AlgoCademy vs. LeetCode: Which Platform Offers Better Dynamic Programming Practice?
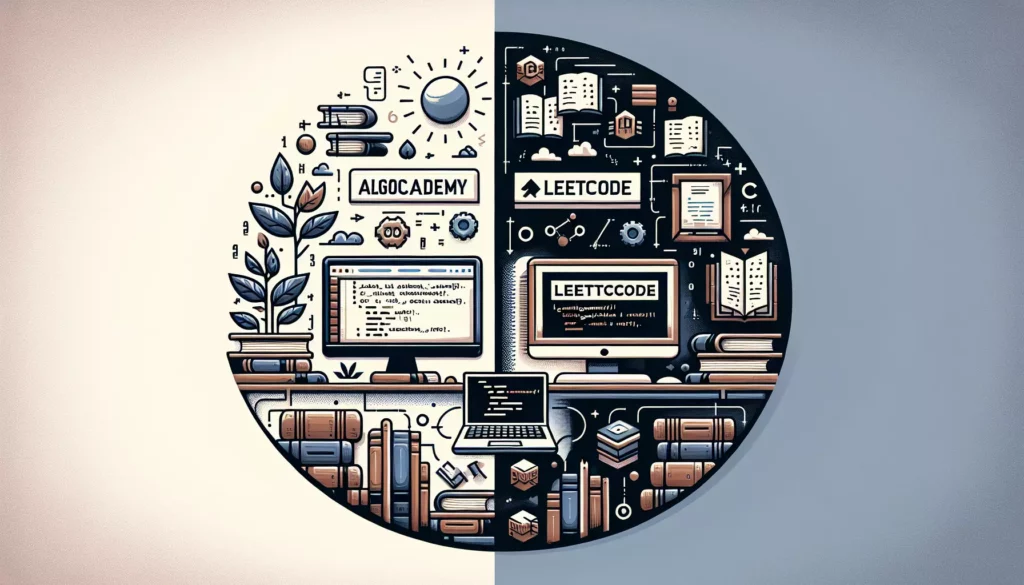
In the ever-evolving world of software development, mastering dynamic programming (DP) is crucial for aspiring programmers and seasoned professionals alike. As the demand for skilled developers continues to grow, platforms like AlgoCademy and LeetCode have emerged as popular choices for honing coding skills, particularly in areas like dynamic programming. But which platform offers better dynamic programming practice? In this comprehensive comparison, we’ll dive deep into the features, strengths, and weaknesses of both AlgoCademy and LeetCode to help you make an informed decision about where to focus your DP learning efforts.
Understanding Dynamic Programming
Before we delve into the comparison, let’s briefly recap what dynamic programming is and why it’s so important in the world of coding and algorithms.
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It is particularly useful for optimization problems and is based on the principle of optimal substructure. The key idea behind DP is to store the results of subproblems to avoid redundant computations, thereby improving the overall efficiency of the algorithm.
Some classic examples of dynamic programming problems include:
- Fibonacci sequence
- Longest Common Subsequence
- Knapsack problem
- Matrix Chain Multiplication
- Shortest Path algorithms
Mastering dynamic programming is essential for several reasons:
- It’s a common topic in technical interviews, especially for positions at top tech companies.
- DP techniques can significantly optimize algorithm performance in real-world applications.
- Understanding DP enhances overall problem-solving skills and algorithmic thinking.
Now that we’ve established the importance of dynamic programming, let’s compare how AlgoCademy and LeetCode approach teaching and practicing this crucial concept.
AlgoCademy: A Comprehensive Coding Education Platform
AlgoCademy is a relatively new player in the coding education space, but it has quickly gained attention for its innovative approach to teaching programming concepts, including dynamic programming.
Key Features of AlgoCademy
- Interactive Tutorials: AlgoCademy offers step-by-step, interactive tutorials that guide learners through complex concepts like dynamic programming.
- AI-Powered Assistance: The platform incorporates AI technology to provide personalized feedback and hints, helping users overcome challenges in their learning journey.
- Comprehensive Curriculum: AlgoCademy covers a wide range of topics, from basic programming concepts to advanced algorithms, including a strong focus on dynamic programming.
- Progress Tracking: Users can easily track their progress and identify areas for improvement through detailed analytics and performance metrics.
- Community Features: AlgoCademy fosters a supportive learning environment through discussion forums and peer collaboration tools.
Dynamic Programming on AlgoCademy
AlgoCademy’s approach to teaching dynamic programming is characterized by its structured, comprehensive methodology:
- Foundational Concepts: The platform starts by introducing the basic principles of dynamic programming, ensuring that learners have a solid grasp of the underlying concepts.
- Gradual Progression: Problems are presented in a carefully curated order, allowing users to build upon their knowledge incrementally.
- Visual Aids: AlgoCademy utilizes diagrams, animations, and interactive visualizations to help users understand the flow of DP algorithms.
- Real-world Applications: The platform emphasizes the practical applications of dynamic programming, helping users understand how these concepts apply in real-world scenarios.
- Hands-on Practice: Each concept is accompanied by coding exercises and challenges, allowing users to implement DP solutions in various programming languages.
Strengths of AlgoCademy for Dynamic Programming Practice
- Structured Learning Path: The platform provides a well-organized curriculum that systematically covers all aspects of dynamic programming.
- Interactive Learning Experience: With its AI-powered assistance and interactive tutorials, AlgoCademy offers a more engaging and personalized learning experience.
- Comprehensive Explanations: Each problem comes with detailed explanations, multiple approaches, and time/space complexity analysis.
- Focus on Understanding: AlgoCademy emphasizes conceptual understanding over rote memorization, helping users develop a deeper grasp of DP principles.
- Beginner-Friendly: The platform is particularly well-suited for beginners and intermediate learners who are new to dynamic programming concepts.
Potential Drawbacks
- Limited Problem Set: As a newer platform, AlgoCademy may have a smaller collection of DP problems compared to more established platforms.
- Less Focus on Competition: For users seeking a more competitive environment, AlgoCademy’s collaborative approach might not provide the same level of challenge.
- Newer Community: The user community on AlgoCademy is still growing, which may result in fewer user-generated solutions and discussions compared to more established platforms.
LeetCode: The Go-To Platform for Coding Interviews
LeetCode has long been considered the gold standard for coding interview preparation, including practice in dynamic programming. Let’s explore what LeetCode offers in terms of DP practice.
Key Features of LeetCode
- Vast Problem Database: LeetCode boasts an extensive collection of coding problems, including a significant number of dynamic programming challenges.
- Company-Specific Questions: The platform offers curated lists of problems that are frequently asked in interviews at specific tech companies.
- Contest and Ranking System: Regular coding contests allow users to test their skills against others and track their global ranking.
- Discussion Forums: Each problem has an associated discussion section where users can share solutions and discuss different approaches.
- Mock Interviews: LeetCode provides mock interview sessions to simulate real interview experiences.
Dynamic Programming on LeetCode
LeetCode’s approach to dynamic programming practice is characterized by its breadth and depth of problems:
- Problem Variety: The platform offers a wide range of DP problems, from easy to hard difficulty levels.
- Tags and Categories: Problems are tagged with relevant concepts, making it easy to find and focus on dynamic programming challenges.
- Solution Submissions: Users can submit their solutions in multiple programming languages and receive immediate feedback on correctness and efficiency.
- Editorial Solutions: Many problems come with official editorial solutions that explain the dynamic programming approach in detail.
- User-generated Content: The discussion forums often contain multiple solutions and explanations from the community, providing diverse perspectives on solving DP problems.
Strengths of LeetCode for Dynamic Programming Practice
- Extensive Problem Set: LeetCode offers a vast array of dynamic programming problems, covering virtually every aspect and variation of DP concepts.
- Interview-Focused: The platform is specifically designed to prepare users for coding interviews, with many DP problems mirroring those asked in real interviews.
- Competitive Environment: Regular contests and global rankings provide motivation and allow users to benchmark their skills against others.
- Community Insights: The active user community contributes a wealth of solutions, explanations, and tips for tackling DP problems.
- Performance Metrics: Detailed statistics on solution runtime and memory usage help users optimize their DP implementations.
Potential Drawbacks
- Steep Learning Curve: For beginners, LeetCode’s vast problem set can be overwhelming, and the platform assumes a certain level of prior knowledge.
- Less Structured Learning Path: Unlike AlgoCademy, LeetCode doesn’t provide a structured curriculum for learning dynamic programming from scratch.
- Limited Explanations: While editorial solutions are available for many problems, they may not always provide the depth of explanation needed for complex DP concepts.
- Focus on Solution over Understanding: The platform’s emphasis on solving problems quickly can sometimes lead to users memorizing solutions rather than truly understanding the underlying principles.
Head-to-Head Comparison: AlgoCademy vs. LeetCode for Dynamic Programming
Now that we’ve examined both platforms in detail, let’s compare them directly across several key aspects of dynamic programming practice:
1. Learning Approach
AlgoCademy: Offers a structured, tutorial-based approach with a focus on building a strong foundation in DP concepts. The platform guides users through progressively more challenging problems, ensuring a thorough understanding of each concept before moving on.
LeetCode: Provides a more self-directed learning experience, where users can choose from a vast array of problems. While this offers flexibility, it may be less optimal for systematic learning of DP concepts.
2. Problem Variety and Quantity
AlgoCademy: While offering a carefully curated selection of DP problems, the total number may be smaller compared to LeetCode. However, each problem is designed to reinforce specific DP concepts and techniques.
LeetCode: Boasts an extensive collection of DP problems, covering a wide range of difficulty levels and variations. This breadth ensures exposure to diverse DP scenarios but may be overwhelming for beginners.
3. Explanation and Guidance
AlgoCademy: Excels in providing detailed explanations, step-by-step guidance, and visual aids to help users understand DP concepts. The AI-powered assistance offers personalized help when users struggle with a problem.
LeetCode: Offers editorial solutions and community discussions, which can be highly informative. However, the depth and quality of explanations can vary, and personalized guidance is limited.
4. Interactive Learning
AlgoCademy: Features interactive tutorials and visualizations that make learning DP more engaging and accessible, especially for visual learners.
LeetCode: Focuses more on problem-solving and less on interactive learning experiences. While effective for practice, it may be less engaging for users who benefit from more interactive content.
5. Community and Collaboration
AlgoCademy: Emphasizes collaborative learning through its community features, encouraging users to work together and share insights. However, as a newer platform, the community may be smaller compared to LeetCode.
LeetCode: Has a large, active community that contributes a wealth of solutions and discussions. This can be invaluable for seeing different approaches to DP problems, but may also be overwhelming for newcomers.
6. Interview Preparation
AlgoCademy: While designed to prepare users for technical interviews, including those at major tech companies, the platform’s primary focus is on comprehensive learning rather than just interview preparation.
LeetCode: Is specifically tailored for coding interview preparation, with features like company-specific problem sets and mock interviews. This makes it particularly valuable for users with immediate interview goals.
7. Skill Level Suitability
AlgoCademy: Is well-suited for beginners and intermediate learners, providing a gentle introduction to DP concepts and gradually increasing in difficulty.
LeetCode: Caters to a wide range of skill levels but may be more challenging for complete beginners. It’s particularly beneficial for intermediate to advanced users looking to sharpen their DP skills.
Code Example: Fibonacci Sequence Implementation
To illustrate how both platforms might approach teaching a classic dynamic programming problem, let’s consider the Fibonacci sequence. Here’s how each platform might present a solution:
AlgoCademy Approach
AlgoCademy would likely start with an explanation of the problem and the concept of memoization, followed by a step-by-step guide to implementing the solution:
def fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo)
return memo[n]
# Example usage
print(fibonacci(10)) # Output: 55
AlgoCademy would then provide a detailed explanation of how the memoization technique is used to optimize the recursive solution, along with time and space complexity analysis.
LeetCode Approach
LeetCode might present the problem as a coding challenge, expecting users to come up with a solution on their own. The platform would then provide an editorial solution, possibly including multiple approaches:
class Solution:
def fib(self, n: int) -> int:
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Example usage
solution = Solution()
print(solution.fib(10)) # Output: 55
LeetCode would likely include a brief explanation of the bottom-up dynamic programming approach used in this solution, along with its time and space complexity.
Making the Choice: AlgoCademy or LeetCode for Dynamic Programming?
Choosing between AlgoCademy and LeetCode for dynamic programming practice ultimately depends on your learning style, current skill level, and specific goals. Here are some recommendations based on different scenarios:
Choose AlgoCademy if:
- You’re new to dynamic programming and want a structured, comprehensive learning path.
- You prefer interactive tutorials and visual learning aids.
- You value personalized guidance and AI-assisted learning.
- You’re looking to build a strong foundation in DP concepts before tackling more advanced problems.
- You enjoy a collaborative learning environment with a focus on understanding rather than competition.
Choose LeetCode if:
- You already have some familiarity with dynamic programming and want to practice a wide variety of problems.
- You’re specifically preparing for coding interviews at major tech companies.
- You thrive in a competitive environment and enjoy participating in coding contests.
- You want access to a vast community of users and their diverse solutions to DP problems.
- You’re looking for a platform that covers not just DP, but a comprehensive range of algorithmic topics.
Conclusion: The Best of Both Worlds
While both AlgoCademy and LeetCode offer valuable resources for practicing dynamic programming, they excel in different areas. AlgoCademy provides a more structured, beginner-friendly approach with interactive learning and personalized guidance. LeetCode, on the other hand, offers an extensive problem set and a competitive environment that closely mimics real coding interviews.
For many learners, the ideal approach might be to use both platforms in tandem. You could start with AlgoCademy to build a strong foundation in dynamic programming concepts, benefiting from its interactive tutorials and AI-assisted learning. Once you’ve grasped the fundamentals, you could then transition to LeetCode to tackle a wider range of problems and test your skills in a more competitive setting.
Remember, mastering dynamic programming is a journey that requires consistent practice and patience. Whether you choose AlgoCademy, LeetCode, or a combination of both, the key is to engage with the material regularly and apply what you learn to solve diverse problems. With dedication and the right resources, you’ll be well on your way to becoming proficient in one of the most powerful techniques in computer science and acing those technical interviews at top tech companies.
Happy coding, and may your dynamic programming skills flourish on whichever platform you choose!