Why You Recognize Patterns But Can’t Implement Them: Bridging the Gap in Coding Skills
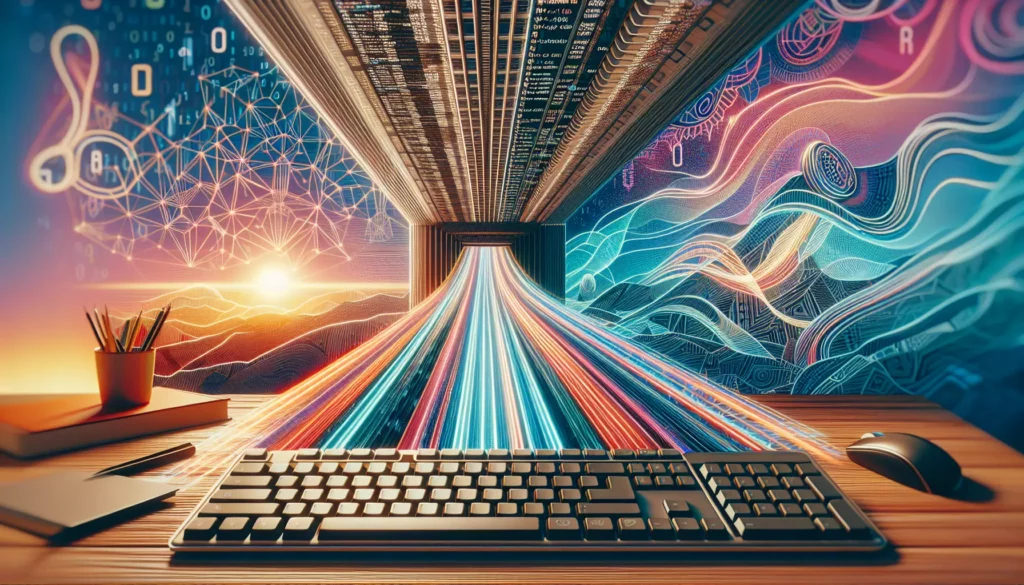
Have you ever found yourself nodding along to a coding tutorial, completely understanding the concept, only to freeze when faced with a blank editor? You’re not alone. Many aspiring programmers experience this frustrating disconnect between recognition and implementation. This phenomenon, often called the “familiarity trap,” affects learners at all levels and can significantly slow down your progress in mastering programming.
In this comprehensive guide, we’ll explore why recognizing patterns doesn’t automatically translate to implementing them, and more importantly, how to bridge this gap effectively. Whether you’re preparing for technical interviews at major tech companies or simply trying to level up your coding skills, understanding this cognitive disconnect is crucial for your development as a programmer.
The Recognition vs. Implementation Gap
The human brain is remarkably efficient at pattern recognition. When you read code or watch someone solve a problem, your brain can follow along and make sense of what’s happening. This creates an illusion of comprehension that feels like learning. However, implementation requires a different set of cognitive processes altogether.
Why Recognition Is Easier Than Implementation
Several cognitive principles explain why recognizing patterns is easier than implementing solutions:
- Recognition uses passive knowledge: When you read or watch code, you’re engaging with information passively. Your brain simply needs to match what you’re seeing with concepts you’ve encountered before.
- Implementation requires active recall: Writing code from scratch forces you to retrieve information from memory without prompts or cues, which is significantly more challenging.
- Recognition has contextual cues: When reviewing solutions, you’re given the context, problem statement, and solution simultaneously, making connections obvious.
- Implementation starts with a blank slate: You must generate the solution structure, syntax, and logic without external scaffolding.
This gap isn’t unique to programming. It appears in many learning contexts, from language acquisition to musical performance. However, in coding, the consequences are particularly noticeable because the gap between understanding and execution is immediately evident when your code doesn’t compile or produce the expected output.
The Illusion of Competence
One of the most insidious aspects of this disconnect is what psychologists call the “illusion of competence.” When you recognize a pattern or understand an explanation, your brain produces a feeling of knowing that can be misleading.
For instance, when you follow a tutorial on implementing a binary search algorithm, the steps might seem logical and straightforward. The instructor explains each line, and you nod along, thinking, “This makes perfect sense.” But when you try to implement it yourself the next day, you might struggle to remember the crucial details or the overall structure.
This illusion leads many learners to:
- Overestimate their readiness to tackle similar problems
- Spend too little time on active practice
- Feel discouraged when implementation proves more difficult than expected
- Question their aptitude for programming altogether
Understanding this psychological trap is the first step toward overcoming it. The feeling of comprehension doesn’t equate to the ability to implement, and acknowledging this gap can help calibrate your learning expectations.
The Cognitive Load of Implementation
When implementing code, your working memory juggles multiple elements simultaneously:
- The problem requirements and constraints
- The algorithmic approach you’ve chosen
- Syntax rules of the programming language
- Edge cases and potential errors
- Testing strategies to verify your solution
This cognitive load quickly exceeds what most people can comfortably manage, especially for complex problems. Recognition, by contrast, distributes this load over time as you process each element sequentially while reading or watching.
Consider this simple example of finding the maximum value in an array:
function findMax(arr) {
if (arr.length === 0) return null;
let max = arr[0];
for (let i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
When reading this code, you process it line by line, understanding each part before moving to the next. But when implementing it, you need to simultaneously consider:
- How to handle empty arrays
- Initializing the max variable appropriately
- Setting up the loop correctly
- Implementing the comparison logic
- Returning the right result
For beginners, this cognitive juggling act can be overwhelming, leading to implementation paralysis even when the concept seems straightforward.
The Expertise Reversal Effect
As you gain experience, the gap between recognition and implementation typically narrows, but another interesting phenomenon occurs: the expertise reversal effect. This cognitive principle suggests that teaching methods that work well for beginners can actually hinder advanced learners.
For novice programmers, detailed step-by-step tutorials with extensive explanations help manage cognitive load. However, as you become more proficient, these same detailed instructions can become redundant and distracting, potentially interfering with your ability to develop efficient mental models.
This explains why experienced programmers often prefer documentation with concise examples over lengthy tutorials, while beginners benefit from more comprehensive guidance. Understanding where you are on this continuum can help you select learning resources that address the recognition-implementation gap appropriately for your skill level.
From Recognition to Implementation: Practical Strategies
Now that we understand the cognitive mechanisms behind the recognition-implementation gap, let’s explore practical strategies to bridge it effectively.
1. Practice Active Recall
Active recall is the process of retrieving information from memory rather than simply reviewing it. This technique is one of the most powerful learning methods for moving knowledge from recognition to implementation.
Try these approaches:
- Close the tutorial: After watching or reading a tutorial, close it and attempt to recreate the solution without looking back.
- Explain the concept aloud: Teaching requires deeper understanding than mere recognition. Explain the algorithm or concept to someone else (or even to an imaginary student).
- Use spaced repetition: Revisit problems after increasing intervals (1 day, 3 days, 1 week) to strengthen recall and prevent forgetting.
For example, after learning about merge sort, don’t just move on to the next algorithm. Close the tutorial and try to implement merge sort from memory. The struggle to recall the steps is precisely what strengthens your implementation ability.
2. Break Down Complex Problems
Complex problems overwhelm working memory, making implementation difficult even when you understand the general approach. Breaking down problems into smaller, manageable components can help bridge the gap:
- Use pseudocode first: Before writing actual code, sketch the algorithm in pseudocode to focus on logic without syntax concerns.
- Implement one function at a time: For multi-part problems, complete and test each function before moving to the next.
- Create a solution roadmap: Outline the steps you’ll need to take before writing any code.
Consider this approach for implementing a binary search tree:
// Step 1: Define the node structure
// Step 2: Implement insertion
// Step 3: Implement search
// Step 4: Implement deletion
// Step 5: Add traversal methods
By focusing on one component at a time, you reduce cognitive load and make implementation more manageable.
3. Practice Deliberate Implementation
Not all practice is created equal. Deliberate practice focuses on targeted improvement rather than mere repetition:
- Implement variations: After solving a problem, modify the constraints and solve it again (e.g., make it work with negative numbers, optimize for space).
- Time-box challenges: Set a timer when implementing solutions to simulate interview conditions and build implementation speed.
- Refactor existing code: Take working code and rewrite it to be more efficient, readable, or maintainable.
For instance, after implementing a function to find duplicates in an array using a hash map, challenge yourself to solve it with constant space complexity or for sorted arrays.
4. Build Mental Models Through Visualization
Strong mental models help bridge the recognition-implementation gap by providing conceptual frameworks you can recall during implementation:
- Draw diagrams: Visualize data structures and algorithms on paper before coding them.
- Trace code execution manually: Step through algorithms with sample inputs, tracking variable changes.
- Use memory aids: Create mnemonics or visual cues for common patterns.
For recursive algorithms like tree traversal, drawing the recursion tree and tracing the execution stack can transform abstract recognition into concrete understanding that supports implementation.
5. Develop Implementation Templates
Many programming problems follow common patterns. Developing templates for these patterns can reduce cognitive load during implementation:
- Create personal libraries: Build a collection of implemented solutions to common problem types.
- Memorize core algorithms: Know the implementation details of fundamental algorithms like binary search, BFS/DFS, and dynamic programming approaches.
- Practice variations on templates: Use your templates as starting points, then modify them for specific problems.
For example, this basic template for two-pointer string problems can be adapted to many scenarios:
function twoPointerStringProblem(s) {
let left = 0;
let right = s.length - 1;
while (left < right) {
// Problem-specific logic here
left++;
right--;
}
return result;
}
Having this structure in mind reduces the implementation burden when tackling problems like palindrome checking or string reversal.
Common Implementation Roadblocks and How to Overcome Them
Even with the strategies above, specific roadblocks often impede the transition from recognition to implementation. Let’s address some of the most common ones:
Syntax Paralysis
Many programmers freeze when faced with syntax details, even when they understand the algorithm conceptually.
Solution: Create syntax cheat sheets for your preferred language. Practice typing common constructs like loops, conditionals, and function definitions until they become muscle memory. Use an IDE with good autocomplete features during practice to reduce syntax burden.
// JavaScript loop syntax examples to memorize
// For loop
for (let i = 0; i < array.length; i++) { }
// While loop
while (condition) { }
// For...of loop (iterables)
for (const item of iterable) { }
// For...in loop (object properties)
for (const key in object) { }
Starting Point Anxiety
It’s common to understand a solution conceptually but struggle with where to begin the implementation.
Solution: Develop a consistent problem-solving framework:
- Define inputs and outputs clearly
- Work through examples manually
- Sketch the high-level approach
- Write function signatures before implementation
For example, when implementing a function to merge two sorted arrays, start by defining:
function mergeSortedArrays(arr1, arr2) {
// Input: Two sorted arrays of integers
// Output: A single sorted array containing all elements
// Implementation will go here
}
This simple scaffolding can overcome the blank page syndrome that often paralyzes implementation.
Edge Case Blindness
Failing to account for edge cases is a common implementation issue, even when the main algorithm is understood.
Solution: Develop an edge case checklist for common data structures and problem types:
- For arrays: empty arrays, single-element arrays, duplicates
- For strings: empty strings, single characters, case sensitivity, special characters
- For trees: null nodes, leaf nodes, unbalanced trees
- For numbers: zero, negative values, overflow, underflow
Review this checklist during implementation to ensure your solution handles all scenarios correctly.
Debugging Paralysis
When code doesn’t work as expected, many programmers struggle to debug effectively, especially under time pressure.
Solution: Practice systematic debugging techniques:
- Use console logs or debugger statements strategically
- Test with simplified inputs first
- Isolate components and test them separately
- Compare actual vs. expected behavior at each step
Developing debugging fluency is as important as implementation skill for bridging the recognition-implementation gap.
From Theory to Practice: Application in Technical Interviews
The recognition-implementation gap becomes particularly evident during technical interviews at companies like Google, Amazon, or Facebook. While you might recognize the pattern of a problem, implementing it under time pressure with an interviewer watching can be challenging.
Interview-Specific Strategies
To bridge the gap effectively during interviews:
- Verbalize your thought process: Explain your approach before and during implementation to clarify your thinking and receive feedback.
- Start with a working solution: Focus first on implementing a correct solution, even if it’s not optimal. You can optimize later if time permits.
- Practice with realistic constraints: Simulate interview conditions during practice, including time limits and explaining your code aloud.
- Prepare implementation templates: Have mental templates ready for common problem types like graph traversal, dynamic programming, and two-pointer techniques.
For example, when faced with a graph problem in an interview, having this template ready can save valuable time:
function breadthFirstSearch(graph, startNode) {
const queue = [startNode];
const visited = new Set([startNode]);
while (queue.length > 0) {
const current = queue.shift();
// Process current node
for (const neighbor of graph[current]) {
if (!visited.has(neighbor)) {
visited.add(neighbor);
queue.push(neighbor);
}
}
}
}
Mock Interviews and Peer Programming
One of the most effective ways to bridge the recognition-implementation gap for interviews is through mock interviews and pair programming:
- Practice with peers who can evaluate your implementation skills
- Record yourself solving problems to identify hesitation points
- Join coding communities where you can receive feedback on your implementations
- Use platforms that offer mock interview services with experienced interviewers
These practices simulate the pressure of real interviews while providing valuable feedback on your implementation approach.
Learning Resources That Bridge the Gap
Not all learning resources are created equal when it comes to bridging the recognition-implementation gap. Here are some characteristics of effective resources:
What to Look For in Learning Materials
- Implementation-focused exercises: Resources that require you to write code, not just read or watch.
- Progressive complexity: Materials that gradually increase difficulty, allowing you to build implementation confidence.
- Spaced repetition: Platforms that revisit concepts over time to strengthen recall.
- Practical applications: Resources that connect theoretical concepts to real-world implementation scenarios.
- Feedback mechanisms: Tools that provide specific feedback on your implementation, not just correctness.
Recommended Platforms and Approaches
Several platforms specifically address the recognition-implementation gap:
- Interactive coding platforms: Sites that combine tutorials with immediate coding exercises.
- Problem-solving websites: Platforms with algorithmic challenges that require implementation from scratch.
- Project-based learning: Building complete applications forces you to implement concepts in context.
- Code review communities: Groups where you can submit implementations for feedback from experienced developers.
- AI-assisted learning tools: Modern platforms that provide step-by-step guidance while still requiring you to implement solutions.
The most effective learning approach combines these resources with deliberate practice focused on implementation, not just comprehension.
The Role of Mental Models in Implementation
Successful implementation relies heavily on robust mental models that connect abstract concepts to concrete code patterns.
Building Transferable Mental Models
To develop mental models that support implementation:
- Connect algorithms to visual representations: Visualize how data structures change during algorithm execution.
- Identify recurring patterns: Notice how similar implementation techniques appear across different problems.
- Develop a personal algorithm catalog: Categorize problems by their underlying patterns and implementation approaches.
- Practice implementation from different angles: Implement the same algorithm using different approaches (iterative vs. recursive, for example).
For instance, understanding the sliding window technique as a mental model allows you to implement solutions for substring problems, array subarrays, and even some graph problems using similar code structures.
From Concrete to Abstract and Back
Effective implementers can move fluidly between abstract concepts and concrete code:
- They recognize when a problem fits a known pattern
- They recall the implementation template for that pattern
- They adapt the template to the specific problem constraints
- They verify the implementation against their mental model
This bidirectional translation skill develops with practice and deliberate attention to the connections between concepts and code.
The Journey from Recognition to Implementation Mastery
Bridging the recognition-implementation gap is not a one-time achievement but an ongoing process that evolves as you grow as a programmer.
Stages of Implementation Proficiency
Most programmers progress through several stages:
- Recognition without implementation: Understanding solutions when presented but struggling to reproduce them.
- Template-dependent implementation: Successfully implementing solutions that closely match known patterns.
- Adaptive implementation: Modifying known patterns to fit new problem constraints.
- Creative implementation: Combining patterns in novel ways to solve complex problems.
- Intuitive implementation: Implementing solutions fluidly without conscious reference to templates.
Recognizing your current stage can help you focus your practice on the skills needed to advance to the next level.
Measuring Implementation Progress
To track your growth in bridging the recognition-implementation gap:
- Monitor implementation speed for familiar problems
- Track the complexity of problems you can implement without assistance
- Notice when you begin to implement solutions without referring to examples
- Observe how quickly you can adapt known patterns to new contexts
Progress may not be linear, but consistent practice with implementation (not just recognition) will steadily improve your capabilities.
Conclusion: Transforming Recognition into Implementation
The gap between recognizing patterns and implementing them is a fundamental challenge in programming education. By understanding the cognitive mechanisms behind this gap and applying targeted strategies to bridge it, you can transform passive knowledge into active coding skills.
Remember that implementation fluency comes from:
- Active practice, not passive consumption
- Deliberate focus on implementation, not just comprehension
- Building robust mental models that connect concepts to code
- Developing templates that reduce cognitive load during implementation
- Consistent practice with feedback and refinement
Whether you’re preparing for technical interviews at top tech companies or simply trying to become a more effective programmer, bridging the recognition-implementation gap is essential for your growth. The strategies outlined in this guide provide a roadmap for that journey.
The next time you recognize a pattern but struggle to implement it, remember that this gap is normal and surmountable. With deliberate practice focused on implementation, not just recognition, you’ll gradually develop the ability to translate your understanding into working code efficiently and confidently.
Your journey from recognition to implementation mastery is a continuous one. Embrace the challenges, celebrate the progress, and keep coding!