Why You Can’t Identify the Core Problem to Solve
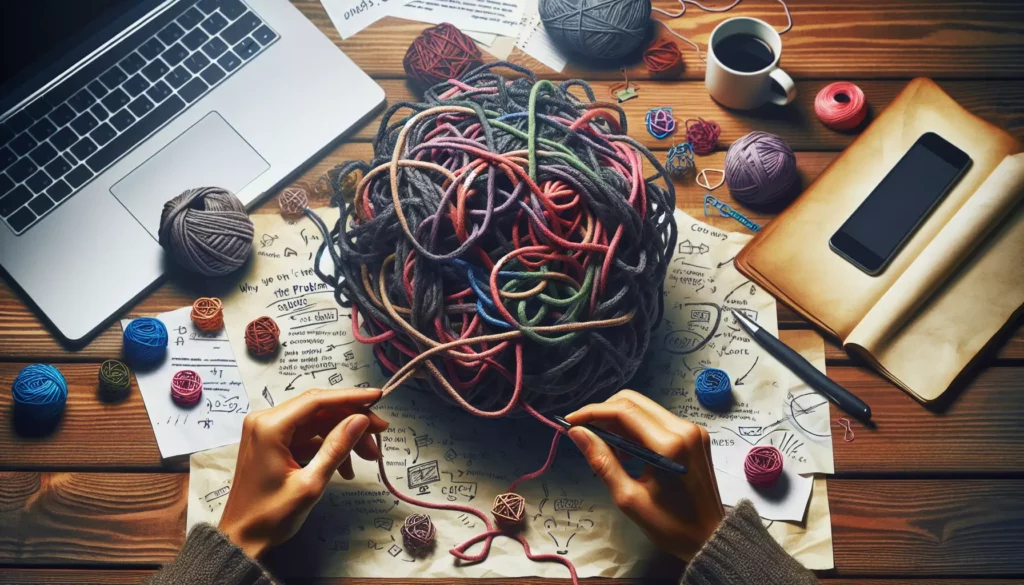
In the world of programming and software development, one of the most valuable skills isn’t actually writing code—it’s identifying the right problem to solve in the first place. Whether you’re a beginner learning your first programming language or an experienced developer preparing for technical interviews at top tech companies, the ability to accurately pinpoint core problems is fundamental to success.
Yet many programmers, regardless of experience level, struggle with this critical skill. They dive into coding solutions before fully understanding what they’re trying to solve, leading to wasted effort, suboptimal solutions, and frustration. This challenge is particularly evident in algorithmic problem solving and technical interviews, where problem identification is often half the battle.
The Problem Identification Crisis in Programming
Think about the last time you faced a coding challenge. Did you immediately start writing code? Did you sketch out several approaches before settling on one? Or did you spend significant time understanding the problem statement itself?
For many programmers, the instinct is to jump straight into solution mode. After all, coding is what we’re trained to do. However, this approach often leads to what experienced developers call “solution jumping”—attempting to solve a problem before fully understanding it.
Common Scenarios Where Problem Identification Fails
- A developer spends hours optimizing an algorithm that addresses the wrong requirement
- A team builds a feature that users don’t actually need or want
- A candidate in a technical interview misunderstands the constraints and delivers an elegant solution to the wrong problem
- A student gets stuck on a coding exercise because they’ve misinterpreted what’s being asked
According to a study by the National Institute of Standards and Technology, requirements errors can cost 10-100 times more to fix after implementation than if caught during the requirements phase. In other words, solving the wrong problem is expensive.
The Psychological Barriers to Problem Identification
Understanding why we struggle to identify core problems requires examining some psychological factors that affect all programmers, from beginners to experts.
Confirmation Bias
Confirmation bias leads us to favor information that confirms our preexisting beliefs. In programming, this manifests as latching onto the first interpretation of a problem that comes to mind, then filtering all subsequent information through that lens.
For example, if you believe a coding problem is about optimizing for speed, you might ignore clues that suggest memory optimization is more important. Your initial assumptions shape how you interpret the entire problem.
The Curse of Knowledge
Once you know something, it’s difficult to imagine not knowing it. This “curse of knowledge” makes it challenging for experienced programmers to approach problems with fresh eyes. They bring assumptions based on past experiences, potentially missing what makes the current problem unique.
This is particularly problematic when mentoring junior developers or explaining solutions to others—what seems obvious to you may be completely opaque to someone else.
Solution Fixation
Many programmers experience “solution fixation”—becoming emotionally attached to their first solution approach. This attachment makes it difficult to abandon an approach even when new information suggests it’s suboptimal.
In technical interviews, candidates often stick with their first approach even when the interviewer provides hints suggesting an alternative direction. This stubbornness stems from a fear of appearing indecisive or wasting the time already invested.
The Role of Problem Framing in Software Development
Problem framing—how a problem is presented and interpreted—significantly influences our ability to solve it. Consider these two descriptions of the same task:
- “Implement a function that finds duplicate elements in an array.”
- “Implement a function that identifies which elements appear more than once in an array.”
While logically equivalent, these descriptions might trigger different mental models and solution approaches. The first might lead you to think about hash tables for tracking occurrences, while the second might suggest a sorting-based approach.
How Problem Framing Affects Solution Quality
Research in cognitive psychology shows that the way problems are framed affects our problem-solving strategies. In programming, poor problem framing can lead to:
- Overly complex solutions to simple problems
- Oversimplified approaches to complex problems
- Solutions that technically work but miss the underlying user need
- Wasted effort optimizing the wrong aspects of an algorithm
The classic example is optimizing code for speed when the actual bottleneck is memory usage, or vice versa. This mismatch occurs when we fail to identify which constraints are most important in the problem context.
Common Problem Identification Pitfalls in Coding Interviews
Technical interviews at major tech companies are as much about problem identification as they are about coding skills. Here are common pitfalls candidates encounter:
Rushing to Code
Many candidates feel pressure to start coding immediately to show productivity. However, interviewers at top companies often value thoughtful problem analysis over quick but misguided implementation. Taking time to understand the problem fully before coding demonstrates maturity and methodical thinking.
Missing Edge Cases
Edge cases are often hints about the true nature of the problem. Missing them suggests you haven’t fully identified the problem space. For instance, if you’re writing a function to find the maximum value in an array, have you considered what should happen with an empty array? The answer reveals whether you’ve truly understood the problem’s boundaries.
Overlooking Constraints
Interview problems often come with explicit or implicit constraints that define the problem:
- Time complexity requirements (e.g., “solve this in O(n) time”)
- Memory limitations (e.g., “use constant extra space”)
- Input restrictions (e.g., “the input array is sorted”)
- Output expectations (e.g., “return the index, not the value”)
Misidentifying or overlooking these constraints leads to solutions that technically work but fail to meet the interview criteria.
Example: The Two Sum Problem
Consider the classic “Two Sum” problem: given an array of integers and a target sum, find the indices of two numbers that add up to the target.
A candidate who rushes might implement a nested loop solution (O(n²) time complexity):
function twoSum(nums, target) {
for (let i = 0; i < nums.length; i++) {
for (let j = i + 1; j < nums.length; j++) {
if (nums[i] + nums[j] === target) {
return [i, j];
}
}
}
return null;
}
But a candidate who carefully identifies the core problem—finding complementary pairs efficiently—might recognize that a hash table provides O(n) time complexity:
function twoSum(nums, target) {
const complements = {};
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
if (complements.hasOwnProperty(complement)) {
return [complements[complement], i];
}
complements[nums[i]] = i;
}
return null;
}
The difference isn’t coding skill but problem identification—recognizing that the core challenge is efficiently finding complements, not checking all possible pairs.
Techniques for Better Problem Identification
Improving your problem identification skills requires deliberate practice. Here are effective techniques to develop this critical ability:
The Five Whys Technique
Originally developed by Toyota for root cause analysis, the Five Whys technique involves asking “why” repeatedly to dig deeper into a problem. For programming problems:
- Why am I being asked to solve this problem? (What’s the purpose?)
- Why is this approach being considered? (What assumptions am I making?)
- Why might this solution fail? (What are the limitations?)
- Why might users struggle with this solution? (What are the usability concerns?)
- Why might this solution be difficult to maintain? (What are the long-term implications?)
This recursive questioning helps reveal hidden aspects of the problem that might otherwise go unnoticed.
Problem Restatement
Restating a problem in your own words forces you to process it more deeply. Try these approaches:
- Explain the problem to someone else (or imagine doing so)
- Rewrite the problem statement in simpler terms
- Draw a diagram representing the problem
- Create examples that illustrate the problem
The act of translation from one format to another highlights misunderstandings and gaps in your comprehension.
Working Backward from Examples
Examples are powerful tools for problem identification. When given examples:
- Trace through them manually to understand the expected transformations
- Create additional examples to test your understanding
- Look for patterns across multiple examples
- Try to generate counterexamples that might break your understanding
This approach is particularly useful for algorithmic problems, where the transformation from input to output contains clues about the underlying logic.
The Rubber Duck Method
The rubber duck debugging method—explaining your code line-by-line to an inanimate object—works equally well for problem identification. By verbalizing the problem to your “rubber duck,” you force yourself to articulate aspects that might remain fuzzy when kept as internal thoughts.
Many programmers report having “aha moments” while explaining problems to others, even when those others don’t contribute to the conversation. The act of explanation itself clarifies thinking.
Systematic Problem Breakdown for Algorithmic Challenges
For algorithmic problems specifically, a systematic breakdown approach can help identify the core challenge:
1. Identify the Input and Output
Start by clearly defining:
- What form does the input take? (data types, structures, sizes)
- What form should the output take?
- Are there any constraints on the input or output?
2. Analyze the Transformation
Consider what needs to happen to transform the input to the output:
- What operations need to be performed?
- What information needs to be tracked?
- Are there patterns in how input maps to output?
3. Identify Efficiency Requirements
Determine what kind of efficiency the problem demands:
- Is time complexity critical?
- Is space complexity a concern?
- Are there specific performance targets mentioned?
4. Consider Edge Cases
Edge cases often reveal the true nature of a problem:
- What happens with empty inputs?
- What about extremely large inputs?
- Are there special cases that need handling?
Example: Finding the Longest Substring Without Repeating Characters
Let’s apply this framework to a classic problem: finding the length of the longest substring without repeating characters.
Input and Output Analysis:
- Input: A string (could be empty, could contain any characters)
- Output: An integer representing the length of the longest substring without repeats
Transformation Analysis:
- We need to identify substrings (contiguous sequences of characters)
- We need to check if characters repeat within each substring
- We need to find the maximum length among valid substrings
Efficiency Requirements:
- No explicit requirements given, but naive O(n³) solution would be too slow for interviews
- Should aim for O(n) time complexity if possible
Edge Cases:
- Empty string (should return 0)
- String with all identical characters (should return 1)
- String with all unique characters (should return the string length)
With this analysis, we can identify that the core problem is efficiently tracking character uniqueness while expanding and contracting a sliding window. This leads to the sliding window solution:
function lengthOfLongestSubstring(s) {
const charMap = new Map();
let maxLength = 0;
let windowStart = 0;
for (let windowEnd = 0; windowEnd < s.length; windowEnd++) {
const currentChar = s[windowEnd];
// If we've seen this character before and it's in our current window
if (charMap.has(currentChar) && charMap.get(currentChar) >= windowStart) {
// Move window start to just after the previous occurrence
windowStart = charMap.get(currentChar) + 1;
}
// Update our map with the current character position
charMap.set(currentChar, windowEnd);
// Update max length if needed
maxLength = Math.max(maxLength, windowEnd - windowStart + 1);
}
return maxLength;
}
By systematically breaking down the problem, we identified that a sliding window approach with character tracking is the optimal solution—a conclusion we might have missed with a less structured analysis.
Real-world Problem Identification Beyond Algorithms
While algorithmic challenges highlight problem identification skills, the same principles apply to everyday software development tasks:
Feature Development
When tasked with building a new feature, the first step should be identifying the core user need, not the implementation details. Ask:
- What problem does this feature solve for users?
- How will users measure success?
- What alternatives have users tried?
- What constraints must the solution work within?
These questions help identify the true problem behind the feature request, which might differ from the stated requirements.
Debugging
Effective debugging starts with problem identification. Instead of randomly changing code to see what works, skilled debuggers:
- Clearly define the expected vs. actual behavior
- Identify patterns in when the bug occurs
- Isolate the minimal reproduction case
- Form and test hypotheses about the root cause
This structured approach prevents the common debugging trap of fixing symptoms rather than root causes.
Code Reviews
When reviewing code, the primary task is identifying potential problems before they reach production. Effective reviewers look for:
- Misalignment between implementation and requirements
- Edge cases that aren’t handled
- Performance implications under different conditions
- Maintainability concerns for future developers
Each of these aspects requires identifying problems that may not be immediately obvious from the code itself.
How Learning Environments Impact Problem Identification Skills
The way programming is taught significantly impacts how developers approach problem identification throughout their careers.
Traditional Coding Education
Many traditional programming courses and bootcamps focus on teaching syntax and implementation details rather than problem-solving strategies. Students learn how to write code but not necessarily how to identify what code to write.
This implementation-first approach can create developers who are technically proficient but struggle with complex, ambiguous problems—precisely the kind encountered in professional environments and technical interviews.
Modern Learning Approaches
More effective learning environments emphasize problem identification as a distinct skill:
- Problem-based learning: Starting with real-world problems rather than programming concepts
- Incremental complexity: Gradually introducing ambiguity into problem statements
- Peer discussion: Encouraging learners to discuss problems before implementing solutions
- Multiple solution exploration: Comparing different approaches to the same problem
These approaches help learners develop the mental models needed for effective problem identification alongside their coding skills.
The Role of Interactive Learning Platforms
Interactive coding platforms like AlgoCademy are changing how problem identification is taught. By providing immediate feedback, guided hints, and multiple problem representations, these platforms help learners develop stronger problem identification skills.
Features that specifically enhance problem identification include:
- Interactive examples that learners can manipulate
- Visualization tools that make abstract concepts concrete
- Step-by-step guidance that models expert problem breakdown
- AI-powered assistance that adapts to individual learning patterns
These tools help bridge the gap between knowing how to code and knowing what to code—a distinction that becomes increasingly important as developers progress in their careers.
Developing a Problem Identification Mindset
Becoming skilled at problem identification requires more than techniques; it requires developing a particular mindset:
Embrace Uncertainty
Problems worth solving are rarely perfectly defined. Comfort with ambiguity and willingness to explore undefined spaces are hallmarks of strong problem identifiers. Practice:
- Working with incomplete information
- Asking clarifying questions
- Making and testing assumptions
- Revising your understanding as new information emerges
Cultivate Curiosity
Curiosity drives deeper problem exploration. Cultivate it by:
- Asking “why” more often than “how”
- Exploring problems from multiple perspectives
- Reading widely across disciplines
- Questioning assumptions, especially your own
Practice Metacognition
Metacognition—thinking about your thinking—helps identify flaws in your problem-solving approach. Develop this skill by:
- Journaling about your problem-solving process
- Reviewing past solutions with fresh eyes
- Analyzing where and why you get stuck
- Comparing your approach with others’ approaches
Build a Problem Pattern Library
Experienced problem solvers recognize patterns across seemingly different problems. Build your pattern recognition by:
- Studying classic problem-solving paradigms (divide and conquer, dynamic programming, etc.)
- Categorizing problems you encounter
- Looking for similarities between new problems and ones you’ve solved
- Abstracting specific problems into general patterns
Conclusion: The Path to Better Problem Identification
The ability to identify core problems is what separates exceptional programmers from merely competent ones. It’s the foundation upon which all technical skills build, yet it often receives less explicit attention than coding techniques or language features.
Improving this skill requires deliberate practice and a shift in mindset—from viewing programming as implementation to seeing it as problem exploration. This shift pays dividends not just in technical interviews but throughout your programming career, as it transforms how you approach everything from debugging to system design.
The next time you face a programming challenge, resist the urge to dive straight into coding. Instead, invest time in truly understanding the problem. Ask questions, restate the problem in your own words, explore examples, and consider alternative framings. This upfront investment will save hours of implementation time and lead to more elegant, effective solutions.
Remember that identifying the right problem is often more than half the solution. As Einstein reportedly said, “If I had an hour to solve a problem, I’d spend 55 minutes thinking about the problem and 5 minutes thinking about solutions.” While software development rarely affords such luxury, the principle remains sound—the clearer your problem identification, the more direct your path to an effective solution.
By developing your problem identification skills, you’ll not only perform better in technical interviews and algorithmic challenges but also become a more valuable contributor to any software team. After all, solving the wrong problem perfectly is still failure; solving the right problem adequately is success.