A Comprehensive Guide to Designing Robust Algorithms
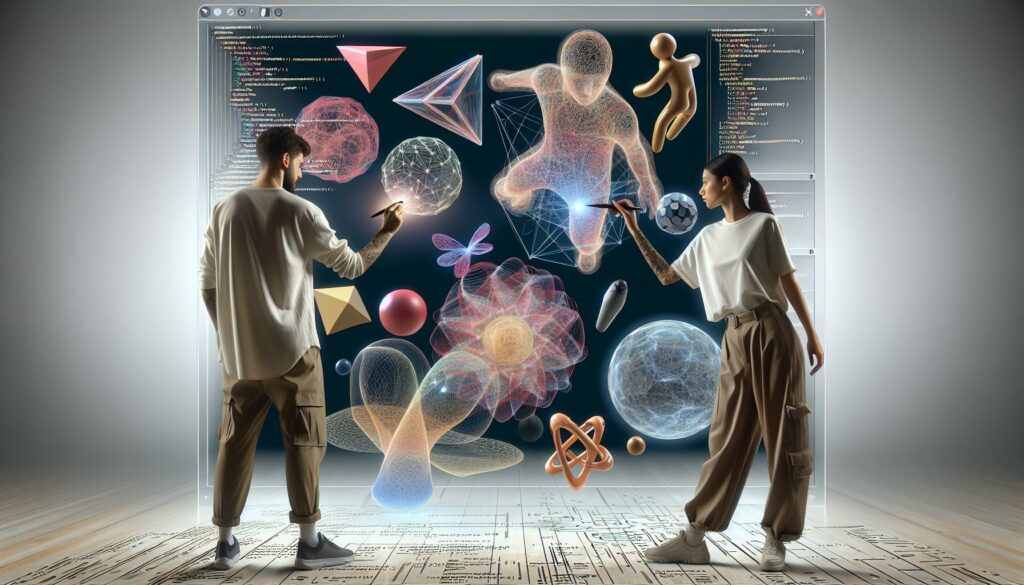
In the world of computer science and software engineering, the ability to design robust algorithms is a crucial skill that separates good programmers from great ones. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at top tech companies, understanding how to create efficient and reliable algorithms is essential. This comprehensive guide will walk you through the process of designing robust algorithms, providing you with the knowledge and techniques needed to excel in your programming career.
1. Understanding the Importance of Robust Algorithms
Before diving into the specifics of algorithm design, it’s crucial to understand why robust algorithms are so important in the field of computer science and software development.
1.1 Defining Robust Algorithms
A robust algorithm is one that can handle a wide range of inputs, including edge cases and unexpected scenarios, while still producing correct and efficient results. Robust algorithms are characterized by their reliability, scalability, and ability to gracefully handle errors or exceptions.
1.2 The Impact of Robust Algorithms
Robust algorithms have a significant impact on various aspects of software development and system performance:
- Improved reliability and stability of software systems
- Enhanced user experience due to fewer errors and crashes
- Better scalability and performance under varying workloads
- Reduced maintenance costs and easier debugging
- Increased confidence in the software’s ability to handle real-world scenarios
2. Key Principles of Robust Algorithm Design
To design robust algorithms, it’s essential to follow certain principles that guide the development process. These principles help ensure that your algorithms can handle a wide range of inputs and scenarios effectively.
2.1 Correctness
The most fundamental principle of robust algorithm design is correctness. An algorithm must produce the correct output for all valid inputs. This involves:
- Thoroughly understanding the problem requirements
- Considering all possible input scenarios, including edge cases
- Implementing proper error handling and validation
- Rigorously testing the algorithm with various inputs
2.2 Efficiency
A robust algorithm should not only be correct but also efficient in terms of time and space complexity. This means:
- Analyzing and optimizing the algorithm’s time complexity (Big O notation)
- Minimizing memory usage and considering space complexity
- Balancing trade-offs between time and space efficiency
- Choosing appropriate data structures and algorithms for the specific problem
2.3 Scalability
Robust algorithms should be able to handle growing amounts of data or increased workloads without significant performance degradation. To ensure scalability:
- Design algorithms with large-scale datasets in mind
- Consider parallelization and distributed computing techniques
- Implement efficient data structures that can handle growth
- Optimize for both average and worst-case scenarios
2.4 Maintainability
A robust algorithm should be easy to understand, modify, and maintain over time. This involves:
- Writing clean, well-documented code
- Using meaningful variable and function names
- Breaking down complex algorithms into smaller, modular components
- Following established coding standards and best practices
2.5 Flexibility and Adaptability
Robust algorithms should be designed to accommodate future changes and extensions. This principle includes:
- Designing algorithms with modularity and extensibility in mind
- Using appropriate design patterns and abstraction layers
- Anticipating potential future requirements or modifications
- Implementing configurable parameters or options when appropriate
3. The Algorithm Design Process
Designing robust algorithms involves a systematic approach that helps ensure all aspects of the problem are considered and addressed. Here’s a step-by-step process to guide you through algorithm design:
3.1 Problem Analysis
The first step in designing a robust algorithm is to thoroughly analyze the problem at hand. This involves:
- Clearly defining the problem statement and requirements
- Identifying input and output specifications
- Recognizing any constraints or limitations
- Breaking down the problem into smaller subproblems if necessary
3.2 Conceptual Design
Once you have a clear understanding of the problem, start developing a high-level conceptual design:
- Brainstorm potential approaches and solutions
- Consider different algorithms and data structures that might be applicable
- Sketch out flowcharts or pseudocode to visualize the algorithm’s logic
- Identify potential bottlenecks or challenging aspects of the problem
3.3 Algorithm Development
With a conceptual design in place, proceed to develop the algorithm in more detail:
- Choose appropriate data structures to represent the problem’s elements
- Implement the core logic of the algorithm
- Consider edge cases and handle them appropriately
- Optimize the algorithm for efficiency and scalability
3.4 Implementation and Testing
Translate your algorithm design into actual code and thoroughly test it:
- Implement the algorithm in your chosen programming language
- Write unit tests to verify correctness for various inputs
- Test edge cases and boundary conditions
- Perform performance testing to evaluate efficiency and scalability
3.5 Analysis and Refinement
After implementation and initial testing, analyze the algorithm’s performance and refine as needed:
- Analyze time and space complexity
- Identify areas for optimization or improvement
- Refactor code for better readability and maintainability
- Consider alternative approaches or optimizations
4. Common Techniques for Designing Robust Algorithms
Several techniques and strategies can help you design more robust algorithms. Here are some common approaches:
4.1 Divide and Conquer
The divide and conquer technique involves breaking down a complex problem into smaller, more manageable subproblems. This approach often leads to efficient and scalable algorithms. Key aspects of divide and conquer include:
- Recursively dividing the problem into smaller instances
- Solving the base cases directly
- Combining the solutions of subproblems to solve the original problem
Example: The merge sort algorithm is a classic example of divide and conquer.
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
4.2 Dynamic Programming
Dynamic programming is a powerful technique for solving optimization problems by breaking them down into simpler subproblems. It’s particularly useful for problems with overlapping subproblems and optimal substructure. Key aspects of dynamic programming include:
- Identifying overlapping subproblems
- Storing solutions to subproblems in a table (memoization)
- Building up the solution to the original problem from the solutions of subproblems
Example: The fibonacci sequence calculation using dynamic programming:
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
4.3 Greedy Algorithms
Greedy algorithms make locally optimal choices at each step with the hope of finding a global optimum. While not always guaranteed to find the best solution, greedy algorithms can be efficient and effective for certain problems. Key aspects of greedy algorithms include:
- Making the locally optimal choice at each step
- Never reconsidering previous choices
- Aiming for a global optimum through a series of local optima
Example: The coin change problem using a greedy approach:
def coin_change(amount, coins):
coins.sort(reverse=True)
result = []
for coin in coins:
while amount >= coin:
result.append(coin)
amount -= coin
return result if amount == 0 else None
4.4 Backtracking
Backtracking is a general algorithm for finding all (or some) solutions to computational problems, particularly constraint satisfaction problems. It incrementally builds candidates for the solutions and abandons a candidate as soon as it determines that the candidate cannot lead to a valid solution. Key aspects of backtracking include:
- Exploring all possible solutions
- Pruning branches that cannot lead to a valid solution
- Efficiently searching through a potentially large solution space
Example: Solving the N-Queens problem using backtracking:
def solve_n_queens(n):
def is_safe(board, row, col):
# Check if a queen can be placed on board[row][col]
# Check this row on left side
for i in range(col):
if board[row][i] == 1:
return False
# Check upper diagonal on left side
for i, j in zip(range(row, -1, -1), range(col, -1, -1)):
if board[i][j] == 1:
return False
# Check lower diagonal on left side
for i, j in zip(range(row, n, 1), range(col, -1, -1)):
if board[i][j] == 1:
return False
return True
def solve(board, col):
if col >= n:
return True
for i in range(n):
if is_safe(board, i, col):
board[i][col] = 1
if solve(board, col + 1):
return True
board[i][col] = 0
return False
board = [[0 for _ in range(n)] for _ in range(n)]
if solve(board, 0):
return board
else:
return None
5. Advanced Concepts in Algorithm Design
As you progress in your algorithm design skills, it’s important to explore more advanced concepts that can help you create even more robust and efficient algorithms.
5.1 Approximation Algorithms
For some NP-hard problems, finding an exact solution in polynomial time may not be feasible. Approximation algorithms aim to find a solution that is guaranteed to be within a certain factor of the optimal solution. Key aspects of approximation algorithms include:
- Trading off optimality for efficiency
- Providing guarantees on the quality of the approximation
- Analyzing the approximation ratio
5.2 Randomized Algorithms
Randomized algorithms incorporate a degree of randomness into their logic. These algorithms can often achieve better average-case performance than deterministic algorithms. Key aspects of randomized algorithms include:
- Using random numbers or choices in the algorithm’s execution
- Analyzing expected running time or probability of correctness
- Balancing randomness with deterministic components
5.3 Online Algorithms
Online algorithms are designed to process input piece-by-piece in a serial fashion, without having the entire input available from the start. These algorithms are crucial for scenarios where data arrives in real-time or when the input is too large to fit in memory. Key aspects of online algorithms include:
- Making decisions with partial information
- Adapting to changing inputs over time
- Analyzing competitive ratios against offline algorithms
5.4 Parallel and Distributed Algorithms
With the increasing prevalence of multi-core processors and distributed systems, designing algorithms that can take advantage of parallelism is becoming more important. Key aspects of parallel and distributed algorithms include:
- Dividing work among multiple processors or machines
- Managing communication and synchronization between parallel components
- Balancing workload and minimizing overhead
- Designing algorithms that scale with additional resources
6. Best Practices for Implementing Robust Algorithms
Designing a robust algorithm is only part of the process. Implementing it correctly and efficiently is equally important. Here are some best practices to follow when implementing your algorithms:
6.1 Code Organization and Modularity
Organize your code into logical modules and functions to improve readability and maintainability:
- Break down complex algorithms into smaller, manageable functions
- Use meaningful names for variables, functions, and classes
- Group related functionality into classes or modules
- Follow the Single Responsibility Principle (SRP) for functions and classes
6.2 Error Handling and Input Validation
Implement robust error handling and input validation to ensure your algorithm can handle unexpected inputs gracefully:
- Validate input parameters at the beginning of functions
- Use appropriate exception handling mechanisms
- Provide meaningful error messages for different types of errors
- Consider using assertions for internal consistency checks
6.3 Testing and Verification
Thoroughly test your algorithm implementation to ensure correctness and robustness:
- Write unit tests for individual functions and components
- Test edge cases and boundary conditions
- Use property-based testing for more comprehensive coverage
- Implement integration tests for complex algorithms
- Perform stress testing with large inputs to verify scalability
6.4 Documentation and Comments
Provide clear documentation and comments to explain the algorithm’s purpose, implementation details, and usage:
- Write a high-level description of the algorithm and its purpose
- Document function parameters, return values, and exceptions
- Explain complex logic or non-obvious implementation details
- Include examples of usage and expected outputs
- Keep comments up-to-date as the code evolves
6.5 Performance Optimization
Optimize your algorithm implementation for better performance without sacrificing readability or maintainability:
- Profile your code to identify performance bottlenecks
- Use appropriate data structures for efficient operations
- Consider space-time trade-offs when optimizing
- Implement caching or memoization for expensive computations
- Optimize critical loops and frequently executed code paths
7. Common Pitfalls in Algorithm Design and How to Avoid Them
Even experienced programmers can fall into common traps when designing algorithms. Being aware of these pitfalls can help you avoid them and create more robust algorithms:
7.1 Overlooking Edge Cases
Failing to consider edge cases can lead to algorithms that work for most inputs but fail in specific scenarios. To avoid this:
- Identify and list potential edge cases during the problem analysis phase
- Include edge case handling in your algorithm design
- Write specific test cases for edge scenarios
- Consider inputs like empty collections, negative numbers, or maximum values
7.2 Premature Optimization
Trying to optimize an algorithm before it’s fully designed and working correctly can lead to unnecessary complexity. Instead:
- Focus on correctness first, then optimize if necessary
- Use profiling tools to identify actual performance bottlenecks
- Consider the trade-offs between readability and performance
- Optimize only when you have concrete evidence of performance issues
7.3 Ignoring Scalability
Designing algorithms that work well for small inputs but fail to scale can lead to problems in real-world applications. To address this:
- Consider the expected scale of inputs during the design phase
- Analyze the time and space complexity of your algorithm
- Test with large datasets to verify scalability
- Consider distributed or parallel approaches for very large-scale problems
7.4 Overengineering
Creating overly complex solutions for simple problems can lead to difficult-to-maintain code. To avoid overengineering:
- Start with the simplest solution that solves the problem correctly
- Add complexity only when necessary to meet performance or functionality requirements
- Regularly refactor and simplify your code
- Seek feedback from peers to ensure your design is appropriate for the problem
7.5 Neglecting Error Handling
Failing to properly handle errors and exceptions can lead to unstable and unreliable algorithms. To improve error handling:
- Identify potential error conditions during the design phase
- Implement appropriate error handling and recovery mechanisms
- Provide clear and informative error messages
- Test error scenarios to ensure proper handling
8. Resources for Further Learning
To continue improving your algorithm design skills, consider exploring these resources:
8.1 Books
- “Introduction to Algorithms” by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein
- “The Algorithm Design Manual” by Steven S. Skiena
- “Algorithms” by Robert Sedgewick and Kevin Wayne
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
8.2 Online Courses
- Coursera: “Algorithms Specialization” by Stanford University
- edX: “Algorithms and Data Structures” by MIT
- Udacity: “Intro to Algorithms”
- AlgoCademy: Interactive coding tutorials and problem-solving exercises
8.3 Coding Practice Platforms
- LeetCode: A platform with a wide variety of coding problems and competitions
- HackerRank: Offers coding challenges and contests in various domains
- CodeForces: Competitive programming platform with regular contests
- Project Euler: A series of challenging mathematical/computer programming problems
8.4 Research Papers and Journals
- Journal of the ACM (JACM)
- SIAM Journal on Computing
- Algorithmica
- Annual ACM Symposium on Theory of Computing (STOC) proceedings
Conclusion
Designing robust algorithms is a critical skill for any programmer or computer scientist. By following the principles and techniques outlined in this guide, you can create algorithms that are not only correct and efficient but also scalable, maintainable, and adaptable to changing requirements. Remember that algorithm design is both an art and a science, requiring creativity, analytical thinking, and continuous learning.
As you practice and gain experience, you’ll develop an intuition for choosing the right approach for different problems and be better equipped to tackle complex algorithmic challenges. Whether you’re preparing for technical interviews at top tech companies or working on large-scale software systems, the ability to design robust algorithms will be invaluable throughout your career.
Keep exploring, practicing, and refining your skills, and you’ll be well on your way to becoming a master algorithm designer. Happy coding!