A Comprehensive Guide to Graph Theory and Algorithms
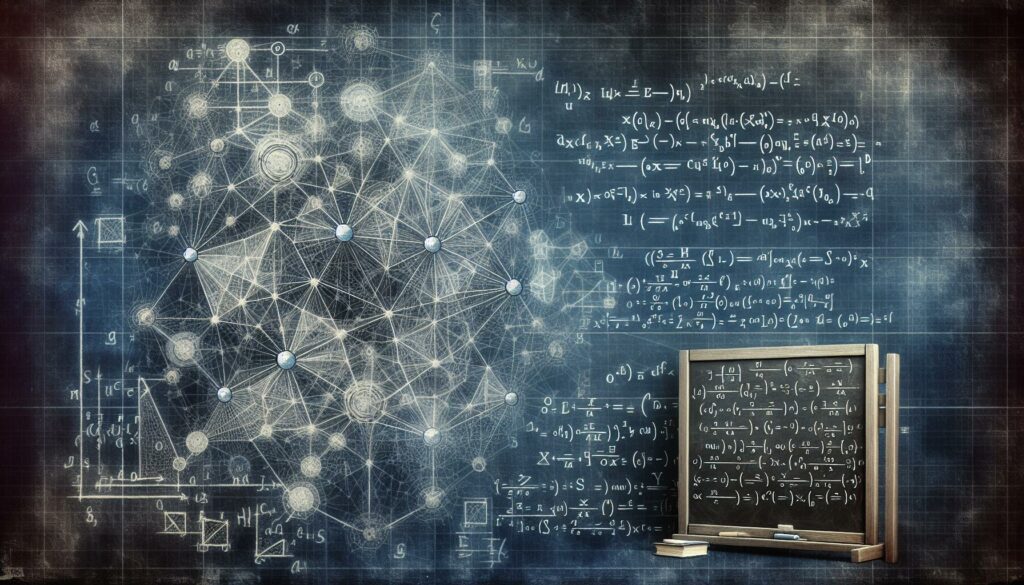
In the world of computer science and mathematics, graph theory stands as a fundamental concept with wide-ranging applications. From social networks to transportation systems, graphs provide a powerful framework for modeling and analyzing complex relationships. This guide will dive deep into graph theory and explore essential algorithms that every programmer should know. Whether you’re preparing for technical interviews at top tech companies or simply looking to enhance your problem-solving skills, understanding graphs is crucial.
Table of Contents
- Introduction to Graph Theory
- Graph Representation
- Graph Traversal Algorithms
- Shortest Path Algorithms
- Minimum Spanning Tree Algorithms
- Topological Sorting
- Strongly Connected Components
- Network Flow Algorithms
- Real-world Applications
- Conclusion
1. Introduction to Graph Theory
Graph theory is the study of graphs, which are mathematical structures used to model pairwise relations between objects. A graph consists of vertices (also called nodes) and edges that connect these vertices. Graphs can be used to represent a wide variety of real-world scenarios, from social networks to computer networks, and from transportation systems to dependency relationships in software projects.
Types of Graphs
- Undirected Graph: Edges have no direction and represent symmetric relationships.
- Directed Graph (Digraph): Edges have a direction, representing asymmetric relationships.
- Weighted Graph: Edges have associated weights or costs.
- Cyclic Graph: Contains at least one cycle (a path that starts and ends at the same vertex).
- Acyclic Graph: Contains no cycles.
- Connected Graph: There is a path between every pair of vertices.
- Disconnected Graph: There are vertices that cannot be reached from others.
Understanding these different types of graphs is crucial for selecting the appropriate algorithms and data structures for specific problems.
2. Graph Representation
Before diving into algorithms, it’s essential to understand how graphs are represented in code. The two most common representations are:
Adjacency Matrix
An adjacency matrix is a 2D array where matrix[i][j] represents an edge from vertex i to vertex j. For an undirected graph, the matrix is symmetric.
def create_adjacency_matrix(n, edges):
matrix = [[0] * n for _ in range(n)]
for u, v in edges:
matrix[u][v] = 1
matrix[v][u] = 1 # For undirected graph
return matrix
# Example usage
edges = [(0, 1), (0, 2), (1, 2), (2, 3)]
adj_matrix = create_adjacency_matrix(4, edges)
print(adj_matrix)
# Output: [[0, 1, 1, 0], [1, 0, 1, 0], [1, 1, 0, 1], [0, 0, 1, 0]]
Adjacency List
An adjacency list uses a list or dictionary to store the neighbors of each vertex. This representation is more space-efficient for sparse graphs.
from collections import defaultdict
def create_adjacency_list(edges):
adj_list = defaultdict(list)
for u, v in edges:
adj_list[u].append(v)
adj_list[v].append(u) # For undirected graph
return adj_list
# Example usage
edges = [(0, 1), (0, 2), (1, 2), (2, 3)]
adj_list = create_adjacency_list(edges)
print(adj_list)
# Output: defaultdict(<class 'list'>, {0: [1, 2], 1: [0, 2], 2: [0, 1, 3], 3: [2]})
Each representation has its advantages. Adjacency matrices allow for constant-time edge lookup but require O(V^2) space, where V is the number of vertices. Adjacency lists are more space-efficient for sparse graphs and allow for faster iteration over a vertex’s neighbors.
3. Graph Traversal Algorithms
Graph traversal is the process of visiting all the vertices in a graph. The two fundamental traversal algorithms are Depth-First Search (DFS) and Breadth-First Search (BFS).
Depth-First Search (DFS)
DFS explores as far as possible along each branch before backtracking. It can be implemented recursively or using a stack.
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start, end=' ')
for neighbor in graph[start]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
# Example usage
graph = {0: [1, 2], 1: [2], 2: [3], 3: [1, 2]}
dfs(graph, 0)
# Output: 0 1 2 3
Breadth-First Search (BFS)
BFS explores all the neighbors at the present depth before moving to vertices at the next depth level. It uses a queue for implementation.
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
visited.add(start)
while queue:
vertex = queue.popleft()
print(vertex, end=' ')
for neighbor in graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
# Example usage
graph = {0: [1, 2], 1: [2], 2: [3], 3: [1, 2]}
bfs(graph, 0)
# Output: 0 1 2 3
Both DFS and BFS have a time complexity of O(V + E), where V is the number of vertices and E is the number of edges.
4. Shortest Path Algorithms
Finding the shortest path between vertices is a common problem in graph theory. Here are two important algorithms for solving this problem:
Dijkstra’s Algorithm
Dijkstra’s algorithm finds the shortest path from a single source vertex to all other vertices in a weighted graph with non-negative edge weights.
import heapq
def dijkstra(graph, start):
distances = {vertex: float('infinity') for vertex in graph}
distances[start] = 0
pq = [(0, start)]
while pq:
current_distance, current_vertex = heapq.heappop(pq)
if current_distance > distances[current_vertex]:
continue
for neighbor, weight in graph[current_vertex].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(pq, (distance, neighbor))
return distances
# Example usage
graph = {
'A': {'B': 4, 'C': 2},
'B': {'D': 3, 'E': 1},
'C': {'B': 1, 'D': 5},
'D': {'E': 2},
'E': {}
}
print(dijkstra(graph, 'A'))
# Output: {'A': 0, 'B': 3, 'C': 2, 'D': 6, 'E': 4}
Floyd-Warshall Algorithm
The Floyd-Warshall algorithm finds the shortest paths between all pairs of vertices in a weighted graph, including those with negative edge weights (but no negative cycles).
def floyd_warshall(graph):
distances = {v: {u: float('infinity') for u in graph} for v in graph}
for v in graph:
distances[v][v] = 0
for neighbor, weight in graph[v].items():
distances[v][neighbor] = weight
for k in graph:
for i in graph:
for j in graph:
distances[i][j] = min(distances[i][j], distances[i][k] + distances[k][j])
return distances
# Example usage
graph = {
'A': {'B': 3, 'C': 6},
'B': {'C': 2, 'D': 1},
'C': {'D': 4},
'D': {}
}
print(floyd_warshall(graph))
# Output: {'A': {'A': 0, 'B': 3, 'C': 5, 'D': 4}, 'B': {'A': inf, 'B': 0, 'C': 2, 'D': 1}, 'C': {'A': inf, 'B': inf, 'C': 0, 'D': 4}, 'D': {'A': inf, 'B': inf, 'C': inf, 'D': 0}}
5. Minimum Spanning Tree Algorithms
A minimum spanning tree (MST) is a subset of edges in a weighted, undirected graph that connects all vertices with the minimum total edge weight. Two popular algorithms for finding MSTs are:
Kruskal’s Algorithm
Kruskal’s algorithm builds the MST by adding edges in order of increasing weight, skipping edges that would create a cycle.
class UnionFind:
def __init__(self, vertices):
self.parent = {v: v for v in vertices}
self.rank = {v: 0 for v in vertices}
def find(self, item):
if self.parent[item] != item:
self.parent[item] = self.find(self.parent[item])
return self.parent[item]
def union(self, x, y):
xroot = self.find(x)
yroot = self.find(y)
if self.rank[xroot] < self.rank[yroot]:
self.parent[xroot] = yroot
elif self.rank[xroot] > self.rank[yroot]:
self.parent[yroot] = xroot
else:
self.parent[yroot] = xroot
self.rank[xroot] += 1
def kruskal(graph):
edges = [(w, u, v) for u in graph for v, w in graph[u].items()]
edges.sort()
vertices = list(graph.keys())
uf = UnionFind(vertices)
mst = []
for w, u, v in edges:
if uf.find(u) != uf.find(v):
uf.union(u, v)
mst.append((u, v, w))
return mst
# Example usage
graph = {
'A': {'B': 4, 'C': 2},
'B': {'A': 4, 'C': 1, 'D': 5},
'C': {'A': 2, 'B': 1, 'D': 8, 'E': 10},
'D': {'B': 5, 'C': 8, 'E': 2, 'F': 6},
'E': {'C': 10, 'D': 2, 'F': 3},
'F': {'D': 6, 'E': 3}
}
print(kruskal(graph))
# Output: [('B', 'C', 1), ('A', 'C', 2), ('D', 'E', 2), ('E', 'F', 3), ('A', 'B', 4)]
Prim’s Algorithm
Prim’s algorithm builds the MST by starting from an arbitrary vertex and always adding the lowest-weight edge that connects a vertex in the tree to a vertex outside the tree.
import heapq
def prim(graph, start):
mst = []
visited = set([start])
edges = [(w, start, v) for v, w in graph[start].items()]
heapq.heapify(edges)
while edges:
w, u, v = heapq.heappop(edges)
if v not in visited:
visited.add(v)
mst.append((u, v, w))
for next_v, next_w in graph[v].items():
if next_v not in visited:
heapq.heappush(edges, (next_w, v, next_v))
return mst
# Example usage
graph = {
'A': {'B': 4, 'C': 2},
'B': {'A': 4, 'C': 1, 'D': 5},
'C': {'A': 2, 'B': 1, 'D': 8, 'E': 10},
'D': {'B': 5, 'C': 8, 'E': 2, 'F': 6},
'E': {'C': 10, 'D': 2, 'F': 3},
'F': {'D': 6, 'E': 3}
}
print(prim(graph, 'A'))
# Output: [('A', 'C', 2), ('C', 'B', 1), ('B', 'D', 5), ('D', 'E', 2), ('E', 'F', 3)]
6. Topological Sorting
Topological sorting is used to linearly order the vertices of a directed acyclic graph (DAG) such that for every directed edge (u, v), vertex u comes before v in the ordering. This is particularly useful in scheduling tasks with dependencies.
from collections import defaultdict
def topological_sort(graph):
def dfs(v):
visited.add(v)
for neighbor in graph[v]:
if neighbor not in visited:
dfs(neighbor)
stack.append(v)
visited = set()
stack = []
for vertex in graph:
if vertex not in visited:
dfs(vertex)
return stack[::-1]
# Example usage
graph = {
'A': ['C'],
'B': ['C', 'D'],
'C': ['E'],
'D': ['F'],
'E': ['H', 'F'],
'F': ['G'],
'G': [],
'H': []
}
print(topological_sort(graph))
# Output: ['B', 'A', 'C', 'E', 'D', 'F', 'H', 'G']
7. Strongly Connected Components
A strongly connected component (SCC) in a directed graph is a subgraph where every vertex is reachable from every other vertex. Kosaraju’s algorithm is an efficient method for finding SCCs.
from collections import defaultdict
def kosaraju(graph):
def dfs(v, visited, stack):
visited.add(v)
for neighbor in graph[v]:
if neighbor not in visited:
dfs(neighbor, visited, stack)
stack.append(v)
def reverse_graph(graph):
reversed_graph = defaultdict(list)
for v in graph:
for neighbor in graph[v]:
reversed_graph[neighbor].append(v)
return reversed_graph
def dfs_scc(v, visited, scc):
visited.add(v)
scc.append(v)
for neighbor in reversed_graph[v]:
if neighbor not in visited:
dfs_scc(neighbor, visited, scc)
stack = []
visited = set()
for vertex in graph:
if vertex not in visited:
dfs(vertex, visited, stack)
reversed_graph = reverse_graph(graph)
visited.clear()
sccs = []
while stack:
vertex = stack.pop()
if vertex not in visited:
scc = []
dfs_scc(vertex, visited, scc)
sccs.append(scc)
return sccs
# Example usage
graph = {
0: [1, 3],
1: [2],
2: [0],
3: [4],
4: [5],
5: [3]
}
print(kosaraju(graph))
# Output: [[5, 4, 3], [2, 1, 0]]
8. Network Flow Algorithms
Network flow algorithms are used to solve problems related to the flow of resources through a network. The Ford-Fulkerson algorithm is a classic method for finding the maximum flow in a flow network.
def ford_fulkerson(graph, source, sink):
def bfs(graph, source, sink, parent):
visited = [False] * len(graph)
queue = [source]
visited[source] = True
while queue:
u = queue.pop(0)
for v, capacity in enumerate(graph[u]):
if not visited[v] and capacity > 0:
queue.append(v)
visited[v] = True
parent[v] = u
if v == sink:
return True
return False
parent = [-1] * len(graph)
max_flow = 0
while bfs(graph, source, sink, parent):
path_flow = float('inf')
s = sink
while s != source:
path_flow = min(path_flow, graph[parent[s]][s])
s = parent[s]
max_flow += path_flow
v = sink
while v != source:
u = parent[v]
graph[u][v] -= path_flow
graph[v][u] += path_flow
v = parent[v]
return max_flow
# Example usage
graph = [
[0, 16, 13, 0, 0, 0],
[0, 0, 10, 12, 0, 0],
[0, 4, 0, 0, 14, 0],
[0, 0, 9, 0, 0, 20],
[0, 0, 0, 7, 0, 4],
[0, 0, 0, 0, 0, 0]
]
source = 0
sink = 5
print(f"The maximum possible flow is {ford_fulkerson(graph, source, sink)}")
# Output: The maximum possible flow is 23
9. Real-world Applications
Graph theory and its algorithms have numerous real-world applications across various domains:
- Social Networks: Analyzing connections between users, detecting communities, and recommending friends.
- Transportation: Optimizing routes for navigation systems, traffic flow analysis, and logistics planning.
- Computer Networks: Designing efficient network topologies, routing protocols, and analyzing network reliability.
- Biology: Modeling protein interactions, analyzing gene regulatory networks, and studying disease spread.
- Artificial Intelligence: Implementing search algorithms, knowledge representation, and decision-making systems.
- Recommendation Systems: Suggesting products, content, or connections based on user preferences and behavior.
- Compiler Design: Optimizing code generation and data flow analysis.
- Operations Research: Solving scheduling problems, resource allocation, and supply chain optimization.
Understanding graph theory and its algorithms is crucial for tackling complex problems in these domains and developing efficient solutions.
10. Conclusion
Graph theory and its algorithms form a fundamental part of computer science and have wide-ranging applications in solving real-world problems. From social network analysis to optimizing transportation systems, graphs provide a powerful framework for modeling and analyzing complex relationships.
In this comprehensive guide, we’ve covered the basics of graph theory, various graph representations, and essential algorithms such as graph traversal, shortest path finding, minimum spanning trees, topological sorting, strongly connected components, and network flow. Understanding these concepts and algorithms is crucial for any programmer looking to excel in technical interviews or tackle complex computational problems.
As you continue your journey in programming and computer science, remember that graph theory is not just a theoretical concept but a practical tool that can be applied to solve a wide range of problems. Practice implementing these algorithms, and try to identify graph structures in the problems you encounter. With time and experience, you’ll develop a strong intuition for when and how to apply graph theory to solve complex challenges efficiently.
Keep exploring, keep coding, and don’t hesitate to dive deeper into the fascinating world of graph theory and algorithms!