Inverse Document Frequency: How This Key NLP Concept Powers Modern Search Engines
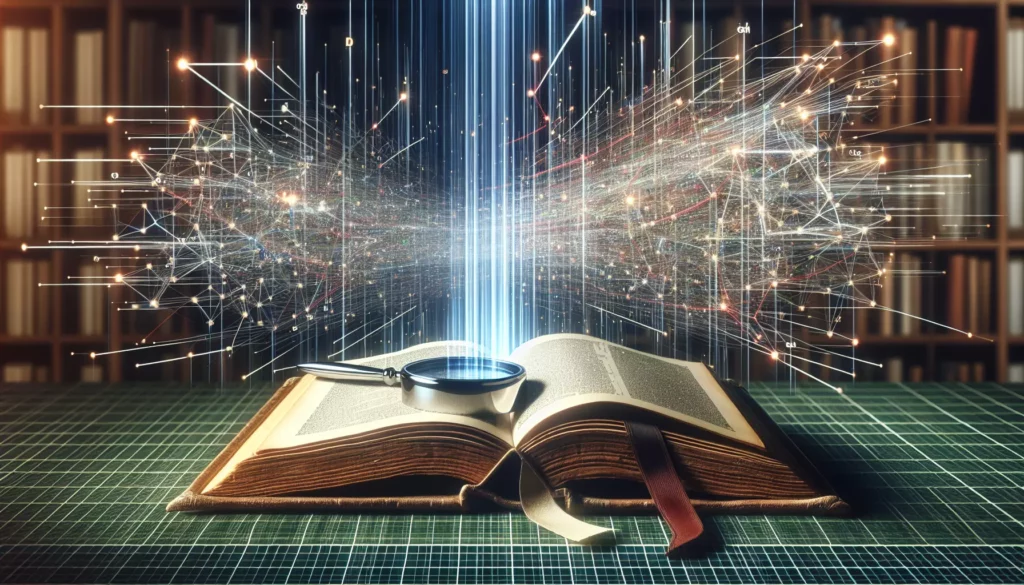
In the world of Natural Language Processing (NLP) and information retrieval, few concepts are as fundamental as Inverse Document Frequency (IDF). This powerful statistical measure helps computers understand the importance of words within documents, enabling everything from Google searches to recommendation systems to work effectively.
Whether you’re a beginner programmer or preparing for technical interviews at major tech companies, understanding IDF is essential for anyone working with text data. In this comprehensive guide, we’ll explore what IDF is, how it works, and how to implement it in your own projects.
What is Inverse Document Frequency?
Inverse Document Frequency (IDF) is a numerical statistic that reflects how important a word is to a document in a collection or corpus. The core idea behind IDF is beautifully simple: words that appear in many documents are less informative than words that appear in few documents.
Think about it this way: common words like “the,” “and,” or “is” appear in virtually every English document. These words don’t tell us much about the specific content of a document. In contrast, specialized terms like “machine learning,” “neural networks,” or “algorithmic complexity” appear in fewer documents and are much more indicative of the document’s subject matter.
IDF quantifies this intuition by assigning lower weights to common terms and higher weights to rare terms. This makes it an invaluable tool for:
- Search engines ranking relevant documents
- Content recommendation systems
- Document classification algorithms
- Text summarization tools
- Sentiment analysis applications
The Mathematics Behind IDF
The formula for calculating the Inverse Document Frequency of a term is:
IDF(t) = log(N / df(t))
Where:
- N is the total number of documents in the corpus
- df(t) is the number of documents containing the term t
- log is typically the natural logarithm, though base 10 is sometimes used
A small modification is often made to avoid division by zero (in case a term doesn’t appear in any document):
IDF(t) = log(N / (1 + df(t))) + 1
The logarithm ensures that the effect of very common or very rare terms is dampened, preventing them from having too extreme an influence on the final scores.
IDF in the Context of TF-IDF
IDF rarely stands alone. It’s most commonly used as part of the TF-IDF (Term Frequency-Inverse Document Frequency) weighting scheme, which combines:
- Term Frequency (TF): How often a word appears in a document
- Inverse Document Frequency (IDF): How rare the word is across all documents
The TF-IDF score for a term in a document is calculated as:
TF-IDF(t, d) = TF(t, d) × IDF(t)
This combination creates a balanced measure that gives high weights to terms that appear frequently in a specific document but are rare across the entire corpus. These are precisely the terms that best characterize a document’s content.
Implementing IDF from Scratch in Python
Let’s implement IDF and TF-IDF from scratch in Python to solidify our understanding. We’ll use a small corpus of documents as an example:
import numpy as np
from collections import Counter
import math
# Our sample corpus
documents = [
"This is the first document about machine learning",
"This document is the second document about NLP",
"And this is the third one about machine learning and NLP",
"Is this the first document about programming?"
]
# Step 1: Tokenize the documents (convert to lowercase and split by space)
tokenized_docs = [doc.lower().split() for doc in documents]
# Step 2: Calculate document frequency for each term
def calculate_df(tokenized_docs):
df = {}
for doc in tokenized_docs:
for term in set(doc): # Using set to count each term only once per document
df[term] = df.get(term, 0) + 1
return df
document_frequency = calculate_df(tokenized_docs)
print("Document Frequency:")
print(document_frequency)
# Step 3: Calculate IDF for each term
def calculate_idf(df, n_docs):
idf = {}
for term, freq in df.items():
idf[term] = math.log(n_docs / (1 + freq)) + 1
return idf
idf_scores = calculate_idf(document_frequency, len(documents))
print("\nIDF Scores:")
print(idf_scores)
# Step 4: Calculate TF-IDF for each document
def calculate_tf_idf(tokenized_docs, idf_scores):
tf_idf_docs = []
for doc in tokenized_docs:
term_counts = Counter(doc)
total_terms = len(doc)
tf_idf = {}
for term, count in term_counts.items():
tf = count / total_terms
tf_idf[term] = tf * idf_scores.get(term, 0)
tf_idf_docs.append(tf_idf)
return tf_idf_docs
tf_idf_scores = calculate_tf_idf(tokenized_docs, idf_scores)
print("\nTF-IDF Scores for Document 1:")
print(tf_idf_scores[0])
This implementation breaks down the process into clear steps:
- Tokenizing each document into individual words
- Calculating how many documents each term appears in (document frequency)
- Computing the IDF score for each term
- Combining TF and IDF to get the final TF-IDF scores
Using Scikit-learn for IDF Calculations
While implementing IDF from scratch is educational, in practice, you’ll often use libraries like scikit-learn, which offer optimized implementations:
from sklearn.feature_extraction.text import TfidfVectorizer
# Our sample corpus
documents = [
"This is the first document about machine learning",
"This document is the second document about NLP",
"And this is the third one about machine learning and NLP",
"Is this the first document about programming?"
]
# Initialize the TF-IDF vectorizer
vectorizer = TfidfVectorizer()
# Fit and transform the documents
tfidf_matrix = vectorizer.fit_transform(documents)
# Get feature names (terms)
feature_names = vectorizer.get_feature_names_out()
# Print the TF-IDF scores for the first document
print("TF-IDF scores for first document:")
first_document_vector = tfidf_matrix[0]
for i, score in enumerate(first_document_vector.toarray()[0]):
if score > 0:
print(f"{feature_names[i]}: {score:.4f}")
The scikit-learn implementation handles all the details automatically, including tokenization, IDF calculation, and vector normalization.
Applications of IDF in Real-world Systems
1. Search Engines
Search engines like Google use IDF (or variants of it) to determine which documents are most relevant to a search query. When you search for “machine learning tutorial,” documents containing these relatively rare terms will be ranked higher than documents containing only common words.
2. Document Classification
IDF helps in building better feature vectors for document classification tasks. By emphasizing distinctive terms, it makes it easier for classification algorithms to distinguish between different categories of documents.
3. Recommendation Systems
Content-based recommendation systems use TF-IDF to create profiles of items (like articles or products) and match them with user preferences. This helps in suggesting content that contains similar distinctive terms to what a user has previously engaged with.
4. Information Extraction
When extracting key information from documents, IDF helps identify which terms are likely to be important and worthy of extraction, filtering out common words that don’t carry significant meaning.
Advanced Considerations for IDF
Smoothing Techniques
In practice, various smoothing techniques are applied to the basic IDF formula to handle edge cases and improve performance:
# Smoothed IDF
IDF_smooth(t) = log((N + 1) / (1 + df(t))) + 1
# Probabilistic IDF
IDF_prob(t) = log((N - df(t)) / df(t))
Handling Out-of-Vocabulary Terms
When applying a pre-computed IDF model to new documents, you might encounter terms that weren’t in the original corpus. Common strategies include:
- Assigning a default maximum IDF value (assuming the term is very rare)
- Ignoring the term entirely
- Using subword tokenization to handle unknown words
IDF in the Age of Word Embeddings
While modern NLP often uses dense vector representations like Word2Vec or BERT embeddings, IDF remains relevant. In fact, IDF-based weighting is sometimes applied to these embeddings to emphasize important terms when aggregating word vectors into document vectors.
Common Challenges and Solutions When Working with IDF
Challenge 1: Corpus Size and Domain Specificity
IDF values are highly dependent on the corpus they’re calculated from. A term might be rare in a general corpus but common in a specialized domain.
Solution: Use domain-specific corpora for calculating IDF when working with specialized text. For example, medical text analysis should use a corpus of medical documents to calculate meaningful IDF values.
Challenge 2: Dynamic Content
In systems where new documents are constantly added, IDF values can become outdated.
Solution: Implement periodic recalculation of IDF values, or use incremental updating techniques that adjust IDF scores as new documents arrive without reprocessing the entire corpus.
Challenge 3: Short Texts
IDF can be less effective for very short texts like tweets or search queries, where the term frequency component is less meaningful.
Solution: For short texts, consider modifying the TF component (e.g., using binary term presence rather than frequency) or incorporate additional features beyond TF-IDF.
Preparing for Technical Interviews: IDF Questions
If you’re preparing for technical interviews at major tech companies, here are some common questions related to IDF that might come up:
- Question: How would you modify the IDF formula to handle a very large corpus where some terms appear in millions of documents?
Approach: Discuss logarithmic scaling and potential normalization techniques. - Question: How would you efficiently update IDF scores in a system where documents are continuously added and removed?
Approach: Explain incremental updating approaches and the tradeoffs involved. - Question: Compare and contrast IDF with more modern NLP techniques like word embeddings.
Approach: Discuss how they serve different purposes and can be complementary. - Question: How would you implement IDF in a distributed system processing billions of documents?
Approach: Outline a MapReduce or similar approach for counting document frequencies at scale.
Conclusion
Inverse Document Frequency stands as one of the most elegant and enduring concepts in information retrieval and natural language processing. Despite being developed decades ago, it remains a cornerstone of many text processing systems today due to its simplicity, effectiveness, and interpretability.
Understanding IDF is not just about implementing a formula; it’s about grasping a fundamental insight about information theory: the rarity of a term across documents is a strong signal of its importance and specificity. This principle extends beyond text analysis and applies broadly to many types of pattern recognition problems.
As you continue your programming journey, whether you’re building search functionality, analyzing customer reviews, or creating content recommendation systems, the concept of IDF will serve as a powerful tool in your algorithmic toolkit.
For further learning, consider implementing IDF-based systems on real-world datasets, exploring variants of the TF-IDF formula, or investigating how IDF principles are incorporated into modern deep learning approaches to NLP.