Why You Can’t Explain Your Problem Solving Approach (And How to Fix It)
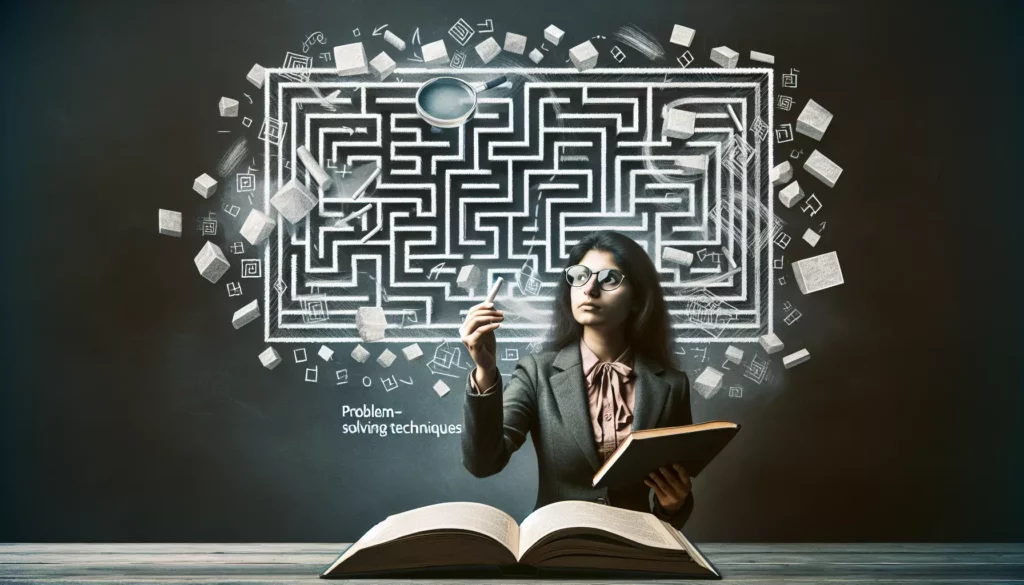
Have you ever solved a coding problem, felt that rush of satisfaction, and then completely fumbled when asked to explain your approach? Or perhaps you’ve aced a technical assessment only to freeze during the follow-up interview when the interviewer asks, “Can you walk me through your solution?”
You’re not alone. Many programmers, from beginners to experienced developers, struggle to articulate their problem solving process, even when they can code effective solutions. This disconnect between doing and explaining is more than just an interview hurdle—it reflects a gap in your understanding that can limit your growth as a programmer.
In this article, we’ll explore why explaining your problem solving approach is challenging, why it matters tremendously for your career, and practical strategies to transform how you think about and communicate your coding solutions.
Table of Contents
- Why Being Able to Explain Your Approach Matters
- 5 Reasons You Struggle to Explain Your Problem Solving Process
- The Intuition Gap: When You “Just Know” But Can’t Explain Why
- Building Your Technical Vocabulary
- Developing a Structured Problem Solving Framework
- Practice Techniques to Improve Your Explanation Skills
- Mastering Explanations in Interview Contexts
- How Teaching Others Enhances Your Explanation Abilities
- Case Studies: Before and After Explanation Transformations
- Conclusion: Becoming a Thoughtful Communicator and Programmer
Why Being Able to Explain Your Approach Matters
Before diving into the why and how, let’s address a fundamental question: Does it really matter if you can explain your solution, as long as the code works?
The answer is an emphatic yes, for several compelling reasons:
Technical Interviews Demand It
The ability to clearly communicate your thought process is often weighted as heavily as the solution itself in technical interviews. Companies like Google, Amazon, and Microsoft don’t just want to see if you can solve problems—they want to understand how you approach them. This reveals your analytical thinking, problem-solving methodology, and ability to work through challenges systematically.
Team Collaboration Requires It
In professional settings, code reviews, pair programming, and architectural discussions all rely on your ability to explain your approach. When you can clearly articulate why you chose a particular solution, you facilitate better collaboration, knowledge sharing, and ultimately, better code.
It Reveals Knowledge Gaps
The famous physicist Richard Feynman once said, “If you can’t explain something in simple terms, you don’t understand it.” When you struggle to explain your solution, it often indicates areas where your understanding is intuitive rather than explicit—pointing to opportunities for deeper learning.
It Makes You a Better Problem Solver
The process of verbalizing your approach forces you to organize your thoughts, identify assumptions, and recognize patterns in your thinking. This metacognitive skill enhances your problem-solving abilities over time.
5 Reasons You Struggle to Explain Your Problem Solving Process
Now that we understand why explanation matters, let’s examine why it’s often so difficult:
1. Tacit Knowledge vs. Explicit Knowledge
Much of programming expertise develops as tacit knowledge—things you know how to do but struggle to articulate. This is similar to riding a bicycle; you can do it, but explaining exactly how you balance would be challenging. Your problem solving approach often includes intuitive leaps and pattern recognition that happen below the level of conscious thought.
2. Backward Reasoning After the Fact
When asked to explain your solution, you often engage in backward reasoning—trying to rationalize decisions you made intuitively. This creates a disjointed narrative that doesn’t accurately reflect your actual problem solving process.
3. Vocabulary Limitations
Programming requires a precise technical vocabulary to discuss algorithms, data structures, and design patterns. Without this vocabulary, you might understand concepts implicitly but lack the language to express them clearly.
4. Nonlinear Problem Solving
Actual problem solving rarely follows a clean, linear path. You may have tried multiple approaches, hit dead ends, or made intuitive leaps. Condensing this messy reality into a coherent explanation is challenging.
5. Anxiety and Performance Pressure
Particularly in interview settings, anxiety can impair your ability to organize thoughts and articulate your process clearly. The pressure to sound intelligent can paradoxically make your explanations more convoluted.
The Intuition Gap: When You “Just Know” But Can’t Explain Why
One of the most common explanatory roadblocks is what we might call the “intuition gap”—that moment when you know a solution is correct but can’t articulate why.
Consider this example: You might immediately recognize that a problem requires a hash map for O(1) lookups, but if asked “Why did you choose a hash map here?” you might struggle beyond saying “It’s faster” or “It seemed appropriate.”
This intuition gap stems from how our brains develop expertise. As you gain experience, your brain forms neural patterns that allow you to recognize solutions without consciously working through every step. This is called pattern recognition, and it’s a hallmark of expertise in any field.
While this intuitive problem solving is efficient, it creates a communication problem. The very mental shortcuts that make you a good programmer can make you a poor explainer.
To bridge this gap, try these approaches:
Reverse Engineer Your Intuition
When you have an intuitive solution, take time to ask yourself: “Why do I think this is right?” Force yourself to articulate the underlying principles that guided your choice.
Compare Alternatives
One powerful way to explain why your solution works is to contrast it with alternatives. “I chose a hash map instead of an array because lookups would be O(n) with an array but O(1) with a hash map, which matters for this input size.”
Connect to First Principles
Trace your solution back to fundamental computing concepts. For example, explain how your approach optimizes for time complexity, space complexity, or readability.
Building Your Technical Vocabulary
A rich technical vocabulary is the foundation of clear explanation. Without the right words, you’ll find yourself using vague descriptions and imprecise analogies.
Here are key vocabulary domains to develop:
Algorithm Pattern Terminology
Familiarize yourself with names for common algorithmic patterns:
- Two-pointer technique
- Sliding window
- Breadth-first search / Depth-first search
- Divide and conquer
- Dynamic programming
- Greedy algorithms
Being able to say “I used a sliding window approach because…” immediately communicates a wealth of information to a technical listener.
Computational Complexity Vocabulary
Practice discussing your solutions in terms of:
- Time complexity (Big O notation)
- Space complexity
- Best, worst, and average case scenarios
- Amortized analysis
Data Structure Properties
Be comfortable explaining why certain data structures are appropriate:
- Access patterns (random vs. sequential)
- Insertion/deletion characteristics
- Memory overhead
- Ordering properties
Code Organization Concepts
Develop vocabulary around:
- Design patterns
- Modularity
- Coupling and cohesion
- Abstraction layers
A practical way to build this vocabulary is to read explanations from experts. When studying algorithm solutions, pay attention not just to the code but to how authors explain their approaches. Technical blogs, algorithm textbooks, and solution walkthroughs are excellent resources.
Developing a Structured Problem Solving Framework
One of the most effective ways to improve your explanations is to adopt a consistent problem solving framework. This gives you a mental template to organize both your approach and your explanation.
Here’s a framework that works well for most algorithmic problems:
1. Problem Understanding
Begin by restating the problem and clarifying requirements:
- What are the inputs and their constraints?
- What is the expected output?
- Are there any edge cases to consider?
- What are the performance requirements?
2. Approach Exploration
Discuss potential approaches and their tradeoffs:
- What are the naive or brute force solutions?
- What optimizations are possible?
- What data structures might be helpful?
- Are there similar problems you’ve solved before?
3. Solution Design
Outline your chosen approach before coding:
- The high-level algorithm steps
- Key data structures and why they’re appropriate
- Expected time and space complexity
4. Implementation Details
Explain key implementation decisions:
- How you’re handling edge cases
- Any optimizations in your code
- Potential areas for improvement
5. Verification Strategy
Discuss how you validate your solution:
- Test cases you’d use
- Potential bugs to watch for
- Performance analysis
Here’s how this might look in practice for a simple problem like finding a pair of numbers in an array that sum to a target value:
Problem Understanding:
“We need to find two numbers in the array that add up to the target sum. The input is an array of integers and a target integer. We need to return the indices of the two numbers.”
Approach Exploration:
“The naive approach would be to use nested loops to check every pair, which would be O(n²). A more efficient approach would use a hash map to store values we’ve seen, allowing us to find complements in O(1) time.”
Solution Design:
“I’ll use a single pass with a hash map. As I iterate through the array, I’ll check if the complement (target – current number) exists in the hash map. If it does, I’ve found the pair. If not, I’ll add the current number to the hash map.”
Implementation Details:
“The hash map will store each number as a key and its index as the value. This approach has O(n) time complexity since we only need one pass through the array, and O(n) space complexity for the hash map.”
Verification Strategy:
“I’d test with various cases: an array with a valid pair, an array with multiple valid pairs, an array with no valid pairs, and edge cases like empty arrays or arrays with duplicate elements.”
Using a consistent framework like this helps organize your thoughts and ensures you cover all the important aspects of your solution.
Practice Techniques to Improve Your Explanation Skills
Like any skill, explaining your problem solving approach improves with deliberate practice. Here are effective techniques to sharpen this ability:
The Rubber Duck Method
Rubber duck debugging is a method where you explain your code line-by-line to an inanimate object (traditionally a rubber duck). This forces you to articulate your thought process without the pressure of a human audience.
Try this: After solving a problem, explain your solution to a rubber duck (or any object) as if teaching it to a junior developer. Speak aloud, even if it feels awkward. You’ll often find that verbalization reveals gaps in your understanding or opportunities for improvement.
Record and Review
Use your smartphone to record yourself explaining a solution, then listen back critically:
- Do you use vague terms like “basically” or “just” too often?
- Are there long pauses that indicate conceptual gaps?
- Does your explanation follow a logical structure?
- Would someone new to the problem understand your approach?
Write Before You Speak
Writing forces clearer thinking than speaking. After solving a problem:
- Write a paragraph explaining your approach
- Identify any unclear portions
- Revise until the explanation is concise and complete
- Then try explaining verbally, using your written explanation as a guide
The Five-Year-Old Test
Challenge yourself to explain your solution using vocabulary a five-year-old could understand. This forces you to distill complex concepts to their essence, which actually demonstrates deeper understanding. Once you can explain simply, you can layer in technical vocabulary as needed.
Pair Programming with Explanation Focus
When pair programming, take turns having one person code while the other narrates what they’re doing and why. The narrator should explain:
- What problem they’re solving at each step
- Why they chose a particular approach
- What alternatives they considered
- What they expect to happen next
This real-time narration builds your explanation muscles in a practical context.
Mastering Explanations in Interview Contexts
Technical interviews present unique challenges for explanation. You’re under time pressure, being evaluated, and often need to code and explain simultaneously.
Pre-Interview Preparation
Before interviews, practice explaining common algorithms you might be asked about:
- Binary search
- Graph traversal algorithms (BFS/DFS)
- Dynamic programming approaches
- Sorting algorithms
For each, prepare a 30-second explanation of:
- What problem it solves
- How it works at a high level
- Its time and space complexity
- When you would use it versus alternatives
Think Aloud Protocol
Interviewers want to see your thought process, not just your final solution. The “think aloud protocol” involves verbalizing your thoughts as you work:
“I’m thinking about this problem as a graph traversal issue. First, I need to determine if I should use BFS or DFS. Since we’re looking for the shortest path, BFS makes more sense because…”
This gives the interviewer insight into your reasoning and allows them to provide hints if you’re heading in the wrong direction.
Structured Answer Format
For interview explanations, use this structure:
- Restate the problem to confirm understanding
- Outline your approach before coding
- Explain key decisions as you implement
- Analyze the solution after completion
Handling “I Don’t Know” Moments
If you can’t explain a part of your solution, don’t fake it. Instead:
“I’m not entirely sure why this works in all cases. My intuition is that [explain your best guess], but I’d want to verify that with further analysis. Here’s how I would test it to confirm…”
This shows intellectual honesty and a methodical approach to uncertainty—both valuable traits to interviewers.
How Teaching Others Enhances Your Explanation Abilities
One of the most powerful ways to improve your explanation skills is to teach others. The act of teaching forces you to organize knowledge in accessible ways and anticipate questions and misconceptions.
Formal Teaching Opportunities
Consider these formal teaching opportunities:
- Lead a study group where you explain algorithms to peers
- Volunteer as a mentor for junior developers or coding bootcamp students
- Create tutorial content on a blog or video platform
- Give presentations at meetups or within your company
The Teaching Feedback Loop
Teaching creates a valuable feedback loop:
- You explain a concept
- Learners ask questions that reveal gaps in your explanation
- You refine your understanding to address these gaps
- Your explanation improves for future teaching
Pay particular attention to questions that begin with “But why…” or “What if…” These often point to areas where your explanation lacks depth or nuance.
Creating Teaching Materials
The process of creating teaching materials—slides, code examples, diagrams—forces you to distill concepts to their essence. Ask yourself:
- What is the most important thing learners should understand?
- What analogies would make this concept more accessible?
- How can I visualize this algorithm or data structure?
- What misconceptions might learners have?
This preparation process dramatically improves your ability to explain concepts clearly.
Case Studies: Before and After Explanation Transformations
Let’s examine how explanation skills can transform with practice through two case studies:
Case Study 1: Binary Search Explanation
Before:
“Binary search is when you divide the array in half repeatedly. You check the middle and see if your target is greater or less than it. Then you keep dividing until you find it. It’s O(log n) which is better than linear search.”
After:
“Binary search is an efficient algorithm for finding a target value in a sorted array. Here’s how it works: we maintain a search range, initially the entire array. In each step, we compare the middle element with our target. If they match, we’re done. If the target is smaller, we narrow our search to the left half; if larger, to the right half. This divides our search space in half each time, giving us O(log n) time complexity.
The key insight is that by requiring a sorted array, we gain the ability to eliminate half the remaining elements with a single comparison. This makes binary search dramatically faster than linear search (O(n)) for large datasets. For example, finding an element in a sorted array of 1 million items would take at most 20 comparisons with binary search, compared to up to 1 million comparisons with linear search.
The implementation needs careful handling of boundary conditions to avoid infinite loops or off-by-one errors, particularly in determining the middle index and updating the search boundaries.”
Case Study 2: Graph Traversal Explanation
Before:
“For this problem I’d use BFS because we need to find the shortest path. I’d add nodes to a queue and keep track of visited nodes. Then process each node, add its neighbors to the queue, and continue until we find the target.”
After:
“This problem involves finding the shortest path in an unweighted graph, which makes Breadth-First Search (BFS) the ideal choice. BFS guarantees that we’ll discover nodes in order of their distance from the starting point.
My approach has three key components:
- Queue for frontier nodes: I’ll use a queue to maintain the nodes we’ve discovered but haven’t processed yet, starting with our source node.
- Visited set: To prevent cycles, I’ll track visited nodes in a hash set, ensuring O(1) lookup time.
- Distance tracking: I’ll maintain a mapping from each node to its distance from the source, which will give us our answer when we reach the target.
The algorithm works by exploring all nodes at the current distance before moving to nodes that are farther away. This “level by level” exploration ensures we find the shortest path.
The time complexity is O(V + E) where V is the number of vertices and E is the number of edges, as we potentially need to visit all nodes and edges. The space complexity is O(V) for the queue, visited set, and distance mapping.
If the graph were weighted, BFS wouldn’t guarantee the shortest path, and we’d need to use Dijkstra’s algorithm instead.”
Notice how the “after” explanations:
- Provide context for why the algorithm is appropriate
- Break down the approach into clear components
- Explain the underlying principles, not just the steps
- Address complexity and tradeoffs
- Consider alternative approaches when relevant
Conclusion: Becoming a Thoughtful Communicator and Programmer
The ability to clearly explain your problem solving approach isn’t just an interview skill—it’s a fundamental aspect of programming mastery. When you can articulate how and why your solutions work, you demonstrate a deeper understanding that makes you a more effective developer, collaborator, and leader.
The journey from “I just coded it” to clear, insightful explanations involves:
- Bridging the gap between intuitive and explicit knowledge
- Building a rich technical vocabulary
- Adopting structured problem solving frameworks
- Practicing explanation techniques deliberately
- Teaching others to solidify your understanding
Remember that explanation skills, like coding skills, improve with practice. Each time you force yourself to articulate your thinking—whether to a rubber duck, a peer, or in an interview—you strengthen the neural pathways that connect your problem solving abilities with your communication abilities.
As you develop these skills, you’ll likely notice benefits beyond just better interview performance. Your code reviews will become more insightful, your architectural discussions more productive, and your mentoring more impactful. You may even find that problems that once seemed impenetrably complex become more approachable as you develop the habit of clear explanation.
The next time you solve a programming problem, challenge yourself to explain it as if teaching someone else. Your future self—especially during your next technical interview—will thank you.