Wix: Understanding Async and Await for Better Web Development
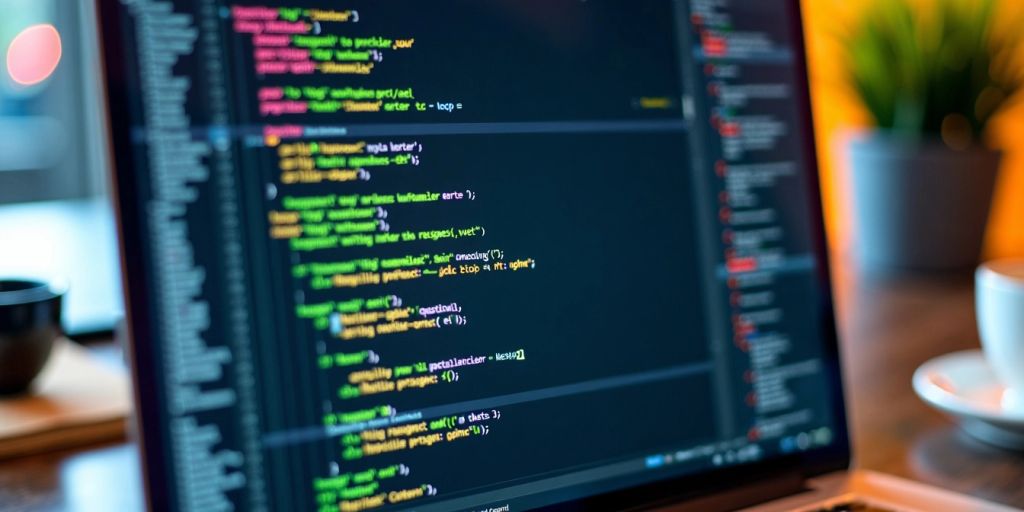
In the world of web development, understanding how to manage asynchronous tasks is essential. This article explores the concepts of async and await in Wix, providing insights that can help improve your coding skills. By breaking down complex topics into simpler ideas, we aim to make learning about these powerful tools accessible to everyone, even beginners. Let’s dive in!
Key Takeaways
- Async and await help make asynchronous code easier to read and write.
- Using async functions allows you to handle tasks without blocking the main program.
- Avoiding callback hell is crucial for keeping your code clean and manageable.
- Proper error handling is essential when working with async functions.
- Optimizing your use of async and await can enhance your website’s performance.
Introduction to Async and Await in Wix
Understanding Asynchronous JavaScript
Asynchronous JavaScript allows your code to run without waiting for tasks to finish. This means you can do other things while waiting for a response, like fetching data from a server. Async and await are tools that help make this easier and cleaner. In 2024, these features have become standard practice for modern web development in platforms like Wix.
The Evolution of Async and Await
Before async and await, developers used callbacks and promises to handle asynchronous tasks. With the introduction of async and await in ES2017, writing asynchronous code became simpler. Instead of chaining multiple .then()
calls, you can write code that looks more like regular, synchronous code. This evolution has significantly improved code readability and maintainability, especially in complex Wix applications.
Why Use Async and Await in Wix
Using async and await in Wix can improve your web development experience. Here are some compelling reasons:
- Cleaner Code: It reduces the complexity of your code, making it more maintainable.
- Easier Debugging: Errors are easier to track down with more straightforward execution flow.
- Better Performance: It allows your application to run more smoothly by preventing UI blocking.
- Improved User Experience: Your website remains responsive even during data-intensive operations.
Async and await make it easier to handle tasks that take time, like fetching data or waiting for user input. This leads to a better user experience and more efficient code.
In Wix, you can leverage async/await with backend functions and web methods. This integration allows for more dynamic and responsive web applications, enabling seamless communication between frontend and backend components.
Setting Up Your Wix Environment for Async/Await
Installing Necessary Tools
To start using async and await in Wix, you need to set up your environment properly. Here are the updated steps for 2024:
- Install the Wix CLI: This tool helps you manage your Wix projects efficiently. Use npm to install it globally with
npm install -g @wix/cli
. - Create a New Project: Use the command
wix create-app my-app
to create a new Wix project with the latest templates. - Add API Extensions: You can add API extensions with the CLI. For example, navigate to your project repo and run
wix generate extension api
. The CLI will display a menu of extensions to generate.
Configuring Your Wix Project
Once you have the necessary tools, configure your project for optimal async/await usage:
- Open your project in the Wix Editor or Wix Studio.
- For Wix Editor: Go to the Settings tab and enable Velo by Wix.
- For Wix Studio: Velo is enabled by default, allowing you to immediately start coding.
- Set up your database collections, as they will be essential for async operations.
- Configure your package.json to include any additional dependencies you might need.
Testing Your Setup
After configuration, it’s important to test your setup with a simple async function:
- Create a test async function to fetch data from your database.
- Use console logs to verify that the data is being fetched correctly.
- Check that your UI updates as expected when the data is retrieved.
- Test error scenarios to ensure proper error handling is in place.
Setting up your environment correctly is crucial for effective web development. It ensures that you can utilize async and await features without issues and sets the foundation for a robust application.
Basic Concepts of Async and Await
Promises vs Callbacks
Asynchronous programming in JavaScript can be handled using callbacks or promises. Here’s an updated comparison for 2024:
Feature | Callbacks | Promises |
---|---|---|
Readability | Can become messy with nesting (callback hell) | More readable and manageable |
Error Handling | Difficult to manage consistently | Easier with .catch() and centralized handling |
Execution Flow | Sequential, can lead to blocking | Non-blocking, allows parallel tasks |
Composition | Challenging to compose multiple operations | Easy to chain and compose with Promise.all() |
Modern Support | Legacy approach, still used in some libraries | Modern standard, widely supported |
Async Functions Explained
An async function is a special type of function that allows you to use the await
keyword inside it. This makes it easier to work with asynchronous code. When you declare a function as async
, it automatically returns a promise, even if you don’t explicitly return one.
Here’s a simple example of an async function in Wix:
async function getUserData(userId) {
// This function automatically returns a promise
const userRecord = await wixData.get("Users", userId);
return userRecord;
}
Async functions enable asynchronous, promise-based behavior to be written in a cleaner style, avoiding the need to explicitly configure promise chains.
The Role of Await in JavaScript
The await
keyword is used to pause the execution of an async function until a promise is resolved or rejected. This means you can write code that looks synchronous, making it easier to read and understand. Here’s a practical example:
async function fetchUserAndOrders() {
// Execution pauses here until the promise resolves
const user = await wixData.get("Users", currentUserId);
// Once user data is available, fetch their orders
const orders = await wixData.query("Orders")
.eq("userId", user._id)
.find();
return {
userData: user,
userOrders: orders.items
};
}
Using async and await can greatly improve the clarity of your code, making it easier to follow the flow of operations. It transforms promise-based code into something that resembles synchronous code, without blocking the main thread.
In summary, understanding these basic concepts is crucial for effectively using async and await in your Wix projects. They help in managing asynchronous operations without getting lost in complex callback structures or promise chains.
Implementing Async and Await in Wix Velo
Writing Your First Async Function
To get started with async functions in Wix Velo, you need to define a function using the async
keyword. This allows you to use await
inside the function. Here’s an updated example for 2024:
import wixData from 'wix-data';
export async function fetchProducts() {
try {
// Await the query result
const results = await wixData.query("Products")
.limit(10)
.sort("createdDate", "desc")
.find();
// Return the items array from the results
return results.items;
} catch (error) {
console.error("Error fetching products:", error);
throw error; // Rethrow to allow proper handling by caller
}
}
Using Await with Wix Data API
When working with the Wix Data API, using await
helps you manage data fetching without blocking the rest of your code. Here’s a comprehensive approach:
- Define your async function with proper error handling.
- Use
await
for database operations like querying, inserting, or updating. - Process the results once the data is ready.
- Update UI elements with the processed data.
Here’s an example of loading data into a repeater:
import wixData from 'wix-data';
$w.onReady(function() {
loadProducts();
});
async function loadProducts() {
try {
// Show loading indicator
$w("#loadingIndicator").show();
// Fetch data with await
const results = await wixData.query("Products")
.eq("inStock", true)
.limit(20)
.find();
// Process data if needed
const processedItems = results.items.map(item => {
return {
...item,
formattedPrice: "$" + item.price.toFixed(2),
isOnSale: item.discount > 0
};
});
// Update UI
$w("#productsRepeater").data = processedItems;
// Hide loading indicator
$w("#loadingIndicator").hide();
} catch (error) {
// Handle errors
console.error("Failed to load products:", error);
$w("#errorMessage").text = "Failed to load products. Please try again.";
$w("#errorMessage").show();
$w("#loadingIndicator").hide();
}
}
Common Pitfalls and How to Avoid Them
When using async and await in Wix, be aware of these common mistakes and their solutions:
- Forgetting to use
await
: If you don’t await a promise, you’ll get a Promise object instead of its resolved value. Always use await with promise-returning functions. - Missing error handling: Wrap async code in try-catch blocks to prevent unhandled promise rejections.
- Awaiting inside loops: This runs operations sequentially. Use
Promise.all()
for parallel execution. - Not handling loading states: Always show loading indicators during async operations to improve user experience.
- Mixing sync and async code improperly: Be consistent in your approach to avoid confusion.
Here’s an example of properly handling multiple async operations in parallel:
async function loadDashboardData() {
try {
// Start all requests simultaneously
const [userPromise, ordersPromise, productsPromise] = [
wixData.get("Users", currentUserId),
wixData.query("Orders").eq("userId", currentUserId).find(),
wixData.query("Products").limit(5).find()
];
// Wait for all to complete
const [user, orders, products] = await Promise.all([
userPromise, ordersPromise, productsPromise
]);
// Now update UI with all data available
updateDashboard(user, orders, products);
} catch (error) {
handleError(error);
}
}
Remember: Async functions always return promises, so handle them properly throughout your application to maintain a consistent flow and avoid unexpected behavior.
By following these guidelines, you can effectively implement async and await in your Wix Velo projects, making your web development smoother and more efficient. This approach allows you to call backend code from the frontend seamlessly, enhancing your application’s performance and user experience.
Advanced Async/Await Techniques in Wix
Error Handling in Async Functions
Proper error handling is crucial when working with async functions in Wix. Here are some advanced techniques for 2024:
- Structured try-catch blocks: Organize your code to catch specific errors at different levels.
- Custom error classes: Create specialized error types for better error identification.
- Centralized error handling: Implement a consistent approach across your application.
Example of advanced error handling:
// Custom error class
class DataFetchError extends Error {
constructor(message, entityType, operation) {
super(message);
this.name = "DataFetchError";
this.entityType = entityType;
this.operation = operation;
this.timestamp = new Date();
}
}
async function fetchUserProfile(userId) {
try {
// Main data fetch
const user = await wixData.get("Users", userId);
// Check if user exists
if (!user) {
throw new DataFetchError(
"User not found",
"User",
"get"
);
}
try {
// Nested operation that might fail independently
const preferences = await wixData.query("UserPreferences")
.eq("userId", userId)
.find();
return {
...user,
preferences: preferences.items[0] || null
};
} catch (preferencesError) {
// Log but don't fail the whole operation
console.error("Failed to fetch preferences:", preferencesError);
// Return user data without preferences
return {
...user,
preferences: null,
preferencesError: true
};
}
} catch (error) {
// Log detailed error information
console.error(`Error in fetchUserProfile: ${error.message}`, {
error,
userId,
timestamp: new Date()
});
// Rethrow with additional context if needed
if (error instanceof DataFetchError) {
throw error;
} else {
throw new DataFetchError(
"Failed to fetch user profile",
"User",
"get"
);
}
}
}
Chaining Promises with Async/Await
Chaining promises becomes much cleaner with async/await. Here are some advanced patterns:
- Sequential processing: Execute operations in a specific order when each depends on the previous result.
- Parallel processing with dependencies: Start independent operations in parallel, then process their results together.
- Dynamic chaining: Create chains based on conditional logic.
Example of advanced promise chaining:
async function processOrderWorkflow(orderId) {
// Get the order details
const order = await wixData.get("Orders", orderId);
// Process payment and inventory in parallel
const [paymentResult, inventoryResult] = await Promise.all([
processPayment(order),
updateInventory(order.items)
]);
// Sequential steps that depend on both payment and inventory
if (paymentResult.success && inventoryResult.success) {
// Update order status
const updatedOrder = await wixData.update("Orders", {
...order,
status: "confirmed",
paymentId: paymentResult.transactionId
});
// Send confirmation email
await sendOrderConfirmation(updatedOrder);
// Return final result
return {
success: true,
order: updatedOrder,
message: "Order processed successfully"
};
} else {
// Handle failures
await handleOrderProcessingFailure(
order,
paymentResult,
inventoryResult
);
return {
success: false,
message: "Order processing failed",
paymentSuccess: paymentResult.success,
inventorySuccess: inventoryResult.success
};
}
}
Optimizing Performance with Async/Await
To ensure your Wix site runs efficiently, consider these advanced optimization techniques:
- Strategic parallelization: Use
Promise.all()
for operations that can run simultaneously. - Implement caching: Store frequently accessed data to reduce database calls.
- Lazy loading: Load data only when needed, especially for content below the fold.
- Request batching: Combine multiple small requests into a single larger request.
- Prioritization: Load critical data first, then less important data.
Example of performance optimization:
// Cache implementation
const cache = new Map();
const CACHE_TTL = 5 * 60 * 1000; // 5 minutes in milliseconds
async function getCachedData(collectionName, query, forceRefresh = false) {
const cacheKey = `${collectionName}:${JSON.stringify(query)}`;
// Check if data is in cache and not expired
const cachedItem = cache.get(cacheKey);
const now = Date.now();
if (!forceRefresh && cachedItem && (now - cachedItem.timestamp < CACHE_TTL)) {
console.log(`Cache hit for ${cacheKey}`);
return cachedItem.data;
}
// Cache miss or forced refresh, fetch from database
console.log(`Cache miss for ${cacheKey}, fetching fresh data`);
const freshData = await wixData.query(collectionName)
.hasSome(query.field, query.values)
.find();
// Update cache
cache.set(cacheKey, {
data: freshData,
timestamp: now
});
return freshData;
}
// Usage in page code
async function loadProductPage() {
// Start loading critical data immediately
const productPromise = wixData.get("Products", productId);
// Update UI with critical data as soon as it's available
const product = await productPromise;
$w("#productTitle").text = product.title;
$w("#productPrice").text = `$${product.price.toFixed(2)}`;
$w("#productImage").src = product.mainImage;
// Now load non-critical data in parallel
$w("#relatedProductsSection").collapse();
$w("#reviewsSection").collapse();
const [relatedProducts, reviews] = await Promise.all([
// Use cached data for related products
getCachedData("Products", {
field: "category",
values: [product.category]
}),
// Always get fresh reviews
wixData.query("Reviews")
.eq("productId", productId)
.limit(5)
.find()
]);
// Update UI with non-critical data
displayRelatedProducts(relatedProducts.items);
displayReviews(reviews.items);
$w("#relatedProductsSection").expand();
$w("#reviewsSection").expand();
}
Tip: Always test your async functions to ensure they perform well under load. Use the Wix Editor's built-in profiling tools to identify performance bottlenecks in your code.
By mastering these advanced techniques, you can enhance your web development skills and create more efficient applications in Wix Velo. Remember, effective error handling and performance optimization are key to successful async programming!
Practical Examples of Async and Await in Wix
Fetching Data Asynchronously
When working with data in Wix, you often need to fetch it from a database. Using async and await makes this process smoother. Here's a modern example for 2024:
import wixData from 'wix-data';
// Function to fetch products with filters
export async function fetchFilteredProducts(category, minPrice, maxPrice, sortBy) {
console.log("Starting to fetch filtered products...");
try {
// Build query dynamically
let query = wixData.query("Products");
// Apply filters conditionally
if (category) {
query = query.eq("category", category);
}
if (minPrice !== undefined) {
query = query.ge("price", minPrice);
}
if (maxPrice !== undefined) {
query = query.le("price", maxPrice);
}
// Apply sorting
if (sortBy === "priceLowToHigh") {
query = query.ascending("price");
} else if (sortBy === "priceHighToLow") {
query = query.descending("price");
} else if (sortBy === "newest") {
query = query.descending("_createdDate");
}
// Execute query
const results = await query.find();
console.log(`Fetched ${results.items.length} products successfully`);
return {
items: results.items,
totalCount: results.totalCount,
hasNext: results.hasNext()
};
} catch (error) {
console.error("Error fetching filtered products:", error);
throw error;
}
}
In this code:
- The function accepts multiple parameters for filtering and sorting.
- It dynamically builds a query based on the provided filters.
- It awaits the query execution without blocking the UI.
- It returns a structured response with the results and metadata.
Updating UI Elements with Async Data
You can use async functions to create dynamic and responsive UI elements. Here's an example of a product page that loads data progressively:
import wixData from 'wix-data';
import wixUsers from 'wix-users';
$w.onReady(function() {
loadProductDetails();
$w("#addToCartButton").onClick(() => addToCart());
});
async function loadProductDetails() {
try {
// Show loading states
$w("#productContainer").hide();
$w("#loadingIndicator").show();
// Get product ID from URL
const productId = getProductIdFromUrl();
// Load main product data
const product = await wixData.get("Products", productId);
// Update main product information
$w("#productTitle").text = product.title;
$w("#productDescription").html = product.description;
$w("#productPrice").text = `$${product.price.toFixed(2)}`;
$w("#productImage").src = product.mainImage;
// Show the product container
$w("#productContainer").show();
$w("#loadingIndicator").hide();
// Load additional data in the background
loadAdditionalProductData(product);
} catch (error) {
console.error("Error loading product:", error);
$w("#errorMessage").text = "Failed to load product details.";
$w("#errorMessage").show();
$w("#loadingIndicator").hide();
}
}
async function loadAdditionalProductData(product) {
try {
// Show loading states for secondary sections
$w("#reviewsContainer").hide();
$w("#relatedProductsContainer").hide();
$w("#secondaryLoading").show();
// Load reviews and related products in parallel
const [reviews, relatedProducts] = await Promise.all([
wixData.query("Reviews")
.eq("productId", product._id)
.limit(5)
.find(),
wixData.query("Products")
.eq("category", product.category)
.ne("_id", product._id)
.limit(4)
.find()
]);
// Update reviews section
if (reviews.items.length > 0) {
$w("#reviewsRepeater").data = reviews.items;
$w("#reviewsContainer").show();
$w("#noReviewsMessage").hide();
} else {
$w("#noReviewsMessage").show();
}
// Update related products
if (relatedProducts.items.length > 0) {
$w("#relatedProductsRepeater").data = relatedProducts.items;
$w("#relatedProductsContainer").show();
}
// Hide loading indicator
$w("#secondaryLoading").hide();
} catch (error) {
console.error("Error loading additional data:", error);
$w("#secondaryLoading").hide();
}
}
async function addToCart() {
try {
// Show loading state on button
$w("#addToCartButton").disable();
$w("#addToCartButton").label = "Adding...";
// Get current user
const currentUser = wixUsers.currentUser;
const isLoggedIn = await currentUser.loggedIn();
if (!isLoggedIn) {
// Handle guest checkout or prompt login
$w("#loginPrompt").show();
return;
}
// Get product ID and quantity
const productId = getProductIdFromUrl();
const quantity = $w("#quantityInput").value;
// Add to cart via backend function
await addProductToCart(productId, quantity);
// Show success message
$w("#successMessage").show();
setTimeout(() => $w("#successMessage").hide(), 3000);
} catch (error) {
console.error("Error adding to cart:", error);
$w("#errorMessage").text = "Failed to add product to cart.";
$w("#errorMessage").show();
} finally {
// Reset button state
$w("#addToCartButton").enable();
$w("#addToCartButton").label = "Add to Cart";
}
}
Handling Multiple Async Operations
For complex scenarios requiring multiple async operations, you can use Promise.all()
for parallel execution or sequential await calls when order matters. Here's an example of a checkout process:
import wixData from 'wix-data';
import wixPay from 'wix-pay';
async function processCheckout(cartId) {
try {
// Step 1: Validate cart contents
const cart = await validateCart(cartId);
// Step 2: Check inventory for all items in parallel
const inventoryChecks = cart.items.map(item =>
checkInventory(item.productId, item.quantity)
);
const inventoryResults = await Promise.all(inventoryChecks);
// If any inventory check failed, abort checkout
const inventoryIssue = inventoryResults.find(result => !result.available);
if (inventoryIssue) {
return {
success: false,
error: "inventoryIssue",
productId: inventoryIssue.productId,
message: `Sorry, ${inventoryIssue.productName} is out of stock.`
};
}
// Step 3: Process payment
const paymentResult = await processPayment(cart);
if (!paymentResult.success) {
return {
success: false,
error: "paymentFailed",
message: paymentResult.message
};
}
// Step 4: Update inventory and create order (these must happen together)
const [inventoryUpdateResult, orderResult] = await Promise.all([
updateInventoryQuantities(cart.items),
createOrder(cart, paymentResult.transactionId)
]);
// Step 5: Send confirmation email (can happen after response)
sendOrderConfirmationEmail(orderResult.orderId)
.catch(error => console.error("Failed to send confirmation email:", error));
// Return success result
return {
success: true,
orderId: orderResult.orderId,
message: "Your order has been placed successfully!"
};
} catch (error) {
console.error("Checkout process failed:", error);
return {
success: false,
error: "systemError",
message: "An unexpected error occurred. Please try again."
};
}
}
Using async and await can greatly improve the readability and maintainability of your code. It allows you to write asynchronous code that looks and behaves like synchronous code, making it easier to understand and debug, while still maintaining the performance