Why You Struggle to Learn From Your Mistakes (And How to Fix It)
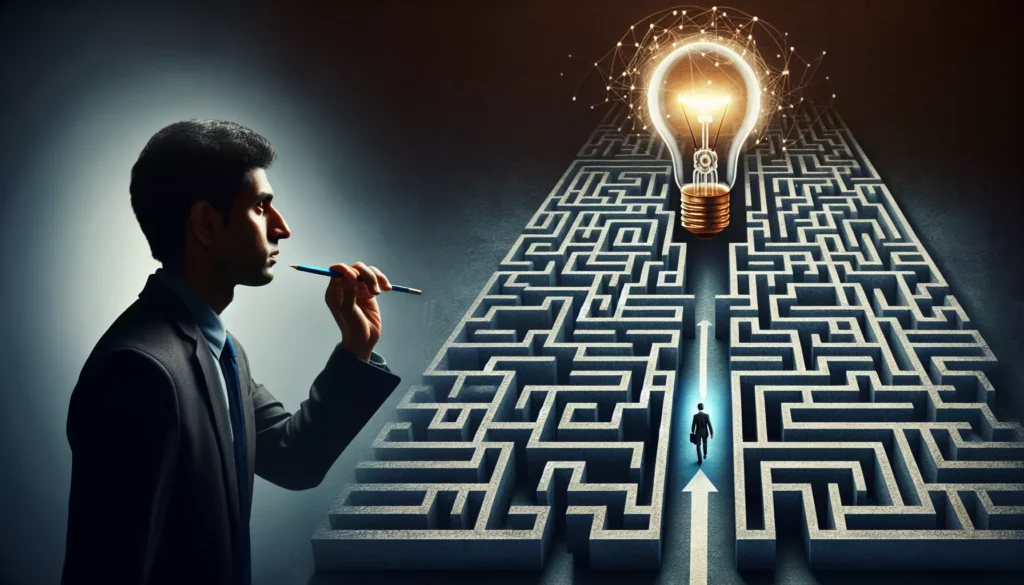
Have you ever found yourself making the same coding error multiple times? Or perhaps you’ve bombed several technical interviews in the same way? Learning from mistakes is often touted as one of the most effective ways to grow as a programmer, yet many of us continue to repeat the same errors despite our best intentions.
In the world of programming and algorithmic thinking, mistakes are inevitable. What separates successful developers from those who stagnate isn’t avoiding errors altogether—it’s the ability to extract valuable lessons from each misstep and apply them moving forward.
In this comprehensive guide, we’ll explore the psychological and practical barriers that prevent effective learning from mistakes, particularly in the context of coding and technical skill development. More importantly, we’ll provide actionable strategies to transform your relationship with errors and accelerate your growth as a programmer.
The Science Behind Learning From Mistakes
Before diving into specific coding scenarios, it’s important to understand the fundamental cognitive processes that influence how we learn from errors.
The Neurological Basis of Error Processing
When you make a mistake while coding, your brain produces a distinct neural signature. Research in cognitive neuroscience has identified an “error-related negativity” (ERN) signal that occurs within milliseconds of making an error. This signal, originating in the anterior cingulate cortex, acts as an alarm system to alert you that something has gone wrong.
However, the mere presence of this signal doesn’t guarantee learning. What matters is what happens next: the conscious processing of the error, the emotional response, and the steps taken to correct and understand the mistake.
The Role of Emotional Responses
Your emotional reaction to mistakes significantly impacts your ability to learn from them. When you encounter a bug in your code or fail a technical interview question, you might experience:
- Frustration: “Why isn’t this working? I’ve been stuck for hours!”
- Embarrassment: “My team will think I’m incompetent if they see this error.”
- Anxiety: “What if I can’t solve this? Maybe I’m not cut out for programming.”
- Defensiveness: “The requirements weren’t clear. Anyone would have made this mistake.”
These emotional responses can either facilitate or hinder learning. Moderate levels of frustration might motivate you to dig deeper into understanding an error, while excessive anxiety could trigger avoidance behaviors that prevent effective learning.
Common Barriers to Learning From Coding Mistakes
Let’s examine the specific obstacles that programmers face when trying to learn from their errors.
1. The “Quick Fix” Mentality
In the pressure to meet deadlines or move on to the next feature, many developers fall into the trap of implementing quick fixes without understanding the root cause of a problem.
Consider this scenario: You encounter a bug where your sorting algorithm occasionally produces incorrect results. Instead of investigating why, you add an extra validation check that seems to resolve the immediate issue:
// Quick fix without understanding the root cause
if (result.length > 0 && !isValidSortOrder(result)) {
// Force a resort with a more reliable but slower algorithm
return backupSort(array);
}
While this approach might temporarily solve the problem, it fails to address the underlying issue in your primary sorting algorithm. The next time you encounter a similar problem, you’ll lack the deep understanding needed to solve it effectively.
2. Cognitive Biases in Error Attribution
Our brains are wired with various cognitive biases that can distort how we interpret our mistakes:
- Fundamental Attribution Error: Attributing failures to external factors (“The documentation was unclear”) while crediting successes to internal factors (“My coding skills are exceptional”).
- Confirmation Bias: Seeking information that confirms our existing beliefs about our abilities or the source of errors.
- Hindsight Bias: After finding a bug, thinking “I should have seen that coming” without acknowledging the complexity of the problem when you first encountered it.
These biases can prevent an accurate assessment of what went wrong and why, limiting the lessons you extract from each mistake.
3. Inadequate Reflection Practices
Many programmers don’t have structured approaches for reflecting on mistakes. After fixing a bug or failing an interview question, they move on without:
- Documenting the error and solution
- Identifying patterns across multiple mistakes
- Creating systems to prevent similar errors in the future
- Sharing lessons learned with teammates or the broader community
Without deliberate reflection, valuable insights remain uncaptured and the opportunity for deeper learning is lost.
4. Fear of Failure and Perfectionism
In the competitive world of programming, many developers develop perfectionist tendencies and a heightened fear of failure. This is particularly common among those preparing for technical interviews at prestigious companies.
This fear can manifest as:
- Avoiding challenging problems that might result in failure
- Hiding mistakes from teammates
- Experiencing paralyzing anxiety when errors occur
- Being overly critical of oneself when making mistakes
Such perfectionism creates an environment where mistakes are seen as threats rather than learning opportunities.
5. Isolation and Lack of Feedback
Learning from mistakes is significantly more difficult without external feedback. Programmers who work in isolation or in environments with limited code reviews often miss out on valuable perspectives that could enhance their understanding of errors.
Even experienced developers benefit from having others review their approach to problem-solving and identify blind spots in their thinking.
The Impact on Technical Interview Preparation
These barriers to learning from mistakes can be particularly detrimental when preparing for technical interviews at major tech companies. Let’s examine how they manifest in this specific context:
The High Stakes of Technical Interviews
Technical interviews, especially at FAANG companies (Facebook/Meta, Amazon, Apple, Netflix, Google) and other top tech firms, are known for their challenging algorithmic problems and high pressure environments.
When practicing for these interviews, candidates often:
- Rush through problems without thoroughly understanding solutions
- Move on to new problems rather than deeply reviewing failed attempts
- Focus on quantity of problems solved rather than quality of learning
- Become discouraged after failing to solve complex problems
This approach leads to superficial understanding and missed opportunities for growth.
The Pattern Recognition Problem
Success in technical interviews often depends on recognizing problem patterns and applying appropriate algorithms and data structures. However, many candidates fail to extract these patterns from their practice sessions.
For example, a candidate might solve several dynamic programming problems without recognizing the common principles that connect them. When faced with a novel dynamic programming problem in an interview, they struggle to apply previous learnings.
Transforming Your Approach: Strategies for Effective Learning from Mistakes
Now that we understand the barriers, let’s explore practical strategies to overcome them and maximize learning from each coding mistake.
1. Implement a “Post-Mortem” Practice
After encountering a significant bug or failing to solve a coding problem, conduct a structured review:
- Document the error: Record what happened, including the specific code or approach that didn’t work.
- Analyze the root cause: Go beyond the immediate fix to understand why the error occurred.
- Identify warning signs: What early indicators might have helped you catch this issue sooner?
- Extract principles: What broader lesson can you apply to future situations?
Here’s a template you might use:
## Error Post-Mortem
**Problem Description:**
[Describe the bug or failed approach]
**Root Cause Analysis:**
[Explain why this error occurred at a fundamental level]
**What I Initially Missed:**
[Identify what you overlooked or misunderstood]
**Solution Implemented:**
[Describe how you fixed the issue]
**Prevention Strategies:**
[List specific actions to prevent similar errors]
**Broader Principles:**
[Note any general lessons applicable to other situations]
2. Build a Personal Error Catalog
Create a systematic way to document and categorize your mistakes. This could be:
- A dedicated section in your programming notes
- A GitHub repository of common errors and solutions
- A digital journal organized by problem type or programming concept
The act of categorizing errors helps identify patterns in your mistakes. You might discover that you consistently struggle with:
- Edge cases in recursive algorithms
- Off-by-one errors in array manipulations
- Memory management in particular contexts
- Time complexity analysis of nested operations
This awareness allows for targeted improvement in specific areas.
3. Adopt a Growth Mindset Toward Errors
Psychologist Carol Dweck’s research on mindset shows that how we view challenges significantly impacts our ability to learn from them. Cultivate a growth mindset by:
- Reframing errors as data points rather than judgments of ability
- Using language that emphasizes learning over performance (“I haven’t mastered this yet” vs. “I’m bad at this”)
- Celebrating the process of working through difficult problems, not just successful outcomes
- Seeking challenges that stretch your abilities rather than staying in your comfort zone
This mindset shift transforms mistakes from threats to valuable learning opportunities.
4. Practice Deliberate Error Analysis
When solving coding problems, especially when preparing for technical interviews, implement a more structured approach to reviewing incorrect solutions:
- Solve without help first: Try to solve each problem independently before looking at solutions.
- Document your approach: Write down your thought process and solution attempt.
- Compare with correct solutions: Identify gaps in your reasoning or implementation.
- Reattempt from scratch: After understanding the correct approach, try solving the problem again without referring to the solution.
- Teach the solution: Explain the correct approach to someone else (or pretend to), which forces deeper understanding.
This method ensures you’re not just passively consuming solutions but actively engaging with your mistakes.
5. Leverage Social Learning
Learning from mistakes accelerates dramatically when done in a social context:
- Participate in code reviews: Both giving and receiving feedback deepens understanding.
- Join programming communities: Platforms like LeetCode, HackerRank, or specialized Discord servers provide spaces to discuss approaches and errors.
- Find a study partner: Regular sessions with a peer can provide accountability and fresh perspectives.
- Contribute to open source: Having your code reviewed by experienced developers offers invaluable learning opportunities.
The external perspective helps identify blind spots in your thinking that would be difficult to recognize independently.
6. Implement Error-Driven Practice Sessions
Rather than always moving forward to new concepts or problems, occasionally structure your practice around revisiting past mistakes:
- Error replay sessions: Set aside time to reattempt problems you previously struggled with.
- Pattern-based practice: Group similar errors and work through multiple problems that target the same weakness.
- Time-delayed repetition: Return to challenging problems after several weeks to test retention and understanding.
This approach ensures that lessons from mistakes are reinforced and internalized.
Advanced Techniques for Technical Interview Preparation
For those specifically focused on technical interviews at top companies, here are specialized strategies for learning from practice interview mistakes:
Mock Interview Analysis
Mock interviews are invaluable, but their true benefit comes from careful analysis afterward:
- Record your mock interviews (with permission) to review your verbal communication and problem-solving approach.
- Create a feedback template for interviewers to provide structured input on specific aspects of your performance.
- Identify both technical and communication patterns across multiple mock interviews.
Sample feedback template:
## Mock Interview Feedback
**Problem Understanding:**
[How well did the candidate clarify the problem? (1-5)]
**Approach Discussion:**
[Did they explain their thinking before coding? (1-5)]
**Algorithm Efficiency:**
[How optimal was their solution? (1-5)]
**Code Quality:**
[Was the code clean, readable, and correct? (1-5)]
**Edge Case Handling:**
[Did they identify and address edge cases? (1-5)]
**Communication:**
[How clear was their explanation throughout? (1-5)]
**Areas for Improvement:**
[Specific suggestions for growth]
**Strengths:**
[What the candidate did particularly well]
Creating a Personalized Error Pattern Map
As you practice more interview problems, you’ll likely notice recurring patterns in your mistakes. Create a visual map of these patterns to guide your study:
- Categorize errors by problem type (arrays, graphs, dynamic programming, etc.)
- Identify common triggers for each error type
- Connect related errors to see broader patterns
- Prioritize areas with the highest error density for focused practice
This visual representation helps transform isolated mistakes into a coherent learning roadmap.
Implementing a Spaced Repetition System
Spaced repetition is a learning technique that incorporates increasing intervals of time between reviews of previously learned material. For programming concepts and problems:
- After successfully solving a problem that you previously struggled with, schedule reviews at increasing intervals (1 day, 3 days, 1 week, 2 weeks, etc.)
- During each review, attempt to solve the problem from scratch
- If you struggle during a review, reset the interval to a shorter timeframe
This approach ensures that lessons from mistakes are retained long-term rather than quickly forgotten.
Case Study: Transforming a Recursive Solution Error into Deep Learning
Let’s walk through a concrete example of how to apply these principles to a common coding mistake.
The Problem: Implement a function to find the maximum depth of a binary tree.
Initial Incorrect Approach:
function maxDepth(root) {
if (!root) {
return 0;
}
return 1 + Math.max(maxDepth(root.left), maxDepth(root.right));
}
This solution seems correct but fails on certain edge cases. Let’s apply our learning framework:
1. Error Documentation
## Error Analysis: Binary Tree Max Depth
**Problem:** Find maximum depth of binary tree
**Failed Test Case:** Empty tree (null input)
**Expected Output:** 0
**Actual Output:** TypeError: Cannot read property 'left' of null
2. Root Cause Analysis
The error occurs because we’re not properly handling the case where the root is null. Our base case should catch this, but there might be an issue with how we’re implementing it.
Upon closer inspection, the base case is correct, but the error must be occurring elsewhere. After further investigation, we realize the issue is with how we’re calling the function recursively without checking if root.left
or root.right
exist.
3. Corrected Solution
function maxDepth(root) {
// Base case: empty tree
if (!root) {
return 0;
}
// Recursive case
const leftDepth = root.left ? maxDepth(root.left) : 0;
const rightDepth = root.right ? maxDepth(root.right) : 0;
return 1 + Math.max(leftDepth, rightDepth);
}
4. Extracting Broader Principles
From this specific error, we can extract several valuable lessons:
- Null checking in recursion: Always verify that child nodes exist before recursive calls.
- Edge case identification: Consider empty structures, single nodes, and unbalanced trees when working with tree problems.
- Recursive function safety: Structure recursive functions to avoid null pointer exceptions by checking conditions before making recursive calls.
5. Creating Prevention Strategies
To prevent similar errors in the future:
- Create a mental checklist for tree problems that includes verifying null handling at each level
- Implement test cases for empty structures and single-node structures before writing the solution
- When using recursion, explicitly map out the call stack for simple examples to verify base cases are correctly handled
6. Application to Similar Problems
This learning can be applied to other tree traversal problems like:
- Finding the minimum depth of a binary tree
- Determining if a binary tree is balanced
- Counting the number of nodes in a binary tree
By thoroughly analyzing this single error, we’ve generated insights that apply to an entire category of problems.
Building Systems for Continuous Learning
Individual strategies for learning from mistakes are powerful, but creating systems that support continuous learning yields even greater results.
Integrating Error Analysis into Your Development Workflow
Make learning from mistakes a formal part of your programming routine:
- Schedule regular review sessions: Set aside time weekly to review recent errors and extract lessons.
- Create templates for bug documentation: Standardize how you record and analyze mistakes for consistency.
- Implement checklists: Develop pre-submission checklists based on common errors to catch mistakes before they happen.
By systematizing the process, learning from mistakes becomes a habit rather than an occasional practice.
Leveraging Technology for Error Tracking
Various tools can support your learning process:
- Spaced repetition software like Anki for reviewing programming concepts you’ve struggled with
- Error journals in notion, obsidian, or specialized programming notebooks
- GitHub issue templates for personal projects that include sections for error analysis
- IDE extensions that highlight common mistake patterns specific to your history
Creating Feedback Loops with Mentors and Peers
Structured feedback accelerates learning:
- Establish regular code review sessions with peers or mentors
- Join or create a study group focused on interviewing for top tech companies
- Participate in pair programming sessions where you can verbalize your thinking
- Teach concepts you’ve struggled with to others, which forces deeper understanding
Measuring Progress in Learning from Mistakes
How do you know if you’re getting better at learning from your coding errors? Here are some metrics to track:
Quantitative Indicators
- Error recurrence rate: How often you make the same mistake multiple times
- Time to resolution: How quickly you can identify and fix bugs
- Breadth of understanding: Number of different problem types you can solve confidently
- Technical interview pass rate: If preparing for interviews, track your success rate over time
Qualitative Indicators
- Confidence in approaching new problems: Do you feel better equipped to tackle unfamiliar challenges?
- Reduced emotional reactivity: Are you less frustrated when encountering errors?
- Improved ability to explain solutions: Can you clearly articulate why a particular approach works?
- Recognition of patterns: Do you more quickly identify similarities between new problems and ones you’ve solved before?
Conclusion: From Error Avoidance to Error Embracement
The journey from struggling with mistakes to learning effectively from them represents a fundamental shift in how you approach programming and technical skill development. It’s a transition from seeing errors as failures to recognizing them as essential components of growth.
By implementing the strategies outlined in this guide, you can transform your relationship with coding mistakes from one of frustration and avoidance to one of curiosity and growth. This shift not only accelerates your technical development but also builds resilience that serves you throughout your programming career.
Remember that learning to learn from mistakes is itself a skill that improves with practice. Each error you encounter is not just an opportunity to fix a specific issue but to refine your entire approach to problem-solving and skill development.
As you prepare for technical interviews or work on complex programming projects, your ability to extract maximum value from each mistake will increasingly set you apart from other developers who remain trapped in patterns of error avoidance and missed learning opportunities.
The most successful programmers aren’t those who make the fewest mistakes—they’re the ones who learn the most from each error they encounter.