Algorithmic Strategies for Resource Allocation: Optimizing Efficiency in Complex Systems
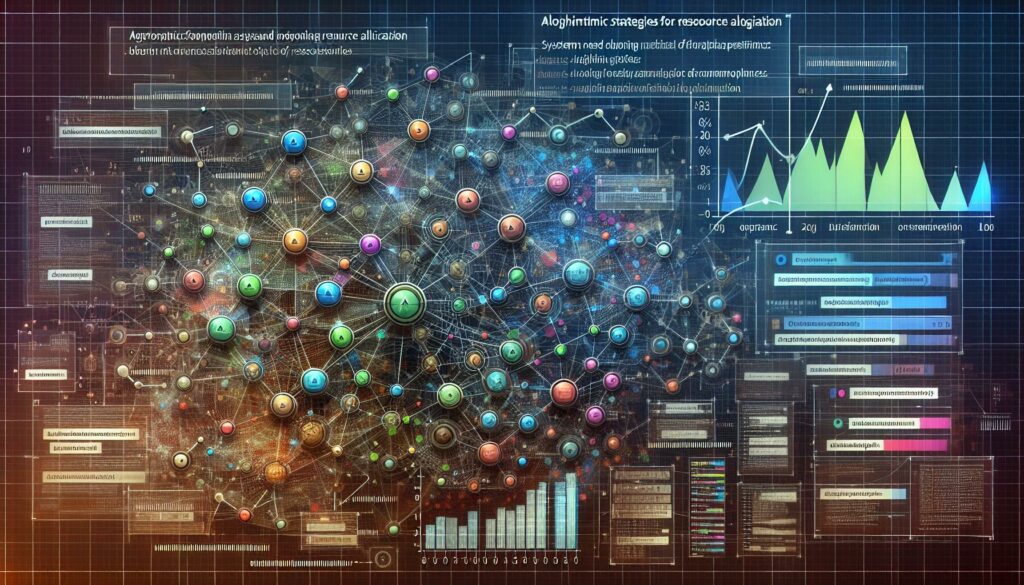
In the world of computer science and software engineering, resource allocation is a critical aspect of system design and optimization. As systems become increasingly complex and the demand for efficient resource utilization grows, developing effective algorithmic strategies for resource allocation has become more important than ever. This article will explore various algorithmic approaches to resource allocation, their applications, and the challenges they address in different domains.
Understanding Resource Allocation
Resource allocation refers to the process of assigning and managing limited resources to different tasks, processes, or entities within a system. These resources can include computational power, memory, network bandwidth, time slots, or any other finite asset that needs to be distributed efficiently. The goal of resource allocation algorithms is to optimize the use of these resources while meeting specific objectives, such as maximizing throughput, minimizing latency, or ensuring fairness among users.
Key Challenges in Resource Allocation
- Scarcity: Resources are often limited and must be carefully distributed to meet competing demands.
- Dynamism: Resource requirements and availability can change rapidly, requiring adaptive allocation strategies.
- Heterogeneity: Different tasks or users may have varying resource needs and priorities.
- Fairness: Ensuring equitable distribution of resources among multiple users or processes.
- Efficiency: Maximizing resource utilization while minimizing waste or idle time.
- Scalability: Allocation algorithms must be able to handle increasing system sizes and complexities.
Common Algorithmic Strategies for Resource Allocation
Let’s explore some of the most widely used algorithmic strategies for resource allocation, along with their strengths and limitations.
1. Greedy Algorithms
Greedy algorithms make locally optimal choices at each step, hoping to find a global optimum. While they don’t always guarantee the best overall solution, they are often simple to implement and can provide good approximations in many scenarios.
Example: Interval Scheduling
Consider a scenario where we need to schedule a maximum number of non-overlapping events. A greedy approach would be to sort the events by their end times and then iterate through them, selecting each event that doesn’t overlap with previously selected ones.
def interval_scheduling(events):
events.sort(key=lambda x: x[1]) # Sort by end time
scheduled = []
last_end = 0
for start, end in events:
if start >= last_end:
scheduled.append((start, end))
last_end = end
return scheduled
This greedy algorithm is optimal for the interval scheduling problem and runs in O(n log n) time due to the sorting step.
2. Dynamic Programming
Dynamic programming is a powerful technique for solving optimization problems by breaking them down into smaller subproblems. It’s particularly useful when the problem exhibits overlapping subproblems and optimal substructure.
Example: Knapsack Problem
The 0/1 Knapsack problem is a classic resource allocation problem where we need to select items with given weights and values to maximize the total value while staying within a weight limit.
def knapsack(values, weights, capacity):
n = len(values)
dp = [[0 for _ in range(capacity + 1)] for _ in range(n + 1)]
for i in range(1, n + 1):
for w in range(1, capacity + 1):
if weights[i-1] <= w:
dp[i][w] = max(values[i-1] + dp[i-1][w-weights[i-1]], dp[i-1][w])
else:
dp[i][w] = dp[i-1][w]
return dp[n][capacity]
This dynamic programming solution runs in O(n * capacity) time and space, where n is the number of items.
3. Linear Programming
Linear programming is a method for optimizing a linear objective function subject to linear constraints. It’s widely used in operations research and can be applied to various resource allocation problems.
Example: Production Planning
Suppose a company needs to decide how many units of two products to produce to maximize profit, given constraints on raw materials and labor hours. This can be formulated as a linear programming problem:
from scipy.optimize import linprog
# Objective function coefficients (negative for maximization)
c = [-20, -12] # Profit per unit for products A and B
# Inequality constraint matrix
A = [[1, 2], # Raw material 1 usage
[3, 1], # Raw material 2 usage
[1, 1]] # Labor hours
# Inequality constraint vector
b = [100, # Raw material 1 limit
150, # Raw material 2 limit
80] # Labor hours limit
# Solve the linear programming problem
res = linprog(c, A_ub=A, b_ub=b, method='simplex')
print(f"Optimal production: {res.x}")
print(f"Maximum profit: {-res.fun}")
This example uses the SciPy library to solve the linear programming problem, determining the optimal production quantities for each product.
4. Genetic Algorithms
Genetic algorithms are inspired by the process of natural selection and can be effective for complex optimization problems where traditional methods may struggle. They work by evolving a population of potential solutions over multiple generations.
Example: Task Scheduling
Consider a scenario where we need to assign tasks to processors to minimize the overall completion time. A genetic algorithm approach might look like this:
import random
def genetic_algorithm(tasks, processors, population_size, generations):
# Initialize population
population = [random_schedule(tasks, processors) for _ in range(population_size)]
for _ in range(generations):
# Evaluate fitness
fitness_scores = [evaluate_fitness(schedule) for schedule in population]
# Select parents
parents = selection(population, fitness_scores)
# Create new population through crossover and mutation
new_population = []
for i in range(0, len(parents), 2):
child1, child2 = crossover(parents[i], parents[i+1])
new_population.extend([mutate(child1), mutate(child2)])
population = new_population
# Return best solution
return min(population, key=evaluate_fitness)
def random_schedule(tasks, processors):
return [random.choice(processors) for _ in tasks]
def evaluate_fitness(schedule):
# Calculate completion time (lower is better)
return max(sum(task for task, proc in zip(tasks, schedule) if proc == p) for p in processors)
def selection(population, fitness_scores):
# Tournament selection
return [min(random.sample(population, 3), key=lambda x: fitness_scores[population.index(x)]) for _ in range(len(population))]
def crossover(parent1, parent2):
# Single-point crossover
point = random.randint(1, len(parent1) - 1)
return parent1[:point] + parent2[point:], parent2[:point] + parent1[point:]
def mutate(schedule):
# Random mutation
if random.random() < 0.1: # 10% mutation rate
i = random.randint(0, len(schedule) - 1)
schedule[i] = random.choice(processors)
return schedule
# Example usage
tasks = [10, 20, 15, 25, 30]
processors = [0, 1, 2]
best_schedule = genetic_algorithm(tasks, processors, population_size=50, generations=100)
print(f"Best schedule: {best_schedule}")
print(f"Completion time: {evaluate_fitness(best_schedule)}")
This genetic algorithm evolves schedules over multiple generations, using selection, crossover, and mutation operations to explore the solution space.
5. Reinforcement Learning
Reinforcement learning (RL) is a machine learning approach where an agent learns to make decisions by interacting with an environment. It can be particularly useful for dynamic resource allocation problems where the optimal policy may change over time.
Example: Dynamic Resource Allocation in Cloud Computing
In a cloud computing environment, we might use reinforcement learning to dynamically allocate virtual machines (VMs) to incoming tasks. Here’s a simplified example using Q-learning:
import numpy as np
class CloudEnvironment:
def __init__(self, num_vms, max_tasks):
self.num_vms = num_vms
self.max_tasks = max_tasks
self.state = np.zeros(num_vms, dtype=int)
self.task_queue = []
def step(self, action):
# Allocate task to VM
self.state[action] += 1
reward = -np.sum(self.state) # Negative sum of allocated tasks (we want to minimize)
# Simulate task completion
self.state = np.maximum(self.state - 1, 0)
# Generate new task with 50% probability
if np.random.random() < 0.5 and len(self.task_queue) < self.max_tasks:
self.task_queue.append(1)
done = len(self.task_queue) == 0 and np.sum(self.state) == 0
return self.get_state(), reward, done
def get_state(self):
return tuple(self.state) + (len(self.task_queue),)
def q_learning(env, num_episodes, learning_rate, discount_factor, epsilon):
q_table = {}
for episode in range(num_episodes):
state = env.get_state()
done = False
while not done:
if np.random.random() < epsilon:
action = np.random.randint(env.num_vms)
else:
action = np.argmin([q_table.get((state, a), 0) for a in range(env.num_vms)])
next_state, reward, done = env.step(action)
if state not in q_table:
q_table[state] = np.zeros(env.num_vms)
best_next_action = np.argmin([q_table.get((next_state, a), 0) for a in range(env.num_vms)])
td_target = reward + discount_factor * q_table.get((next_state, best_next_action), 0)
td_error = td_target - q_table[state][action]
q_table[state][action] += learning_rate * td_error
state = next_state
return q_table
# Example usage
env = CloudEnvironment(num_vms=3, max_tasks=5)
q_table = q_learning(env, num_episodes=10000, learning_rate=0.1, discount_factor=0.9, epsilon=0.1)
# Use the learned policy
state = env.get_state()
while True:
action = np.argmin([q_table.get((state, a), 0) for a in range(env.num_vms)])
print(f"State: {state}, Action: Allocate to VM {action}")
state, reward, done = env.step(action)
if done:
break
This reinforcement learning approach learns a policy for allocating tasks to VMs over time, adapting to the dynamic nature of the cloud environment.
Choosing the Right Strategy
Selecting the most appropriate algorithmic strategy for resource allocation depends on various factors:
- Problem characteristics: Consider the nature of the problem, its constraints, and objectives.
- Scalability requirements: Ensure the chosen algorithm can handle the expected system size and growth.
- Time complexity: Consider the runtime performance needed for real-time or near-real-time allocation decisions.
- Adaptability: For dynamic environments, choose strategies that can adapt to changing conditions.
- Implementation complexity: Balance the sophistication of the algorithm with the ease of implementation and maintenance.
- Domain-specific considerations: Take into account any industry-specific requirements or best practices.
Advanced Topics in Resource Allocation
As systems become more complex and the demand for efficient resource utilization grows, researchers and practitioners are exploring advanced topics in resource allocation:
1. Multi-objective Optimization
Many real-world resource allocation problems involve multiple, often conflicting objectives. For example, in a cloud computing environment, we might want to minimize energy consumption while maximizing performance and ensuring fairness among users. Multi-objective optimization techniques, such as Pareto optimization or weighted sum methods, can help find solutions that balance these competing goals.
2. Distributed Resource Allocation
In large-scale distributed systems, centralized resource allocation algorithms may not be feasible due to communication overhead and scalability issues. Distributed algorithms that allow nodes to make local decisions based on limited information are becoming increasingly important. Techniques like gossip protocols, market-based approaches, and distributed consensus algorithms are being applied to solve resource allocation problems in distributed settings.
3. Online and Stochastic Algorithms
Many resource allocation problems involve uncertainty and require decisions to be made in real-time without complete information about future events. Online algorithms and stochastic optimization techniques are designed to handle such scenarios. These approaches aim to make decisions that perform well in expectation or provide guarantees on worst-case performance.
4. Machine Learning for Resource Allocation
Beyond reinforcement learning, other machine learning techniques are being applied to resource allocation problems. For example:
- Supervised learning can be used to predict resource demands based on historical data.
- Unsupervised learning techniques like clustering can help identify patterns in resource usage and group similar tasks or users.
- Deep learning models can capture complex relationships between system states and optimal allocation decisions.
5. Quantum Algorithms for Resource Allocation
As quantum computing technology advances, researchers are exploring quantum algorithms for solving resource allocation problems. Quantum approaches have the potential to solve certain optimization problems exponentially faster than classical algorithms, which could lead to breakthroughs in handling large-scale resource allocation challenges.
Real-world Applications of Resource Allocation Algorithms
Resource allocation algorithms find applications in numerous domains, including:
1. Cloud Computing and Data Centers
Efficient allocation of virtual machines, containers, and other resources to maximize utilization and minimize energy consumption while meeting service level agreements (SLAs).
2. Wireless Networks
Allocation of spectrum, time slots, and power in cellular networks to optimize coverage, capacity, and quality of service.
3. Supply Chain Management
Optimizing the distribution of inventory, production capacity, and transportation resources across a supply chain network.
4. Healthcare Systems
Allocating medical resources such as hospital beds, staff, and equipment to maximize patient care and minimize waiting times.
5. Financial Portfolio Management
Allocating investment capital across different assets to balance risk and return according to investor preferences.
6. Transportation and Logistics
Optimizing routes, vehicle assignments, and cargo distribution in logistics networks to minimize costs and delivery times.
Challenges and Future Directions
As we continue to develop and refine resource allocation algorithms, several challenges and opportunities lie ahead:
1. Handling Uncertainty and Dynamism
Developing algorithms that can effectively adapt to rapidly changing environments and handle uncertainty in resource availability and demand remains an ongoing challenge.
2. Scalability and Real-time Performance
As systems grow in size and complexity, ensuring that resource allocation algorithms can scale efficiently and make decisions in real-time becomes increasingly important.
3. Fairness and Ethical Considerations
Ensuring fair allocation of resources, especially in systems that impact human lives (e.g., healthcare, education), requires careful consideration of ethical implications and the development of algorithms that can balance efficiency with equity.
4. Integration with Emerging Technologies
As new technologies like edge computing, 5G networks, and Internet of Things (IoT) devices become more prevalent, resource allocation algorithms will need to evolve to handle the unique challenges posed by these distributed, heterogeneous environments.
5. Explainability and Transparency
As resource allocation algorithms become more complex, especially those based on machine learning techniques, ensuring their decisions are explainable and transparent to stakeholders becomes crucial for building trust and enabling effective oversight.
Conclusion
Algorithmic strategies for resource allocation play a crucial role in optimizing the efficiency of complex systems across various domains. From classical approaches like greedy algorithms and dynamic programming to more advanced techniques leveraging machine learning and quantum computing, the field continues to evolve to meet the challenges of increasingly complex and dynamic environments.
As we look to the future, the development of resource allocation algorithms that can handle uncertainty, scale to large systems, ensure fairness, and integrate with emerging technologies will be key to addressing the resource management challenges of tomorrow. By combining insights from computer science, operations research, and domain-specific knowledge, we can continue to push the boundaries of what’s possible in resource allocation, leading to more efficient, sustainable, and equitable systems across industries.