Why You Struggle to Start Coding From Scratch (And How to Overcome It)
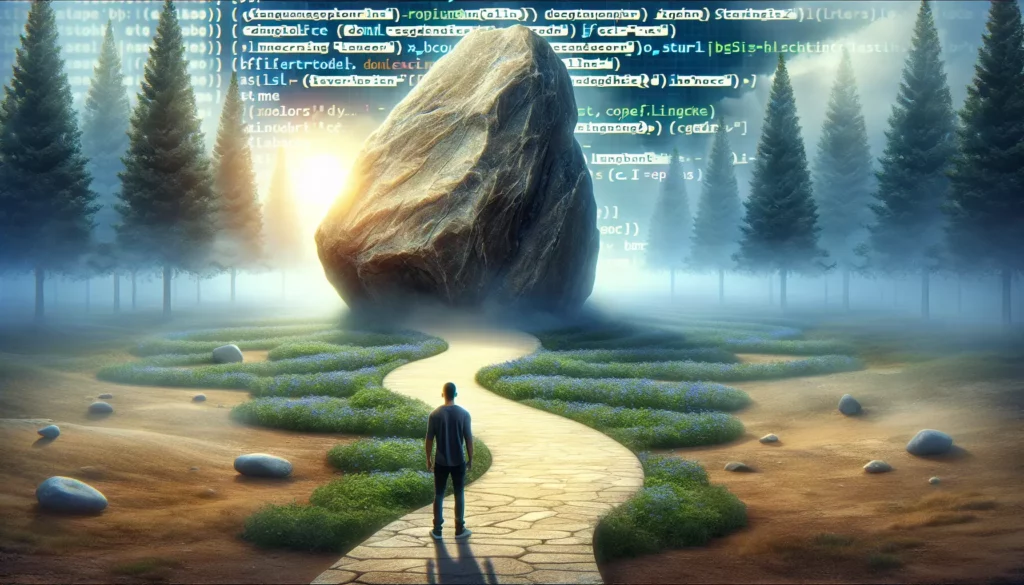
Learning to code is like learning to swim. You can watch countless YouTube tutorials about proper technique, read books about the physics of buoyancy, and listen to podcasts from Olympic swimmers. But until you actually get in the water, you won’t truly learn to swim.
Similarly, many aspiring programmers find themselves trapped in an endless cycle of tutorials and courses without ever building something independently from scratch. This phenomenon, often called “tutorial hell,” keeps many talented individuals from reaching their full potential.
In this comprehensive guide, we’ll explore why starting to code from scratch feels so intimidating, identify the common barriers that hold learners back, and provide actionable strategies to break through these obstacles.
The Blank Screen Paralysis: Understanding the Fear
There’s something uniquely intimidating about a blank code editor. The cursor blinks patiently, waiting for your input, but your mind freezes. This experience is so common that it deserves a proper name: blank screen paralysis.
What causes this paralysis? Several psychological factors are at play:
The Perfection Trap
Many beginners believe their code needs to be perfect from the start. They compare their first attempts to polished projects they see online, creating an impossible standard. This perfectionism leads to analysis paralysis, where the fear of writing imperfect code prevents writing any code at all.
Overwhelming Possibilities
Programming offers nearly infinite ways to solve a problem. For beginners, this freedom can be paralyzing. When there are too many choices and no clear “right” way, decision-making becomes exhausting.
Fear of the Unknown
Starting from scratch means venturing into the unknown. You’ll encounter errors you don’t understand and problems you don’t immediately know how to solve. This uncertainty triggers our brain’s threat detection system, making us uncomfortable and eager to retreat to the safety of structured tutorials.
The Tutorial Comfort Zone
Tutorials provide a comfortable learning environment. They offer:
- Clear step-by-step instructions
- Immediate validation when you complete each step correctly
- A defined endpoint
- Protection from major errors and roadblocks
While these characteristics make tutorials excellent learning tools, they create a significant gap between guided learning and independent creation. In tutorials, someone else has:
- Already broken down the problem
- Determined the architecture
- Made the major design decisions
- Anticipated potential errors
When you code from scratch, all these responsibilities shift to you. This dramatic increase in cognitive load explains why making the leap feels so difficult.
The Hidden Skills Gap
There’s a set of meta-skills that tutorials rarely teach explicitly but are essential for independent coding:
Problem Decomposition
Breaking down a large problem into smaller, manageable pieces is a crucial skill. Tutorials typically present problems that are already decomposed, depriving learners of practice with this fundamental skill.
Research and Resource Finding
Knowing how to find information, evaluate sources, and adapt solutions to your specific needs is essential. When following tutorials, the resources are pre-selected and information is presented exactly when needed.
Debugging and Troubleshooting
In tutorials, errors are often anticipated and solutions provided immediately. Independent coding requires developing your own debugging strategies and the persistence to solve unexpected problems.
Project Planning and Architecture
Deciding how to structure your code, what features to prioritize, and how different components will interact are skills developed through experience. Tutorials make these decisions for you.
The absence of these skills creates a significant barrier when transitioning from following tutorials to building projects independently.
The Knowledge Integration Challenge
Another major struggle comes from the difficulty of integrating knowledge from different sources. Modern programming requires combining multiple technologies, libraries, and concepts.
For example, building even a simple web application might require:
- HTML/CSS for structure and styling
- JavaScript for interactivity
- A framework like React or Vue
- API integration
- Authentication systems
- Database knowledge
- Deployment processes
Most tutorials focus on one or two of these aspects at a time. The challenge comes when you need to integrate these separate pieces of knowledge into a cohesive whole. This integration process is rarely taught explicitly but is essential for independent development.
Strategies to Overcome the Blank Screen
Now that we understand the barriers, let’s explore practical strategies to overcome them and build confidence in coding from scratch.
1. Start Extremely Small
Many beginners aim too high with their first independent projects. Instead of trying to build the next Facebook, start with something truly tiny:
- A function that calculates the average of an array of numbers
- A program that prints a pattern to the console
- A simple HTML page with basic styling
These micro-projects might seem trivial, but they build the confidence to tackle slightly larger challenges. Each successful completion reinforces your ability to create without guidance.
2. Use the Skeleton Technique
Instead of starting with a completely blank file, create a skeleton of your project first:
// 1. Import necessary libraries
// TODO
// 2. Define main function
function main() {
// 3. Get user input
// TODO
// 4. Process data
// TODO
// 5. Display results
// TODO
}
// 6. Call main function
main();
This approach gives you a roadmap to follow and transforms the intimidating blank page into a series of smaller, more manageable tasks.
3. Embrace Incremental Development
Professional developers rarely write large chunks of code without testing. Instead, they:
- Write a small piece of code (maybe just a few lines)
- Test it to make sure it works
- Refine as needed
- Move on to the next piece
This incremental approach prevents the overwhelming feeling of trying to solve everything at once and provides regular positive reinforcement as each small piece works correctly.
4. Practice Deliberate Deconstruction
Next time you follow a tutorial, actively practice the skill of deconstruction:
- Before watching/reading, look at what will be built
- Pause and write down how you would break this project into parts
- Compare your breakdown with the tutorial’s approach
- Note the differences and learn from them
This exercise helps develop the critical skill of problem decomposition that’s essential for independent coding.
5. Use the “Extend the Tutorial” Method
After completing a tutorial, challenge yourself to add one new feature that wasn’t covered. This provides a middle ground between following instructions and creating from scratch. The existing code gives you a foundation, but the new feature requires independent problem-solving.
For example, if you completed a tutorial for a todo list app, try adding:
- A priority level for tasks
- The ability to sort tasks by different criteria
- A dark/light mode toggle
Each extension builds confidence and skills for independent development.
Building Your Technical Toolkit
Beyond psychological strategies, developing specific technical practices will make coding from scratch less intimidating.
1. Master Pseudocode
Pseudocode bridges the gap between human language and programming language. Before writing actual code, describe your solution in plain English (or your native language):
// To find the largest number in an array:
// 1. Set a variable 'largest' to the first element
// 2. Loop through each element in the array
// 3. If the current element is larger than 'largest', update 'largest'
// 4. After the loop, 'largest' contains the maximum value
This approach separates the problem-solving logic from syntax concerns, making both aspects more manageable.
2. Develop a Personal Code Snippet Library
Create a collection of code snippets for operations you frequently need:
- Reading/writing files
- Making API requests
- Setting up a basic server
- Common data transformations
Having these snippets ready reduces the cognitive load when starting new projects. Tools like GitHub Gists or VS Code snippets make this easy to maintain.
3. Learn to Read Documentation Effectively
Documentation is often intimidating for beginners, but it’s an essential skill for independent coding. Practice by:
- Looking up functions you already understand to see how documentation describes familiar concepts
- Using the search function to find specific features rather than reading sequentially
- Focusing on examples and use cases rather than exhaustive details initially
As your comfort with documentation increases, you’ll rely less on tutorials for information.
4. Build a Debugging Protocol
When errors occur (and they will), having a systematic approach prevents panic:
- Read the error message carefully
- Check the line number and surrounding code
- Verify variable values with console.log() or a debugger
- Search for the error message online if still stuck
- Isolate the problem by commenting out sections of code
This methodical approach transforms debugging from a frustrating roadblock into a solvable puzzle.
The Project Selection Strategy
Choosing the right projects is crucial for building the confidence to code independently. Here’s a strategic approach:
1. The “Familiar but Different” Principle
Select projects that use familiar concepts but apply them in slightly different contexts. This creates the right balance of comfort and challenge.
For example, if you’ve built a weather app that fetches data from an API, try building:
- A movie information app using a film database API
- A cryptocurrency price tracker using a financial API
- A recipe finder that uses a food and ingredient API
The core concepts (API requests, data display, user input) remain similar, but the context changes enough to require independent problem-solving.
2. The Feature-First Approach
Instead of thinking about entire applications, focus on implementing specific features:
- Add user authentication to any project
- Implement a dark mode toggle
- Create a data visualization component
- Build a form with validation
This approach makes the scope more manageable and gives you a clear focus for your learning.
3. The Clone-and-Extend Method
Start by cloning a simple existing application, then add your own extensions:
- Build a basic clone of a simple app (todo list, calculator, etc.)
- Get the core functionality working
- Add your own unique features and improvements
This method provides structure while still requiring creativity and independent problem-solving.
Overcoming Common Roadblocks
Even with good strategies, specific roadblocks often arise when coding independently. Here’s how to handle them:
The “I Don’t Know Where to Start” Problem
When you’re completely stuck on how to begin:
- Start with what you can see: the user interface
- Sketch the UI on paper, identifying key components
- For each component, list the functionality it needs
- Begin with the simplest component and implement just the visual part
- Add functionality one small piece at a time
This outside-in approach provides concrete starting points rather than abstract concepts.
The “Too Many Moving Parts” Problem
When your project feels overwhelming due to complexity:
- Create a minimal viable product (MVP) definition
- Ruthlessly eliminate features that aren’t essential
- Implement the core functionality first
- Add complexity only after the basics work
This simplification makes projects more approachable and gives you early wins to build momentum.
The “I Keep Getting Stuck” Problem
When you frequently hit barriers that stop your progress:
- Set a “stuck timer” (15-30 minutes) before seeking help
- Document what you’ve tried and what didn’t work
- Explain the problem out loud (rubber duck debugging)
- Take a short break to let your subconscious work
- If still stuck after your timer, seek targeted help
This approach builds problem-solving resilience while ensuring you don’t waste excessive time on a single issue.
The Power of Structured Practice
Improving your ability to code from scratch requires deliberate practice. Here’s how to structure that practice effectively:
1. The Code Kata Routine
Code katas are small, focused programming exercises designed to be practiced repeatedly. Regular practice with katas builds muscle memory for common programming patterns:
- Dedicate 20-30 minutes daily to solving a small programming challenge
- Implement the solution from scratch without looking at previous attempts
- After completing it, review and refactor your code
- Compare with other solutions to learn alternative approaches
Platforms like Codewars, LeetCode, and HackerRank offer thousands of katas at various difficulty levels.
2. The Reconstruction Exercise
This powerful technique involves rebuilding applications you use regularly:
- Choose a simple application you’re familiar with (calculator, stopwatch, etc.)
- List all the features and functionality
- Close the application and build your own version from memory
- Compare your implementation with the original
This exercise develops your ability to plan and implement complete applications independently.
3. The Time-Boxed Challenge
Set a specific time limit (1-2 hours) and challenge yourself to build something within that constraint:
- A simple game like Tic-Tac-Toe
- A currency converter
- A password generator
The time constraint prevents perfectionism and forces you to focus on core functionality first, which mirrors real-world development priorities.
Leveraging Community and Resources
Independent coding doesn’t mean coding in isolation. Strategic use of community and resources accelerates your progress:
1. Find a Code Review Partner
Having someone review your independent projects provides invaluable feedback:
- Join coding Discord servers or Reddit communities to find partners
- Participate in pair programming sessions
- Offer to review others’ code in exchange for reviews of yours
External feedback highlights blind spots in your approach and exposes you to different problem-solving strategies.
2. Use GitHub Effectively
GitHub isn’t just for storing code; it’s a learning resource:
- Study open-source projects similar to what you want to build
- Examine commit histories to see how projects evolved
- Read issues and pull requests to understand how problems are discussed and solved
- Contribute small fixes to build confidence
This exposure to real-world code and development processes provides context that tutorials often lack.
3. Build a Reference Project Library
Collect examples of well-implemented features and patterns:
- Bookmark repositories with clean implementations of common features
- Save articles that explain architectural decisions
- Create a personal wiki of solutions to problems you’ve solved
This library becomes your personalized reference when tackling new projects.
Mental Models for Independent Coding
Developing effective mental models transforms how you approach coding from scratch:
1. The Iterative Refinement Model
View coding as sculpting rather than building:
- Start with a rough, functioning version (the block of marble)
- Gradually refine and improve it through multiple passes
- Accept that the first version will be imperfect
This model reduces the pressure to get everything right immediately and acknowledges that good code emerges through iteration.
2. The Experimental Mindset
Approach coding as a series of experiments:
- Form hypotheses about how to implement features
- Test these hypotheses with small code samples
- Learn from both successes and failures
- Use this knowledge to inform your next steps
This scientific approach removes the emotional burden of “getting it right” and frames errors as valuable data rather than failures.
3. The Building Block Perspective
See applications as compositions of reusable components:
- Identify common patterns across different applications
- Build a mental library of these patterns
- Approach new projects by assembling and connecting familiar patterns
This perspective transforms the intimidating task of building an entire application into the more manageable process of implementing familiar components and connecting them.
From Struggle to Strength: The Learning Journey
The struggle to code from scratch isn’t a sign of inadequacy; it’s a natural part of the learning process. Every professional developer has faced the same challenges.
What separates successful developers isn’t innate talent but persistence and strategic learning. The ability to start from a blank editor and create something meaningful is a skill developed through practice, not a talent you either have or don’t.
The strategies outlined in this guide provide a roadmap for this development:
- Start with tiny projects to build confidence
- Use scaffolding techniques to overcome blank screen paralysis
- Develop the meta-skills of problem decomposition and research
- Practice incrementally with gradually increasing independence
- Build a personal toolkit of snippets and resources
- Engage with community for feedback and support
As you apply these approaches, you’ll notice a gradual shift. What once seemed impossibly complex becomes manageable. The blank screen transforms from an intimidating void to an exciting canvas of possibility.
Practical Next Steps
To put this guide into action:
- Complete a tutorial you’re currently following, then immediately add one new feature independently
- Set aside 30 minutes tomorrow to create something tiny from scratch
- Start building your personal code snippet library with 2-3 patterns you use frequently
- Join a coding community and share your progress
- Schedule regular “from scratch” practice sessions in your calendar
Remember that learning to code independently is not a destination but a continuous journey of growth. Each project builds your capabilities, and even experienced developers constantly learn new approaches.
The struggle you feel is not just normal; it’s necessary. It’s through this productive struggle that you develop the problem-solving skills and resilience that define successful developers.
Start small, persist through challenges, and celebrate your progress. The ability to transform a blank screen into functioning code is a superpower worth developing, and it’s within your reach.