Unlocking the Power of React Components: A Beginner’s Guide to Building Dynamic User Interfaces
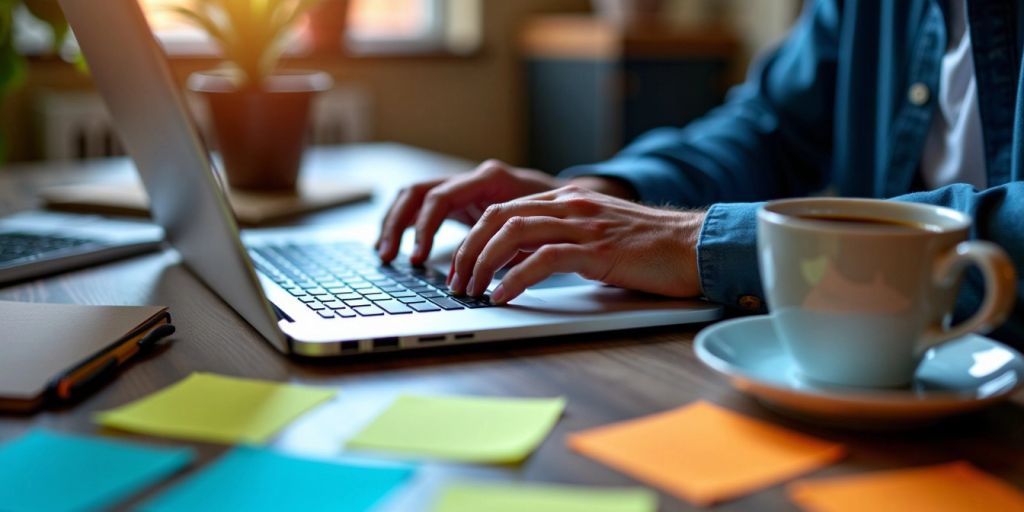
React is a powerful tool for creating user interfaces. This guide will help beginners understand the basics of React components and how to build dynamic web applications. You’ll learn about setting up your development environment, creating components, and managing data flow, all in a simple and easy-to-understand way.
Key Takeaways
- React components are like building blocks for web apps, making it easier to create and manage user interfaces.
- You can set up a React project quickly using Create React App, which saves time and effort.
- JSX is a special syntax that lets you mix HTML with JavaScript, making your code more readable.
- State and props are essential for managing data in your app; state is internal while props are external inputs.
- Understanding lifecycle methods helps you control how components behave during their existence.
Understanding React Components
What Are React Components?
React components are the building blocks of any React application. They allow developers to create reusable pieces of UI that can be combined to form complex interfaces. Each component can manage its own state and behavior, making it easier to build dynamic applications.
Types of React Components
There are two main types of components in React:
- Functional Components: These are simple JavaScript functions that return JSX. They are easier to write and understand.
- Class Components: These are more complex and can hold their own state. They are defined using ES6 classes.
Type | Description |
---|---|
Functional | Simpler, stateless components |
Class | More complex, can manage state and lifecycle |
Benefits of Using React Components
Using React components offers several advantages:
- Reusability: Components can be reused across different parts of an application, saving time and effort.
- Maintainability: Smaller, focused components are easier to manage and debug.
- Separation of Concerns: Each component handles its own logic, making the code cleaner and more organized.
React components help in creating dynamic user interfaces by allowing developers to break down complex UIs into manageable parts. This modular approach enhances both development speed and application performance.
Setting Up Your React Development Environment
To start building with React, you need to set up your development environment. In this article, we will show you a step-by-step guide to installing and configuring a working react development environment. Here’s how to get started:
Installing Node.js and npm
- Download Node.js: Go to the official Node.js website and download the installer for your operating system.
- Install Node.js: Run the installer and follow the instructions. This will also install npm (Node Package Manager), which is essential for managing packages.
- Verify Installation: Open your terminal and type:
node -v npm -v
This will show you the installed versions of Node.js and npm.
Using Create React App
- Open Terminal: Launch your terminal or command prompt.
- Create a New React App: Run the following command:
npx create-react-app my-react-app
This command sets up a new React project in a folder named
my-react-app
. - Navigate to Your Project: Change to your project directory:
cd my-react-app
- Start the Development Server: Run:
npm start
This will launch your React app in the browser.
Exploring the Project Structure
Once your app is running, take a moment to explore the project structure:
src/
: Contains your source files.public/
: Holds static files likeindex.html
.package.json
: Lists your project dependencies.
Setting up your environment is the first step to creating amazing applications with React. Embrace the learning process and enjoy coding!
Creating Your First React Component
Functional Components
Functional components are the simplest way to create a React component. They are just JavaScript functions that return JSX. Here’s how to create one:
- Define a function that returns JSX.
- Use the function as a component in your app.
- Pass props to customize the component.
For example:
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
Class Components
Class components are more complex and allow for additional features like state management. To create a class component:
- Extend React.Component.
- Implement a render method that returns JSX.
- Use state to manage data.
Example:
class Greeting extends React.Component {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
Rendering Components
To display your components, you need to render them in your main application file. Here’s how:
- Import your component into the main file.
- Use ReactDOM.render() to display it.
Example:
import React from 'react';
import ReactDOM from 'react-dom';
import Greeting from './Greeting';
ReactDOM.render(<Greeting name="World" />, document.getElementById('root'));
Creating components is essential for building dynamic user interfaces. They help organize your code and make it reusable.
In summary, whether you choose functional or class components, understanding how to create and render them is crucial for your React journey. Getting started with React is all about mastering these foundational concepts!
JSX: The Syntax of React
What is JSX?
JSX, or JavaScript XML, is a special syntax used in React. It looks a lot like HTML, which makes it easier to create user interfaces. With JSX, you can write your UI structure directly in your JavaScript code. This means you can mix HTML-like tags with JavaScript logic, making your code more readable and organized.
Embedding Expressions in JSX
You can also include JavaScript expressions inside JSX. To do this, you wrap your expressions in curly braces {}
. Here are some examples:
- Displaying a variable:
<h1>{title}</h1>
- Performing calculations:
<p>{2 + 2}</p>
- Calling functions:
<p>{getGreeting()}</p>
JSX Best Practices
To make your JSX code clean and efficient, consider these best practices:
- Use parentheses for multi-line JSX to improve readability.
- Keep components small and focused on a single task.
- Avoid using index as a key in lists to prevent issues with component state.
Remember, JSX is not HTML. It has its own rules and quirks, so take time to learn them well!
Managing State and Props
Understanding State
State is like a component’s personal memory. It holds data that can change over time. When the state updates, React automatically re-renders the component to reflect those changes. State is crucial for creating interactive applications. Here are some key points about state:
- It is local to the component.
- It can be updated using the
setState
method. - Changes to state trigger a re-render of the component.
Using Props to Pass Data
Props, short for properties, are used to transfer data from a parent component to a child. They are read-only and help in making components reusable. Here’s what you need to know about props:
- Props are passed down from parent to child components.
- They cannot be modified by the child component.
- Props are essential for data flow in React applications.
State and Props Best Practices
To effectively manage state and props, consider the following best practices:
- Keep state as simple as possible.
- Use props to pass data and callbacks to child components.
- Avoid unnecessary state updates to improve performance.
Managing state and props effectively is key to building dynamic and responsive applications.
Concept | Description |
---|---|
State | Internal data of a component that can change. |
Props | External data passed from parent to child components. |
In summary, understanding the difference between state and props is vital for React development. State is used to manage data inside a component, while props are used to transfer data between components. Mastering these concepts will help you build more dynamic user interfaces.
Lifecycle Methods in React
React components go through a series of stages from creation to removal. Understanding these stages is essential for managing how components behave. Lifecycle methods allow developers to run code at specific points in a component’s life.
Component Lifecycle Overview
The lifecycle of a React component can be divided into three main phases:
- Mounting: This is when the component is being created and inserted into the DOM.
- Updating: This occurs when the component is being re-rendered due to changes in state or props.
- Unmounting: This is when the component is being removed from the DOM.
Common Lifecycle Methods
Here are some important lifecycle methods:
Method | Phase | Description |
---|---|---|
componentDidMount |
Mounting | Invoked immediately after a component is added. |
componentDidUpdate |
Updating | Invoked immediately after updating occurs. |
componentWillUnmount |
Unmounting | Invoked just before a component is removed. |
Using Lifecycle Methods Effectively
To make the most of lifecycle methods, consider these tips:
- Use
componentDidMount
for fetching data. - Use
componentDidUpdate
to respond to prop changes. - Clean up resources in
componentWillUnmount
to prevent memory leaks.
Understanding lifecycle methods is crucial for controlling component behavior and optimizing performance.
By mastering these methods, you can enhance your React applications significantly.
Handling Events in React
Adding Event Handlers
In React, you can easily add event handlers to your components. This allows you to respond to user actions like clicks, key presses, and more. Here are some common events you might handle:
- onClick: Triggered when an element is clicked.
- onChange: Used for input fields when the value changes.
- onSubmit: Fired when a form is submitted.
Event Binding
When using event handlers, you need to bind them to the component’s context. This ensures that the this
keyword refers to the correct component instance. You can bind events in a few ways:
- Using arrow functions: This automatically binds the context.
- Binding in the constructor: You can bind methods in the constructor of your class component.
- Using public class fields: This is a modern approach that simplifies binding.
Synthetic Events
React uses a system called Synthetic Events to handle events consistently across different browsers. This means you can write your event handling code without worrying about browser differences. Here’s a quick overview of how it works:
- Synthetic events wrap the native events.
- They have the same interface as native events, making them easy to use.
- They are automatically pooled for performance, meaning they are reused for efficiency.
React facilitates event handling with built-in methods, allowing developers to create listeners for dynamic interfaces and responses.
By mastering event handling in React, you can create interactive and engaging user experiences. Remember to practice these concepts to become more comfortable with them!
Styling React Components
Inline Styling
Inline styling in React allows you to apply styles directly to elements using the style
attribute. This method is straightforward but can become cumbersome for larger applications. Here are some key points about inline styling:
- Dynamic Styles: You can use JavaScript expressions to set styles dynamically.
- CamelCase Properties: CSS properties are written in camelCase, like
backgroundColor
instead ofbackground-color
. - No Pseudo-classes: Inline styles do not support pseudo-classes like
:hover
.
CSS Modules
CSS Modules provide a way to scope your CSS to specific components, preventing style conflicts. This method is beneficial for larger projects. Here’s how it works:
- Create a CSS file with the
.module.css
extension. - Import the CSS file into your component.
- Use the styles as an object, e.g.,
styles.container
.
Styled Components
Styled Components is a popular library that allows you to write CSS in your JavaScript files. This method promotes component-level styling. Here are some advantages:
- Automatic Vendor Prefixing: It handles browser compatibility for you.
- Dynamic Styling: You can easily change styles based on props.
- Theming Support: It allows for easy theme management across your application.
Using the right styling method can greatly enhance your React application’s maintainability and performance. Choose wisely based on your project needs!
Advanced React Concepts
Context API
The Context API is a powerful feature in React that allows you to share values between components without having to pass props down manually at every level. This is especially useful for global data like themes or user information. Here are some key points about the Context API:
- Simplifies Prop Drilling: Avoids the need to pass props through many layers of components.
- Improves Code Organization: Keeps related data together, making your code cleaner.
- Enhances Performance: Reduces unnecessary re-renders by allowing components to subscribe to only the data they need.
Higher-Order Components
Higher-Order Components (HOCs) are functions that take a component and return a new component. They are used to share common functionality between components. Here are some benefits of using HOCs:
- Code Reusability: Write logic once and apply it to multiple components.
- Separation of Concerns: Keep your components focused on their primary tasks.
- Enhanced Functionality: Add features like logging, data fetching, or access control easily.
React Hooks
React Hooks are functions that let you use state and other React features without writing a class. They simplify component logic and make it easier to share stateful logic between components. Some popular hooks include:
- useState: For managing state in functional components.
- useEffect: For handling side effects like data fetching or subscriptions.
- useContext: For accessing context values directly in functional components.
The Context API, HOCs, and Hooks are essential tools for building scalable and maintainable React applications. They help you manage state and share functionality effectively, making your code cleaner and more efficient.
Resources for Learning
If you’re eager to dive deeper into these advanced concepts, here are some great resources:
- React Official Documentation
- Epic React by Kent C. Dodds
- FreeCodeCamp — Advanced React Challenges
By mastering these advanced concepts, you can unlock the full potential of React and build dynamic user interfaces with ease.
Testing React Components
Unit Testing with Jest
Unit testing is a crucial part of ensuring your React components work as expected. Jest is a popular testing framework that makes it easy to write and run tests. Here are some key points about unit testing in React:
- Test individual components to ensure they render correctly.
- Mock functions to simulate user interactions and test component behavior.
- Check for expected outputs based on given inputs.
Integration Testing
Integration testing focuses on how different components work together. This type of testing helps catch issues that might not be apparent when testing components in isolation. Here are some steps to perform integration testing:
- Render multiple components together to see how they interact.
- Simulate user actions to ensure the components respond correctly.
- Verify the overall behavior of the application as a whole.
End-to-End Testing
End-to-end testing checks the entire application flow from start to finish. This ensures that all parts of your app work together seamlessly. Here are some important aspects:
- Use tools like Cypress or Selenium for automated testing.
- Test real user scenarios to mimic how users will interact with your app.
- Identify any broken links or features that don’t work as intended.
Testing is not just about finding bugs; it’s about ensuring your application behaves as expected and provides a great user experience.
In summary, testing React components is essential for building reliable applications. By using Jest for unit tests, performing integration tests, and conducting end-to-end tests, you can ensure your components function correctly and provide a smooth user experience. Remember, testing is a vital part of the development process that helps maintain code quality and reliability.
Optimizing React Performance
Using the React Profiler
The React Profiler is a powerful tool that helps you understand how your components render. It allows you to track rendering times and identify performance bottlenecks. To use it:
- Open your React app in the browser.
- Click on the Profiler tab in the React Developer Tools.
- Start recording and interact with your app to see how long each component takes to render.
Memoization Techniques
Memoization can significantly improve performance by caching the results of expensive function calls. Here are some techniques:
- React.memo: Wrap functional components to prevent unnecessary re-renders.
- useMemo: Use this hook to memoize values that are expensive to compute.
- useCallback: Memoize callback functions to avoid re-creating them on every render.
Code Splitting
Code splitting allows you to load parts of your application only when needed, reducing the initial load time. You can achieve this using:
- React.lazy: Dynamically import components as needed.
- React.Suspense: Show a fallback UI while loading components.
By optimizing your React app’s performance, you can create a smoother user experience and reduce loading times. Implementing these strategies can lead to significant improvements.
Summary Table of Optimization Techniques
Technique | Description | Benefits |
---|---|---|
React Profiler | Tool for tracking rendering times | Identify performance issues |
Memoization | Caching results of expensive calls | Reduce unnecessary re-renders |
Code Splitting | Load parts of the app only when needed | Faster initial load times |
To make your React apps run faster, focus on optimizing your components. This means using tools like React.memo to prevent unnecessary re-renders and keeping your state management efficient. Want to learn more about coding and improve your skills? Visit our website and start coding for free today!
Conclusion
Starting your journey with React opens up a new way to create modern web apps. By learning the basics of React components, how to manage data, using JSX, and understanding lifecycle methods, you build a strong base for making interactive and flexible user interfaces. Keep exploring, practice often, and connect with the lively React community to improve your skills and stay updated with new trends. Happy coding!
Frequently Asked Questions
What is React?
React is a popular JavaScript library used for building user interfaces. It helps developers create interactive web apps easily.
Why should I learn React?
Learning React can help you build modern web applications. It’s widely used and has a large community, making it easier to find help and resources.
What are components in React?
Components are the building blocks of a React app. They are reusable pieces of code that represent parts of the user interface.
What is JSX?
JSX stands for JavaScript XML. It’s a syntax extension that lets you write HTML-like code within JavaScript, making it easier to create React components.
How do I manage state in React?
State in React is used to manage data that can change over time. You can use hooks like useState to create and update state in functional components.
What are props in React?
Props, short for properties, are used to pass data from one component to another. They help components communicate with each other.
What are lifecycle methods?
Lifecycle methods are special functions in class components that help you run code at specific times in a component’s life, like when it’s created or updated.
How can I style my React components?
You can style React components using various methods such as inline styles, CSS modules, or libraries like styled-components.