Mastering the Python ls Command: A Comprehensive Guide to Listing Files in Directories
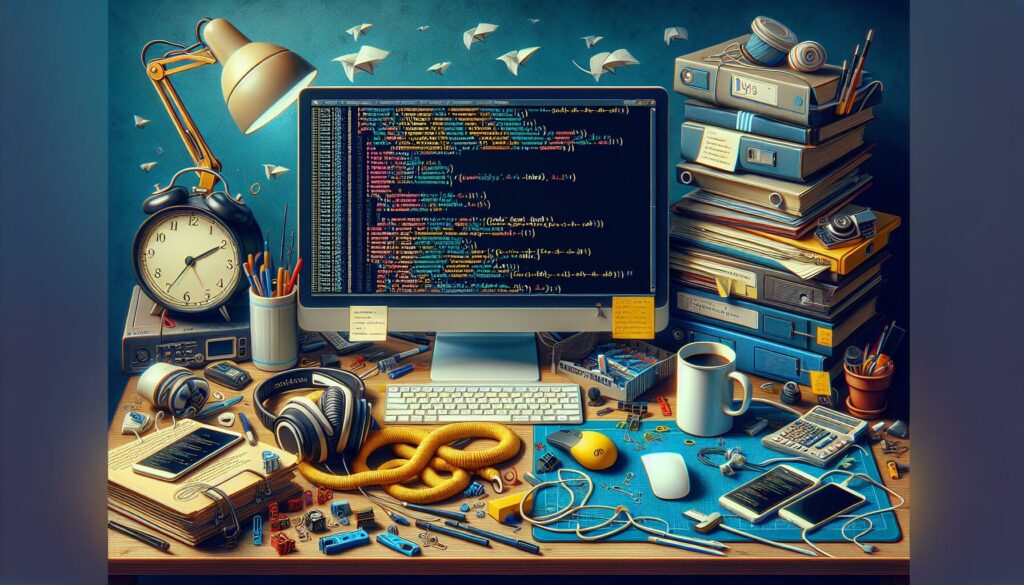
This article provides a simple and clear guide to using the Python ‘ls’ command, which helps in listing files within directories. Whether you’re a beginner or looking to enhance your skills, this guide covers everything from basic usage to advanced techniques. You’ll learn about different methods in Python for listing files and how to handle common issues you might face along the way.
Key Takeaways
- The ‘ls’ command is essential for listing files in directories in Python.
- Using the os.listdir() function is a straightforward way to get a list of files.
- The os.scandir() function provides more detailed information about directory entries.
- The pathlib library offers a modern approach to file handling in Python.
- Combining ‘ls’ with other commands can enhance your file management capabilities.
Getting Started with the Python ls Command
Basic Usage of the ls Command
The ls
command is a fundamental tool in Python for listing files in a directory. It helps you see what’s inside a folder quickly. To use it, simply type ls
in your terminal, and it will show you the contents of the current directory. Here’s a simple example:
ls
This command will display all files and folders in the current directory.
Common Flags and Options
When using the ls
command, you can add flags to change how it works. Here are some common options:
-l
: Shows detailed information about each file, like size and permissions.-a
: Lists all files, including hidden ones.-h
: Makes file sizes easier to read (e.g., KB, MB).
Flag | Description |
---|---|
-l |
Detailed view of files |
-a |
Show hidden files |
-h |
Human-readable sizes |
Examples of Simple ls Commands
Here are a few examples of how to use the ls
command:
- List all files:
ls -a
- Detailed view:
ls -l
- Human-readable sizes:
ls -lh
Understanding the ls command is essential for navigating your files effectively. It’s a simple yet powerful tool that can help you manage your directories with ease.
By mastering the basics of the ls
command, you’ll be well on your way to executing shell commands with Python effectively!
Understanding the os.listdir() Function
Introduction to os.listdir()
The os.listdir() function is a handy tool in Python for listing files in a directory. It’s part of the built-in os module, which helps you interact with the operating system. This function returns a list of all files and folders in a specified directory.
Basic Examples and Syntax
Here’s a simple example of how to use os.listdir():
import os
files = os.listdir('/path/to/directory')
print(files)
When you run this code, it will show you a list of all the files in the given directory. For instance, if the directory contains three files, the output will look like this:
['file1.txt', 'file2.txt', 'file3.txt']
Advantages and Limitations
One of the main advantages of using os.listdir() is its simplicity. You can quickly get a list of files with just a few lines of code. However, it has some limitations:
- It does not sort the files in any specific order.
- It includes all entries, such as subdirectories and special files.
Remember, os.listdir() does not filter out hidden files or directories, so you might see more than you expect.
In summary, os.listdir() is a great starting point for listing files in a directory, but be aware of its limitations when working with larger or more complex directories.
Using os.scandir() for Directory Listing
Introduction to os.scandir()
The os.scandir()
function is a powerful tool in Python for listing files and directories. It was introduced in Python 3.5 and allows you to get more than just names; it provides details about each file and directory. This makes it easier to manage files.
How to Use os.scandir()
To use os.scandir()
, you can follow these simple steps:
- Import the
os
module. - Use
os.scandir()
with the path of the directory you want to explore. - Loop through the entries to access their properties.
Here’s a quick example:
import os
with os.scandir('my_directory/') as entries:
for entry in entries:
print(entry.name)
This code will print the names of all files and folders in my_directory/
.
Benefits Over os.listdir()
Using os.scandir()
has several advantages:
- More Information: It provides file attributes like size and modification date.
- Efficiency: It returns an iterator, which is more memory-efficient than a list.
- Cleaner Code: The syntax is often simpler and easier to read.
Feature | os.listdir() | os.scandir() |
---|---|---|
Returns | List of names | Iterator of entries |
File Attributes | No | Yes |
Memory Efficiency | Less efficient | More efficient |
Using os.scandir() is a great way to loop through folders and files in a directory. It helps you get detailed information without much hassle.
In summary, os.scandir()
is a modern and efficient way to list directory contents in Python, making it a preferred choice for many developers.
Exploring pathlib for File Listing
Introduction to pathlib
The pathlib
module in Python makes it easy to work with file paths. Navigating the filesystem is pretty straightforward with pathlib. You can easily list files and directories, rename them, and resolve paths without much hassle.
Using pathlib.Path() for Listing Files
To list files in a directory using pathlib
, you can use the Path
class. Here’s a simple way to do it:
from pathlib import Path
# Specify the directory
basepath = Path('my_directory/')
# List all files
for item in basepath.iterdir():
if item.is_file():
print(item.name)
This code will print the names of all files in the specified directory. You can also filter for directories by using item.is_dir()
instead.
Comparing pathlib with os.listdir() and os.scandir()
When comparing pathlib
with other methods like os.listdir()
and os.scandir()
, pathlib
offers a cleaner and more intuitive approach. Here’s a quick comparison:
Function | Description |
---|---|
os.listdir() |
Returns a list of all files and folders in a directory |
os.scandir() |
Returns an iterator of all objects in a directory, including file attributes |
pathlib.Path.iterdir() |
Returns an iterator of all objects in a directory, including file attributes |
Using pathlib
not only simplifies your code but also reduces the number of imports needed for file operations.
In summary, pathlib combines many of the best features of the os and glob modules into one single module, making it a joy to use.
Advanced File Listing Techniques in Python
Filtering Files by Extension
Filtering files by their extension is a common task when listing files in a directory. Here’s how you can do it:
- Use the
os
module to list files. - Check the file extension using string methods.
- Print or store the filtered files.
For example:
import os
def filter_files_by_extension(directory, extension):
return [file for file in os.listdir(directory) if file.endswith(extension)]
# Example usage
print(filter_files_by_extension('/path/to/directory', '.txt'))
Sorting Files by Name, Size, or Date
Sorting files can help you organize your data better. You can sort files by:
- Name: Use the
sorted()
function. - Size: Use
os.path.getsize()
to get file sizes. - Date: Use
os.path.getmtime()
to sort by modification date.
Here’s a simple example of sorting files by size:
import os
def sort_files_by_size(directory):
files = os.listdir(directory)
return sorted(files, key=lambda x: os.path.getsize(os.path.join(directory, x)))
# Example usage
print(sort_files_by_size('/path/to/directory'))
Recursive File Listing
To list files in a directory and all its subdirectories, you can use the os.walk()
function. This function allows you to traverse through directories easily:
import os
def recursive_file_listing(directory):
for root, dirs, files in os.walk(directory):
for file in files:
print(os.path.join(root, file))
# Example usage
recursive_file_listing('/path/to/directory')
In summary, mastering these advanced techniques can greatly enhance your file handling capabilities in Python. These methods not only improve efficiency but also make your code cleaner and more readable. Remember, this guide provides an in-depth exploration of file handling in python, addressing advanced techniques and best practices.
Handling Common Challenges and Errors
When using the Python ls
command, you might face some common challenges. Here, we will discuss how to deal with these issues effectively.
Permission Errors
One frequent problem is the Permission Denied error. This happens when you try to access a directory without the right permissions. For example:
ls /root
# Output:
# ls: cannot open directory '/root': Permission denied
To fix this, you can use the sudo
command to run ls
with higher privileges:
sudo ls /root
# Output:
# file1.txt file2.txt
Dealing with Hidden Files
Hidden files start with a dot (.) and are not shown by default. To include them in your listing, you can modify your code:
import os
def list_files(directory):
files = os.listdir(directory)
for file in files:
if not file.startswith('.'): # Exclude hidden files
print(file)
Handling Large Directories
When listing files in a directory with many items, the output can be overwhelming. To manage this, you can use the | less
command to scroll through the results:
ls /usr/bin | less
This allows you to view the output one page at a time.
Summary of Common Issues
Issue | Solution |
---|---|
Permission Denied | Use sudo to gain access. |
Hidden Files | Modify code to include hidden files. |
Large Directories | Use ` |
Remember: Handling errors effectively is key to mastering the ls command. Always check your permissions and be aware of hidden files!
Combining ls with Other Commands
The ls command is a powerful tool that can be combined with other commands to enhance its functionality. Here are some common ways to use it:
Using ls with grep
You can use ls
with the grep
command to filter files based on specific patterns. For example, to find all Python files in a directory, you can run:
ls | grep '.py$'
This command lists all files and then filters the output to show only those that end with .py
.
Using ls with sort
The sort
command can be used with ls
to organize files in a specific order. For instance, to sort files by size, you can use:
ls -l | sort -k5 -n
This command lists files in long format and sorts them by their size in ascending order.
Scripting with ls for Automation
You can also use ls
in shell scripts to automate tasks. Here’s a simple example:
#!/bin/bash
for file in $(ls *.txt); do
cp $file backup/$file
done
This script copies all .txt
files to a backup directory.
Combining the ls command with other commands can greatly enhance your file management capabilities.
By mastering these combinations, you can streamline your workflow and make file handling much easier!
Best Practices and Optimization Tips
Using Flags Effectively
To make the most of the ls
command, consider these tips:
- Use flags to customize your output. For example,
-l
gives detailed information, while-a
shows hidden files. - Combine flags for more control, like
ls -la
to see all files in detail. - Remember that using too many flags can clutter your output.
Optimizing Performance
Performance is key when listing files, especially in large directories. Here are some strategies:
- Use
os.scandir()
instead ofos.listdir()
for faster performance. - Limit the number of files displayed by using filters.
- Consider using
| less
to manage large outputs effectively.
Avoiding Common Pitfalls
To prevent issues while using the ls
command, keep these points in mind:
- Always check your permissions to avoid
Permission denied
errors. - Be cautious with large directories; use pagination to avoid overwhelming output.
- Regularly clean up your directories to keep them organized and manageable.
By following these best practices, you can enhance your experience with the ls command and improve your overall Python optimization skills.
Alternative Commands to ls
While the ls
command is widely used, there are other commands that can help you list files and directories effectively. Here are some alternatives:
Using the find Command
The find
command is a powerful tool for searching files and directories based on specific criteria. It can be particularly useful when you need to locate files in a complex directory structure. Here’s how you can use it:
- Basic Usage: To list all files in the current directory, you can use:
find . -type f
- Output Example:
./file1.txt ./file2.txt ./dir1/file3.txt ./dir2/file4.txt
Using the tree Command
The tree
command displays the directory structure in a tree-like format, making it easier to visualize the relationships between files and folders. Here’s a simple way to use it:
- Basic Usage: Just type:
tree
- Output Example:
. ├── file1.txt ├── file2.txt ├── dir1 │ └── file3.txt └── dir2 └── file4.txt
Using the ll Command
The ll
command is a shortcut for ls -l
, which provides detailed information about files and directories. It’s a quick way to get more context about your files:
- Basic Usage: Simply type:
ll
- Output Example:
total 8 drwxr-xr-x 2 user group 4096 Jan 1 00:00 dir1 drwxr-xr-x 2 user group 4096 Jan 1 00:00 dir2 -rw-r--r-- 1 user group 0 Jan 1 00:00 file1.txt -rw-r--r-- 1 user group 0 Jan 1 00:00 file2.txt
In summary, while ls
is a great command, exploring alternatives like find
, tree
, and ll
can enhance your file management experience. Each command has its own strengths, so choose the one that fits your needs best!
Practical Applications of the Python ls Command
The ls
command in Python is not just for listing files; it has many practical uses that can help you manage your files and directories effectively. Understanding these applications can enhance your coding skills.
File Management
- Organizing Files: Use
ls
to quickly see what files are in a directory, helping you keep things tidy. - Deleting Unwanted Files: Combine
ls
with other commands to identify and remove files you no longer need. - Moving Files: Easily list files before moving them to ensure you’re transferring the right ones.
Data Processing
- Analyzing File Types: Use
ls
to filter files by type, which is useful for data analysis tasks. - Batch Processing: List files and process them in bulk, such as converting image formats or renaming files.
- Data Validation: Check if all expected files are present in a directory before running a data pipeline.
Automation Scripts
- Automating Backups: Create scripts that use
ls
to list files for backup purposes, ensuring you don’t miss anything important. - Scheduled Tasks: Use
ls
in cron jobs to monitor directories and trigger actions based on file changes. - Log Management: List log files to monitor system performance or errors, helping in troubleshooting.
By mastering the ls command, you can streamline your workflow and improve your efficiency in handling files.
In summary, the ls
command is a versatile tool that can be applied in various scenarios, from file management to data processing and automation scripts. Understanding its practical applications can significantly enhance your programming capabilities.
Resources for Further Learning
Books and Guides
- Python Crash Course: A hands-on introduction to programming with Python.
- Automate the Boring Stuff with Python: Learn to automate everyday tasks with Python.
- Fluent Python: Dive deep into Python’s features and libraries.
Online Tutorials
- Real Python: Offers a variety of tutorials and articles for all skill levels.
- W3Schools: Great for beginners to learn Python basics.
- Codecademy: Interactive courses to practice Python coding.
Community Forums
- Stack Overflow: A place to ask questions and share knowledge.
- Reddit (r/learnpython): A community for Python learners to discuss and share resources.
- Python Discord: Join live discussions and get help from fellow Python enthusiasts.
Explore these resources to enhance your Python skills and tackle advanced topics.
Additional Learning Paths
- Advanced Python Topics Tutorial: In this advanced python topics tutorial, learn about various advanced python concepts with additional resources and master python programming language.
If you’re eager to dive deeper into coding, check out our website for more resources! We offer a variety of interactive tutorials and helpful guides that can boost your skills and prepare you for coding interviews. Don’t wait—start your journey today!
Conclusion
In conclusion, we’ve taken a deep dive into the ‘ls’ command, a vital tool for managing files and directories in Linux. We started with the basics, learning how to use ‘ls’ in simple ways, and then moved on to more advanced features, including various options and flags that enhance its functionality. We also looked at other commands like ‘find’ and ‘tree’ to give you a wider view of file management. Throughout the guide, we addressed common problems you might encounter with ‘ls’, such as permission issues and handling large directories, offering solutions and tips to make your experience smoother. Whether you’re new to the command line or looking to improve your skills, this guide aims to equip you with the knowledge to navigate your Linux directories confidently. Happy exploring!
Frequently Asked Questions
What is the purpose of the Python ls command?
The Python ls command is used to list files and directories in a specific location.
How do I use os.listdir() in Python?
You can use os.listdir() by importing the os module and calling it with the path of the directory you want to list.
What are some common issues when using the ls command?
Common issues include permission errors, hidden files not showing up, and difficulties with large directories.
Can I filter files by type using os.listdir()?
Yes, you can filter files by type using list comprehension with os.listdir() to only include specific file extensions.
What is the difference between os.listdir() and os.scandir()?
os.listdir() returns a simple list of file names, while os.scandir() provides more detailed information about each file.
How can I sort files listed by the ls command?
You can sort files by using the sorted() function in Python, which allows you to sort by name, size, or date.
What are some best practices for using the ls command?
Some best practices include using flags wisely, being cautious with hidden files, and optimizing performance.
Are there alternatives to the ls command in Python?
Yes, alternatives include using the find command, the tree command, or using pathlib for a more object-oriented approach.