Why Solving Math Problems Isn’t the Same as Solving Programming Problems
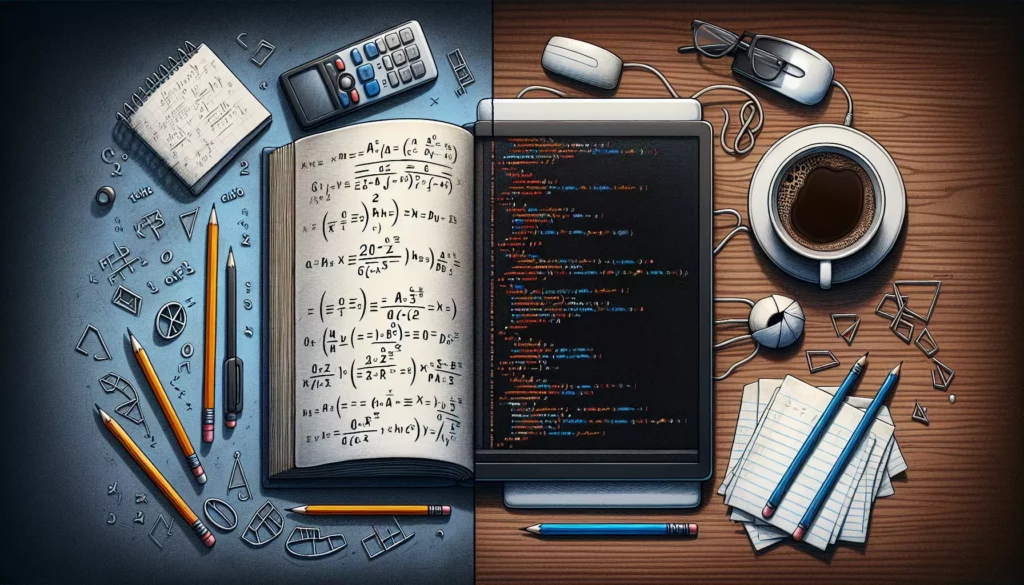
Many newcomers to programming arrive with the assumption that coding is essentially applied mathematics. After all, both fields involve problem-solving, logical thinking, and systematic approaches. While there’s certainly overlap between mathematical problem-solving and programming problem-solving, treating them as identical processes can lead to frustration and inefficiency in learning to code.
In this article, we’ll explore the key differences between solving math problems and solving programming problems, and why understanding these differences is crucial for becoming a proficient programmer.
The Apparent Similarities
Before diving into the differences, let’s acknowledge why these two problem-solving domains seem so similar:
- Both require logical thinking and structured approaches
- Both involve breaking down complex problems into simpler components
- Both use abstract concepts and symbolic representations
- Both benefit from pattern recognition
These similarities often lead educators to use mathematical aptitude as a predictor for programming success. However, this correlation isn’t as strong as many assume, and treating programming as “just math with a computer” can create unnecessary barriers for learners.
Fundamental Differences Between Math and Programming Problems
1. State and Mutation vs. Pure Functions
In mathematics, functions are typically pure: given the same input, they always produce the same output without side effects. The equation y = 2x + 3
will always give the same y
for a specific x
.
Programming, however, often deals with state and mutation. Consider this simple JavaScript counter:
let counter = 0;
function incrementCounter() {
counter++;
return counter;
}
console.log(incrementCounter()); // 1
console.log(incrementCounter()); // 2
The function incrementCounter()
doesn’t just compute a value; it changes the state of the program. Calling it multiple times produces different results, even with identical inputs (in this case, no inputs).
This state-based thinking is fundamental to programming but mostly absent in mathematical problem-solving.
2. Implementation Details Matter
In mathematics, once you’ve found a solution, you’re done. The “how” of arriving at the answer rarely matters as long as the solution is correct.
In programming, implementation details are crucial. Consider two functions that find the maximum value in an array:
// Solution 1: O(n) time complexity
function findMax1(array) {
let max = array[0];
for (let i = 1; i < array.length; i++) {
if (array[i] > max) {
max = array[i];
}
}
return max;
}
// Solution 2: O(n²) time complexity
function findMax2(array) {
for (let i = 0; i < array.length; i++) {
let isMax = true;
for (let j = 0; j < array.length; j++) {
if (array[j] > array[i]) {
isMax = false;
break;
}
}
if (isMax) return array[i];
}
}
Both functions produce the correct answer, but the first is dramatically more efficient. In programming, the “how” matters just as much as the “what.”
3. The Environment Matters
Mathematical problems exist in an idealized, abstract space. Programming problems exist within specific environments with constraints and peculiarities.
A program that works perfectly on your machine might fail spectacularly on another due to differences in operating systems, available memory, processor architecture, or installed dependencies.
Consider this Python code that reads a file:
def read_config():
with open('config.txt', 'r') as file:
return file.read()
# This might work on your development machine,
# but fail in production if the file doesn't exist
# or the program doesn't have permission to read it
Programmers must consider the environment their code will run in, which has no parallel in mathematical problem-solving.
4. Precision vs. Approximation
Mathematics typically demands exact answers. Programming often requires balancing precision with practical constraints.
Consider calculating π. In mathematics, π is a precise value. In programming, we might use:
const pi = 3.14159;
Or more precisely:
const pi = Math.PI; // In JavaScript, approximately 3.141592653589793
But even this is an approximation due to the limitations of floating-point representation in computers. Programmers must constantly make decisions about acceptable levels of precision based on the context.
5. Error Handling
Mathematical problems typically assume ideal conditions. Programming requires anticipating and handling errors.
Consider a function to divide two numbers:
// Mathematical approach (incomplete as a program)
function divide(a, b) {
return a / b;
}
// Programming approach
function divide(a, b) {
if (b === 0) {
throw new Error("Division by zero is not allowed");
}
return a / b;
}
Error handling is a substantial part of programming that has no direct equivalent in mathematical problem-solving.
The Different Mental Models Required
Computational Thinking vs. Mathematical Thinking
Programming requires computational thinking, which includes:
- Algorithmic thinking: Defining clear steps to solve a problem
- Decomposition: Breaking problems into manageable parts
- Pattern recognition: Identifying similarities among problems
- Abstraction: Focusing on relevant details while ignoring others
While mathematical thinking shares some of these aspects, it places different emphasis on them. Mathematical thinking tends to focus more on proof, axiomatic systems, and formal logic.
For example, consider sorting a list of numbers:
- A mathematician might focus on proving that a sorting algorithm is correct and analyzing its theoretical properties.
- A programmer focuses on implementing the algorithm efficiently, handling edge cases, and ensuring it works within memory constraints.
Iterative Development vs. Complete Solutions
Mathematical problem-solving often aims for complete, elegant solutions. Programming embraces iterative development:
- Create a minimal working solution
- Test it with real data
- Identify issues or improvements
- Refine the solution
- Repeat
This iterative approach can feel uncomfortable to those trained in mathematical problem-solving, where the goal is typically to arrive at a complete, correct solution before moving on.
Programming Problems Unique Challenges
1. Debugging
Debugging has no real equivalent in mathematical problem-solving. When solving a math problem, you either get the right answer or you don’t. In programming, your solution might work for most cases but fail mysteriously in others.
Consider this JavaScript function that’s supposed to check if a number is even:
function isEven(num) {
return num % 2 == 0;
}
console.log(isEven(2)); // true
console.log(isEven(3)); // false
console.log(isEven("2")); // true (Wait, what?)
The function unexpectedly returns true
for the string "2"
due to JavaScript’s type coercion. Debugging requires understanding not just the algorithm but also the language’s behavior.
2. Dealing with Legacy Code
Programmers rarely write code from scratch. They often need to understand, modify, and extend existing code written by others.
This has no real parallel in mathematical problem-solving, where you typically start with a clean slate and work toward a solution.
3. Balancing Multiple Constraints
Programming problems often involve balancing competing constraints:
- Performance vs. readability
- Memory usage vs. processing speed
- Simplicity vs. flexibility
- Development time vs. code quality
Consider implementing a search function:
// Option 1: Simple linear search - O(n) time complexity
function linearSearch(array, target) {
for (let i = 0; i < array.length; i++) {
if (array[i] === target) return i;
}
return -1;
}
// Option 2: Binary search (requires sorted array) - O(log n) time complexity
function binarySearch(array, target) {
let left = 0;
let right = array.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (array[mid] === target) return mid;
if (array[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
The binary search is faster but requires a sorted array and is more complex to implement. The “best” solution depends on the specific context and constraints.
When Mathematical Problem-Solving Skills Do Help in Programming
Despite the differences, mathematical problem-solving skills can certainly benefit programmers in specific contexts:
1. Algorithm Design and Analysis
Understanding computational complexity (Big O notation) and algorithm efficiency draws heavily on mathematical concepts. When designing algorithms for sorting, searching, or graph traversal, mathematical thinking is invaluable.
2. Specific Domains
Certain programming domains rely heavily on mathematical concepts:
- Computer graphics and game development (linear algebra, geometry)
- Machine learning and AI (statistics, calculus, linear algebra)
- Cryptography (number theory)
- Financial software (probability, statistics)
3. Formal Verification
Proving program correctness uses techniques from mathematical logic. In critical systems where bugs could be catastrophic (aerospace, medical devices), formal verification methods draw heavily on mathematical reasoning.
Learning to Program: A Different Approach Than Learning Math
Understanding that programming is not just “applied math” has important implications for how we learn and teach coding:
1. Embrace Trial and Error
Unlike math problems where you’re expected to think through the entire solution before writing anything down, programming benefits from experimentation. Write code, run it, see what happens, adjust, and repeat.
Consider learning how string manipulation works:
// Try in a JavaScript console or Node.js REPL
let name = "John Smith";
// Experiment with different methods
console.log(name.toUpperCase());
console.log(name.toLowerCase());
console.log(name.split(" "));
console.log(name.replace("John", "Jane"));
console.log(name.includes("Smith"));
This hands-on experimentation is often more effective than trying to memorize all string methods beforehand.
2. Build Projects, Not Just Exercises
Math education often focuses on isolated problems. Programming education is most effective when centered around projects that:
- Integrate multiple concepts
- Require understanding the broader context
- Involve making design decisions
- Necessitate debugging real issues
A simple project like building a to-do list application teaches more about programming than dozens of isolated exercises on loops and conditionals.
3. Focus on Reading Code, Not Just Writing It
In mathematics, you primarily learn by solving problems. In programming, reading and understanding existing code is equally important.
Experienced programmers spend more time reading code than writing it. They learn new patterns, techniques, and approaches by studying others’ solutions.
4. Understand That “Correct” Has Many Dimensions
In mathematics, a solution is typically either correct or incorrect. In programming, correctness has multiple dimensions:
- Functional correctness (does it produce the right output?)
- Performance (does it run efficiently?)
- Readability (can other developers understand it?)
- Maintainability (can it be easily modified or extended?)
- Robustness (does it handle errors and edge cases?)
Learning to balance these aspects is a key part of becoming a proficient programmer.
Common Pitfalls When Applying Mathematical Thinking to Programming
When people with strong mathematical backgrounds learn to program, they often encounter specific challenges:
1. Over-Optimization Too Early
Mathematicians often seek the most elegant, efficient solution from the start. In programming, this can lead to premature optimization—making code complex for performance gains that might be unnecessary.
Consider this example:
// Straightforward solution
function sumArray(arr) {
let sum = 0;
for (let i = 0; i < arr.length; i++) {
sum += arr[i];
}
return sum;
}
// "Optimized" but less readable solution
function sumArray(arr) {
return arr.length ?
arr.reduce((a, b) => a + b) :
0;
}
The second solution might be marginally faster in some contexts, but the readability trade-off is rarely worth it for such a simple function.
2. Reluctance to Use Libraries
In mathematics, solving problems yourself is valued. In programming, reinventing the wheel is often counterproductive. Experienced programmers leverage existing libraries and frameworks to solve common problems.
3. Perfectionism
Mathematical training often emphasizes finding perfect solutions. Programming often requires accepting “good enough” solutions that can be improved iteratively.
The software development mantra “Make it work, make it right, make it fast”—in that order—can be difficult for mathematically-trained thinkers who want to optimize from the beginning.
4. Underestimating the Importance of Testing
In mathematics, once you’ve proven a solution correct, it’s correct forever. In programming, code that works today might break tomorrow due to changes in dependencies, environment, or requirements.
Testing is not just verifying correctness; it’s creating a safety net for future changes:
// A simple function
function add(a, b) {
return a + b;
}
// Tests for the function
test('add correctly sums two positive numbers', () => {
expect(add(2, 3)).toBe(5);
});
test('add correctly handles negative numbers', () => {
expect(add(-2, 3)).toBe(1);
expect(add(2, -3)).toBe(-1);
expect(add(-2, -3)).toBe(-5);
});
test('add correctly handles zero', () => {
expect(add(0, 3)).toBe(3);
expect(add(2, 0)).toBe(2);
});
This level of verification might seem excessive for such a simple function, but it becomes invaluable as systems grow more complex.
The Different Skills That Make Great Programmers
While mathematical ability can certainly help in programming, many other skills are equally or more important:
1. Communication Skills
Programming is rarely a solitary activity. Great programmers can:
- Explain technical concepts clearly
- Document their code effectively
- Collaborate with team members
- Understand and translate user requirements
2. Attention to Detail
A missing semicolon, an off-by-one error, or a misnamed variable can cause entire systems to fail. While mathematics also requires precision, the consequences of small errors in programming are often more immediate and visible.
3. Systems Thinking
Understanding how components interact in complex systems is crucial for programming. This includes:
- Recognizing dependencies between modules
- Anticipating how changes in one area affect others
- Designing interfaces between components
- Managing state across a system
4. Persistence and Problem-Solving
Debugging can require methodical investigation and persistence. The ability to approach problems from multiple angles and not give up when solutions aren’t immediately apparent is invaluable.
5. Adaptability
The programming field evolves rapidly. Technologies that are standard today may be obsolete in five years. Great programmers are adaptable and committed to continuous learning.
Finding the Right Balance
The most effective approach to programming combines elements of both mathematical and programming-specific thinking:
1. Use Mathematical Thinking When Appropriate
When designing algorithms, analyzing complexity, or working in mathematically-intensive domains, leverage mathematical thinking. It provides powerful tools for certain classes of problems.
2. Embrace Programming-Specific Approaches
For issues of state management, error handling, and system design, use approaches specific to programming rather than trying to force mathematical frameworks onto them.
3. Learn Multiple Paradigms
Different programming paradigms incorporate different amounts of mathematical thinking:
- Functional programming (Haskell, Clojure) is closer to mathematical thinking with its emphasis on pure functions and immutability
- Object-oriented programming (Java, C#) emphasizes state encapsulation and behavior
- Procedural programming (C, early Python) focuses on sequences of operations
Learning multiple paradigms expands your problem-solving toolkit.
4. Focus on the Problem Domain
The most important skill in programming is understanding the problem you’re trying to solve. This often requires domain knowledge beyond both mathematics and programming.
For example, building a financial application requires understanding financial concepts; building a healthcare system requires healthcare knowledge.
Conclusion
While mathematical problem-solving and programming problem-solving share important characteristics, they are distinct activities requiring different approaches and mindsets. Understanding these differences can help:
- Newcomers to programming avoid common pitfalls and frustrations
- Educators design more effective programming curricula
- Experienced mathematicians transition more smoothly into programming roles
- Teams better leverage the diverse problem-solving styles of their members
The best programmers recognize when to apply mathematical thinking and when to embrace programming-specific approaches. They don’t try to force one paradigm onto problems better suited for another.
By understanding that programming is not just “math on a computer,” we can approach coding education and practice in ways that acknowledge its unique challenges and opportunities. This recognition opens the door to more effective learning, teaching, and problem-solving in the programming domain.
Whether you’re a mathematician exploring programming, a programmer looking to strengthen your mathematical foundations, or a beginner trying to understand the relationship between these fields, recognizing both the connections and the distinctions will make you a more effective problem-solver in both domains.