Understanding Network Interface Controllers: A Comprehensive Guide for Programmers
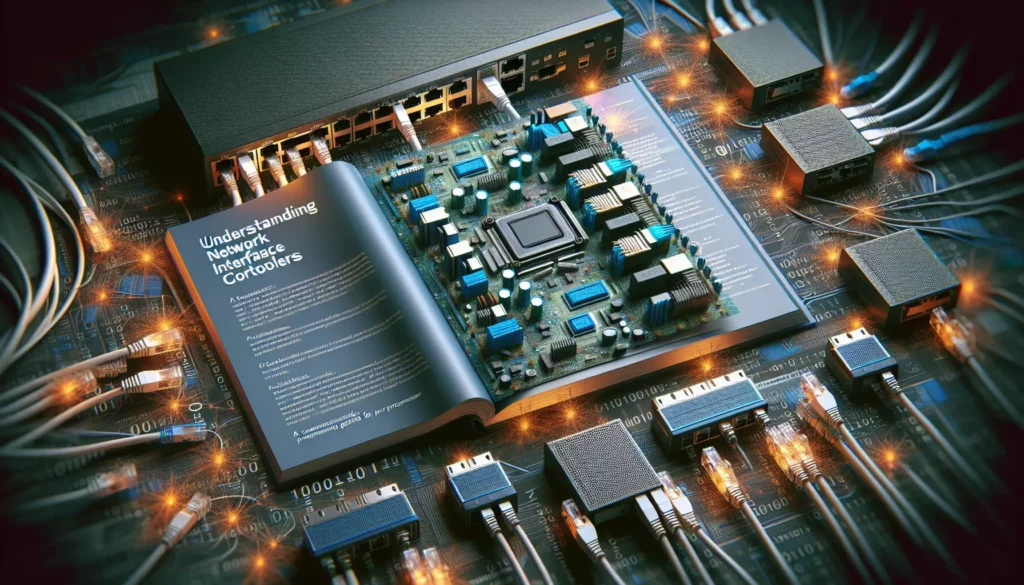
In today’s interconnected world, network interface controllers (NICs) play a crucial role in enabling communication between computing devices. As a programmer, understanding how NICs function is essential for developing efficient network applications, troubleshooting connectivity issues, and optimizing network performance. This article provides an in-depth exploration of network interface controllers, their functionality, types, programming interfaces, and practical applications.
What is a Network Interface Controller?
A Network Interface Controller (NIC), also known as a network interface card, network adapter, or LAN adapter, is a hardware component that connects a computer to a computer network. It serves as the interface between a computer’s internal system and the physical network medium, such as Ethernet cables, fiber optic cables, or wireless signals.
The primary function of a NIC is to prepare, send, and control the flow of data on the network. It operates at both the physical layer (Layer 1) and the data link layer (Layer 2) of the OSI model, handling the transmission of data frames between devices on the same network.
Components of a Network Interface Controller
A typical NIC consists of several key components:
- Physical Connection Interface: This is the port where the network cable connects (like RJ45 for Ethernet) or where wireless antennas are attached.
- MAC Address: Each NIC has a unique Media Access Control (MAC) address burned into its ROM, which serves as its identifier on the network.
- Bus Interface: This allows the NIC to communicate with the computer’s central processing unit (CPU) and memory via interfaces like PCI, PCIe, USB, etc.
- Controller Chipset: The main processor on the NIC that manages network communication protocols.
- Buffer Memory: Temporary storage for data packets being sent or received.
- Boot ROM Socket: Some NICs include this for network booting capabilities.
Types of Network Interface Controllers
Based on Connection Method
1. Internal NICs
These are expansion cards that plug directly into a computer’s motherboard. Modern internal NICs typically use PCI Express slots, though older systems might use PCI or even ISA slots. Many motherboards now come with integrated NICs, eliminating the need for separate expansion cards.
2. External NICs
These connect to a computer through external ports like USB, Thunderbolt, or other peripheral connections. They’re useful for adding network capabilities to devices with limited internal expansion options or for adding additional network interfaces.
Based on Network Technology
1. Ethernet NICs
The most common type, supporting various speeds:
- Fast Ethernet (100 Mbps)
- Gigabit Ethernet (1 Gbps)
- 10 Gigabit Ethernet (10 Gbps)
- 40/100 Gigabit Ethernet (for high-performance computing and data centers)
2. Wireless NICs
These enable connection to wireless networks using standards like:
- Wi-Fi 4 (802.11n)
- Wi-Fi 5 (802.11ac)
- Wi-Fi 6/6E (802.11ax)
3. Fiber Channel NICs
Used primarily in Storage Area Networks (SANs) for high-speed data transfer between servers and storage systems.
4. InfiniBand NICs
Designed for high-performance computing applications with extremely low latency and high throughput.
How NICs Work: The Technical Process
The operation of a NIC involves several steps in the data transmission process:
Sending Data
- The operating system passes data to the NIC driver.
- The driver formats the data into packets with appropriate headers.
- The NIC controller adds the necessary frame information, including MAC addresses.
- The NIC converts the digital signals to the appropriate electrical, optical, or radio signals.
- These signals are transmitted over the network medium.
Receiving Data
- The NIC receives signals from the network medium.
- It converts these signals back into digital data.
- The NIC examines the MAC address to determine if the packet is intended for this device.
- If it is, the NIC generates an interrupt to notify the CPU that data has arrived.
- The data is transferred to the system memory where the operating system can process it.
Programming Interfaces for NICs
As a programmer, you’ll interact with NICs through various programming interfaces. Here are the most common ones:
Socket API
The Socket API is the most widely used interface for network programming. It provides a set of functions for creating network connections, sending and receiving data, and managing network resources. Here’s a simple example of a TCP socket client in C:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <arpa/inet.h>
#include <sys/socket.h>
int main() {
int sock = socket(AF_INET, SOCK_STREAM, 0);
struct sockaddr_in server_addr;
server_addr.sin_family = AF_INET;
server_addr.sin_port = htons(8080);
server_addr.sin_addr.s_addr = inet_addr("127.0.0.1");
connect(sock, (struct sockaddr*)&server_addr, sizeof(server_addr));
char message[] = "Hello from client";
send(sock, message, strlen(message), 0);
char buffer[1024];
recv(sock, buffer, 1024, 0);
printf("Server response: %s\n", buffer);
close(sock);
return 0;
}
Raw Sockets
Raw sockets provide direct access to the underlying network protocols, allowing you to create custom packet headers and implement your own protocols. This is useful for network monitoring, packet sniffing, and developing security tools.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/socket.h>
#include <netinet/ip.h>
#include <netinet/in.h>
int main() {
int sock = socket(AF_INET, SOCK_RAW, IPPROTO_RAW);
if (sock == -1) {
perror("Failed to create raw socket");
exit(1);
}
// Now you can craft custom packets
// ...
close(sock);
return 0;
}
Packet Capture Libraries
Libraries like libpcap (for Unix-like systems) and WinPcap/Npcap (for Windows) provide high-level interfaces for capturing and analyzing network packets. These are commonly used in network analysis tools and intrusion detection systems.
#include <pcap.h>
#include <stdio.h>
void packet_handler(u_char *user_data, const struct pcap_pkthdr *pkthdr, const u_char *packet) {
printf("Packet captured. Length: %d\n", pkthdr->len);
}
int main() {
char errbuf[PCAP_ERRBUF_SIZE];
pcap_t *handle;
handle = pcap_open_live("eth0", BUFSIZ, 1, 1000, errbuf);
if (handle == NULL) {
fprintf(stderr, "Could not open device: %s\n", errbuf);
return 1;
}
pcap_loop(handle, 10, packet_handler, NULL); // Capture 10 packets
pcap_close(handle);
return 0;
}
NDIS (Network Driver Interface Specification)
NDIS is Microsoft’s API for network interface cards. It provides a standard interface between the transport and network layers and the data link layer. Developers can create NDIS drivers or use the NDIS API to interact with network adapters at a low level.
DPDK (Data Plane Development Kit)
DPDK is a set of libraries and drivers for fast packet processing. It bypasses the kernel networking stack to achieve high-performance packet processing, making it ideal for applications like network function virtualization.
Advanced NIC Features for Programmers
Modern NICs offer several advanced features that programmers can leverage for improved performance and functionality:
1. TCP Offloading Engine (TOE)
TOE moves TCP/IP processing from the CPU to the NIC, reducing CPU load and improving network performance. This is particularly useful for high-throughput applications.
2. RSS (Receive Side Scaling)
RSS distributes network receive processing across multiple CPU cores, which is essential for scaling network applications on multi-core systems.
// Example of enabling RSS in Linux using ethtool
system("ethtool -L eth0 combined 4"); // Set 4 combined channels for RSS
3. SR-IOV (Single Root I/O Virtualization)
SR-IOV allows a single physical NIC to appear as multiple virtual NICs, enabling efficient network virtualization. This is particularly important in cloud computing and virtualized environments.
4. RDMA (Remote Direct Memory Access)
RDMA enables direct memory access from the memory of one computer to another without involving the operating system, resulting in high-throughput, low-latency networking.
#include <rdma/rdma_cma.h>
// Simplified RDMA connection setup
struct rdma_cm_id *id;
struct rdma_event_channel *channel;
channel = rdma_create_event_channel();
rdma_create_id(channel, &id, NULL, RDMA_PS_TCP);
// Continue with connection setup and data transfer
Practical Applications and Programming Considerations
Network Programming Best Practices
- Use Non-blocking I/O: For high-performance applications, non-blocking I/O or asynchronous I/O can significantly improve throughput.
- Buffer Management: Efficient buffer management is crucial for minimizing memory usage and maximizing throughput.
- Error Handling: Network programming requires robust error handling to deal with disconnections, timeouts, and other network issues.
- Protocol Selection: Choose the appropriate protocol (TCP, UDP, SCTP) based on your application’s requirements for reliability, ordering, and speed.
Common Use Cases
1. High-Performance Network Applications
For applications requiring maximum throughput, consider:
- Using DPDK for kernel bypass
- Leveraging hardware offloading features
- Implementing zero-copy techniques to reduce memory operations
2. Network Monitoring and Analysis
For building tools like packet sniffers or network analyzers:
- Use libpcap/WinPcap for cross-platform packet capture
- Implement efficient filtering to process only relevant packets
- Consider hardware acceleration for pattern matching
3. Virtualized Environments
When working with virtual machines or containers:
- Utilize SR-IOV for direct hardware access
- Consider DPDK virtual device interfaces for high performance
- Implement proper isolation between virtual interfaces
Troubleshooting NIC Issues in Code
When developing network applications, you may encounter various NIC-related issues. Here are some common problems and their solutions:
1. Performance Bottlenecks
Symptoms: High CPU usage, low throughput, high latency
Solutions:
- Use profiling tools to identify bottlenecks
- Enable hardware offloading features
- Consider using kernel bypass frameworks like DPDK
- Optimize buffer sizes and memory allocation
2. Packet Loss
Symptoms: Missing data, retransmissions, degraded performance
Solutions:
- Increase socket buffer sizes
- Check for NIC buffer overflows using monitoring tools
- Implement flow control mechanisms
- Consider using a more reliable protocol if applicable
// Example of increasing socket buffer size in C
int buffer_size = 262144; // 256KB
setsockopt(sock, SOL_SOCKET, SO_RCVBUF, &buffer_size, sizeof(buffer_size));
3. Compatibility Issues
Symptoms: Connection failures, unexpected behavior across different systems
Solutions:
- Use standard, well-supported APIs
- Implement feature detection and fallback mechanisms
- Test on multiple platforms and with different NIC models
Future Trends in NIC Technology
As a programmer, it’s important to stay informed about emerging NIC technologies that may impact your network applications:
1. SmartNICs and DPUs
Smart Network Interface Cards (SmartNICs) and Data Processing Units (DPUs) include programmable processors that can run custom code directly on the NIC. This enables offloading complex networking tasks, security functions, and even application-specific processing to the network card.
2. 400GbE and Beyond
With the continual increase in network bandwidth requirements, 400 Gigabit Ethernet is becoming more common, and 800GbE and 1.6TbE standards are in development. These ultra-high-speed interfaces will require new programming approaches to fully utilize their capabilities.
3. Software-Defined Networking (SDN) Integration
NICs are increasingly incorporating features to support SDN, allowing for more flexible network configuration and management through programmable interfaces.
Conclusion
Network Interface Controllers are fundamental components in modern computing systems, serving as the bridge between computers and networks. For programmers, understanding how NICs work and how to effectively program them is essential for developing efficient network applications.
From basic socket programming to advanced techniques like RDMA and kernel bypass, the way you interact with NICs can significantly impact your application’s performance and capabilities. By leveraging the appropriate programming interfaces and taking advantage of modern NIC features, you can develop network applications that achieve optimal performance, reliability, and scalability.
As network technologies continue to evolve, staying informed about the latest NIC developments will be crucial for programmers looking to build next-generation network applications. Whether you’re developing high-performance trading systems, real-time multimedia applications, or cloud infrastructure, a deep understanding of NICs will be an invaluable asset in your programming toolkit.