Why You Can’t Predict Edge Cases Before They Occur
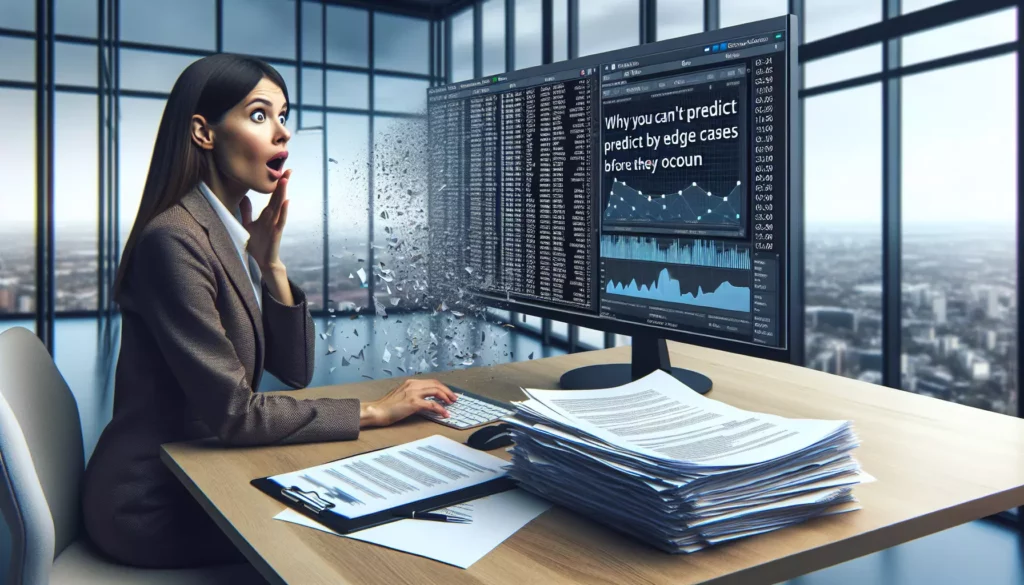
Every programmer has experienced that moment of dread. You’ve spent hours meticulously crafting code, testing it thoroughly, and confidently deploying it to production. Then, seemingly out of nowhere, your application crashes spectacularly when a user inputs something you never considered possible. Welcome to the world of edge cases—those unexpected scenarios that lurk in the shadows of your codebase, waiting for the perfect moment to emerge and wreak havoc.
In the realm of software development, edge cases represent the extreme or unusual situations that occur at the boundaries of normal operation. They’re the scenarios that fall outside the typical use cases you envision when designing your solution. Despite our best efforts to anticipate them, edge cases have an uncanny ability to surprise even the most experienced developers.
This article explores why predicting all edge cases before they occur is fundamentally impossible, how to approach this reality, and strategies to minimize their impact when they inevitably arise.
The Fundamental Unpredictability of Edge Cases
Let’s start with a bold claim: it is theoretically impossible to predict all edge cases before they occur. This isn’t due to a lack of skill or diligence—it’s a fundamental limitation rooted in the nature of complex systems and human cognition.
The Complexity Problem
Modern software systems are incredibly complex, with countless interactions between components, users, and external systems. Consider a simple web application that processes user input. Even with just a handful of input fields, the number of possible combinations grows exponentially. Factor in different browsers, devices, network conditions, and user behaviors, and you’re dealing with a virtually infinite state space.
This complexity means that even if you could test a million different scenarios, you’d still only cover a tiny fraction of the possible states your system might encounter. It’s mathematically impossible to test all permutations.
Take this seemingly simple function that divides two numbers:
function divide(a, b) {
return a / b;
}
At first glance, this looks straightforward. But what if:
- b is zero (division by zero)
- a or b is not a number
- a or b is infinity
- a or b is undefined or null
- a or b is a string that can be converted to a number
- a or b is a string that can’t be converted to a number
- The result exceeds the maximum value that can be represented
And that’s just for a two-line function! Imagine the explosion of possible edge cases in a real-world application with thousands of functions interacting with each other.
The Cognitive Limitation
Human cognition itself poses another barrier to predicting edge cases. Our brains are wired to think about typical scenarios and common use cases. We naturally focus on the “happy path”—the expected flow of operations when everything works correctly.
This cognitive bias makes it difficult to imagine all the ways things could go wrong. We tend to overlook rare events and unusual combinations of circumstances. Even when we try to think about edge cases, we’re limited by our own experiences and imagination.
As Donald Rumsfeld famously put it, there are “unknown unknowns”—the things we don’t know that we don’t know. These represent the edge cases we can’t predict because they lie completely outside our frame of reference.
The Real-World Complexity
Software doesn’t exist in a vacuum. It operates in the messy, unpredictable real world, interacting with users who behave in unexpected ways and systems that may not follow specifications.
Consider these real-world edge cases that would be difficult to predict:
- A user with an unusually long name that breaks your UI layout
- A database query that works fine with small datasets but times out with production-scale data
- A third-party API that occasionally returns malformed data
- A leap year bug that only manifests every four years
- A user who copies and pastes emoji characters into a form field designed for addresses
These scenarios often emerge from the intersection of multiple systems and human behavior, making them particularly difficult to anticipate during development.
Historical Examples of Unpredictable Edge Cases
The history of software development is littered with examples of edge cases that caused significant problems despite the best efforts of talented engineering teams.
The Y2K Bug
Perhaps the most famous edge case in computing history, the Y2K bug resulted from a design decision to represent years using only two digits to save precious memory. This seemed reasonable in the 1960s and 1970s when storage was expensive and no one imagined these systems would still be running in the year 2000.
When the millennium approached, there was widespread concern that systems would interpret “00” as 1900 instead of 2000, potentially causing catastrophic failures. While the actual impact was less severe than feared (partly due to extensive remediation efforts), this remains a classic example of an edge case that became apparent only decades after the original code was written.
The Mars Climate Orbiter Disaster
In 1999, NASA lost the $125 million Mars Climate Orbiter because of a simple unit conversion error. One team used metric units (newtons) while another used imperial units (pound-force) without proper conversion. This resulted in the spacecraft’s navigation commands being off by a factor of 4.45, causing it to enter Mars’ atmosphere at the wrong angle and disintegrate.
This edge case arose from the interaction between two teams with different assumptions—something that unit tests or code reviews might not have caught if no one specifically looked for this type of inconsistency.
The Ariane 5 Rocket Explosion
In 1996, the Ariane 5 rocket exploded just 40 seconds after launch due to a software error. The cause? A piece of code from the Ariane 4 was reused without considering that the Ariane 5 had different flight characteristics. Specifically, a 64-bit floating-point number was converted to a 16-bit signed integer, which caused an overflow when the rocket accelerated faster than the Ariane 4 ever could.
This edge case couldn’t have been discovered through normal testing because it only manifested under the actual flight conditions of the new rocket.
Modern Edge Case Examples
More recent examples abound:
- In 2017, a typo in an Amazon S3 command during routine server maintenance took down a significant portion of the internet
- The Heartbleed bug exposed millions of servers due to a missing bounds check in OpenSSL’s implementation of the TLS heartbeat extension
- A leap second added on June 30, 2012, crashed applications and websites including Reddit, LinkedIn, and Qantas Airways’ reservation system
Each of these cases represents an edge condition that wasn’t anticipated during development or testing, despite rigorous processes at these organizations.
The Theoretical Impossibility of Complete Testing
From a theoretical computer science perspective, the problem of predicting all edge cases is related to several fundamental limitations.
The Halting Problem
Alan Turing proved in 1936 that it’s impossible to create a general algorithm that can determine whether any given program will eventually halt or run forever for all possible inputs. This result, known as the Halting Problem, establishes a fundamental limit on what we can know about program behavior.
By extension, we cannot create a program that can automatically identify all possible edge cases in another program. Some edge cases might only be discoverable by actually running the program with specific inputs under specific conditions.
State Space Explosion
The number of possible states in even a moderately complex program is astronomical. Consider a program with just 100 boolean variables—a trivial example by modern standards. This program has 2^100 possible states, which is more than the estimated number of atoms in the observable universe.
No amount of testing can cover all these states, forcing us to be selective about which scenarios we examine. This selection process inevitably leaves gaps where edge cases can hide.
Combinatorial Explosion
The problem gets exponentially worse when we consider the interactions between different components. If a system has 10 components that can each be in 10 different states, there are 10^10 (10 billion) possible combinations to test. Add external factors like timing, load conditions, and user inputs, and complete testing becomes practically impossible.
This is why even companies with enormous testing resources like Google, Microsoft, and Apple still release software with bugs and vulnerabilities—it’s not for lack of trying, but due to the fundamental impossibility of exhaustive testing.
Practical Limitations in Development Environments
Beyond the theoretical limitations, several practical factors make edge case prediction difficult in real-world development environments.
Time and Resource Constraints
Software development operates under business constraints. Teams have deadlines, budgets, and competing priorities. Given these limitations, it’s impossible to spend unlimited time exploring every potential edge case.
When faced with shipping a product on time versus exploring obscure edge cases, business pressures often favor shipping. This isn’t necessarily wrong—software that’s never released provides no value—but it does mean that some edge cases will inevitably slip through.
Changing Requirements and Environments
Software requirements and environments constantly evolve. What works perfectly today may break tomorrow when:
- A new browser version is released
- A third-party API changes its response format
- User behavior shifts in unexpected ways
- New features interact with existing code
- Hardware capabilities change
These changing conditions create new edge cases that couldn’t have been predicted during initial development because they didn’t exist yet.
Human Factors
Software is built by humans, and humans make mistakes. Even with code reviews, pair programming, and other quality practices, errors will occur. Sometimes these errors create edge cases that aren’t apparent until specific conditions arise in production.
Additionally, knowledge silos and communication gaps between teams can create edge cases at the boundaries between components. When one team misunderstands the assumptions or guarantees provided by another team’s code, edge cases can emerge in the integration points.
Strategies for Dealing with Unpredictable Edge Cases
Given that we can’t predict all edge cases, how should developers approach this reality? Here are some strategies that help manage the unpredictable nature of edge cases:
Defensive Programming
Defensive programming assumes that your code will encounter unexpected inputs and conditions, and designs accordingly. This includes:
- Input validation
- Error handling
- Boundary checking
- Fail-safe defaults
- Graceful degradation
By assuming that things will go wrong, defensive programming creates code that’s more resilient when edge cases occur.
For example, instead of our naive division function from earlier, a defensively programmed version might look like:
function safeDivide(a, b) {
// Check if inputs are valid numbers
if (typeof a !== 'number' || isNaN(a)) {
throw new Error('First argument must be a valid number');
}
if (typeof b !== 'number' || isNaN(b)) {
throw new Error('Second argument must be a valid number');
}
// Check for division by zero
if (b === 0) {
throw new Error('Cannot divide by zero');
}
// Perform the division
const result = a / b;
// Check for overflow or other issues
if (!isFinite(result)) {
throw new Error('Result is not a finite number');
}
return result;
}
This function handles many potential edge cases explicitly, making the behavior more predictable even in unusual situations.
Property-Based Testing
Traditional unit testing checks specific examples, but property-based testing generates many random inputs to test properties that should always hold true. This approach can uncover edge cases that wouldn’t be found through manual test case creation.
Libraries like QuickCheck (Haskell), ScalaCheck (Scala), and jest-property-based (JavaScript) enable this style of testing. For example, with jest-property-based, you might write:
test('safeDivide should always return a finite number for valid inputs', () => {
fc.assert(
fc.property(
fc.float().filter(a => isFinite(a) && !isNaN(a)),
fc.float().filter(b => isFinite(b) && !isNaN(b) && b !== 0),
(a, b) => {
const result = safeDivide(a, b);
return isFinite(result) && !isNaN(result);
}
)
);
});
This test generates hundreds of random input pairs and verifies that our function behaves correctly for all of them, potentially revealing edge cases we wouldn’t have thought to test manually.
Chaos Engineering
Pioneered by Netflix, chaos engineering deliberately introduces failures into systems to test their resilience. Tools like Chaos Monkey randomly terminate instances in production to ensure the system can handle unexpected failures.
This approach acknowledges that failures will occur and focuses on building systems that degrade gracefully rather than catastrophically when they do. By intentionally creating “controlled accidents,” teams can discover and address edge cases before they cause significant problems.
Monitoring and Observability
Since we can’t prevent all edge cases, robust monitoring and observability become critical. This includes:
- Comprehensive logging
- Error tracking
- Performance monitoring
- User behavior analytics
- Alerting systems
When edge cases do occur, these tools help teams quickly identify what went wrong and how to fix it. They also provide data that can inform future development and testing efforts.
Gradual Rollouts and Feature Flags
Rather than deploying new code to all users simultaneously, gradual rollouts limit the impact of unexpected edge cases. Feature flags allow teams to enable functionality for specific user segments or quickly disable problematic features without a full deployment.
This approach turns edge case discovery into a controlled process rather than an emergency response situation.
Building a Culture of Learning
Perhaps most importantly, teams should foster a culture that treats edge cases as learning opportunities rather than failures. Blameless postmortems, shared incident reports, and knowledge bases of past issues help the entire organization learn from edge cases when they occur.
By systematically capturing and sharing these lessons, teams can improve their collective ability to anticipate similar issues in the future.
Case Study: Learning from Real-World Edge Cases
Let’s examine a real-world scenario that illustrates both the unpredictability of edge cases and effective strategies for handling them.
The Unicode Username Problem
Imagine a social media platform that allows users to choose usernames. The developers implement reasonable restrictions: usernames must be 3-20 characters and contain only alphanumeric characters, underscores, and hyphens. They test extensively with various valid and invalid usernames.
After launch, everything works fine until a user discovers they can register a username that appears identical to another user’s name but uses different Unicode characters. For example, the Latin “a” (U+0061) looks identical to the Cyrillic “а” (U+0430) in many fonts. This enables impersonation attacks where malicious users create accounts that visually match those of other users.
This edge case wasn’t anticipated because:
- The developers were thinking in terms of ASCII characters, not the full Unicode space
- The visual similarity of different Unicode characters wasn’t considered during security testing
- The regex pattern used for validation (/^[a-zA-Z0-9_-]{3,20}$/) correctly enforced the stated rules but didn’t address this specific security concern
The Solution
After discovering this issue through user reports, the team implements several mitigations:
- Immediate fix: They add Unicode normalization to convert all usernames to a canonical form before storing and comparing them
- Long-term solution: They implement a confusable character detection system based on Unicode’s “confusables” data
- Monitoring: They add alerts for suspicious registration patterns that might indicate impersonation attempts
- Knowledge sharing: They document the issue in their security guidelines and share the lesson with other teams
The code for the improved username validation might look like:
function isValidUsername(username) {
// Basic validation
if (!/^[a-zA-Z0-9_-]{3,20}$/.test(username)) {
return { valid: false, reason: 'Username must be 3-20 characters and contain only letters, numbers, underscores, and hyphens' };
}
// Normalize the username
const normalizedUsername = username.normalize('NFKC');
// Check if normalization changed anything
if (normalizedUsername !== username) {
return { valid: false, reason: 'Username contains invalid Unicode characters' };
}
// Check for confusable characters
if (containsConfusableCharacters(username)) {
return { valid: false, reason: 'Username contains characters that could be confused with others' };
}
// Check if this normalized username already exists
if (usernameExistsInDatabase(normalizedUsername)) {
return { valid: false, reason: 'This username is too similar to an existing username' };
}
return { valid: true };
}
This example demonstrates how an unpredictable edge case led to improved systems and processes. The team couldn’t have reasonably anticipated this specific issue before it occurred, but they were able to learn from it and strengthen their system as a result.
Embracing Uncertainty in Software Development
The unpredictability of edge cases isn’t a failure of software engineering—it’s an inherent characteristic of complex systems. Rather than striving for the impossible goal of predicting all edge cases, successful teams embrace this uncertainty and build processes that acknowledge it.
The Shift from Prevention to Resilience
This reality requires a mindset shift from trying to prevent all problems to building resilient systems that can detect, contain, and recover from unexpected issues. This doesn’t mean abandoning careful design and testing—these remain essential practices. But it does mean supplementing them with strategies that assume some edge cases will inevitably slip through.
Continuous Learning and Adaptation
The most successful teams view edge cases as valuable feedback that drives continuous improvement. Each edge case discovered in production becomes a learning opportunity that makes the system more robust over time.
This perspective transforms edge cases from frustrating failures into essential components of the development process—each one reveals something about the system that wasn’t previously understood.
Building Fault-Tolerant Systems
Given that we can’t predict all edge cases, designing systems to be fault-tolerant becomes crucial. This includes:
- Microservice architectures that contain failures to specific components
- Circuit breakers that prevent cascading failures
- Redundancy and fallback mechanisms
- Automatic recovery processes
- Data validation at system boundaries
These approaches acknowledge that failures will occur and focus on minimizing their impact rather than trying to eliminate them entirely.
Conclusion: Thriving in the Face of Uncertainty
The fundamental unpredictability of edge cases isn’t a reason for despair—it’s an invitation to adopt more sophisticated approaches to software development. By embracing uncertainty and building systems that can adapt to the unexpected, we create more resilient software that delivers value even in the face of edge cases we couldn’t have predicted.
Remember that every major software system in existence today operates successfully despite containing undiscovered edge cases. The goal isn’t perfection—it’s creating systems that provide value while gracefully handling the inevitable surprises that emerge as they interact with the real world.
As you design and build your next system, consider not just how it will handle the scenarios you can imagine, but how it will respond to the ones you can’t. This perspective—acknowledging the limits of prediction while building for resilience—is the mark of truly mature software engineering.
Edge cases will always exist beyond the boundaries of our imagination, but with the right approaches, they need not define the success of our systems. By combining thorough testing with defensive programming, monitoring, and a culture of learning, we can build software that thrives even when confronted with the unexpected corners of its possibility space.
After all, it’s not about predicting every possible edge case—it’s about building systems that can handle them when they inevitably appear.