Why You Know What to Code But Not Where to Start
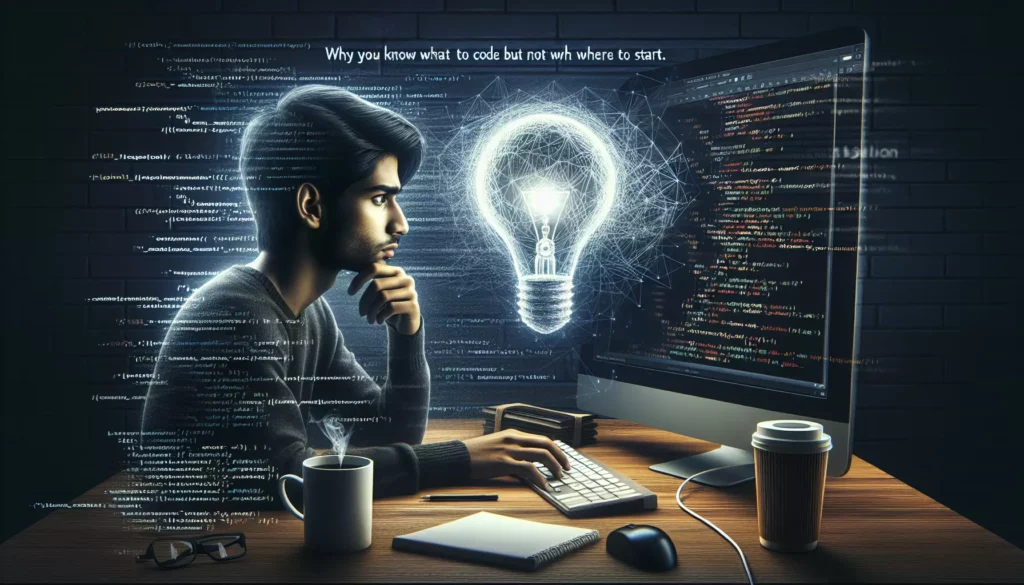
Have you ever sat down to code, armed with knowledge about programming languages, data structures, and algorithms, but found yourself staring at a blank screen, unsure where to begin? This phenomenon is more common than you might think, even among experienced developers. Understanding the theory behind coding is one thing, but applying that knowledge to start and complete a project is an entirely different challenge.
In this comprehensive guide, we’ll explore the gap between knowing how to code and knowing where to start, and provide practical strategies to bridge this divide. Whether you’re a beginner feeling overwhelmed by possibilities or an experienced developer facing a complex new project, these insights will help you overcome that initial paralysis and start writing code with confidence.
The Knowledge Application Gap in Programming
The disconnect between theoretical knowledge and practical application is what educators often call the “knowledge application gap.” In programming, this gap can feel particularly wide.
Understanding the Paradox
You might be proficient in Python, understand object oriented programming, know how to implement a binary search tree, and even recite the time complexity of various sorting algorithms. Yet, when faced with a blank editor and a project requirement, you freeze. Why does this happen?
This paradox exists because:
- Theory vs. Practice: Learning programming concepts is different from applying them in real world scenarios
- Analysis Paralysis: Too many possible approaches can lead to decision fatigue
- Missing Context: Textbook problems have clear boundaries; real projects are messier
- Integration Challenges: Knowing individual components doesn’t automatically translate to understanding how they work together
A common analogy is knowing all the chess pieces and their moves but struggling to develop a coherent strategy in an actual game. The rules are clear, but the application is complex.
The Blank Canvas Syndrome
Artists often talk about “blank canvas syndrome”—the intimidation of starting with nothing. Programmers face a similar challenge with empty code editors. This intimidation can manifest as:
- Overthinking the initial architecture
- Worrying about making the “perfect” first move
- Concern about committing to an approach that might need significant reworking later
- Uncertainty about which problems to tackle first
The irony is that this hesitation often leads to no progress at all, which is objectively worse than starting with an imperfect approach.
Common Barriers to Getting Started
Before we discuss solutions, let’s identify the specific barriers that might be holding you back from starting your coding projects.
Perfectionism and Fear of Failure
Many programmers, especially those who excel academically, struggle with perfectionism. This manifests as:
- Wanting to create the most elegant solution from the beginning
- Fear that your code won’t be “good enough”
- Anxiety about potential criticism from peers or mentors
- Reluctance to commit to code that might need refactoring later
Remember that even senior developers write code they later improve. As the saying goes, “Perfect is the enemy of done.”
Overwhelming Project Scope
Large projects can seem insurmountable when viewed in their entirety. This is especially true when:
- Requirements are extensive or complex
- The project involves unfamiliar technologies
- Multiple systems need to interact
- The end goal seems distant from your starting point
Without breaking down these large projects, the sheer scope can prevent you from taking the first step.
Decision Overload in Technology Choices
The programming ecosystem offers countless tools, frameworks, libraries, and approaches. This abundance can lead to decision paralysis:
- Which framework should I use?
- What’s the best database for this project?
- Should I use a specific design pattern?
- Is this technology still relevant, or should I learn something newer?
Each decision can feel high stakes, especially when you’re uncertain about the long term implications of your choices.
Lack of Clear Starting Points
Unlike academic exercises with clear instructions, real world projects rarely come with step by step guides. You might face:
- Ambiguous requirements
- Multiple valid entry points
- Uncertainty about which components to prioritize
- No obvious “correct” first step
This ambiguity can leave you second guessing where to begin, leading to procrastination.
Strategies to Overcome the Starting Hurdle
Now that we’ve identified the common barriers, let’s explore practical strategies to help you start coding with confidence.
Embrace Incremental Development
One of the most powerful approaches to starting a project is breaking it down into smaller, manageable pieces:
Start with a Minimum Viable Product (MVP)
Define the simplest version of your project that could still provide value:
- Identify core functionality that represents the essence of your project
- Postpone nice to have features for later iterations
- Focus on getting a working skeleton before adding complexity
For example, if building a todo app, start with just adding and displaying items—no categories, due dates, or reminders yet.
Use the “Tracer Bullet” Approach
As described in “The Pragmatic Programmer,” a tracer bullet is a thin implementation that connects all major components:
- Build a minimal end to end implementation that touches all layers
- Ensure data can flow through the entire system
- Create a framework you can flesh out incrementally
For a web application, this might mean creating a simple page that connects to your database and displays something—even if it’s just “Hello World” from your database.
Implement Feature Slices
Rather than building all components of one feature before moving to the next:
- Implement thin vertical slices of functionality
- Complete one user journey at a time
- Add depth to features iteratively
This approach gives you working features sooner and provides motivation to continue.
Practical Planning Techniques
Before writing code, use these planning techniques to create clarity:
Write Pseudocode First
Pseudocode bridges the gap between human language and programming language:
// Instead of jumping straight into code, write:
// 1. Fetch user data from API
// 2. Filter users based on active status
// 3. Sort users by last login date
// 4. Display users in a paginated table
This approach lets you focus on logic without getting caught up in syntax details.
Create a Component Diagram
Visualize the structure of your application:
- Identify major components and their relationships
- Determine data flow between components
- Establish system boundaries and interfaces
Even a simple hand drawn diagram can provide clarity on where to begin.
Use the Five Ws Method
Answer the fundamental questions about your project:
- What exactly are you building?
- Why are you building it? (What problem does it solve?)
- Who will use it?
- When will different components be needed?
- Where will it be deployed or used?
These answers can help identify natural starting points and priorities.
Technical Approaches to Getting Started
Once you’re ready to code, these technical strategies can help you make progress:
Start with Data Structures
Define how your data will be structured before implementing functionality:
// For a task management app, start by defining:
class Task {
constructor(id, title, description, dueDate, status) {
this.id = id;
this.title = title;
this.description = description;
this.dueDate = dueDate;
this.status = status;
}
}
class TaskList {
constructor() {
this.tasks = [];
}
addTask(task) {
this.tasks.push(task);
}
// Other methods will go here
}
With your data structures in place, the functionality often becomes clearer.
Begin with Interfaces
Define the interfaces between components before implementing them:
- Create API contracts
- Define function signatures
- Establish communication protocols
This approach forces you to think about how components will interact.
Write Tests First
Test Driven Development (TDD) provides a structured starting point:
// Write a test that defines expected behavior
test('should add a new task to the task list', () => {
const taskList = new TaskList();
const task = new Task(1, 'Learn TDD', 'Study test-driven development', '2023-12-31', 'pending');
taskList.addTask(task);
expect(taskList.tasks.length).toBe(1);
expect(taskList.tasks[0]).toBe(task);
});
With tests in place, you have a clear target for your implementation.
Overcoming Psychological Barriers
Sometimes the biggest hurdles are psychological. Here’s how to address them:
Use Timeboxing
Set a specific time limit for starting:
- “I’ll work on this for just 25 minutes”
- Use the Pomodoro Technique
- Commit to writing any code, even if imperfect
Often, the momentum from starting will carry you forward.
Apply the Two Minute Rule
Borrowed from productivity expert David Allen:
- If a task takes less than two minutes, do it immediately
- For coding, create a “two minute version” of starting your project
- Example: Set up a project folder and initialize a Git repository
These small steps make the project feel real and reduce the activation energy needed to continue.
Embrace “Worse is Better”
This philosophy suggests that:
- A simple but imperfect solution now is better than a perfect solution later
- Working code provides immediate feedback
- You can refactor and improve once you have something tangible
Give yourself permission to write code that’s “good enough” for now.
Practical Starting Points for Different Project Types
Different types of projects lend themselves to different starting approaches. Here are specific suggestions based on project type:
Web Applications
For web applications, consider these starting points:
Frontend First Approach
- Create static mockups of key screens
- Implement UI components with hardcoded data
- Add interactivity before connecting to backend
This approach gives you visible progress and helps clarify data requirements.
Backend First Approach
- Define your API endpoints
- Implement basic CRUD operations
- Set up database models and relationships
Starting with the backend ensures your data layer is solid before adding UI complexity.
Authentication Starting Point
- Implement user registration and login
- Set up session management
- Configure authorization rules
Authentication is often a prerequisite for other features, making it a logical first step.
Mobile Applications
Mobile apps have their own ideal starting points:
Navigation Structure
- Define the app’s screen hierarchy
- Implement navigation between key screens
- Set up placeholder content
This creates the skeleton of your app that you can flesh out incrementally.
Core Functionality
- Identify the app’s primary purpose
- Implement the central feature in its simplest form
- Ensure it works offline before adding cloud features
Focus on what makes your app unique before adding standard features.
Data Science Projects
Data science projects benefit from these starting approaches:
Data Exploration Notebook
- Load a sample of your dataset
- Perform basic exploration and visualization
- Identify patterns and potential challenges
Understanding your data is always the right first step in data science.
Pipeline Skeleton
- Create a basic data processing pipeline
- Implement placeholder functions for each stage
- Ensure data can flow through the entire process
This approach helps you focus on the overall structure before optimizing individual components.
Algorithmic Challenges
For algorithm problems or competitive programming:
Input/Output Framework
- Set up code to handle the expected input format
- Create a structure to produce the required output
- Implement a naive solution that connects input to output
With the I/O framework in place, you can focus on optimizing the algorithm.
Brute Force First
- Implement the simplest solution that works
- Verify correctness with test cases
- Optimize incrementally
Starting with a brute force approach ensures you understand the problem before optimizing.
Learning from Others: Templates and Examples
There’s no need to start completely from scratch. Learn from existing resources:
Project Templates and Boilerplates
Many frameworks offer starter templates that provide structure:
- Create React App for React projects
- Django’s startproject command
- Spring Initializr for Java applications
- Flutter’s starter templates
These templates handle configuration and provide a working foundation.
Open Source Inspiration
Studying similar open source projects can provide insights:
- Examine project structure and organization
- Review how features are implemented
- Understand common patterns and practices
Don’t copy code directly, but use these projects as learning resources.
Starter Kits and Generators
Tools that generate project scaffolding can help you start quickly:
- Yeoman generators for various project types
- Next.js create-next-app
- Ruby on Rails generators
- NestJS CLI
These tools create conventional project structures based on best practices.
Building Starting Momentum
Once you’ve taken the first step, maintain momentum with these strategies:
Celebrate Small Wins
Acknowledge progress, no matter how small:
- Recognize each completed component
- Take satisfaction in solving challenging problems
- Share progress with peers or mentors
Small wins compound into significant progress over time.
Create a Development Routine
Establish habits that reduce the activation energy for coding:
- Set aside specific times for coding
- Create a comfortable development environment
- Develop pre coding rituals (like reviewing your plan)
Routine reduces the decision fatigue that can prevent you from starting.
Use Continuous Integration from Day One
Set up CI/CD pipelines early:
- Automated testing for each commit
- Deployment pipelines, even to development environments
- Code quality checks
This creates accountability and ensures your code remains in a working state.
Case Study: From Paralysis to Progress
Let’s examine how these principles might apply to a real world scenario:
The Challenge: Building a Personal Finance Tracker
Imagine you want to build a web application that helps users track expenses, categorize spending, and visualize financial patterns.
Initial Paralysis
You might find yourself stuck with questions like:
- Should I use React, Vue, or Angular?
- What’s the best database schema for financial data?
- How should I handle security for sensitive information?
- What visualizations should I include?
These questions can prevent you from writing any code at all.
Applied Strategy: Incremental Development
Here’s how you might apply the strategies we’ve discussed:
Step 1: Define MVP Features
- Manual expense entry (amount, date, category)
- List view of recent expenses
- Simple monthly total calculation
Step 2: Choose a Starting Point
Decide to start with data structures:
class Expense {
constructor(id, amount, date, category, description) {
this.id = id;
this.amount = amount;
this.date = date;
this.category = category;
this.description = description;
}
}
class ExpenseTracker {
constructor() {
this.expenses = [];
}
addExpense(expense) {
this.expenses.push(expense);
}
getMonthlyTotal(year, month) {
return this.expenses
.filter(exp => {
const expDate = new Date(exp.date);
return expDate.getFullYear() === year && expDate.getMonth() === month;
})
.reduce((total, exp) => total + exp.amount, 0);
}
}
Step 3: Create a Tracer Bullet Implementation
Build a simple UI that connects to your data layer:
- A form to add expenses
- A table to display expenses
- A component to show monthly totals
Use local storage before implementing a backend.
Step 4: Iterate and Expand
With the core functionality working, add features incrementally:
- Implement expense categories with color coding
- Add date filtering
- Create a simple bar chart for monthly comparisons
Result: A Working Application
Instead of being paralyzed by all possible features and technical decisions, you now have:
- A functioning application that delivers core value
- A solid foundation for adding more complex features
- Momentum and motivation to continue development
This approach transforms the overwhelming task of “build a finance app” into a series of manageable steps, each building on the last.
When to Pause and Rethink
While starting is important, there are times when you should pause before coding:
Legitimate Planning Needs
Some projects genuinely require more planning:
- Systems with complex data relationships
- Applications with strict security requirements
- Projects where architectural mistakes are costly to fix
In these cases, more upfront design may be justified.
Research vs. Procrastination
Learn to distinguish between necessary research and avoidance:
- Necessary research answers specific questions relevant to starting
- Procrastination disguised as research lacks focus and clear endpoints
Set time limits for research to prevent indefinite delays.
When to Prototype First
Some situations call for throwaway prototypes:
- Testing technical feasibility
- Exploring user experience options
- Validating performance assumptions
A prototype can answer critical questions before you invest in a production implementation.
Conclusion: From Knowledge to Action
The gap between knowing what to code and knowing where to start is bridged through deliberate practice and strategic approaches. Remember these key principles:
- Start small with minimal viable implementations
- Break down complex projects into manageable pieces
- Use planning techniques to create clarity before coding
- Embrace imperfection in early iterations
- Build momentum through small wins
The most important step in any coding project is the first one. By applying the strategies we’ve discussed, you can transform theoretical knowledge into practical progress, one line of code at a time.
Remember that every experienced developer has faced the blank editor problem. What separates successful programmers isn’t just their knowledge, but their ability to start, persist, and iterate. With practice, your starting point will become clearer, your initial steps more confident, and your progress more consistent.
Now, close this article and write that first line of code. Your future self will thank you for starting today.