Why Knowing Algorithms Isn’t Teaching You Algorithmic Thinking
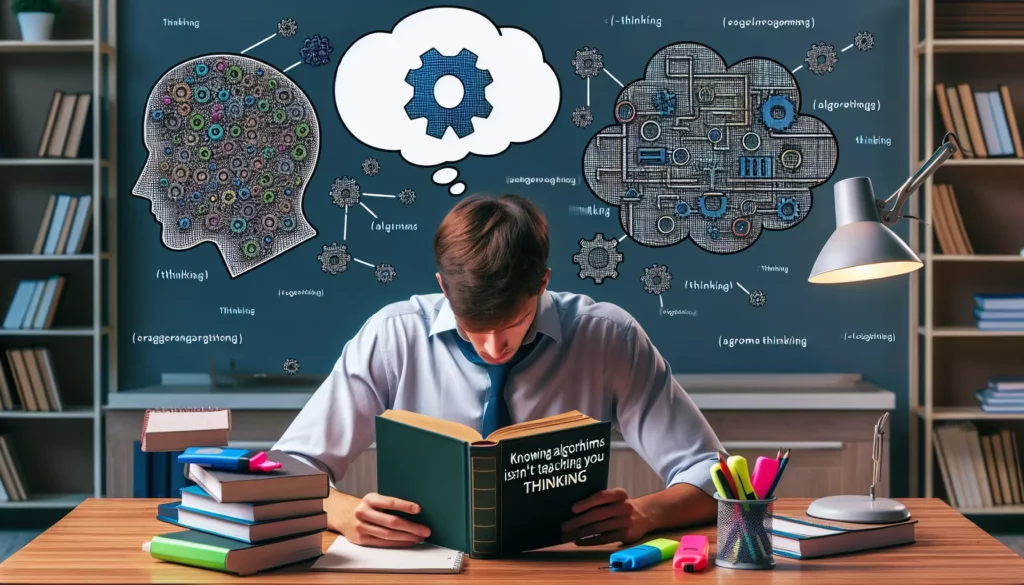
In the world of programming and computer science education, there’s often an overwhelming emphasis on learning algorithms. From sorting algorithms to graph traversals, these fundamental procedures form the backbone of computer science curricula worldwide. Yet, a concerning pattern has emerged: many students can recite algorithms verbatim but struggle when faced with novel problems requiring creative application of algorithmic principles.
This disconnect reveals a critical gap in coding education. Knowing algorithms is not the same as developing algorithmic thinking. While the former involves memorization and implementation of established procedures, the latter represents a deeper cognitive skill that enables programmers to approach unfamiliar challenges with confidence and creativity.
In this article, we’ll explore why simply learning algorithms often fails to cultivate true algorithmic thinking, and what approaches might better develop this essential skill for aspiring programmers and computer scientists.
The Difference Between Knowing Algorithms and Algorithmic Thinking
Before diving deeper, let’s clarify the distinction between these two related but different concepts.
What Does It Mean to “Know Algorithms”?
Knowing algorithms typically involves:
- Memorizing the steps of common algorithms (quicksort, breadth first search, etc.)
- Understanding their time and space complexity
- Being able to implement them in code
- Recognizing when to apply specific algorithms to matching problems
This knowledge is valuable and necessary, but it’s only one piece of the puzzle.
What Is Algorithmic Thinking?
Algorithmic thinking, on the other hand, encompasses:
- The ability to break complex problems into manageable components
- Pattern recognition across seemingly different problems
- Creating novel approaches by adapting or combining existing algorithms
- Evaluating multiple possible solutions to find optimal approaches
- Thinking systematically about efficiency, edge cases, and trade-offs
In essence, algorithmic thinking is a mental framework that enables you to solve problems you’ve never encountered before, not just apply memorized solutions to familiar patterns.
The Limitations of Algorithm-Centric Education
Traditional computer science education often emphasizes learning specific algorithms and data structures. This approach has several limitations that can hinder the development of true algorithmic thinking.
The Template Matching Problem
When students learn algorithms in isolation, they often develop a “template matching” approach to problem solving. They scan a problem looking for clues that match with algorithms they’ve memorized, rather than developing a deeper understanding of the problem itself.
Consider a student who has learned Dijkstra’s algorithm for finding shortest paths in a graph. When faced with a problem involving distances or paths, they might immediately reach for Dijkstra’s algorithm without considering whether:
- The problem actually requires finding shortest paths
- There might be special properties of the graph that allow for more efficient solutions
- A different algorithm might be more appropriate given the constraints
This template-matching approach breaks down when faced with problems that don’t neatly match the algorithms they’ve learned.
The Implementation vs. Design Gap
Many programming courses focus heavily on implementation: writing code that correctly executes an algorithm. While implementation skills are important, they don’t necessarily develop algorithm design skills.
A student might be able to perfectly implement quicksort when told to do so, but struggle to design a novel algorithm for a problem that doesn’t match any standard algorithm they’ve learned. This implementation-focused approach can create the illusion of mastery while leaving significant gaps in problem-solving abilities.
The Missing Context Problem
Algorithms are often taught in isolation, without sufficient context about:
- The problems they were originally designed to solve
- The evolution of the algorithm through various refinements
- The trade-offs and design decisions that shaped them
- The broader principles and patterns they exemplify
Without this context, students miss out on the metacognitive aspects of algorithm design that would help them develop their own algorithmic thinking skills.
The Signs of Underdeveloped Algorithmic Thinking
How can you tell if you or your students have developed algorithmic thinking versus just knowing algorithms? Here are some common signs:
Paralysis When Facing Novel Problems
Those who have memorized algorithms but lack algorithmic thinking often freeze when faced with problems that don’t clearly match their algorithm “catalog.” They struggle to take the first step or break down unfamiliar problems into manageable parts.
Overengineering Simple Problems
Another sign is the tendency to apply complex algorithms to problems that could be solved more simply. For example, using dynamic programming when a greedy approach would suffice, or implementing a sophisticated data structure when a simple array would work better.
Difficulty Explaining Their Approach
Those who’ve merely memorized algorithms often struggle to explain the rationale behind their solution. They can tell you what they did but not why they chose that approach or how they arrived at it.
Inconsistent Problem-Solving Success
Perhaps the most telling sign is inconsistent success with problem-solving. They might solve problems that closely match algorithms they’ve learned but completely fail on problems requiring similar thinking but different implementation.
Why Memorizing Algorithms Isn’t Enough
Let’s examine more deeply why the “learn algorithms” approach falls short of developing true algorithmic thinking.
Real-World Problems Are Messy
In educational settings, algorithm problems are often presented in a clean, well-defined format that maps clearly to a specific algorithm. Real-world problems, however, are messy, ambiguous, and rarely present themselves as “a Dijkstra problem” or “a dynamic programming problem.”
Effective problem solvers need to:
- Extract the relevant information from noisy contexts
- Reformulate ambiguous problems into precise terms
- Recognize when multiple approaches might work and select the most appropriate one
- Adapt algorithms to fit the specific constraints of their situation
These skills aren’t developed by memorizing algorithms in their canonical form.
Innovation Requires Synthesis, Not Just Application
The most valuable solutions often come from combining or adapting multiple algorithms or creating entirely new approaches based on algorithmic principles. This creative synthesis is a hallmark of algorithmic thinking that goes beyond knowing individual algorithms.
Consider how PageRank, the algorithm that powered Google’s early success, wasn’t just an application of a standard graph algorithm but a novel approach that combined ideas from link analysis, probability theory, and linear algebra.
The Landscape of Algorithms Is Vast
There are countless algorithms across different domains of computer science. No one could possibly memorize them all. A more sustainable approach is to develop the thinking skills that allow you to:
- Quickly understand new algorithms when you encounter them
- Adapt existing algorithms to new contexts
- Create new algorithmic approaches when needed
This adaptability comes from understanding the principles behind algorithms, not just the algorithms themselves.
Cultivating True Algorithmic Thinking
If knowing algorithms isn’t enough, how can we develop genuine algorithmic thinking? Here are approaches that foster deeper algorithmic thinking skills:
Focus on Problem Decomposition
Before introducing specific algorithms, focus on the skill of breaking down complex problems into smaller, more manageable parts. This foundational skill underlies all algorithmic thinking.
Practice exercises might include:
- Taking a complex problem and identifying its subproblems
- Determining dependencies between different parts of a problem
- Finding ways to simplify a problem without changing its essential nature
For example, consider this approach to teaching the classic “find the shortest path” problem:
- Start by having students think about how they would manually find a path between two points on a map
- Ask them to identify the decision points and what information they need at each step
- Have them articulate a general strategy before introducing formal algorithms
Teach Algorithm Design, Not Just Implementation
Rather than starting with established algorithms, give students problems and guide them through the process of designing their own solutions. This might be messier and less efficient than teaching canonical algorithms, but it builds stronger thinking skills.
For instance, instead of teaching quicksort directly, you might:
- Present the problem of sorting efficiently
- Have students brainstorm different approaches
- Guide them to discover the divide-and-conquer paradigm
- Help them refine their approach until they arrive at something resembling quicksort
- Only then introduce the standard algorithm and compare it to their solution
Emphasize Multiple Solutions
For any given problem, encourage finding multiple valid solutions with different trade-offs. This helps students understand that algorithmic design involves choices, not just finding “the right answer.”
For example, when solving a searching problem, explore:
- Linear search (simplest but slowest)
- Binary search (faster but requires sorted data)
- Hash-based search (potentially O(1) but with memory trade-offs)
- Tree-based approaches (balanced performance characteristics)
Discussing the trade-offs between these approaches develops a nuanced understanding of algorithm selection.
Teach the “Why” Behind Algorithms
When teaching established algorithms, focus on:
- The problem the algorithm was designed to solve
- The insight or key idea that makes the algorithm work
- How the algorithm evolved from simpler approaches
- The trade-offs inherent in the design
For example, when teaching dynamic programming, emphasize the principle of optimal substructure and overlapping subproblems rather than just the mechanics of filling in a table.
Provide Varied and Progressive Challenges
Design problem sets that gradually build algorithmic thinking by:
- Starting with problems that have clear algorithmic solutions
- Progressing to problems that require adapting known algorithms
- Culminating in problems that require synthesizing multiple algorithmic ideas
- Including open-ended problems with multiple valid approaches
This progression challenges students to move beyond template matching to true algorithmic thinking.
Practical Exercises for Developing Algorithmic Thinking
Let’s explore some specific exercises that can help develop algorithmic thinking beyond just learning algorithms.
Algorithm Derivation Exercises
Instead of presenting an algorithm, present a problem and walk through the process of deriving an algorithm:
- Start with a naive solution
- Identify inefficiencies
- Make incremental improvements
- Analyze each iteration’s performance
- Continue until reaching an optimal or satisfactory solution
For example, deriving an efficient algorithm for finding all prime numbers up to n:
- Start with checking each number individually (O(n²) approach)
- Improve by only checking up to the square root of each number
- Further improve by using the Sieve of Eratosthenes
- Analyze how each improvement changes the time complexity
Algorithm Adaptation Challenges
Present a problem that’s similar to one solvable by a known algorithm, but with a twist that requires adaptation:
For example, “You know how to find the shortest path in a graph with Dijkstra’s algorithm. Now solve a problem where the cost of each edge changes depending on how much total distance you’ve already traveled.”
This forces students to understand the core principles of the algorithm deeply enough to modify it appropriately.
Algorithm Design Competitions
Organize friendly competitions where students design algorithms for specific problems, with evaluation based on:
- Correctness (does it solve the problem?)
- Efficiency (time and space complexity)
- Elegance (clarity and simplicity of approach)
- Robustness (handling edge cases)
The competitive element can motivate deeper engagement with the algorithmic thinking process.
Reverse Engineering Exercises
Present the solution to a problem and ask students to work backward to understand the algorithm and the thinking process behind it:
- Analyze what the algorithm does step by step
- Identify the key insights that make it work
- Consider what alternative approaches might have been considered
- Discuss how one might have arrived at this solution
This helps students understand the thought process behind algorithm design, not just the end result.
Real-World Problem Modeling
Present messy, real-world scenarios and practice the skill of translating them into well-defined algorithmic problems:
- Identify the core computational problem
- Determine what information is relevant
- Define precise inputs and outputs
- Consider constraints and requirements
For example, taking a problem like “optimize delivery routes for a package delivery service” and translating it into a specific graph problem with appropriate constraints.
Case Study: Learning Graph Algorithms
Let’s examine how the traditional approach to teaching graph algorithms differs from an approach focused on developing algorithmic thinking.
Traditional Approach
In a traditional approach, students might learn graph algorithms in this sequence:
- Learn graph representations (adjacency matrix, adjacency list)
- Learn Breadth-First Search (BFS) algorithm
- Learn Depth-First Search (DFS) algorithm
- Learn Dijkstra’s algorithm for shortest paths
- Learn algorithms for minimum spanning trees
- Solve practice problems that clearly map to these algorithms
While this approach certainly teaches the algorithms, it often fails to develop deeper algorithmic thinking about graphs.
Algorithmic Thinking Approach
An approach focused on algorithmic thinking might look like this:
- Explore the concept of graphs through real-world examples (social networks, maps, dependencies)
- Pose exploration problems: “How would you find all possible routes between two cities?” before introducing formal search algorithms
- Guide discovery of the key insights behind BFS and DFS through guided problem-solving
- Present variations of graph problems that require adapting the basic algorithms
- Discuss the evolution of graph algorithms and how they build on each other
- Challenge students with problems that don’t clearly map to a single algorithm
Example Problem Progression
A thoughtful progression of problems might look like:
- Basic connectivity: “Is there a path between nodes A and B?” (introduces search)
- Shortest path in unweighted graphs: “What’s the minimum number of steps between A and B?” (naturally leads to BFS)
- Shortest path with weights: “What’s the lowest-cost path between A and B?” (builds toward Dijkstra’s)
- Constrained paths: “Find the shortest path that visits nodes C and D” (requires adapting basic algorithms)
- Dynamic graphs: “How does the shortest path change if edge weights change over time?” (pushes beyond standard algorithms)
This progression gradually builds algorithmic thinking skills while still teaching the fundamental algorithms.
The Role of Pattern Recognition in Algorithmic Thinking
One crucial aspect of algorithmic thinking is pattern recognition—the ability to see similarities between different problems and apply appropriate approaches. This skill goes beyond knowing specific algorithms to recognizing classes of problems.
Common Algorithmic Patterns
Effective algorithmic thinkers recognize patterns like:
- Divide and conquer: Breaking a problem into smaller, similar subproblems
- Dynamic programming: Solving a problem by combining solutions to overlapping subproblems
- Greedy approaches: Making locally optimal choices at each step
- Graph traversal: Exploring connected structures systematically
- Sliding window: Maintaining a view into a subset of data that moves through the dataset
- Two-pointer techniques: Using multiple pointers to traverse data efficiently
Understanding these patterns allows programmers to approach new problems by considering which pattern might apply, rather than which specific algorithm to use.
Developing Pattern Recognition Skills
To develop this aspect of algorithmic thinking:
- Categorize problems by the patterns they exemplify, not just the algorithms they use
- Compare and contrast problems that seem different but share underlying patterns
- Practice identifying which algorithmic patterns might apply to a new problem
- Study problem transformations—how one problem can be reframed as another
For example, recognizing that finding the longest increasing subsequence, calculating edit distance, and determining optimal matrix chain multiplication all share the pattern of optimal substructure that makes them amenable to dynamic programming approaches.
Beyond Individual Algorithms: Systems Thinking
Advanced algorithmic thinking extends beyond individual algorithms to thinking about systems of algorithms and their interactions. This systems-level thinking is crucial for solving complex real-world problems.
Algorithmic Components and Composition
Complex systems often require multiple algorithms working together:
- A search engine combines text processing, indexing, ranking, and retrieval algorithms
- A recommendation system might use clustering, collaborative filtering, and personalization algorithms
- A compiler employs lexical analysis, parsing, optimization, and code generation algorithms
Understanding how to compose algorithms into systems is an advanced form of algorithmic thinking.
Performance and Trade-offs at Scale
Systems-level algorithmic thinking considers how algorithms perform at scale and make appropriate trade-offs:
- An algorithm that’s theoretically efficient might perform poorly due to hardware constraints
- Sometimes approximation algorithms provide better real-world performance than exact algorithms
- Distributed algorithms introduce new considerations beyond traditional complexity analysis
This level of thinking prepares programmers for real-world engineering challenges that go beyond textbook algorithm problems.
The Connection Between Algorithmic Thinking and Problem-Solving Mindset
Algorithmic thinking isn’t just a technical skill—it’s connected to broader problem-solving approaches and mindsets.
Growth Mindset in Algorithm Learning
Developing algorithmic thinking requires embracing challenges and learning from failures:
- Viewing difficult problems as opportunities to grow, not threats to avoid
- Understanding that algorithmic skills develop through effort and practice
- Being willing to try multiple approaches when stuck
- Learning from unsuccessful solution attempts
This growth mindset is essential for continuing to develop as an algorithmic thinker.
Metacognition in Problem Solving
Strong algorithmic thinkers actively monitor their own problem-solving process:
- Reflecting on which approaches tend to work for which types of problems
- Recognizing when they’re stuck in unproductive thinking patterns
- Consciously varying their approach when initial attempts fail
- Analyzing successful solutions to extract generalizable principles
This metacognitive awareness accelerates the development of algorithmic thinking skills.
Practical Application: Bridging Learning and Practice
How can learners bridge the gap between learning algorithms and developing algorithmic thinking in practical settings?
Deliberate Practice Strategies
Effective practice for algorithmic thinking goes beyond solving lots of problems:
- Varied problem selection: Tackle problems that require different types of algorithmic thinking
- Incremental challenge: Gradually increase difficulty rather than jumping to very hard problems
- Solution comparison: Study multiple solutions to the same problem to understand different approaches
- Time constraints: Practice both with and without time pressure to develop different aspects of thinking
- Reflection: After solving a problem, reflect on what made it challenging and what insights were key
Collaborative Learning Approaches
Algorithmic thinking develops well in collaborative environments:
- Pair programming on algorithm problems to verbalize thinking processes
- Code reviews focused on algorithmic approach, not just correctness
- Discussion groups that analyze and compare different solutions
- Teaching others, which requires deep understanding of algorithmic principles
These collaborative approaches expose learners to diverse thinking patterns and approaches.
The Future of Algorithmic Thinking Education
As we look to the future, how might education evolve to better develop algorithmic thinking?
Integration with AI and Machine Learning
The rise of AI presents both challenges and opportunities:
- AI systems can now solve many standard algorithm problems, shifting focus to higher-level thinking
- Understanding algorithmic thinking becomes crucial for effectively working with AI tools
- New types of algorithms related to machine learning require different thinking approaches
- AI-assisted teaching tools can provide personalized guidance for developing algorithmic thinking
Interdisciplinary Approaches
Algorithmic thinking increasingly crosses disciplinary boundaries:
- Computational biology applies algorithmic thinking to biological systems
- Computational social science uses algorithms to understand human behavior
- Algorithmic approaches in arts and humanities offer new ways to analyze cultural artifacts
- Business analytics applies algorithmic thinking to organizational problems
Education that connects algorithmic thinking to diverse domains may better prepare students for future applications.
Conclusion: From Algorithm Knowledge to Algorithmic Thinking
While knowing specific algorithms remains valuable, the true power comes from developing algorithmic thinking as a flexible, creative problem-solving approach. This transition from knowledge to thinking represents a fundamental shift in how we approach coding education.
Algorithmic thinking enables programmers to:
- Approach unfamiliar problems with confidence
- Create novel solutions rather than just applying known algorithms
- Adapt to changing technological landscapes
- Collaborate effectively on complex computational challenges
By focusing educational efforts on developing these thinking skills—not just teaching algorithm catalogs—we can better prepare the next generation of problem solvers for the challenges they’ll face.
The journey from knowing algorithms to thinking algorithmically isn’t easy or quick. It requires deliberate practice, mindful reflection, and embracing challenges outside your comfort zone. But this transition is what separates programmers who can implement solutions from those who can create them—a distinction that makes all the difference in a world of increasingly complex computational challenges.
Remember that algorithmic thinking, like any complex cognitive skill, develops gradually through practice and reflection. Be patient with yourself or your students as you work to cultivate this valuable way of thinking. The rewards—in problem-solving capability, professional opportunities, and intellectual satisfaction—are well worth the effort.