The Ultimate Guide to Writing Prompts for Coding Education
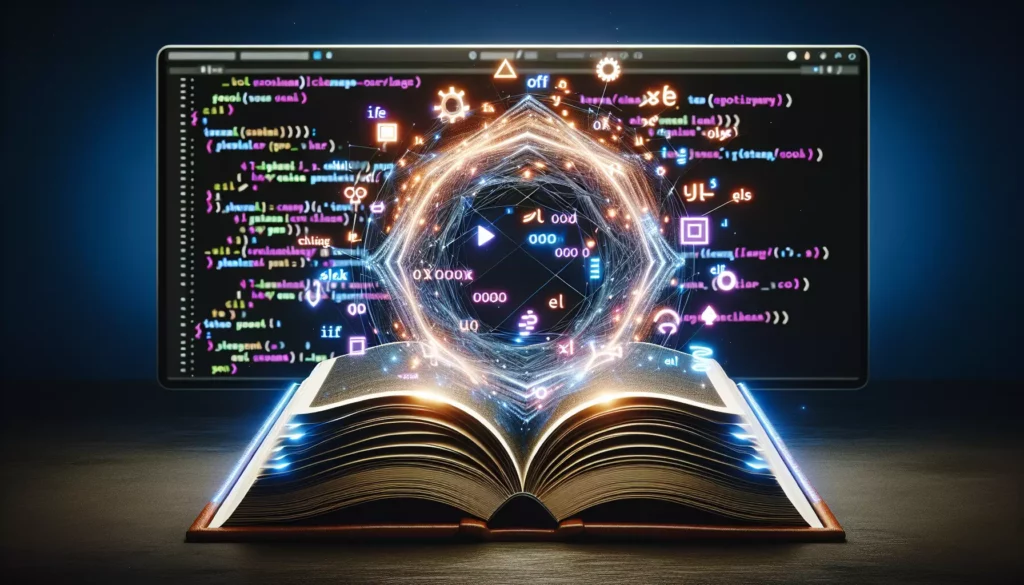
Effective writing prompts are essential tools in coding education. They guide learners through the process of understanding complex programming concepts, developing algorithmic thinking, and building practical skills. Whether you’re an educator looking to create engaging coding challenges or a student seeking structured practice opportunities, understanding how to craft or utilize quality writing prompts can dramatically enhance the learning experience.
In this comprehensive guide, we’ll explore the art and science of writing prompts for coding education, with practical examples and strategies that can be implemented immediately to improve programming skills development.
What Are Coding Writing Prompts?
Coding writing prompts are structured instructions that guide programmers through specific problem-solving tasks. Unlike traditional writing prompts that focus on narrative or expository writing, coding prompts are designed to develop technical skills, algorithmic thinking, and programming knowledge.
These prompts typically include:
- A clear problem statement
- Constraints and requirements
- Input/output examples
- Hints or guidance (optional)
- Learning objectives
Effective coding prompts create a bridge between theoretical knowledge and practical application, allowing learners to reinforce concepts through active problem-solving.
Why Coding Prompts Matter in Programming Education
The journey from beginner programmer to interview-ready professional requires structured practice that builds skills progressively. Here’s why coding prompts are invaluable:
Skill Development Through Deliberate Practice
Research shows that deliberate, focused practice is more effective than passive learning. Coding prompts provide the structure for this deliberate practice, targeting specific skills and concepts.
Bridging Theory and Application
Understanding programming concepts in theory is different from applying them in practice. Prompts create opportunities to translate knowledge into functional code.
Building Problem-Solving Frameworks
Well-designed prompts teach not just syntax but problem-solving approaches that can be applied to novel situations—a critical skill for technical interviews.
Preparing for Real-World Scenarios
The best coding prompts simulate real-world programming challenges, preparing students for actual work environments.
Anatomy of an Effective Coding Prompt
Let’s break down the components that make coding prompts effective learning tools:
Clear Problem Statement
The foundation of any good prompt is a clear, concise problem statement that leaves no ambiguity about what needs to be accomplished.
Example of a clear problem statement:
“Create a function that finds the longest palindromic substring within a given string.”
Contextual Background
Providing context helps learners understand the relevance of the problem and can increase engagement.
“As a developer working on a text analysis tool, you need to identify palindromes in user-submitted content to highlight interesting patterns.”
Specific Requirements and Constraints
Detailed requirements guide the implementation and help learners understand the boundaries of the problem.
“Your function should handle strings up to 1000 characters. It must return the first longest palindromic substring if multiple exist. The solution should have a time complexity better than O(n³).”
Input/Output Examples
Examples clarify expectations and give learners a way to validate their solutions.
Input: "babad"
Output: "bab" (Note: "aba" would also be a valid answer)
Input: "cbbd"
Output: "bb"
Input: "a"
Output: "a"
Learning Objectives
Explicitly stating what the prompt is designed to teach helps learners focus on the right aspects of the problem.
“This problem will help you practice dynamic programming techniques and string manipulation.”
Scaffolded Hints (Optional)
For educational purposes, providing progressive hints can guide learners without giving away the solution.
Hint 1: Consider checking each character as a potential center of a palindrome.
Hint 2: Remember that palindromes can be odd or even length.
Hint 3: Think about expanding outward from each potential center.
Types of Coding Prompts for Different Learning Stages
Different stages of learning require different types of prompts. Here’s how to tailor prompts to various skill levels:
Beginner-Level Prompts
For newcomers to programming, prompts should focus on fundamental concepts and provide more guidance.
Example beginner prompt:
Variable Swap Challenge
Write a function that swaps the values of two variables without using a temporary variable.Example:
If a = 5 and b = 10, after your function runs, a should be 10 and b should be 5.
Intermediate-Level Prompts
These prompts introduce more complex algorithms and data structures, requiring deeper problem-solving skills.
Example intermediate prompt:
Binary Search Tree Validator
Write a function that determines if a given binary tree is a valid binary search tree (BST).Requirements:
A valid BST is defined as follows:
– The left subtree of a node contains only nodes with keys less than the node’s key.
– The right subtree of a node contains only nodes with keys greater than the node’s key.
– Both the left and right subtrees must also be binary search trees.
Advanced-Level Prompts
These challenge experienced programmers and often mirror the complexity of technical interview questions at top companies.
Example advanced prompt:
Median of Data Stream
Design a data structure that supports the following operations:1.
addNum(num)
: Add an integer to the data structure.
2.findMedian()
: Return the median of all elements added so far.Constraints:
– Operations should have optimal time complexity
– Your solution should handle a continuous stream of numbers
Creating Prompts That Target Specific Programming Concepts
Effective prompts often focus on specific programming concepts or algorithms. Here are examples for different focus areas:
Data Structures Focus
LRU Cache Implementation
Implement a Least Recently Used (LRU) cache with get and put operations in O(1) time complexity.Requirements:
–get(key)
: Return the value of the key if it exists, otherwise return -1.
–put(key, value)
: Set or insert the value if the key is not already present. When the cache reaches its capacity, it should invalidate the least recently used item before inserting a new item.
Algorithm Strategy Focus
Merge Intervals
Given an array of intervals where intervals[i] = [starti, endi], merge all overlapping intervals and return an array of the non-overlapping intervals.Example:
Input: intervals = [[1,3],[2,6],[8,10],[15,18]]
Output: [[1,6],[8,10],[15,18]]
Optimization Focus
Maximum Subarray Sum
Find the contiguous subarray within an array of integers that has the largest sum. Implement both a naive solution and an optimized solution using Kadane’s algorithm.Learning Objective:
Understand the difference between an O(n²) and an O(n) approach to this problem.
Integrating Real-World Context into Coding Prompts
Connecting coding challenges to real-world applications increases engagement and helps learners understand the practical relevance of what they’re learning.
Industry-Relevant Scenarios
E-Commerce Product Recommendation Engine
Implement a function that recommends products to users based on their purchase history. Given a user’s past purchases and a database of products with categories, recommend items that match their interests.Requirements:
– Analyze purchase patterns to identify preferred categories
– Rank recommendations by relevance
– Filter out items already purchased
Project-Based Prompts
These prompts span multiple related challenges that build toward a complete project.
Building a URL Shortener Service (Part 1 of 3)
Design the core algorithm for a URL shortening service like bit.ly. Your function should convert a long URL into a unique short code and provide a way to retrieve the original URL using the code.Considerations:
– How will you generate unique codes?
– How will you handle collisions?
– What data structure will you use for efficient storage and retrieval?
Using AI to Generate and Enhance Coding Prompts
AI tools can be valuable for creating varied, challenging prompts that target specific learning objectives.
Prompt Generation Strategies with AI
When using AI to generate coding prompts, provide specific parameters:
- Specify the skill level: “Generate a beginner-level prompt on array manipulation.”
- Define the concept focus: “Create a prompt that teaches recursion through a practical example.”
- Include desired constraints: “The solution should require a time complexity of O(n log n) or better.”
- Request learning scaffolding: “Include three progressive hints that guide without revealing the solution.”
Example AI-Generated Prompt Template
Generate a coding prompt with the following structure:
1. Problem Title: [Descriptive title]
2. Difficulty: [Beginner/Intermediate/Advanced]
3. Concept Focus: [Primary programming concept]
4. Problem Statement: [Clear description of the task]
5. Input/Output Examples: [At least 3 examples]
6. Constraints: [Time/space complexity requirements]
7. Learning Objectives: [What the student should learn]
8. Progressive Hints: [3 hints of increasing specificity]
Evaluating and Improving Coding Prompts
Continuous refinement makes prompts more effective teaching tools.
Clarity Assessment
Ask these questions to evaluate prompt clarity:
- Is the problem statement unambiguous?
- Are all terms clearly defined?
- Do the examples adequately illustrate the expected behavior?
- Are edge cases addressed?
Difficulty Calibration
To ensure appropriate difficulty:
- Test with learners at the target skill level
- Track completion rates and time spent
- Gather feedback on perceived difficulty
- Adjust constraints or requirements as needed
Learning Effectiveness
Measure how well prompts achieve their learning objectives:
- Can learners articulate the concepts after completion?
- Are they able to apply similar techniques to new problems?
- What misconceptions or sticking points emerged?
Implementing Coding Prompts in Different Learning Environments
Coding prompts can be adapted for various educational contexts:
Classroom Settings
In formal educational environments:
- Use prompts as in-class exercises with time limits
- Create collaborative prompts for pair programming
- Design prompts that build on concepts from recent lessons
- Include reflection questions to deepen understanding
Self-Directed Learning
For independent learners:
- Provide more comprehensive context and resources
- Include detailed explanations of solutions
- Offer multiple difficulty levels for the same concept
- Create prompt sequences that build skills progressively
Technical Interview Preparation
For interview-focused practice:
- Simulate interview conditions with time constraints
- Include prompts requiring verbal explanation of approach
- Focus on common interview topics from target companies
- Provide example follow-up questions interviewers might ask
Conclusion: Building a Prompt Library for Continuous Learning
Developing a diverse library of coding prompts creates a valuable resource for ongoing learning and skill development. By categorizing prompts by difficulty, concept focus, and application area, learners can systematically build their programming capabilities.
The most effective prompt libraries grow and evolve based on learner feedback and results. They incorporate new challenges that reflect current industry practices and interview trends, ensuring that the learning experience remains relevant and valuable.
Whether you’re an educator developing curriculum materials, a mentor guiding aspiring programmers, or a learner creating your own practice regimen, investing in quality coding prompts will yield significant returns in programming skill development.
Remember that the ultimate goal of coding prompts is not just to solve individual problems but to build transferable skills and mental models that can be applied to novel challenges. By focusing on conceptual understanding alongside technical implementation, well-crafted prompts become powerful tools in the journey from coding beginner to algorithmic problem-solving expert.