A Deep Dive into Heap Sort Algorithm: Mastering Efficient Sorting
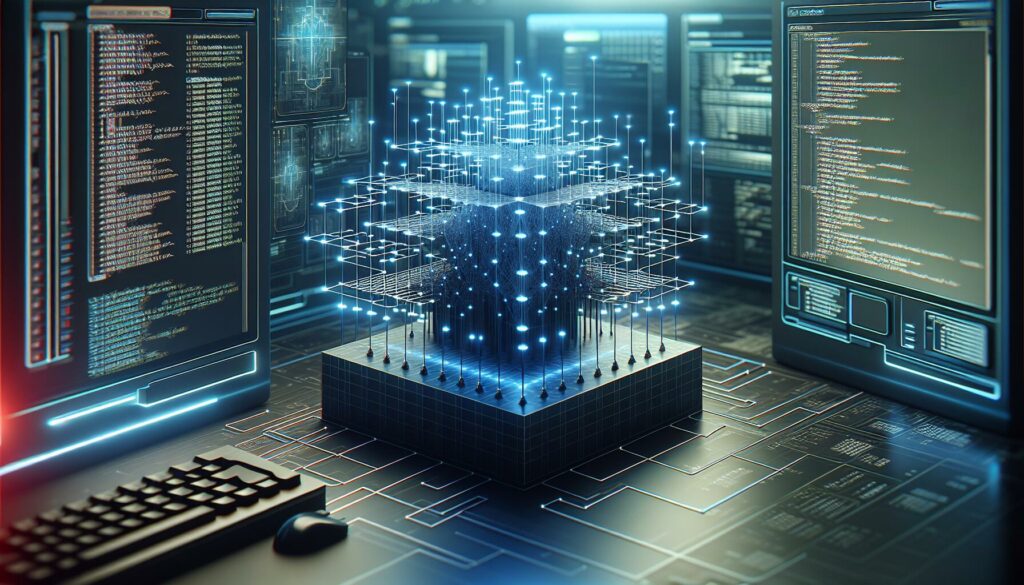
In the world of computer science and programming, sorting algorithms play a crucial role in organizing and managing data efficiently. Among the various sorting algorithms, Heap Sort stands out as a powerful and efficient method for arranging elements in a specific order. In this comprehensive guide, we’ll explore the intricacies of the Heap Sort algorithm, its implementation, time complexity, and practical applications.
Understanding Heap Sort
Heap Sort is a comparison-based sorting algorithm that uses a binary heap data structure to sort elements. It was invented by J.W.J. Williams in 1964 and is an improved version of the selection sort algorithm. Heap Sort works by first building a max-heap (for ascending order) or min-heap (for descending order) from the input array and then repeatedly extracting the root element to obtain the sorted array.
What is a Heap?
Before diving into the Heap Sort algorithm, it’s essential to understand what a heap is. A heap is a specialized tree-based data structure that satisfies the heap property. There are two types of heaps:
- Max Heap: In a max heap, for any given node I, the value of I is greater than or equal to the values of its children.
- Min Heap: In a min heap, the value of a node I is less than or equal to the values of its children.
Heaps are commonly implemented as arrays, where for a node at index i, its left child is at index 2i + 1, and its right child is at index 2i + 2.
The Heap Sort Algorithm
The Heap Sort algorithm consists of two main phases:
- Heap Construction: Build a max heap from the input array.
- Sorting: Repeatedly extract the maximum element from the heap and place it at the end of the array.
Phase 1: Heap Construction
To build a max heap from an unsorted array:
- Start from the last non-leaf node (n/2 – 1, where n is the number of elements) and move towards the root.
- For each node, compare it with its children and swap if necessary to maintain the max heap property.
- Continue this process until the root node is reached.
Phase 2: Sorting
Once we have a max heap:
- Swap the root (maximum element) with the last element of the heap.
- Reduce the size of the heap by 1.
- Heapify the root of the reduced heap.
- Repeat steps 1-3 until the heap size becomes 1.
Implementing Heap Sort in Python
Let’s implement the Heap Sort algorithm in Python:
def heapify(arr, n, i):
largest = i
left = 2 * i + 1
right = 2 * i + 2
if left < n and arr[left] > arr[largest]:
largest = left
if right < n and arr[right] > arr[largest]:
largest = right
if largest != i:
arr[i], arr[largest] = arr[largest], arr[i]
heapify(arr, n, largest)
def heap_sort(arr):
n = len(arr)
# Build max heap
for i in range(n // 2 - 1, -1, -1):
heapify(arr, n, i)
# Extract elements from the heap one by one
for i in range(n - 1, 0, -1):
arr[0], arr[i] = arr[i], arr[0]
heapify(arr, i, 0)
return arr
# Example usage
arr = [12, 11, 13, 5, 6, 7]
sorted_arr = heap_sort(arr)
print("Sorted array:", sorted_arr)
This implementation includes two main functions:
heapify(arr, n, i)
: This function maintains the max heap property for a subtree rooted at index i.heap_sort(arr)
: This function implements the complete Heap Sort algorithm.
Time Complexity Analysis
Let’s analyze the time complexity of the Heap Sort algorithm:
- Heap Construction: O(n)
- Heapify: O(log n) for each call
- Sorting: O(n log n)
The overall time complexity of Heap Sort is O(n log n) in all cases (best, average, and worst). This makes it more efficient than algorithms like Bubble Sort or Insertion Sort, which have a worst-case time complexity of O(n²).
Space Complexity
Heap Sort has a space complexity of O(1) as it sorts the array in-place, requiring only a constant amount of additional memory.
Advantages and Disadvantages of Heap Sort
Advantages:
- Efficiency: Heap Sort has a time complexity of O(n log n) for all cases, making it efficient for large datasets.
- In-place sorting: It doesn’t require additional memory proportional to the input size.
- Consistency: Unlike Quicksort, Heap Sort’s performance is consistent across different input distributions.
Disadvantages:
- Not stable: Heap Sort is not a stable sorting algorithm, meaning it may change the relative order of equal elements.
- Cache unfriendly: Due to its poor locality of reference, Heap Sort may not perform as well as algorithms like Quicksort on modern architectures with caches.
- Complexity: The algorithm is more complex to implement compared to simpler sorting algorithms like Bubble Sort or Insertion Sort.
Practical Applications of Heap Sort
Heap Sort finds applications in various areas of computer science and software development:
- Priority Queues: Heap Sort’s underlying data structure (heap) is commonly used to implement priority queues.
- Operating Systems: Used in process scheduling algorithms.
- Graph Algorithms: Heap Sort is used in implementations of Dijkstra’s shortest path and Prim’s minimum spanning tree algorithms.
- K-way merging: Efficient for merging k sorted arrays.
- Order Statistics: Finding the kth smallest/largest element in an array.
Optimizing Heap Sort
While Heap Sort is already an efficient algorithm, there are ways to optimize its performance:
1. Bottom-up Heap Construction
Instead of starting from the middle of the array and moving towards the root, we can build the heap from the bottom up. This approach is more cache-friendly and can lead to better performance.
2. Heap Sort with Decrease Key
For certain applications where we need to update the priority of elements, implementing a decrease-key operation can be beneficial.
3. Parallel Heap Sort
On multi-core systems, we can parallelize parts of the Heap Sort algorithm to improve performance.
Comparison with Other Sorting Algorithms
Let’s compare Heap Sort with other popular sorting algorithms:
Algorithm | Time Complexity (Average) | Time Complexity (Worst) | Space Complexity | Stability |
---|---|---|---|---|
Heap Sort | O(n log n) | O(n log n) | O(1) | No |
Quick Sort | O(n log n) | O(n²) | O(log n) | No |
Merge Sort | O(n log n) | O(n log n) | O(n) | Yes |
Insertion Sort | O(n²) | O(n²) | O(1) | Yes |
Heap Sort Variations
Several variations of the Heap Sort algorithm have been developed to address specific use cases or improve performance:
1. Smoothsort
Smoothsort is a variation of Heap Sort that takes advantage of pre-existing order in the input data. It has a best-case time complexity of O(n) when the input is already sorted or nearly sorted.
2. Introspective Sort (Introsort)
Introsort begins with Quicksort and switches to Heap Sort when the recursion depth exceeds a certain level. This hybrid approach combines the average-case efficiency of Quicksort with the worst-case efficiency of Heap Sort.
3. External Heap Sort
This variation is used when the data to be sorted is too large to fit into memory. It uses external memory (like disk) to perform the sorting operation.
Implementing Heap Sort in Other Programming Languages
While we’ve seen a Python implementation, let’s look at how Heap Sort can be implemented in other popular programming languages:
Java Implementation
public class HeapSort {
public void sort(int arr[]) {
int n = arr.length;
// Build heap
for (int i = n / 2 - 1; i >= 0; i--)
heapify(arr, n, i);
// One by one extract an element from heap
for (int i = n - 1; i > 0; i--) {
// Move current root to end
int temp = arr[0];
arr[0] = arr[i];
arr[i] = temp;
// call max heapify on the reduced heap
heapify(arr, i, 0);
}
}
void heapify(int arr[], int n, int i) {
int largest = i;
int l = 2 * i + 1;
int r = 2 * i + 2;
if (l < n && arr[l] > arr[largest])
largest = l;
if (r < n && arr[r] > arr[largest])
largest = r;
if (largest != i) {
int swap = arr[i];
arr[i] = arr[largest];
arr[largest] = swap;
heapify(arr, n, largest);
}
}
}
C++ Implementation
#include <iostream>
using namespace std;
void heapify(int arr[], int n, int i) {
int largest = i;
int l = 2 * i + 1;
int r = 2 * i + 2;
if (l < n && arr[l] > arr[largest])
largest = l;
if (r < n && arr[r] > arr[largest])
largest = r;
if (largest != i) {
swap(arr[i], arr[largest]);
heapify(arr, n, largest);
}
}
void heapSort(int arr[], int n) {
for (int i = n / 2 - 1; i >= 0; i--)
heapify(arr, n, i);
for (int i = n - 1; i > 0; i--) {
swap(arr[0], arr[i]);
heapify(arr, i, 0);
}
}
Common Pitfalls and How to Avoid Them
When implementing or using Heap Sort, be aware of these common pitfalls:
- Incorrect heap property: Ensure that you’re maintaining the correct heap property (max heap for ascending order, min heap for descending order).
- Off-by-one errors: Be careful with array indices, especially when calculating child nodes.
- Inefficient heap construction: Use the O(n) bottom-up method for heap construction instead of the O(n log n) top-down approach.
- Unnecessary swaps: Avoid unnecessary swaps by using a temporary variable to store the root value during the sorting phase.
- Ignoring small arrays: For very small arrays, simpler algorithms like Insertion Sort might be more efficient.
Heap Sort in Interview Questions
Heap Sort is a popular topic in technical interviews, especially for roles at major tech companies. Here are some common interview questions related to Heap Sort:
- Implement Heap Sort from scratch.
- Explain the time and space complexity of Heap Sort.
- Compare Heap Sort with other sorting algorithms like Quicksort and Merge Sort.
- How would you modify Heap Sort to sort in descending order?
- Implement a priority queue using a heap.
- Find the k-th largest element in an unsorted array using Heap Sort concepts.
- How would you optimize Heap Sort for nearly sorted data?
Conclusion
Heap Sort is a powerful and efficient sorting algorithm that combines the benefits of good performance with in-place sorting. Its consistent O(n log n) time complexity makes it a reliable choice for sorting large datasets. While it may not be as commonly used as Quicksort in practice due to its cache-unfriendly nature, understanding Heap Sort is crucial for any programmer or computer scientist.
By mastering Heap Sort, you’ll not only add a valuable tool to your algorithmic toolkit but also gain deeper insights into heap data structures, which are fundamental to many advanced algorithms and data structures. As you continue your journey in algorithm design and analysis, remember that each sorting algorithm has its strengths and ideal use cases. The key is to understand these trade-offs and choose the right algorithm for your specific needs.
Keep practicing implementing and analyzing Heap Sort, and don’t hesitate to explore its variations and optimizations. With dedication and consistent effort, you’ll be well-prepared to tackle complex sorting problems and excel in technical interviews at top tech companies.