Why You Can’t Optimize Your Initial Solutions in Coding Problems
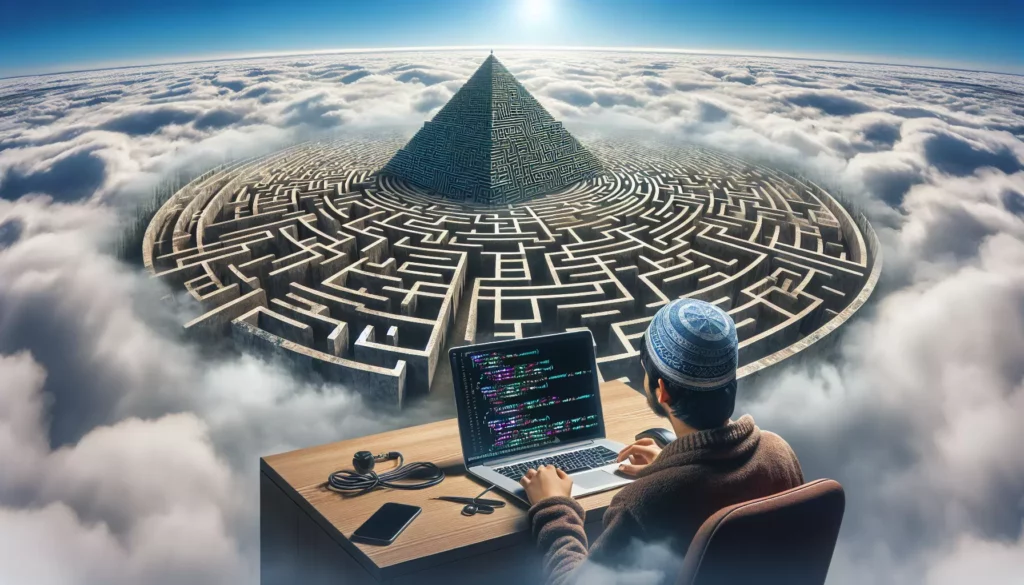
When tackling coding problems, particularly in technical interviews or competitive programming, there’s a common trap that even experienced developers fall into: attempting to optimize solutions prematurely. This approach, while well intentioned, often leads to unnecessary complexity, wasted time, and even failure to solve the problem at all. In this article, we’ll explore why you should resist the urge to optimize your initial solutions and instead follow a more methodical approach to problem solving.
The Premature Optimization Trap
Donald Knuth, the legendary computer scientist, famously stated, “Premature optimization is the root of all evil.” This quote has become a mantra in software development for good reason. When facing a coding challenge, the desire to immediately produce the most efficient solution possible can actually hinder your progress.
Here’s what typically happens when someone tries to optimize too early:
- They get lost in implementation details before understanding the problem fully
- They overcomplicate the solution, making it harder to debug
- They waste precious time optimizing parts of the code that might not need it
- They might miss simpler approaches that would solve the problem adequately
This is especially problematic in high pressure situations like technical interviews, where time constraints make this approach particularly risky.
The Brute Force First Approach
Instead of aiming for the optimal solution right away, experienced problem solvers follow a different strategy: start with the simplest working solution, then refine it step by step. This approach has several names in the programming community: “make it work, make it right, make it fast,” “brute force first,” or simply “incremental refinement.”
Why Brute Force Solutions Matter
A brute force solution is one that solves the problem correctly, but not necessarily efficiently. It might use more time or space than optimal, but it works. Here’s why starting with brute force is valuable:
- Problem understanding: Implementing a simple solution forces you to understand all aspects of the problem
- Verification tool: Your brute force solution can serve as a reference to verify optimized solutions
- Fallback option: Having a working solution, even if inefficient, is better than having no solution
- Starting point: It provides a baseline from which you can identify bottlenecks for optimization
Real World Example: Two Sum Problem
Let’s consider a classic coding interview problem to illustrate this approach:
Given an array of integers and a target sum, return the indices of two numbers that add up to the target.
The Brute Force Approach
The simplest solution involves checking all possible pairs:
def two_sum_brute_force(nums, target):
for i in range(len(nums)):
for j in range(i + 1, len(nums)):
if nums[i] + nums[j] == target:
return [i, j]
return [] # No solution found
This solution has O(n²) time complexity, which isn’t ideal for large arrays. However, it’s:
- Easy to understand
- Guaranteed to be correct (if a solution exists)
- Quick to implement
The Optimized Approach
After implementing the brute force solution, you can think about optimization. The key insight is that for each number, we’re looking for its “complement” (target – current number). We can use a hash map to store numbers we’ve seen:
def two_sum_optimized(nums, target):
num_map = {} # Value -> Index
for i, num in enumerate(nums):
complement = target - num
if complement in num_map:
return [num_map[complement], i]
num_map[num] = i
return [] # No solution found
This optimized solution has O(n) time complexity, a significant improvement over the brute force approach.
The Progression Matters
Notice the progression: we started with a simple, correct solution, understood the problem deeply, and then identified a more efficient approach. If we had tried to jump straight to the optimized solution, we might have missed edge cases or introduced bugs in our rush to be clever.
The Step by Step Problem Solving Framework
Based on this understanding, here’s a framework for approaching coding problems that maximizes your chances of success:
1. Understand the Problem Thoroughly
Before writing any code, make sure you understand:
- The input format and constraints
- The expected output format
- Edge cases and special conditions
- Any implicit requirements or assumptions
Ask clarifying questions if anything is unclear. In an interview setting, this demonstrates thoughtfulness and attention to detail.
2. Devise a Simple Solution First
Implement the most straightforward solution that correctly solves the problem, even if it’s not efficient. This accomplishes several things:
- Demonstrates that you can solve the problem
- Gives you a working implementation to build upon
- Shows your interviewer your thought process
- Provides a safety net in case you run out of time
3. Analyze the Brute Force Solution
Once you have a working solution, analyze its time and space complexity:
- Identify the bottlenecks in your algorithm
- Understand which operations are most expensive
- Consider if the complexity is acceptable for the given constraints
4. Optimize Incrementally
With a clear understanding of the bottlenecks, look for optimizations:
- Can you use a more efficient data structure?
- Are there mathematical properties you can exploit?
- Can you eliminate redundant calculations?
- Is there a well known algorithm that applies to this problem?
5. Implement the Optimized Solution
Once you have a clear optimization strategy, implement it step by step. Compare with your brute force solution to ensure correctness.
6. Test Thoroughly
Test your solution with various inputs, including:
- Normal cases
- Edge cases (empty inputs, single elements, etc.)
- Large inputs (to verify efficiency)
- Inputs that might cause special handling
Case Studies: The Value of Starting Simple
Let’s examine a few more examples to reinforce the importance of starting with brute force solutions.
Merge Intervals Problem
Consider this problem: Given an array of intervals where intervals[i] = [starti, endi], merge all overlapping intervals and return the non overlapping intervals.
Brute Force Approach
def merge_intervals_brute_force(intervals):
if not intervals:
return []
# Sort intervals by start time
intervals.sort(key=lambda x: x[0])
result = [intervals[0]]
for i in range(1, len(intervals)):
# Check if current interval overlaps with the last result interval
last_end = result[-1][1]
current_start = intervals[i][0]
current_end = intervals[i][1]
if current_start <= last_end: # Overlapping intervals
# Merge by updating the end time of the last interval in result
result[-1][1] = max(last_end, current_end)
else: # Non-overlapping interval
result.append(intervals[i])
return result
This solution has O(n log n) time complexity due to the sorting, plus O(n) for the merging process.
In this case, the “brute force” solution is actually quite efficient. This illustrates an important point: sometimes your initial solution might already be optimal or near optimal. By starting simple, you can recognize when further optimization isn’t necessary.
Finding the Kth Largest Element
Let’s look at another example: Find the kth largest element in an unsorted array.
Brute Force Approach
def find_kth_largest_brute_force(nums, k):
# Sort the array in descending order
nums.sort(reverse=True)
# Return the kth element (0-indexed)
return nums[k-1]
This solution has O(n log n) time complexity due to sorting.
Optimized Approach
After implementing the brute force solution, you might realize that you don’t need to sort the entire array. You can use a selection algorithm like QuickSelect for a more efficient solution:
import random
def find_kth_largest_optimized(nums, k):
# Convert to 0-indexed for convenience
k = len(nums) - k
def quick_select(left, right):
if left == right:
return nums[left]
# Choose a random pivot
pivot_index = random.randint(left, right)
pivot = nums[pivot_index]
# Move pivot to the end
nums[pivot_index], nums[right] = nums[right], nums[pivot_index]
# Partition the array
store_index = left
for i in range(left, right):
if nums[i] <= pivot:
nums[store_index], nums[i] = nums[i], nums[store_index]
store_index += 1
# Move pivot to its final place
nums[right], nums[store_index] = nums[store_index], nums[right]
# Return the pivot if it's the kth element
# Otherwise, recurse on the relevant portion of the array
if k == store_index:
return nums[k]
elif k < store_index:
return quick_select(left, store_index - 1)
else:
return quick_select(store_index + 1, right)
return quick_select(0, len(nums) - 1)
This optimized solution has an average time complexity of O(n), which is better than the O(n log n) of the sorting approach.
Common Optimization Techniques
Once you have a working brute force solution, there are several common techniques you can apply to optimize it:
1. Space-Time Tradeoffs
One of the most common optimization strategies is trading space for time. By using additional memory (like hash maps, sets, or arrays), you can often drastically reduce time complexity.
Example: Finding Duplicates
def find_duplicate_brute_force(nums):
for i in range(len(nums)):
for j in range(i + 1, len(nums)):
if nums[i] == nums[j]:
return nums[i]
return -1
This has O(n²) time complexity. Using a hash set trades space for time:
def find_duplicate_optimized(nums):
seen = set()
for num in nums:
if num in seen:
return num
seen.add(num)
return -1
The optimized solution has O(n) time complexity but uses O(n) extra space.
2. Preprocessing
Sometimes, preprocessing the input data can lead to more efficient solutions, especially when you need to perform multiple operations on the same data.
Example: Range Sum Queries
def range_sum_brute_force(nums, queries):
results = []
for start, end in queries:
total = sum(nums[start:end+1])
results.append(total)
return results
This has O(n * q) time complexity where q is the number of queries. Using prefix sums:
def range_sum_optimized(nums, queries):
# Precompute prefix sums
prefix = [0]
for num in nums:
prefix.append(prefix[-1] + num)
results = []
for start, end in queries:
# Sum from start to end = prefix[end+1] - prefix[start]
total = prefix[end+1] - prefix[start]
results.append(total)
return results
The optimized solution has O(n + q) time complexity, which is much better when there are many queries.
3. Dynamic Programming
For problems with overlapping subproblems and optimal substructure, dynamic programming can transform exponential solutions into polynomial ones.
Example: Fibonacci Numbers
def fibonacci_recursive(n):
if n <= 1:
return n
return fibonacci_recursive(n-1) + fibonacci_recursive(n-2)
This has O(2ⁿ) time complexity. Using dynamic programming:
def fibonacci_dp(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
The dynamic programming solution has O(n) time complexity.
4. Binary Search and Divide and Conquer
When working with sorted data or problems that can be divided into subproblems, these techniques can significantly reduce time complexity.
Example: Finding an Element in a Sorted Array
def linear_search(nums, target):
for i, num in enumerate(nums):
if num == target:
return i
return -1
This has O(n) time complexity. Using binary search:
def binary_search(nums, target):
left, right = 0, len(nums) - 1
while left <= right:
mid = (left + right) // 2
if nums[mid] == target:
return mid
elif nums[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
The binary search solution has O(log n) time complexity.
When to Skip the Brute Force Approach
While the “brute force first” approach is generally sound, there are scenarios where you might want to skip directly to a more optimized solution:
1. When the Brute Force Solution is Impractical
For some problems, the brute force solution might be so inefficient that implementing it is impractical. For example, if a problem has a clear exponential or factorial time complexity in its naive approach, and the input size is large, you might need to think of a more efficient algorithm right away.
2. When You Immediately Recognize a Standard Pattern
If you immediately recognize that a problem is a standard one with a well known efficient solution, you might choose to implement the optimized solution directly. For example, if you recognize a problem as a classic graph traversal, shortest path, or dynamic programming problem, you might apply the standard algorithm directly.
3. In Time Sensitive Competitive Programming
In competitive programming where time is extremely limited, experienced competitors might skip the brute force implementation if they’re confident in their optimized solution. However, this requires significant experience and pattern recognition skills.
The Psychological Benefits of Starting Simple
Beyond the technical advantages, there are important psychological benefits to starting with simpler solutions:
Reducing Anxiety and Pressure
Technical interviews and coding challenges can be stressful. By giving yourself permission to start with a simple solution, you reduce the anxiety of having to produce the perfect algorithm immediately. This can help you think more clearly and perform better.
Building Confidence Through Incremental Progress
Each step in the process—understanding the problem, implementing a basic solution, analyzing it, and then optimizing—represents a small win. These incremental successes build confidence and momentum, which is especially valuable in high pressure situations.
Demonstrating Methodical Thinking
In interview settings, showing your step by step approach demonstrates that you’re a methodical thinker who can break down complex problems. This is often more valuable to employers than someone who might occasionally produce brilliant solutions but can’t explain their thought process.
Common Pitfalls to Avoid
Even when following the “brute force first” approach, there are common pitfalls to watch out for:
Getting Stuck in Optimization
Sometimes, developers get so focused on optimizing their solution that they lose sight of the original problem or time constraints. Remember that a working, less efficient solution is better than an incomplete optimization attempt, especially in time limited situations.
Overcomplicating the Initial Solution
The point of the brute force solution is that it should be simple and straightforward. If your “brute force” approach is already complex, you might be overcomplicating the problem. Step back and look for an even simpler approach.
Skipping Analysis
Before jumping to optimization, make sure you’ve properly analyzed your brute force solution to identify the true bottlenecks. Optimizing the wrong parts of your algorithm is a waste of effort.
Ignoring Problem Constraints
Always consider the constraints of the problem. Sometimes, a brute force solution might be perfectly acceptable if the input size is guaranteed to be small. Don’t optimize unless it’s necessary based on the problem constraints.
Teaching and Learning with This Approach
The “brute force first” approach isn’t just effective for solving problems—it’s also an excellent teaching and learning methodology.
For Educators and Mentors
When teaching algorithms and problem solving:
- Start by presenting the simplest solution to build understanding
- Use the brute force solution to illustrate the problem’s core challenges
- Demonstrate how to analyze a solution for bottlenecks
- Show the step by step progression from simple to optimized
This approach helps learners develop a solid foundation before tackling more complex concepts.
For Learners
If you’re learning algorithms and problem solving:
- Don’t be afraid to start with simple, even inefficient solutions
- Focus on correctness before efficiency
- Practice analyzing your own solutions for optimization opportunities
- Learn to recognize common patterns and optimizations over time
This incremental approach builds both confidence and competence.
Conclusion: Embrace the Process
In the world of algorithmic problem solving, the journey from a simple solution to an optimized one is as important as the destination. By resisting the urge to optimize prematurely and instead embracing a methodical approach—understand, implement simply, analyze, then optimize—you’ll not only solve problems more effectively but also grow as a developer.
Remember that even the most elegant algorithms often began as brute force solutions that were gradually refined. The ability to navigate this refinement process is what separates good programmers from great ones.
The next time you face a challenging coding problem, give yourself permission to start simple. Implement a brute force solution, analyze it thoughtfully, and then optimize with purpose. This approach won’t just help you solve the problem at hand—it will make you a better problem solver for all the challenges that lie ahead.
Further Resources
To deepen your understanding of algorithmic problem solving and optimization techniques, consider exploring these resources:
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein
- “The Algorithm Design Manual” by Steven Skiena
- Online platforms like LeetCode, HackerRank, and CodeSignal for practice
- Stanford’s Algorithms specialization on Coursera
Remember that becoming proficient at algorithmic problem solving is a marathon, not a sprint. Consistent practice using a methodical approach will yield better results than trying to memorize solutions or jumping straight to optimizations.