Why You Can’t Create Your Own Test Cases: The Hidden Pitfalls in Coding Education
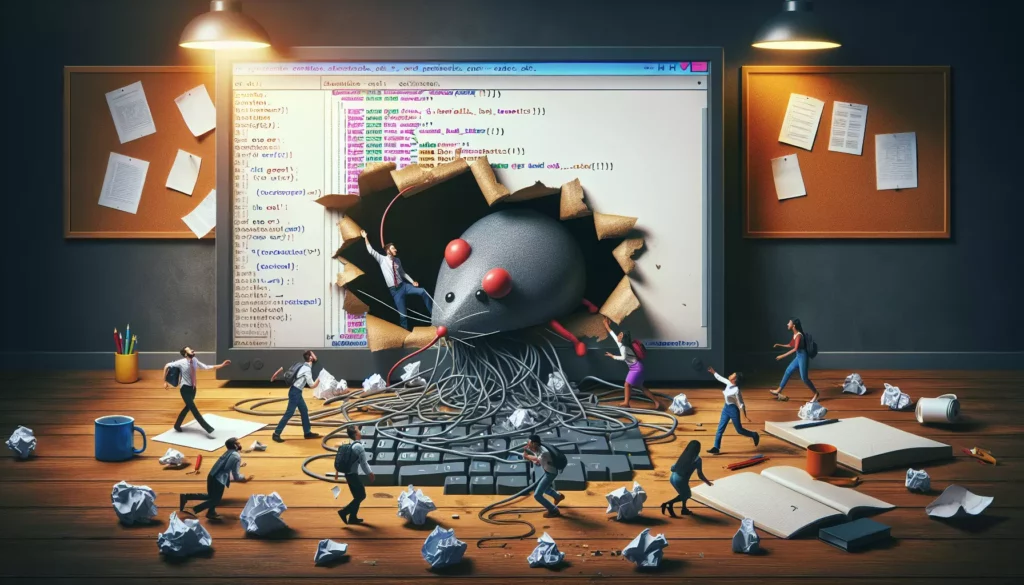
In the world of programming education, there’s a common misconception that creating your own test cases is the best way to validate your code. While self-testing is an important skill, relying solely on your own test cases can lead to a false sense of security and incomplete learning. This article explores why creating your own test cases isn’t enough, and how a more comprehensive approach to testing can make you a better programmer.
Table of Contents
- The Blind Spot Problem: Why Your Test Cases Miss Critical Scenarios
- Cognitive Bias in Self-Testing
- Real-World Testing Requirements
- The Art of Edge Cases
- Performance Testing Limitations
- Why This Matters for Technical Interviews
- A Better Approach to Testing Your Code
- Tools and Resources for Comprehensive Testing
- Conclusion: Building a Testing Mindset
The Blind Spot Problem: Why Your Test Cases Miss Critical Scenarios
When you write code to solve a problem, you naturally develop a mental model of how that problem works. This mental model shapes how you approach the solution and, consequently, how you test it. The fundamental issue with creating your own test cases is that they emerge from the same mental model that produced the code in the first place.
Consider this simple function to find the maximum value in an array:
function findMax(arr) {
let max = arr[0];
for (let i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
If you’re testing this function yourself, you might write test cases like:
console.log(findMax([1, 3, 5, 2, 4])); // Expected output: 5
console.log(findMax([10, 7, 3, 1])); // Expected output: 10
console.log(findMax([5, 5, 5])); // Expected output: 5
These tests pass, so you might conclude your function works correctly. But what about these scenarios?
console.log(findMax([])); // Error: Cannot read property '0' of undefined
console.log(findMax([-10, -20, -5])); // Expected output: -5
Your original test cases missed crucial scenarios: an empty array and an array with only negative numbers. These blind spots exist because they weren’t part of your initial mental model of the problem.
This example is deliberately simple, but as problems become more complex, the potential for blind spots grows exponentially. The mental model that helps you solve a problem can simultaneously limit your ability to test it comprehensively.
Cognitive Bias in Self-Testing
Several cognitive biases affect our ability to create comprehensive test cases:
Confirmation Bias
When testing your own code, you’re naturally inclined to confirm that your solution works rather than find ways it might fail. This confirmation bias leads to test cases that validate your existing approach rather than challenge it.
For example, if you implement a sorting algorithm, you might test it with arrays that you know should be easy to sort, rather than those that might expose weaknesses in your implementation, like nearly-sorted arrays or arrays with many duplicates.
Anchoring Bias
The first few test cases you create often “anchor” your thinking, limiting your ability to imagine drastically different scenarios. If you start testing with small, positive integers, you might not think to test with very large numbers, negative values, or non-numeric inputs.
The Curse of Knowledge
Once you know how your code works, it’s difficult to step back and think about how it might fail. This “curse of knowledge” prevents you from seeing your solution with fresh eyes. You’re aware of the constraints and assumptions you built into your code, but you might not recognize when those assumptions don’t hold in all cases.
Consider this function that checks if a string is a palindrome:
function isPalindrome(str) {
const cleanStr = str.toLowerCase().replace(/[^a-z0-9]/g, '');
return cleanStr === cleanStr.split('').reverse().join('');
}
You might test it with:
console.log(isPalindrome("racecar")); // true
console.log(isPalindrome("A man, a plan, a canal: Panama")); // true
console.log(isPalindrome("hello")); // false
But what about:
console.log(isPalindrome("")); // true (Is this correct?)
console.log(isPalindrome(12321)); // Error: str.toLowerCase is not a function
console.log(isPalindrome(null)); // Error
Because you know your function expects a string and processes it in a certain way, you might not think to test it with non-string inputs or edge cases like empty strings.
Real-World Testing Requirements
In professional software development, testing goes far beyond verifying that code produces the expected output for a few sample inputs. Real-world testing must account for:
Security Vulnerabilities
Can your code be exploited? Consider input validation and sanitization. For web applications, are you protected against common attacks like SQL injection, XSS, or CSRF? These security considerations rarely factor into self-created test cases.
Accessibility
For user-facing applications, does your code work for people with disabilities? Can it be navigated by keyboard or screen reader? These requirements are often overlooked in personal test cases.
Internationalization
Does your code handle different languages, character sets, and cultural conventions? For example, sorting algorithms might behave differently with non-ASCII characters.
// A sorting function that doesn't account for internationalization
function sortNames(names) {
return names.sort();
}
// This might not sort correctly for non-English names
console.log(sortNames(["Zoë", "Ángel", "Bob"]));
Concurrency Issues
If multiple users or processes interact with your code simultaneously, race conditions and deadlocks can occur. These issues are notoriously difficult to reproduce with simple test cases.
let counter = 0;
// This function is not thread-safe
function incrementCounter() {
const current = counter;
// Simulating some delay that could allow another thread to intervene
setTimeout(() => {
counter = current + 1;
}, 0);
}
Self-testing rarely accounts for these complex, real-world scenarios, leaving your code vulnerable to issues that only emerge in production environments.
The Art of Edge Cases
Edge cases are inputs at the extremes of the possible range. They often reveal assumptions in your code that don’t hold universally. Common edge cases include:
- Empty collections (empty arrays, strings, maps)
- Single-element collections
- Very large or very small inputs
- Null or undefined values
- Inputs of unexpected types
- Boundary conditions (e.g., array indices at the beginning or end)
Let’s look at a function that calculates the average of an array of numbers:
function average(numbers) {
let sum = 0;
for (let i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
return sum / numbers.length;
}
Edge cases that might break this function include:
console.log(average([])); // NaN (division by zero)
console.log(average([0])); // 0 (correct, but is this expected?)
console.log(average([1, 2, "3"])); // Not a pure numeric average due to string concatenation
console.log(average([Number.MAX_VALUE, Number.MAX_VALUE])); // Potential overflow
Experienced testers and interviewers are particularly good at identifying these edge cases, which is why relying solely on your own test cases is risky, especially in an interview setting.
Performance Testing Limitations
Beyond correctness, code needs to be efficient. Performance testing evaluates how your code behaves with large inputs or under heavy load. This type of testing is difficult to do manually and often requires specialized tools.
Consider two implementations of a function to find duplicates in an array:
// Implementation 1: O(n²) time complexity
function findDuplicates1(arr) {
const duplicates = [];
for (let i = 0; i < arr.length; i++) {
for (let j = i + 1; j < arr.length; j++) {
if (arr[i] === arr[j] && !duplicates.includes(arr[i])) {
duplicates.push(arr[i]);
}
}
}
return duplicates;
}
// Implementation 2: O(n) time complexity
function findDuplicates2(arr) {
const seen = new Set();
const duplicates = new Set();
for (const item of arr) {
if (seen.has(item)) {
duplicates.add(item);
} else {
seen.add(item);
}
}
return [...duplicates];
}
With small test arrays, both functions will produce correct results and execute quickly. The performance difference only becomes apparent with large inputs, which you might not think to test:
// Generate a large array with some duplicates
const largeArray = Array.from({ length: 10000 }, () => Math.floor(Math.random() * 1000));
// Measure performance
console.time('Implementation 1');
findDuplicates1(largeArray);
console.timeEnd('Implementation 1');
console.time('Implementation 2');
findDuplicates2(largeArray);
console.timeEnd('Implementation 2');
The second implementation will be significantly faster, but this performance difference isn’t captured by simple correctness tests with small inputs.
Why This Matters for Technical Interviews
Technical interviews, especially at top tech companies, are designed to evaluate not just whether you can solve a problem, but how thoroughly you consider edge cases, performance implications, and potential issues.
When an interviewer asks you to write code, they’re watching for:
- Whether you test your code
- The types of test cases you create
- Your ability to identify edge cases
- How you handle unexpected inputs
- Your understanding of time and space complexity
Relying solely on your own test cases in interview preparation can leave you vulnerable to these evaluation points. Interviewers often have prepared edge cases specifically designed to challenge common assumptions or reveal typical blind spots.
Additionally, many technical interviews use automated testing systems that run your code against a comprehensive test suite. If you’ve only tested your solution with a few self-created cases, you might be surprised when the hidden test cases reveal issues you didn’t anticipate.
Interview Example: String Manipulation
Consider this interview question: “Write a function that determines if two strings are anagrams of each other.”
A candidate might write:
function areAnagrams(str1, str2) {
return str1.split('').sort().join('') === str2.split('').sort().join('');
}
And test it with:
console.log(areAnagrams("listen", "silent")); // true
console.log(areAnagrams("hello", "world")); // false
But an interviewer would likely follow up with questions or test cases like:
- What about case sensitivity? (e.g., “Listen” and “Silent”)
- How does your function handle spaces or punctuation? (e.g., “A decimal point” and “I’m a dot in place”)
- What if the inputs aren’t strings?
- What’s the time and space complexity of your solution?
- Could you implement a more efficient solution for very long strings?
By relying only on self-created test cases during preparation, the candidate misses the opportunity to develop a more robust solution that addresses these concerns.
A Better Approach to Testing Your Code
Given the limitations of self-testing, how can you develop a more comprehensive approach to validating your code? Here are strategies to overcome the blind spots in your testing:
Adopt Test-Driven Development (TDD)
Test-Driven Development encourages you to write tests before you write code. This approach helps separate your testing mindset from your implementation mindset:
- Write a failing test for a specific behavior
- Implement the minimum code needed to pass the test
- Refactor your code while keeping the tests passing
- Repeat for the next behavior
By thinking about tests first, you’re less likely to be influenced by implementation details when designing test cases.
Use Testing Frameworks
Testing frameworks like Jest (JavaScript), pytest (Python), or JUnit (Java) provide structured ways to write and organize tests. They also offer features like test runners, assertions, and coverage reports that help ensure comprehensive testing.
// Example using Jest
test('findMax returns the maximum value in an array', () => {
expect(findMax([1, 3, 5, 2, 4])).toBe(5);
expect(findMax([10, 7, 3, 1])).toBe(10);
expect(findMax([5, 5, 5])).toBe(5);
expect(findMax([-10, -20, -5])).toBe(-5);
});
test('findMax throws an error for empty arrays', () => {
expect(() => findMax([])).toThrow();
});
Practice Systematic Test Case Generation
Develop a systematic approach to generating test cases. For each function, consider:
- Normal cases: Typical inputs that should work correctly
- Edge cases: Boundary conditions like empty arrays, single elements, etc.
- Error cases: Inputs that should trigger error handling
- Performance cases: Large or complex inputs that test efficiency
For example, when testing a sorting function, you might include:
- Already sorted array
- Reverse sorted array
- Array with duplicates
- Empty array
- Single-element array
- Array with negative numbers
- Very large array (for performance)
Use Property-Based Testing
Property-based testing generates random inputs and checks that certain properties hold true for all inputs. This approach can uncover edge cases you might not think of.
For example, with a sorting function, properties to test might include:
- The output array length equals the input array length
- The output array contains the same elements as the input
- Each element in the output is less than or equal to the next element
Libraries like fast-check (JavaScript), Hypothesis (Python), or QuickCheck (Haskell) support property-based testing.
Peer Review
Have others review your code and tests. Fresh eyes often spot issues that you’ve overlooked. This is why code reviews are standard practice in professional software development.
Even if you’re learning independently, participating in coding communities or forums can provide valuable feedback on your solutions and testing approaches.
Tools and Resources for Comprehensive Testing
To move beyond self-created test cases, leverage these tools and resources:
Testing Frameworks
- JavaScript: Jest, Mocha, Jasmine
- Python: pytest, unittest
- Java: JUnit, TestNG
- C#: NUnit, MSTest
- Ruby: RSpec, Minitest
Property-Based Testing Libraries
- JavaScript: fast-check, jsverify
- Python: Hypothesis
- Java: jqwik
- Haskell: QuickCheck
Code Coverage Tools
- JavaScript: Istanbul (nyc)
- Python: Coverage.py
- Java: JaCoCo
- C#: Coverlet
Online Platforms with Comprehensive Test Suites
- LeetCode: Provides extensive test cases for algorithmic problems
- HackerRank: Runs your code against multiple test cases, including edge cases
- CodeSignal: Offers detailed feedback on test case failures
- AlgoCademy: Provides guided practice with comprehensive test suites and explanations
Books and Resources
- “Test Driven Development: By Example” by Kent Beck
- “Effective Unit Testing” by Lasse Koskela
- “The Art of Software Testing” by Glenford J. Myers
- Online courses focused on testing methodologies
Conclusion: Building a Testing Mindset
Creating your own test cases is a valuable skill, but it’s not sufficient for developing robust, production-ready code or preparing for technical interviews. The limitations of self-testing—blind spots, cognitive biases, and the difficulty of simulating real-world conditions—mean that relying solely on your own test cases can lead to a false sense of security.
Instead, aim to develop a comprehensive testing mindset:
- Approach testing systematically, considering normal cases, edge cases, error cases, and performance
- Use testing frameworks and property-based testing to uncover issues you might miss
- Seek external feedback through peer review or automated test suites
- Practice with platforms that provide comprehensive test cases
- Study common edge cases and testing patterns in your domain
By recognizing the limitations of self-testing and adopting more rigorous approaches, you’ll not only write more reliable code but also develop the critical thinking skills that technical interviewers at top companies are looking for.
Remember, the goal isn’t just to pass your own tests—it’s to write code that works correctly in all scenarios, even those you haven’t thought of yet. As you practice and prepare, challenge yourself to think beyond the obvious test cases and consider how your code might behave in unexpected situations. This mindset will serve you well both in interviews and in your career as a software developer.
The next time you solve a coding problem, instead of asking “Does my code work for these examples?” ask “What examples might break my code that I haven’t considered?” This shift in perspective is the first step toward more comprehensive testing and more robust solutions.