incrementally and abandoning a partial solution (“backtracking”) as soon as it determines that the solution cannot be completed.
5. Two-Pointer Technique
This technique uses two pointers to traverse a data structure, often moving in opposite directions. It’s commonly used to solve problems involving arrays or linked lists, such as finding a pair of elements that sum to a target value.
Applying Algorithmic Thinking to Real-World Problems
Algorithmic thinking isn’t just for solving coding challenges; it’s a valuable skill that can be applied to various real-world problems. Here are some examples of how algorithmic thinking can be used in different domains:
1. Optimizing Business Processes
Algorithmic thinking can be applied to streamline business operations, such as optimizing supply chain management, scheduling tasks, or improving customer service processes. By breaking down complex business problems into smaller components and designing efficient solutions, companies can significantly improve their productivity and reduce costs.
2. Data Analysis and Machine Learning
In the field of data science and machine learning, algorithmic thinking is crucial for developing efficient data processing pipelines, feature engineering, and model optimization. Data scientists use algorithmic approaches to handle large datasets, implement machine learning algorithms, and extract meaningful insights from complex data structures.
3. Financial Modeling and Trading Strategies
The financial industry heavily relies on algorithmic thinking for developing trading strategies, risk assessment models, and portfolio optimization algorithms. Quantitative analysts and traders use algorithmic approaches to analyze market trends, automate trading decisions, and manage large-scale financial operations.
4. Urban Planning and Transportation
Algorithmic thinking can be applied to solve complex urban planning problems, such as optimizing traffic flow, designing efficient public transportation networks, or managing waste collection routes. By breaking down these problems into smaller components and applying algorithmic solutions, city planners can improve the quality of life for residents and reduce environmental impact.
5. Healthcare and Medical Research
In the healthcare industry, algorithmic thinking is used to develop diagnostic tools, optimize patient scheduling, and analyze medical imaging data. Researchers also apply algorithmic approaches to analyze genomic data, simulate biological processes, and design drug discovery pipelines.
Tools and Resources for Developing Algorithmic Thinking Skills
To support your journey in developing strong algorithmic thinking skills, consider utilizing the following tools and resources:
1. Online Learning Platforms
- Coursera: Offers courses on algorithms and data structures from top universities.
- edX: Provides free online courses on computer science and programming fundamentals.
- AlgoCademy: Offers interactive coding tutorials and resources for algorithmic problem-solving.
2. Coding Challenge Websites
- LeetCode: Features a vast collection of coding problems with varying difficulty levels.
- HackerRank: Offers coding challenges and competitions across multiple domains.
- CodeForces: Hosts regular programming contests and provides a platform for competitive programming.
3. Algorithm Visualization Tools
- VisuAlgo: Provides interactive visualizations of various algorithms and data structures.
- Algorithm Visualizer: Allows users to visualize algorithms in action and create custom visualizations.
4. Books on Algorithms and Problem-Solving
- “Introduction to Algorithms” by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “The Algorithm Design Manual” by Steven S. Skiena
5. Integrated Development Environments (IDEs)
- Visual Studio Code: A versatile, open-source code editor with extensive plugin support.
- PyCharm: An IDE specifically designed for Python development, with features that support algorithmic problem-solving.
- IntelliJ IDEA: A powerful IDE for Java development, offering advanced code analysis and refactoring tools.
Conclusion
Developing strong algorithmic thinking skills is essential for success in programming and software engineering. By mastering the art of breaking down complex problems, designing efficient solutions, and optimizing your code, you’ll be well-equipped to tackle challenges in various domains of computer science and beyond.
Remember that improving your algorithmic thinking abilities is an ongoing process that requires consistent practice and dedication. Engage with coding challenges regularly, study classic algorithms and data structures, and apply these skills to real-world problems. As you progress, you’ll find that your problem-solving capabilities extend far beyond the realm of programming, enabling you to approach complex challenges in any field with confidence and creativity.
Whether you’re preparing for technical interviews at major tech companies or aiming to become a more proficient programmer, investing time in developing your algorithmic thinking skills will pay dividends throughout your career. Embrace the journey of continuous learning and problem-solving, and you’ll be well on your way to becoming a skilled and sought-after software engineer.