Algorithmic Strategies in Resource-Constrained Environments: Optimizing Performance with Limited Resources
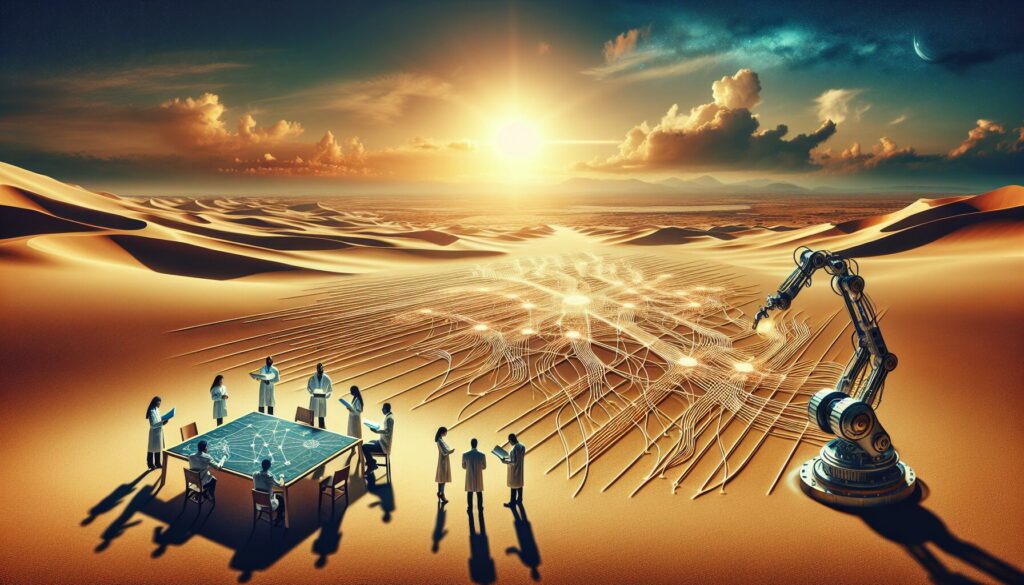
In the world of software development and computer science, efficiency is paramount. This is especially true when working in resource-constrained environments, where limitations on memory, processing power, or other system resources can significantly impact the performance of algorithms and applications. As developers, it’s crucial to understand and implement algorithmic strategies that can optimize performance even when resources are scarce. In this comprehensive guide, we’ll explore various techniques and approaches for designing and implementing efficient algorithms in resource-constrained environments.
Understanding Resource-Constrained Environments
Before diving into specific strategies, it’s essential to understand what we mean by “resource-constrained environments.” These are scenarios where one or more of the following limitations are present:
- Limited memory (RAM)
- Restricted processing power (CPU)
- Constrained storage capacity
- Limited network bandwidth
- Power consumption restrictions (e.g., in mobile or embedded devices)
These constraints can arise in various contexts, such as:
- Embedded systems and IoT devices
- Mobile applications
- Legacy hardware
- Cloud computing with cost constraints
- High-performance computing with large datasets
Now that we have a clear understanding of the challenges, let’s explore some key algorithmic strategies to overcome these limitations.
1. Space-Time Tradeoffs
One of the fundamental concepts in algorithm design is the tradeoff between space (memory) and time (processing). In resource-constrained environments, it’s often necessary to sacrifice one for the other. Here are some approaches to consider:
a) Time-Optimized Algorithms
When memory is scarce but processing power is available, consider algorithms that prioritize speed over memory usage. For example:
- Dynamic Programming: Store intermediate results to avoid redundant calculations
- Caching: Keep frequently accessed data in memory for quick retrieval
- Precomputation: Calculate and store results in advance for faster lookup during runtime
b) Space-Optimized Algorithms
When memory is at a premium, focus on algorithms that minimize space usage, even if they require more processing time:
- In-place algorithms: Modify data structures directly without using additional memory
- Streaming algorithms: Process data in a single pass without storing the entire dataset in memory
- Bit manipulation: Use bitwise operations to compress data and reduce memory footprint
Example: Space-Time Tradeoff in Fibonacci Calculation
Let’s consider the classic problem of calculating Fibonacci numbers to illustrate the space-time tradeoff:
Time-Optimized (Dynamic Programming):
def fibonacci_dp(n):
if n <= 1:
return n
fib = [0] * (n + 1)
fib[1] = 1
for i in range(2, n + 1):
fib[i] = fib[i-1] + fib[i-2]
return fib[n]
Space-Optimized:
def fibonacci_space_optimized(n):
if n <= 1:
return n
a, b = 0, 1
for _ in range(2, n + 1):
a, b = b, a + b
return b
The dynamic programming approach is faster for large values of n but uses O(n) space. The space-optimized version uses only O(1) space but may be slightly slower due to the lack of memoization.
2. Algorithmic Complexity Optimization
In resource-constrained environments, it’s crucial to choose algorithms with the most appropriate time and space complexity for the given constraints. Here are some strategies to optimize algorithmic complexity:
a) Asymptotic Analysis
Always consider the Big O notation of your algorithms and strive for the lowest possible complexity. For example:
- Replace O(n^2) algorithms with O(n log n) alternatives when possible
- Use linear-time algorithms (O(n)) instead of quadratic-time (O(n^2)) when dealing with large datasets
- Implement constant-time (O(1)) operations for frequently accessed data using appropriate data structures
b) Amortized Analysis
Consider the average-case performance of your algorithms over a sequence of operations. Some data structures, like dynamic arrays, have operations that are occasionally costly but offer good amortized performance.
c) Tailored Algorithms for Specific Constraints
Develop or choose algorithms that are specifically designed for resource-constrained environments. For example:
- External sorting algorithms for large datasets that don’t fit in memory
- Approximate algorithms that trade accuracy for reduced resource usage
- Incremental algorithms that can process data in small chunks
Example: Sorting in Constrained Memory
When dealing with large datasets that don’t fit in memory, consider using an external sorting algorithm like external merge sort:
def external_merge_sort(input_file, output_file, chunk_size):
# Step 1: Split the input file into sorted chunks
chunks = []
with open(input_file, 'r') as f:
while True:
chunk = f.readlines(chunk_size)
if not chunk:
break
chunk.sort()
temp_file = tempfile.NamedTemporaryFile(delete=False)
temp_file.writelines(chunk)
temp_file.close()
chunks.append(temp_file.name)
# Step 2: Merge the sorted chunks
with open(output_file, 'w') as out:
files = [open(chunk, 'r') for chunk in chunks]
out.writelines(heapq.merge(*files))
# Clean up temporary files
for file in files:
file.close()
for chunk in chunks:
os.unlink(chunk)
This algorithm allows sorting of datasets much larger than available memory by breaking the data into manageable chunks, sorting them individually, and then merging the sorted chunks.
3. Data Structure Selection and Optimization
Choosing the right data structures is crucial for optimizing both time and space complexity in resource-constrained environments. Here are some considerations:
a) Memory-Efficient Data Structures
- Bit arrays or bit vectors for storing boolean values
- Tries for efficient string storage and retrieval
- Sparse matrices for datasets with many zero or default values
- Bloom filters for space-efficient set membership tests
b) Cache-Friendly Data Structures
Design data structures that maximize cache efficiency:
- Array-based structures for better locality of reference
- Flat data structures instead of deeply nested ones
- Struct of Arrays (SoA) instead of Array of Structs (AoS) for better cache utilization
c) Compressed Data Structures
Use compression techniques to reduce memory footprint:
- Run-length encoding for sequences with repeated values
- Huffman coding for efficient data compression
- Succinct data structures that approach the information-theoretic lower bound for space usage
Example: Implementing a Bloom Filter
A Bloom filter is a space-efficient probabilistic data structure used to test whether an element is a member of a set. Here’s a simple implementation:
import math
import mmh3
class BloomFilter:
def __init__(self, size, num_hash_functions):
self.size = size
self.num_hash_functions = num_hash_functions
self.bit_array = [0] * size
def add(self, item):
for i in range(self.num_hash_functions):
index = mmh3.hash(item, i) % self.size
self.bit_array[index] = 1
def contains(self, item):
for i in range(self.num_hash_functions):
index = mmh3.hash(item, i) % self.size
if self.bit_array[index] == 0:
return False
return True
# Usage
bloom_filter = BloomFilter(size=1000, num_hash_functions=3)
bloom_filter.add("apple")
bloom_filter.add("banana")
print(bloom_filter.contains("apple")) # True
print(bloom_filter.contains("orange")) # False (probably)
This Bloom filter implementation uses significantly less memory than storing the full set of items while providing fast membership tests.
4. Parallel and Distributed Computing
When faced with resource constraints on a single machine, consider leveraging parallel and distributed computing techniques to distribute the workload:
a) Multi-threading and Concurrency
Utilize multiple cores or processors to perform computations in parallel:
- Use thread pools to manage and reuse threads efficiently
- Implement lock-free data structures to reduce contention
- Consider async/await patterns for I/O-bound operations
b) Distributed Algorithms
Design algorithms that can run across multiple machines or nodes:
- Map-Reduce for large-scale data processing
- Distributed hash tables for scalable key-value storage
- Gossip protocols for information dissemination in large networks
c) Load Balancing
Implement strategies to distribute work evenly across available resources:
- Dynamic load balancing to adapt to changing resource availability
- Work stealing algorithms for efficient task distribution
- Consistent hashing for distributed caching and storage systems
Example: Parallel Merge Sort
Here’s an example of how to implement a parallel merge sort using Python’s multiprocessing module:
import multiprocessing
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] <= right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def parallel_merge_sort(arr, num_processes):
if len(arr) <= 1:
return arr
chunk_size = len(arr) // num_processes
chunks = [arr[i:i+chunk_size] for i in range(0, len(arr), chunk_size)]
pool = multiprocessing.Pool(processes=num_processes)
sorted_chunks = pool.map(merge_sort, chunks)
pool.close()
pool.join()
while len(sorted_chunks) > 1:
merged = []
for i in range(0, len(sorted_chunks), 2):
if i + 1 < len(sorted_chunks):
merged.append(merge(sorted_chunks[i], sorted_chunks[i+1]))
else:
merged.append(sorted_chunks[i])
sorted_chunks = merged
return sorted_chunks[0]
# Usage
if __name__ == "__main__":
arr = [64, 34, 25, 12, 22, 11, 90]
num_processes = 4
sorted_arr = parallel_merge_sort(arr, num_processes)
print(sorted_arr)
This implementation divides the input array into chunks, sorts them in parallel using multiple processes, and then merges the sorted chunks.
5. I/O and Network Optimization
In many resource-constrained environments, I/O operations and network communication can be significant bottlenecks. Here are some strategies to optimize these aspects:
a) Efficient I/O Operations
- Use buffered I/O to reduce the number of system calls
- Implement asynchronous I/O for non-blocking operations
- Memory-map files for faster access to large datasets
- Batch write operations to improve throughput
b) Network Communication
- Implement efficient serialization formats (e.g., Protocol Buffers, MessagePack)
- Use compression for network payloads
- Implement connection pooling for reusing network connections
- Consider using UDP for low-latency, non-critical data transfer
c) Caching Strategies
- Implement multi-level caching (e.g., in-memory, disk-based, distributed)
- Use cache eviction policies tailored to your access patterns (e.g., LRU, LFU)
- Consider write-through vs. write-back caching based on consistency requirements
Example: Asynchronous File Reading
Here’s an example of how to implement asynchronous file reading in Python using the aiofiles library:
import asyncio
import aiofiles
async def read_file_async(filename):
async with aiofiles.open(filename, mode='r') as file:
content = await file.read()
return content
async def process_files(filenames):
tasks = [read_file_async(filename) for filename in filenames]
contents = await asyncio.gather(*tasks)
return contents
# Usage
if __name__ == "__main__":
filenames = ["file1.txt", "file2.txt", "file3.txt"]
loop = asyncio.get_event_loop()
results = loop.run_until_complete(process_files(filenames))
for filename, content in zip(filenames, results):
print(f"Content of {filename}: {content[:50]}...")
This asynchronous approach allows for concurrent file reading, which can significantly improve performance when dealing with multiple files or I/O-bound operations.
6. Approximation and Probabilistic Algorithms
In some cases, it may be necessary to trade off accuracy for reduced resource usage. Approximation and probabilistic algorithms can provide efficient solutions with acceptable error bounds:
a) Approximation Algorithms
- Use approximation algorithms for NP-hard problems (e.g., Traveling Salesman Problem)
- Implement polynomial-time approximation schemes (PTAS) when available
- Consider randomized approximation algorithms for faster convergence
b) Probabilistic Data Structures
- Count-Min Sketch for frequency estimation
- HyperLogLog for cardinality estimation
- Reservoir sampling for streaming data analysis
c) Monte Carlo Methods
- Use randomized algorithms for numerical integration
- Implement Monte Carlo simulations for complex system modeling
- Apply randomized algorithms for graph problems (e.g., min-cut)
Example: Reservoir Sampling
Reservoir sampling is a family of randomized algorithms for selecting a random sample of k items from a list of n items, where n is either a very large or unknown number. Here’s an implementation of reservoir sampling:
import random
def reservoir_sampling(stream, k):
reservoir = []
for i, item in enumerate(stream):
if i < k:
reservoir.append(item)
else:
j = random.randint(0, i)
if j < k:
reservoir[j] = item
return reservoir
# Usage
stream = range(1000000) # Simulate a large stream of data
sample_size = 10
result = reservoir_sampling(stream, sample_size)
print(f"Random sample of {sample_size} items: {result}")
This algorithm allows for sampling from a large or infinite stream of data while using only O(k) memory.
Conclusion
Designing and implementing efficient algorithms in resource-constrained environments requires a deep understanding of algorithmic complexity, data structures, and system-level optimizations. By applying the strategies discussed in this article, developers can create high-performance solutions that make the most of limited resources.
Key takeaways include:
- Always consider the space-time tradeoff and choose algorithms appropriate for your specific constraints.
- Optimize algorithmic complexity and choose data structures that minimize resource usage.
- Leverage parallel and distributed computing techniques when possible.
- Optimize I/O and network operations to reduce bottlenecks.
- Consider approximation and probabilistic algorithms when exact solutions are too resource-intensive.
As you work on projects in resource-constrained environments, remember that the best solution often depends on the specific constraints and requirements of your system. Continuously profile and benchmark your code to identify bottlenecks and areas for improvement. With practice and experience, you’ll develop the skills to create efficient algorithms that perform well even under tight resource constraints.