Algorithmic Solutions for Scheduling Problems: Optimizing Time and Resources
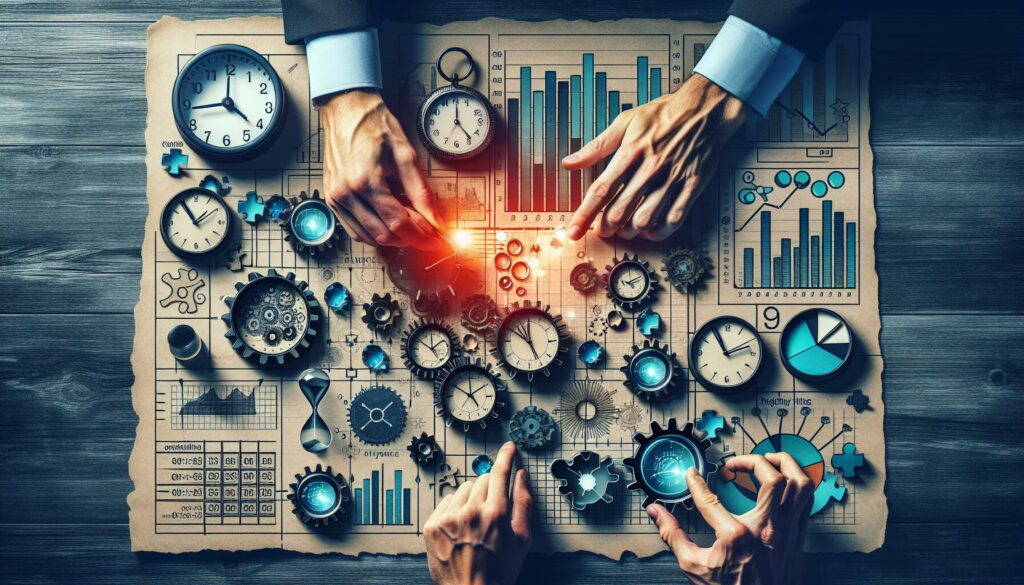
In the fast-paced world of technology and business, efficient scheduling is crucial for maximizing productivity and resource utilization. Whether you’re managing a team of developers, organizing a complex project, or simply trying to optimize your personal time, understanding and implementing algorithmic solutions for scheduling problems can make a significant difference. This article delves into the world of scheduling algorithms, exploring their applications, common challenges, and practical implementations that can help you tackle real-world scheduling issues.
Understanding Scheduling Problems
Scheduling problems are a class of optimization problems that involve assigning resources to tasks over time. These problems are ubiquitous in various domains, including:
- Project management
- Manufacturing and production planning
- Transportation and logistics
- Healthcare (e.g., staff scheduling, patient appointments)
- Education (e.g., course timetabling)
- Computer systems (e.g., CPU scheduling, network packet scheduling)
The goal of scheduling algorithms is to find optimal or near-optimal solutions that satisfy various constraints and objectives, such as minimizing completion time, maximizing resource utilization, or balancing workload distribution.
Common Types of Scheduling Problems
Before diving into algorithmic solutions, it’s essential to understand the different types of scheduling problems you might encounter:
1. Single Machine Scheduling
In this simplest form of scheduling, tasks need to be processed on a single machine or resource. The objective is typically to minimize the total completion time or meet specific deadlines.
2. Parallel Machine Scheduling
This involves scheduling tasks across multiple identical machines or resources. The challenge is to distribute the workload efficiently to minimize the overall completion time or maximize resource utilization.
3. Job Shop Scheduling
In job shop scheduling, multiple jobs need to be processed on different machines in a specific order. This type of problem is common in manufacturing environments where products go through various stages of production.
4. Flow Shop Scheduling
Similar to job shop scheduling, but all jobs follow the same sequence of operations on the machines. The goal is to find the optimal order of jobs to minimize the total completion time.
5. Resource-Constrained Project Scheduling
This involves scheduling tasks in a project while considering limited resources. The objective is to minimize the project duration while respecting resource constraints and task dependencies.
Algorithmic Approaches to Scheduling Problems
Now that we’ve outlined the types of scheduling problems, let’s explore some algorithmic approaches to solve them:
1. Greedy Algorithms
Greedy algorithms make locally optimal choices at each step, hoping to find a global optimum. While they don’t always produce the best solution, they are often fast and can provide good approximations for many scheduling problems.
Example: Shortest Job First (SJF)
The Shortest Job First algorithm is a simple greedy approach for single machine scheduling. It orders jobs based on their processing time, executing the shortest job first.
def shortest_job_first(jobs):
return sorted(jobs, key=lambda x: x.processing_time)
# Usage
jobs = [Job(1, 10), Job(2, 5), Job(3, 8)]
scheduled_jobs = shortest_job_first(jobs)
for job in scheduled_jobs:
print(f"Job {job.id}: Processing time {job.processing_time}")
2. Dynamic Programming
Dynamic programming is a powerful technique for solving optimization problems by breaking them down into smaller subproblems. It’s particularly useful for scheduling problems with overlapping subproblems and optimal substructure.
Example: Weighted Job Scheduling
Consider a problem where each job has a start time, end time, and profit. The goal is to find the maximum profit schedule without overlapping jobs.
def weighted_job_scheduling(jobs):
jobs.sort(key=lambda x: x.end_time)
n = len(jobs)
dp = [0] * n
dp[0] = jobs[0].profit
for i in range(1, n):
profit = jobs[i].profit
last_non_conflicting = binary_search(jobs, i)
if last_non_conflicting != -1:
profit += dp[last_non_conflicting]
dp[i] = max(profit, dp[i-1])
return dp[n-1]
def binary_search(jobs, index):
low, high = 0, index - 1
while low <= high:
mid = (low + high) // 2
if jobs[mid].end_time <= jobs[index].start_time:
if jobs[mid+1].end_time <= jobs[index].start_time:
low = mid + 1
else:
return mid
else:
high = mid - 1
return -1
# Usage
jobs = [Job(1, 2, 50), Job(3, 5, 20), Job(6, 19, 100), Job(2, 100, 200)]
max_profit = weighted_job_scheduling(jobs)
print(f"Maximum profit: {max_profit}")
3. Heuristic Algorithms
Heuristic algorithms are problem-solving approaches that aim to find good solutions quickly, even if they’re not guaranteed to be optimal. They’re particularly useful for complex scheduling problems where finding an exact solution is computationally expensive.
Example: Simulated Annealing for Job Shop Scheduling
Simulated annealing is a probabilistic technique for approximating the global optimum of a given function. It’s inspired by the annealing process in metallurgy and can be applied to job shop scheduling problems.
import random
import math
def simulated_annealing(initial_solution, temperature, cooling_rate, iterations):
current_solution = initial_solution
current_cost = calculate_cost(current_solution)
best_solution = current_solution
best_cost = current_cost
for i in range(iterations):
neighbor = generate_neighbor(current_solution)
neighbor_cost = calculate_cost(neighbor)
if neighbor_cost < current_cost or random.random() < math.exp((current_cost - neighbor_cost) / temperature):
current_solution = neighbor
current_cost = neighbor_cost
if current_cost < best_cost:
best_solution = current_solution
best_cost = current_cost
temperature *= cooling_rate
return best_solution, best_cost
def generate_neighbor(solution):
# Implement a method to generate a neighboring solution
pass
def calculate_cost(solution):
# Implement a method to calculate the cost of a solution
pass
# Usage
initial_solution = generate_initial_solution()
best_solution, best_cost = simulated_annealing(initial_solution, temperature=1000, cooling_rate=0.995, iterations=10000)
print(f"Best solution cost: {best_cost}")
4. Genetic Algorithms
Genetic algorithms are inspired by the process of natural selection and can be effective for complex scheduling problems. They work by evolving a population of potential solutions over multiple generations.
Example: Genetic Algorithm for Flow Shop Scheduling
import random
def genetic_algorithm(population_size, generations, mutation_rate):
population = initialize_population(population_size)
for _ in range(generations):
fitness_scores = [calculate_fitness(individual) for individual in population]
parents = select_parents(population, fitness_scores)
offspring = crossover(parents)
mutate(offspring, mutation_rate)
population = offspring
best_individual = max(population, key=calculate_fitness)
return best_individual
def initialize_population(population_size):
# Initialize a random population of schedules
pass
def calculate_fitness(individual):
# Calculate the fitness of a schedule
pass
def select_parents(population, fitness_scores):
# Select parents for reproduction using tournament selection
pass
def crossover(parents):
# Perform crossover to create offspring
pass
def mutate(offspring, mutation_rate):
# Apply mutation to offspring
pass
# Usage
best_schedule = genetic_algorithm(population_size=100, generations=1000, mutation_rate=0.01)
print(f"Best schedule: {best_schedule}")
Advanced Scheduling Techniques
As scheduling problems become more complex, researchers and practitioners have developed advanced techniques to tackle them effectively:
1. Constraint Programming
Constraint programming is a paradigm for solving combinatorial problems by expressing them as a set of constraints. It’s particularly useful for scheduling problems with complex constraints and objectives.
Example: Using Google OR-Tools for Job Shop Scheduling
from ortools.sat.python import cp_model
def solve_job_shop(jobs_data):
model = cp_model.CpModel()
machines_count = max(task[0] for job in jobs_data for task in job) + 1
all_machines = range(machines_count)
# Compute horizon dynamically
horizon = sum(task[1] for job in jobs_data for task in job)
# Named tuple to store information about created variables.
task_type = collections.namedtuple('task_type', 'start end interval')
# Create job intervals and add to the corresponding machine lists.
all_tasks = {}
machine_to_intervals = collections.defaultdict(list)
for job_id, job in enumerate(jobs_data):
for task_id, task in enumerate(job):
machine = task[0]
duration = task[1]
suffix = '_%i_%i' % (job_id, task_id)
start_var = model.NewIntVar(0, horizon, 'start' + suffix)
end_var = model.NewIntVar(0, horizon, 'end' + suffix)
interval_var = model.NewIntervalVar(start_var, duration, end_var,
'interval' + suffix)
all_tasks[job_id, task_id] = task_type(start=start_var,
end=end_var,
interval=interval_var)
machine_to_intervals[machine].append(interval_var)
# Create and add disjunctive constraints.
for machine in all_machines:
model.AddNoOverlap(machine_to_intervals[machine])
# Precedences inside a job.
for job_id, job in enumerate(jobs_data):
for task_id in range(len(job) - 1):
model.Add(all_tasks[job_id, task_id + 1].start >= all_tasks[job_id, task_id].end)
# Objective: minimize the makespan (maximum end time of all tasks)
obj_var = model.NewIntVar(0, horizon, 'makespan')
model.AddMaxEquality(obj_var, [all_tasks[job_id, len(job) - 1].end
for job_id, job in enumerate(jobs_data)])
model.Minimize(obj_var)
# Solve model.
solver = cp_model.CpSolver()
status = solver.Solve(model)
if status == cp_model.OPTIMAL or status == cp_model.FEASIBLE:
print('Solution:')
for job_id, job in enumerate(jobs_data):
for task_id, task in enumerate(job):
start = solver.Value(all_tasks[job_id, task_id].start)
machine = task[0]
print(' Job %i task %i starts at %i on machine %i' % (job_id, task_id, start, machine))
print('Optimal makespan: %i' % solver.ObjectiveValue())
else:
print('No solution found.')
# Usage
jobs_data = [
[(0, 3), (1, 2), (2, 2)], # Job 0
[(0, 2), (2, 1), (1, 4)], # Job 1
[(1, 4), (2, 3)] # Job 2
]
solve_job_shop(jobs_data)
2. Machine Learning for Scheduling
Recent advancements in machine learning have opened up new possibilities for tackling scheduling problems. Techniques such as reinforcement learning and neural networks can be used to learn effective scheduling policies from data.
Example: Reinforcement Learning for Dynamic Scheduling
import gym
import numpy as np
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
from tensorflow.keras.optimizers import Adam
class SchedulingEnvironment(gym.Env):
def __init__(self):
# Define the action and observation space
self.action_space = gym.spaces.Discrete(num_actions)
self.observation_space = gym.spaces.Box(low=0, high=1, shape=(num_features,), dtype=np.float32)
def step(self, action):
# Implement the environment dynamics
pass
def reset(self):
# Reset the environment to initial state
pass
def build_model(input_shape, output_shape):
model = Sequential([
Dense(24, activation='relu', input_shape=input_shape),
Dense(24, activation='relu'),
Dense(output_shape, activation='linear')
])
model.compile(optimizer=Adam(learning_rate=0.001), loss='mse')
return model
def train_rl_scheduler(env, episodes=1000):
model = build_model(env.observation_space.shape, env.action_space.n)
for episode in range(episodes):
state = env.reset()
done = False
while not done:
action = np.argmax(model.predict(state.reshape(1, -1))[0])
next_state, reward, done, _ = env.step(action)
target = reward + 0.99 * np.max(model.predict(next_state.reshape(1, -1))[0])
target_vec = model.predict(state.reshape(1, -1))[0]
target_vec[action] = target
model.fit(state.reshape(1, -1), target_vec.reshape(1, -1), epochs=1, verbose=0)
state = next_state
return model
# Usage
env = SchedulingEnvironment()
trained_model = train_rl_scheduler(env)
print("RL model trained for dynamic scheduling")
Practical Considerations for Implementing Scheduling Algorithms
When implementing scheduling algorithms in real-world scenarios, consider the following factors:
1. Scalability
Ensure that your chosen algorithm can handle the scale of your scheduling problem. Some algorithms that work well for small instances may become impractical for larger problems.
2. Real-time Performance
In dynamic environments where schedules need to be updated frequently, consider algorithms that can provide good solutions quickly, even if they’re not optimal.
3. Constraint Handling
Real-world scheduling often involves complex constraints. Choose algorithms or frameworks that can effectively handle various types of constraints, such as time windows, resource limitations, and precedence relationships.
4. Robustness
Develop scheduling solutions that can handle uncertainties and disruptions. This might involve incorporating stochastic elements or implementing rescheduling mechanisms.
5. Integration with Existing Systems
Consider how your scheduling solution will integrate with existing systems and processes. This may involve developing APIs, data pipelines, or user interfaces to make the scheduling system accessible and usable.
Conclusion
Algorithmic solutions for scheduling problems are essential tools for optimizing time and resource allocation in various domains. From simple greedy algorithms to advanced machine learning techniques, there’s a wide range of approaches available to tackle different types of scheduling challenges.
As you develop your skills in algorithmic thinking and problem-solving, mastering these scheduling techniques will prove invaluable in your career as a software engineer or data scientist. Whether you’re preparing for technical interviews at top tech companies or working on real-world projects, the ability to design and implement efficient scheduling algorithms will set you apart in the competitive world of technology.
Remember that choosing the right algorithm depends on the specific requirements of your scheduling problem, including the scale, constraints, and performance needs. As you gain experience, you’ll develop intuition for selecting and adapting algorithms to suit different scenarios.
Keep practicing, experimenting with different approaches, and staying up-to-date with the latest advancements in scheduling algorithms. With dedication and continuous learning, you’ll be well-equipped to tackle even the most complex scheduling challenges in your future projects and career.