Achieving Algorithm Mastery: A Comprehensive Approach
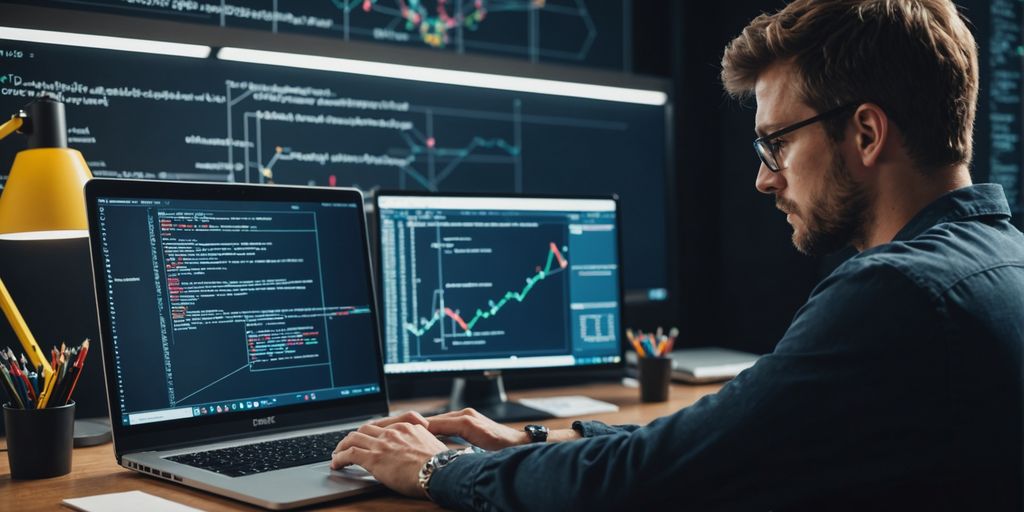
Mastering algorithms is a key skill for anyone interested in computer science or programming. This article will guide you through the basics and help you build a strong foundation. We’ll cover everything from understanding what algorithms are to applying them in real-world situations. Whether you’re a beginner or looking to brush up on your skills, this guide has something for everyone.
Key Takeaways
- Understanding algorithms is essential for solving complex problems effectively.
- Consistent practice and learning are crucial for mastering algorithms and data structures.
- Breaking down problems into smaller steps can make them easier to solve.
- Engaging in coding discussions and mock interviews can improve your problem-solving skills.
- Applying algorithms in real-world scenarios helps in understanding their practical utility.
Understanding the Fundamentals of Algorithm Mastery
Defining Algorithms and Their Importance
Algorithms are step-by-step instructions for solving problems or performing tasks. They are crucial in computer science because they help us create efficient and effective solutions. Understanding algorithms allows us to break down complex problems into simpler, manageable steps.
Common Misconceptions About Algorithms
Many people think algorithms are too hard to learn. This isn’t true. With practice, anyone can understand and use them. It’s important to know that algorithms are not just for experts; they are tools that anyone can master with time and effort.
The Role of Data Structures in Algorithms
Data structures organize and store data so algorithms can work efficiently. They are the backbone of algorithms, making it easier to manage and access data. Learning about data structures helps us understand how to optimize algorithms for better performance.
Building a Strong Foundation in Algorithmic Thinking
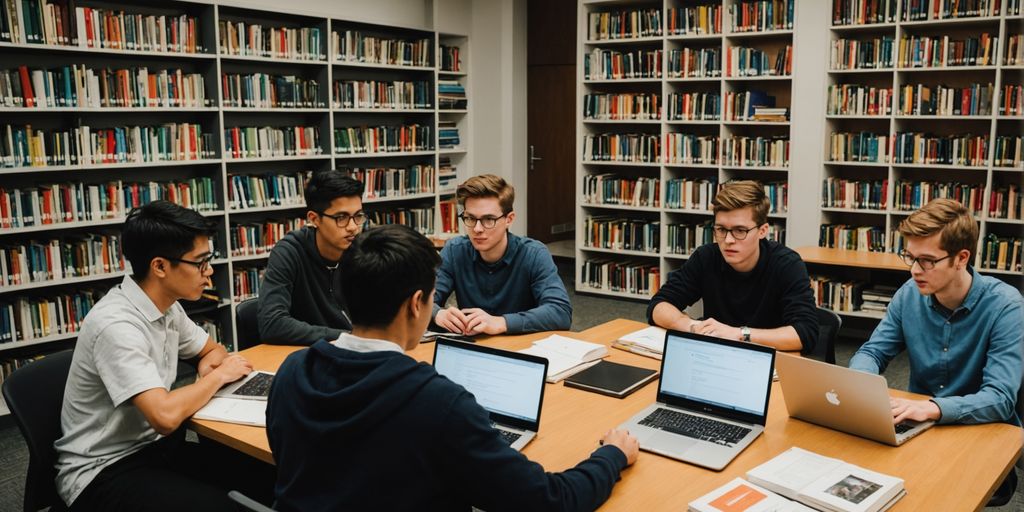
Principles of Algorithmic Thinking
Algorithmic thinking is a mental shift that helps programmers approach problems systematically and logically. Instead of relying on shortcuts, this type of thinking involves clearly defining problems, breaking them down into smaller parts, and finding solutions for each part. By mastering algorithmic thinking, you can create more efficient and scalable software solutions.
Developing Problem-Solving Skills
To develop strong problem-solving skills, it’s important to practice regularly. Here are some steps to follow:
- Understand the problem statement.
- Break the problem into smaller, manageable parts.
- Define solutions for each part.
- Implement the solutions in code.
- Review and improve your solutions.
Practical Exercises to Enhance Algorithmic Thinking
Engaging in practical exercises is crucial for honing your algorithmic thinking. Here are some activities to consider:
- Solve coding problems on online platforms.
- Analyze the time and space complexity of algorithms using Big O notation.
- Collaborate with peers to understand different approaches to problem-solving.
If you’re looking to build a strong foundation in programming, these books are perfect for not just teaching you syntax but also honing your algorithmic thinking.
By consistently practicing these steps and exercises, you can significantly improve your algorithmic thinking and problem-solving skills.
Effective Strategies for Continuous Learning
Setting a Consistent Learning Schedule
To master algorithms, it’s crucial to maintain a consistent learning schedule. This means setting aside regular time for study and practice. Neglecting practice for long periods can make it harder to retain information and solve problems. Even solving one problem a day on platforms like LeetCode can be beneficial. Over a month, solving 30 problems is a significant achievement.
Utilizing Online Platforms for Practice
Online platforms offer a wealth of resources for practicing algorithms. Websites like LeetCode, HackerRank, and CodeSignal provide a variety of problems to solve. These platforms often include discussions and solutions that can help you understand different approaches to a problem.
Engaging in Coding Discussions and Mock Interviews
Participating in coding discussions and mock interviews can greatly enhance your understanding of algorithms. Engaging with others allows you to see different perspectives and solutions. Mock interviews, in particular, can help you prepare for real-world scenarios and improve your problem-solving skills under pressure.
The key to continual learning success is identifying the objective, choosing the right tools, selecting a suitable model architecture, and incrementally improving your skills.
Mastering Core Algorithm Concepts
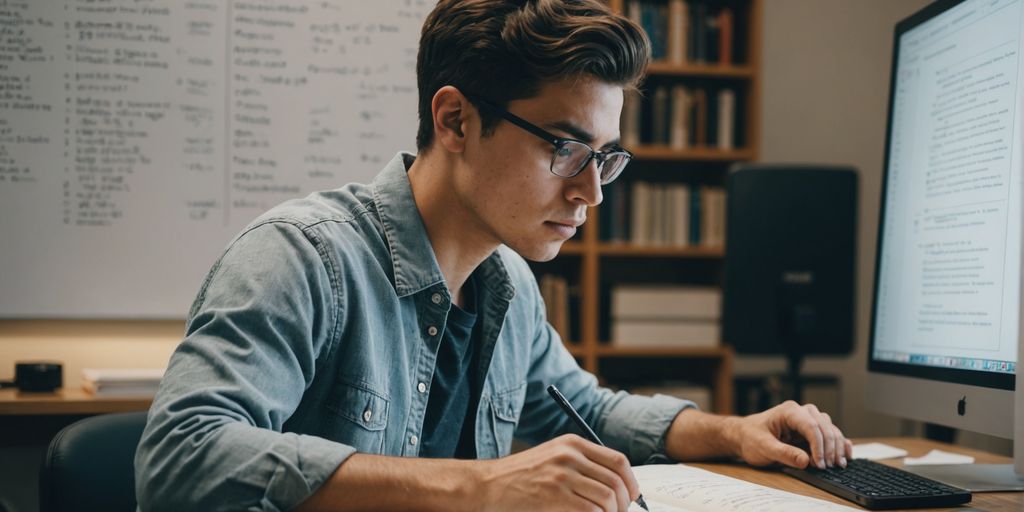
Sorting and Searching Algorithms
Sorting and searching algorithms are fundamental to understanding how to organize and retrieve data efficiently. These algorithms form the backbone of many complex systems. For instance, sorting algorithms like QuickSort and MergeSort help in arranging data, while searching algorithms like Binary Search enable quick data retrieval.
Dynamic Programming and Greedy Algorithms
Dynamic programming and greedy algorithms are powerful techniques for solving optimization problems. Dynamic programming breaks problems into smaller subproblems and solves each one only once, storing the results for future use. Greedy algorithms, on the other hand, make the most optimal choice at each step, aiming for a global optimum.
Graph Algorithms and Their Applications
Graph algorithms are essential for solving problems related to networks, such as social networks, computer networks, and transportation systems. Algorithms like Dijkstra’s and A* are used for finding the shortest path, while algorithms like DFS and BFS are used for traversing or searching tree or graph data structures.
Understanding these core algorithm concepts is crucial for anyone looking to excel in computer science. They not only improve software performance but also enhance problem-solving skills and logical thinking.
Overcoming Challenges in Learning Algorithms
Dealing with Complex Explanations
One of the biggest hurdles in learning algorithms is dealing with complex explanations. Often, explanations are filled with jargon and technical terms that can be confusing. Simplifying these explanations and breaking them down into smaller, more digestible parts can help. It’s also helpful to use real-world examples to illustrate complex concepts.
Understanding Interdependencies of Topics
Algorithms and data structures are often interdependent. This means that understanding one concept might require knowledge of another. To overcome this, follow a well-structured curriculum that builds on each topic progressively. This way, you can avoid the frustration of trying to learn advanced topics without a solid foundation.
Overcoming Fear of Mathematics and Logic
Many people find the mathematical and logical aspects of algorithms intimidating. However, these are essential skills for mastering algorithms. Start with the basics and gradually work your way up. Practice regularly and seek help when needed. Remember, persistence is key.
To overcome the scarcity of data and irrelevance of available data, it’s necessary to use data augmentation techniques and synthetic data generation.
By addressing these challenges head-on, you can make significant progress in mastering algorithms.
Applying Algorithms in Real-World Scenarios
Practical Applications of Algorithms
Algorithms are everywhere in our daily lives. They help us sort, schedule, count, and optimize tasks. For example, search engines use algorithms to rank web pages, and social media platforms use them to show personalized content. Algorithms are also used in e-commerce to suggest products based on what you’ve looked at before. In finance, they help with stock market analysis, credit scoring, and fraud detection. Even in healthcare, algorithms assist in diagnosing diseases and discovering new drugs.
Case Studies of Algorithm Efficiency
Let’s look at some real-life examples of how algorithms make things better. In autonomous vehicles, algorithms process sensor data to help the car navigate and make decisions. In cybersecurity, they detect and prevent cyber threats. Algorithms also help in energy management by optimizing the use of natural resources. These examples show how important and useful algorithms are in making our lives easier and more efficient.
Optimizing Algorithms for Performance
To get the best out of algorithms, it’s important to make them as efficient as possible. This means reducing the number of steps they take to solve a problem. For instance, sorting algorithms like Quick Sort and Merge Sort are designed to handle large amounts of data quickly. By optimizing algorithms, we can save time and resources, making processes faster and more efficient.
Algorithms are not just for computers; they are a part of our everyday lives, helping us make better decisions and solve problems more efficiently.
Enhancing Coding Interview Skills
Communicating Solutions Effectively
During coding interviews, it’s crucial to explain your thoughts clearly. This means talking through your solution as you write the code. Before your interview, explain complex coding topics out loud to a friend. This type of practice makes it easier to recognize how well you understand a topic. Interviewers want to see how you approach problems and how you think.
Writing Efficient and Correct Code
Writing code that is both correct and efficient is key. Here are some tips:
- Understand the problem: Make sure you fully understand the problem before you start coding.
- Plan your solution: Think about the steps you need to take to solve the problem.
- Write clean code: Use meaningful variable names and keep your code organized.
- Test your code: Check for edge cases and make sure your code handles them correctly.
Handling Critical Situations During Interviews
Interviews can be stressful, but staying calm is important. If you get stuck, take a deep breath and think about the problem again. Sometimes, explaining your thought process out loud can help you find a solution. Remember, interviewers are not just looking for the right answer; they want to see how you handle pressure and critical situations.
Practicing these skills can make a big difference in your coding interviews. Stay calm, communicate clearly, and focus on writing efficient and correct code.
Conclusion
Mastering algorithms is not an impossible task. It requires consistent practice, a clear understanding of fundamental concepts, and the ability to think algorithmically. By breaking down complex problems into smaller, manageable parts, and continuously practicing, anyone can improve their skills. Remember, the journey to mastering algorithms is a marathon, not a sprint. Keep practicing, stay curious, and don’t be afraid to ask for help when needed. With dedication and the right approach, achieving algorithm mastery is within your reach.
Frequently Asked Questions
What is an algorithm?
An algorithm is a step-by-step set of instructions to solve a problem or complete a task. Think of it like a recipe that tells you exactly what to do to get a result.
Why are algorithms important?
Algorithms are important because they help us solve problems efficiently. They are used in everything from simple tasks, like sorting numbers, to complex operations, like running search engines.
Are algorithms really hard to learn?
Many people think algorithms are very hard, but with practice, anyone can learn them. Start with simple problems and build up to more complex ones.
How can I get better at solving algorithm problems?
Practice regularly, use online platforms like LeetCode, and participate in coding discussions and mock interviews. Consistency is key.
Do I need to know a lot of math to understand algorithms?
You need some basic math skills, like understanding numbers and logic. Advanced math can help with more complex algorithms, but it’s not always necessary.
What’s the best way to prepare for coding interviews?
Practice solving problems, write efficient and correct code, and learn to communicate your solutions clearly. Mock interviews can also help you get better at handling pressure.